21 Create Click Event Javascript
JavaScript event handling is the basis of all client-side applications. When an event occurs on a target element, e.g. a button click, mouse move, form submit etc, a handler function is executed.... To add an event handler to an HTML element, you can use the addEventListener () method of the element object. The addEventListener () method attaches an event handler to the specified HTML element without overwriting the existing event handlers. To attach a click event handler to the above button, you can use the following example: As you can ...
Create A Public Rfp Rfq Or Other Event Scout Rfp
May 20, 2020 - allow attaching a handler for an event that is defined in an element other than the one adding the handler. ... Which of the following is the correct way to execute a JavaScript when a button element is clicked ?
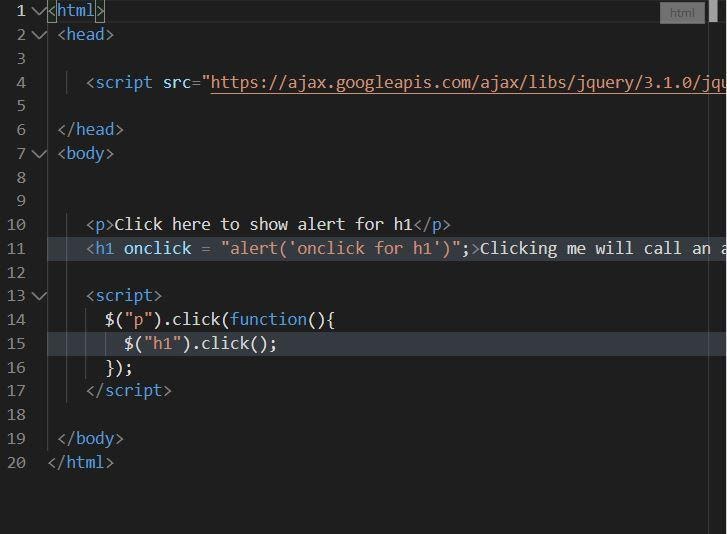
Create click event javascript. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The JavaScript onclick event executes a function when a user clicks a button or another web element. This method is used both inline in an HTML document and in a JavaScript document. When you are coding in JavaScript, it's common to want to run code when a user interacts with the web page. JavaScript Onclick Event Explained. The onclick event in JavaScript lets you as a programmer execute a function when an element is clicked. ... We accomplish this by creating thousands of videos, articles, and interactive coding lessons - all freely available to the public. We also have thousands of freeCodeCamp study groups around the world.
How to create a custom event in JavaScript. Custom events can be created in two ways: Using the Event constructor. Using the CustomEvent constructor. Custom events can also be created using document.createEvent, but most of the methods exposed by the object returned from the function have been deprecated. How to bind callback functions to click events in D3.js-based JavaScript charts. How to bind callback functions to click events in D3.js-based JavaScript charts. 🏿 Black Lives Matter. Please consider donating to Black Girls Code today. ... Create annotation on click event. JavaScript's interaction with HTML is handled through events that occur when the user or the browser manipulates a page. When the page loads, it is called an event. When the user clicks a button, that click too is an event. Other examples include events like pressing any key, closing a window, resizing a window, etc.
Set onClick () Event By Using HTML DOM Object Up to now, we have used a simple element attribute way for the onClick () event. We can also use HTML DOM in order to set a function or code for a specific HTML element for the click event. We will set the elements DOM object onclick attribute. $("button").on("click mouseover", function() { alert("Hi jQuery events"); }); 3. How to Create an Event Listener in JavaScript with addEventListener() Using native JavaScript, we can listen to all the events defined in MDN's Event Reference, including touch events. As this doesn't require the use of a third-party library, it's the most ... Javascript- Onclick Event Handler. The Onclick event handler in Javascript is an event handler that executes when a user clicks on a web element. This web element can be anything, such as a button, an image, a header, or any other various HTML element. Thus, when the element is clicked that has a onclick event handler attached to it, the ...
If you click a link, you will be taken to the link’s target. If you press the down arrow, the browser will scroll the page down. If you right-click, you’ll get a context menu. And so on. For most types of events, the JavaScript event handlers are called before the default behavior takes place. The createEvent () method creates an event object. The event can be of any legal event type, and must be initialized before use. May 17, 2017 - The other day on Twitter, Oliver Williams asked me: How would I write a vanilla equivalent of jQuery .click() (with no arguments) to trigger a click event even when a real click hasn’t occurred? If you Google this, you might get excited to discover that vanilla JavaScript has a click() method.
So far we've attached an onclick event handler to each static item on the page, which is easy. When we click on them now, they will run the dynamicEvent function and the text will change to 'Dynamic event success.'. Dynamically creating elements Mouse button. Click-related events always have the button property, which allows to get the exact mouse button.. We usually don't use it for click and contextmenu events, because the former happens only on left-click, and the latter - only on right-click.. From the other hand, mousedown and mouseup handlers may need event.button, because these events trigger on any button, so button allows ... This article demonstrates how to create and dispatch DOM events. Such events are commonly called synthetic events, as opposed to the events fired by the browser itself.
