20 Javascript Check If First Letter Is Uppercase
JavaScript Basic: Exercise-101 with Solution. Write a JavaScript program to check whether a given string contains only Latin letters and no two uppercase and no two lowercase letters are in adjacent positions. I am learning javascript and Regex and trying to validate a input if the first letter in the string is in uppercase. my current Regex is : var patt1=/[A-Z]{4}$/; but this is validating the string if all the four letters are in uppercase not the first letter only. Any idea how to make it work.
How To Capitalize The First Letter Of Each Word In Javascript
JavaScript Program to Check First Character of Entered String is Uppercase or not Using Regular Expression. Write a JavaScript program to check first character of entered string is uppercase or not using regular expression. Source Code
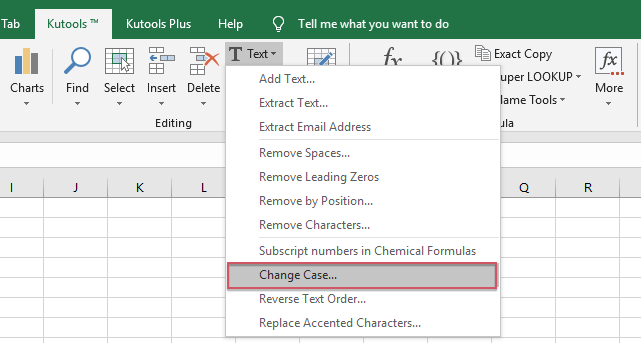
Javascript check if first letter is uppercase. One of the most frequently used operations with strings is to convert the first character of a string to uppercase, and leave the rest of string as it is. There are many ways to capitalize the first letter of a string in JavaScript. The easiest way to do is to use two JavaScript functions together: charAt () and slice (). JavaScript doesn't have any built-in methods to uppercase the first letter of string but we can do it by using the slice() and charAt() methods. First, we need to access the first character of a string by using the charAt() method then convert it to uppercase, and concat with the string by slicing the first character. There are number of ways to capitalize the first letter of the string in JavaScript. Following are the ways: toUpperCase (): This function applies on a string and change the all letters to uppercase. Syntax: string.toUpperCase () Return Value: This function returns the capitalized string. slice (): This function applies on a string and slice it ...
29/11/2011 · This variant returns true if the initial is any capital letter, and only if it's a capital letter: function initialIsCapital ( word ) { return word [0] !== word [0].toLowerCase (); } Use .charAt (0) instead of [0] if you need IE8 support. Which is faster varies between browsers. Traverse the string character by character from start to end. Check the ASCII value of each character for the following conditions: If the ASCII value lies in the range of [65, 90], then it is an uppercase letter.; If the ASCII value lies in the range of [97, 122], then it is a lowercase letter.; If the ASCII value lies in the range of [48, 57], then it is a number. How to check if every first letter of words in a data item is set to uppercase and the rest set to lowercase. ( Variable is the length of words can change in the data item) Data item = "joHn Michael Ryan" = False. Data item = "John Micheal Ryan John" = True. S.
How to uppercase the first letter of a string in JavaScript JavaScript offers many ways to capitalize a string to make the first character uppercase. Learn the various ways, and also find out which one you should use, using plain JavaScript. Published May 09, 2018, Last Updated May 27, 2019 Javascript function to check if a field input contains letters and numbers only. To get a string contains only letters and numbers (i.e. a-z, A-Z or 0-9) we use a regular expression /^ [0-9a-zA-Z]+$/ which allows only letters and numbers. Next the match () method of string object is used to match the said regular expression against the input value. JavaScript: Capitalize the first letter In this tutorial, we shall look at capitalizing the first letter of a string in JavaScript followed by learning how we can capitalize the first letter of each word in a string. Table of Contents. Capitalize the first letter of a string; Capitalize the first letter of each word in a string; Other Related ...
The toUpperCase() method returns the value of the string converted to uppercase. This method does not affect the value of the string itself since JavaScript strings are immutable. This method does not affect the value of the string itself since JavaScript strings are immutable. To capitalize the first letter in a string is easy if you undertake some steps. First of all you should get the first letter of the string by setting the charAt () method at 0 index: Next, you should make the first letter uppercase with the toUpperCase () method: Then, you should get the remainder of the string with the help of the slice () method. What about the input string "STRING123, TEST"? Well, our program will output false for this input value. That is because string contains numbers, space and a comma for which the isUpperCase method returns false. To fix that, before checking if the character is uppercase, we need to first check if it is a letter as given below.