A click is a compound event that is comprised of combined mousedown and mouseup events, which fire when the mouse button is pressed down or lifted, respectively.. Using mouseenter and mouseleave in tandem recreates a hover effect that lasts as long as a mouse pointer is on the element.. Form Events. Form events are actions that pertain to forms, such as input elements being selected or ... Approach: Whenever you want to perform a click event programmatically, at your specific condition, just use JavaScript in-built click () function by DOM object. For example: Example 1: We want to click the input file element automatically (programmatically). When the user clicks one button which is not the 'file upload' button of the input ... how to create a click event in javascript; javascript event click; how to make a function when you click a button in javascript; oclick event; attach onclick event in javascript; onclick event with css; input button on click js event; how to assign an already made function to an event with javascript; onclick jscript; javascript html button pressed
After an event object is created, we should "run" it on an element using the call elem.dispatchEvent (event). Then handlers react on it as if it were a regular browser event. If the event was created with the bubbles flag, then it bubbles. In the example below the click event is initiated in JavaScript. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Simple Click Events. This example demonstrates the use of event listeners. It. listens for the click event on a marker to zoom the map when the marker is clicked. listens for the center_changed event to pan the map back to the marker after 3 seconds. Read the documentation.
Event handlers typically have names that begin with on, for example, the event handler for the click event is onclick. To assign an event handler to an event associated with an HTML element, you can use an HTML attribute with the name of the event handler. For example, to execute some code when a button is clicked, you use the following: Error: Timeout of 2000ms exceeded. For async tests and hooks, ensure "done()" is called; if returning a Promise, ensure it resolves · How do you wait for 5 seconds in JavaScript · UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside ... Using onclick to create a dropdown button: // Get the button, and when the user clicks on it, execute myFunction document.getElementById("myBtn").onclick = function() {myFunction ()}; /* myFunction toggles between adding and removing the show class, which is used to hide and show the dropdown content */
Apr 24, 2020 - Summary: in this tutorial, you will learn about the JavaScript events, event models, and how to handle events. ... An event is an action that occurs in the web browser, which the web browser feedbacks to you so that you can respond to it. For example, when users click a button on a webpage, ... The onclick event executes a certain functionality when a button is clicked. This could be when a user submits a form, when you change certain content on the web page, and other things like that. You place the JavaScript function you want to execute inside the opening tag of the button. Creating custom events. Events can be created with the Event constructor as follows: const event = new Event('build'); elem.addEventListener('build', function (e) { }, false); elem.dispatchEvent( event); Copy to Clipboard. The above code example uses the EventTarget.dispatchEvent () method. This constructor is supported in most modern browsers ...
Read How to Create One-Time Events in JavaScript and learn with SitePoint. Our web development and design tutorials, courses, and books will teach you HTML, CSS, JavaScript, PHP, Python, and more. Simulating an event is similar to creating a custom event. To simulate a mouse event. we gonna have to create MouseEvent using document.createEvent(). Then using initMouseEvent(), we've to set up the mouse event that is going to occur. Then dispatched the mouse event on the element on which you'd like to simulate an event. Aug 20, 2011 - Description: Bind an event handler to the "click" JavaScript event, or trigger that event on an element.
HTMLElement.click () The HTMLElement.click () method simulates a mouse click on an element. When click () is used with supported elements (such as an <input>), it fires the element's click event. This event then bubbles up to elements higher in the document tree (or event chain) and fires their click events. Aug 31, 2019 - Method 2: Creating a new CustomEvent: The CustomEvent constructor is used to create a new event to be used on any element. The CustomEvent interface handles the events initialized by an application for any purpose. The ‘click’ event can be passed to the constructor of CustomEvent to create ... The dispatchEvent delivers an Event at the specified EventTarget, synchronously executing the affected EventListeners in an appropriate order. Unlike native events that are fired by the DOM and call event handlers asynchronously with the help of the event loop, the dispatchEvent execute event handlers synchronously.
The 'click' event can be passed to the constructor of CustomEvent to create a click event. This created Event has various properties which can be accessed to customize the event. The element to be clicked on is first selected. The dispatchEvent () method is used on this element to fire the click event. To generate an event programmatically, you follow these steps: First, create a new Event object using Event constructor. Then, trigger the event using element.dispatchEvent () method. Dec 17, 2015 - A string containing a JavaScript event type, such as click or submit.
An element receives a click event when a pointing device button (such as a mouse's primary mouse button) is both pressed and released while the pointer is located inside the element. Given we now know what a click event is, we can think of a click event handler as a tool that allows us to do something when a click event occurs. A JavaScript can be executed when an event occurs, the user clicks on any HTML tag elements. The onclick and alert events are most frequently used event type in the JavaScript for web pages. If any anonymous function to the HTML elements the onclick attribute will attach event to this element.
Onclick Is Not A Reserved Word So How Does Javascript Know
Move Javascript Code To Different Event
How To Call Javascript Function In Html Javatpoint
Mouse Click Amp Keyboard Event Action Class In Selenium Webdriver
Passing Onclick Event Into Template Literals Without Global
Event Listener Click And Mouseover Stack Overflow
Jquery Bind Onclick Event To Dynamically Created Elements
Call Multiple Javascript Functions In Onclick Event
How To Create An Image Element Dynamically Using Javascript
Javascript Looking To Create A Tooltip For A Mou Esri
How To Create A Simple Crud Application Using Only Javascript
Github Davidfig Clicked Javascript Create Click Event For
Google Tag Manager Button Click Tracking Guide Loves Data
How To Change Text Onclick Event Javascript Errorsea
Javascript Addeventlistener With Examples Geeksforgeeks
Test Automation With Selenium Click Button Method Examples
Can You Predict Event Target For Click Events In Each
Onclick Function In Jquery Explained With Examples Upgrad Blog
0 Response to "21 Create Click Event Javascript"
Post a Comment