Capitalize the First Letter of a String using simple Javascript: To capitalize the first letter of a string use the following JavaScript function. The code takes out the first letter of string & converts it into the capital letter (uppercase letter) using toUpperCase method in JavaScript & then append it to the remaining string (uses substr ... first letter UPPERCASE But the same approach doesn't work for INPUT fields so we're back to using JavaScript. Here's a simple example where we piggy-back off our previous forceKeyPressUppercase method and apply it conditionally according to the position of the cursor in the INPUT field: Javascript Front End Technology Object Oriented Programming. To test if a letter in a string is uppercase or lowercase using javascript, you can simply convert the char to its respective case and see the result.
Concatenate the first letter capitalized with the remainder of the string and return the result; 1. Get the First Letter of the String. You should use the charAt () method, at index 0, to select the first character of the string. var string = "freeCodecamp"; string.charAt (0); // Returns "f". NOTE: charAt is preferable than using [ ] ( bracket ... Secondly to check if first character of each word is uppercase, use isupper () function. Following code lists words starting with capital letters. s1="This is not true that Delhi is the hottest or coldest City in India" for word in s1.split(): if word[0].isupper(): print (word) The output is: This Delhi City India. Here is a shortened version of the popular answer that gets the first letter by treating the string as an array: function capitalize(s) { return s[0].toUpperCase() + s.slice(1); } Update:
javascript letters as number; javascript uppercase first letter of each word; check if a string contains digits js; how to check if a string is alphabetic in javascript; javascript capitalize first letter; if string have any digits javascript; javascript capitalize first letter of each word; javascript capitalize; javascript capitalize words Definition and Usage. The toUpperCase() method converts a string to uppercase letters.. The toUpperCase() method does not change the original string.. Tip: Use the The toLowerCase() method to convert a string to lowercase. In this article, you are going to learn how to capitalize the first letter of any word in JavaScript. After that, you are going to capitalize the first letter of all words from a sentence. The beautiful thing about programming is that there is no one universal solution to solve a problem.
Introduction. In English, it's essential to capitalize the first letter of a sentence. JavaScript has built-in methods to help us with this. In this article we will look at three different ways to check if the first letter of a string is upper case and how to capitalize it. Javascript function to check for all letters in a field. To get a string contains only letters (both uppercase or lowercase) we use a regular expression (/^ [A-Za-z]+$/) which allows only letters. Next the match () method of string object is used to match the said regular expression against the input value. Here is the complete web document. Capitalizing the first letter of a JavaScript string is easy if you combine the string toUpperCase() method with the string slice() method.. const str = 'captain Picard'; const caps = str.charAt(0).toUpperCase() + str.slice(1); caps; // 'Captain Picard'. The first part converts the first letter to upper case, and then appends the rest of the string.
The str[0].toUpperCase() will uppercase the first letter of the word "how", which is "h" letter in the "how to capitalize only the first letter" string. And str.slice(1) is a method to slice a string according to the passed parameters. Check a password between 7 to 16 characters which contain only characters, numeric digit s and underscore and first character must be a letter. Check a password between 6 to 20 characters which contain at least one numeric digit, one uppercase and one lowercase letter. JavaScript Program to Convert the First Letter of a String into UpperCase In this example, you will learn to write a JavaScript program that converts the first letter of a string into uppercase. To understand this example, you should have the knowledge of the following JavaScript programming topics:
JavaScript on the other hand, assuming you're not using php.js, the act of capitalizing the first letter of a string is a bit more involved. At the most basic level, the steps involved to capitalize the first character of a string are: Isolating the first character from the rest of the string. Capitalizing the character.
Ms Excel How To Extract 1st 2nd 3rd 4th Letters From Words
Overview Of Sql Lower And Sql Upper Functions
Javascript Capitalize First Letter Of Each Word In A String
How To Capitalize A Word In Javascript Samanthaming Com
How To Validate If Input In Input Field Has Uppercase Letters
How To Capitalize The First Letter Of String In Javascript
Dart Program To Check If A Character Is Uppercase Codevscolor
Vb Net Tolower And Toupper Examples Dot Net Perls
Converting A String To Camelcase In Javascript By
Javascript Check If First Letter Of A String Is Upper Case
Character Upper Help Uipath Community Forum
How To Change All Caps To Lowercase Except First Letter In Excel
How To Make First Letter Of A String Uppercase In Javascript
How To Capitalize The First Letter Of A String In Javascript
Change The String Into Uppercase In Php
Python Uppercase A Step By Step Guide Career Karma
Capitalize First Letter Of String In Android Kotlin Amp Java
0 Response to "20 Javascript Check If First Letter Is Uppercase"
Post a Comment