32 Split An Array In Javascript
If the limit is zero, the split() returns an empty array. If the limit is 1, the split() returns an array that contains the string. Note that the result array may have fewer entries than the limit in case the split() reaches the end of the string before the limit. JavaScript split() examples. Let's take some examples of using the split() method. The split() Method in JavaScript. The split() method splits (divides) a string into two or more substrings depending on a splitter (or divider). The splitter can be a single character, another string, or a regular expression. After splitting the string into multiple substrings, the split() method puts them in an array and returns it. It doesn't ...
Numpy Array Manipulation Split Function W3resource
Created: June-14, 2021 . Split an Array Using the slice() Method in JavaScript ; Separating Every Element of an Array Using the slice() Method and forEach Loop in JavaScript ; In JavaScript, playing with arrays and performing various operations on the arrays, whether it's the insertion, deletion, or data manipulation inside an array, are very common things that every programmer should know.
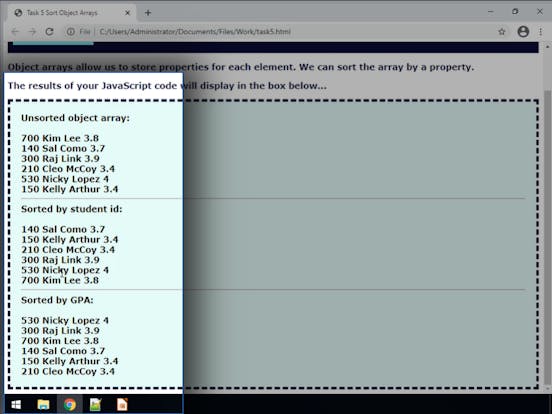
Split an array in javascript. The JavaScript split () method is used to split a string using a specific separator string, for example, comma (, ), space, etc. However, if the separator is an empty string ( "" ), the string will be converted to an array of characters, as demonstrated in the following example: I had a problem. An array contained a lot of items, and I wanted to divide it into multiple chunks. I came up with 2 completely different solutions. A) The first was to divide the array in equal chunks, for example chunks of 2 or 3 items B) The second was to create n chunks and add an equal variable set of items to it It's different how and why we divide. Array.prototype.splice () The splice () method changes the contents of an array by removing or replacing existing elements and/or adding new elements in place. To access part of an array without modifying it, see slice ().
Output: Geeks , Geeks str.split() method is used to split the given string into array of strings by separating it into substrings using a specified separator provided in the argument. Syntax: str.split(separator, limit) Perameters: separator: It is used to specifie the character, or the regular expression, to use for splitting the string. If the separator is unspecified then the entire string ... Split an array into chunks in JavaScript. Last Updated : 16 May, 2019. We have a large array and we want to split it into chunks. So these are few of the methods to do it. splice() This method adds/removes items to/from an array, and returns the list of removed item(s). Sort a Boolean Array in JavaScript, TypeScript, or Node.js Check If an Array Contains a Given Value in JavaScript or Node.js Add an Item to the Beginning of an Array in JavaScript or Node.js Append an Item at the End of an Array in JavaScript or Node.js
JavaScript split array can be done by using a slice () method. The slice () method returns a shallow copy of a portion of an array into a new array object. In the slice method, you have to pass the start and end of the argument. It returns the value of indexed at the given start argument and ends at, but excluding the given end argument. Note ... How can you divide an array in 2 parts, divided exactly in the middle? ... (-half) If the list contains an even number of items, the result is split with exactly half the items. If the number is odd, for example. 🏠 Go back to the homepage How to divide an array in half in JavaScript. Published Apr 18, 2020. How can you divide an array in 2 ... How to Split an Array Into a Group of Arrays in JavaScript Nick Scialli • January 24, 2021 • 🚀 1 minute read Sometimes we have one array that we might want to split into multiple arrays.
JavaScript Array elements can be removed from the end of an array by setting the length property to a value less than the current value. Any element whose index is greater than or equal to the new length will be removed. String.prototype.split () The split () method divides a String into an ordered list of substrings, puts these substrings into an array, and returns the array. The division is done by searching for a pattern; where the pattern is provided as the first parameter in the method's call. STRING.split(SEPARATOR) Convert the string to an array. Elements will be separated by the defined SEPARATOR. Click Here: Array.from(STRING, FUNCTION) Convert the given string, to an array. The mapping FUNCTION is optional. Click Here: Object.assign(TARGET, SOURCE) Assigns the SOURCE to the TARGET. Click Here: JSON.parse(STRING)
So if there is an original array of 20 e-mails I will need to split them up into 2 arrays of 10 each. or if there are 15 e-mails in the original array, then 1 array of 10, and another array of 5. I'm using jQuery, what would be the best way to do this? JavaScript array join() method joins all the elements of an array into a string. The following is the syntax −. Syntax array.join(separator); The following is the parameter −. separator − Specifies a string to separate each element of the array. If omitted, the array elements are separated by a comma. Example. You can try to run the ... # 4 Ways to Convert String to Character Array in JavaScript. Here are 4 ways to split a word into an array of characters. "Split" is the most common and more robust way. But with the addition of ES6, there are more tools in the JS arsenal to play with 🧰
How to split an array into multiple arrays based on a value in JavaScript The next thing to look at is if you want to split an array into multiple arrays based on a value. It is actually pretty easy to do and very similar to the first two examples except instead of finding the halfway point in an array, we need to find a value within the array. Two complementary methods in JavaScript for breaking apart String values and converting them into Arrays OR combining Array elements into a single String.Cod... Removing Elements from End of a JavaScript Array. String split() Method: The str.split() function is used to split the given string into array of strings by separating it into substrings using a specified separator provided in the argument. It is called for each of the array arr elements. Trim and split string to form array in JavaScript.
split () is a method of String Object. It splits a string by separator into a new array. The split () method splits a String object into an array of strings by separating the string into ... < h2 > JavaScript Strings </ h2 > < p > The split() method splits a string into an array of substrings, and returns the array. </ p > ... In this article, you'll learn to split a Javascript array into chunks with a specified size using different implementations. 1. Using a for loop and the slice function. Basically, every method will use the slice method in order to split the array, in this case what makes this method different is the for loop.
The split () method splits a string into an array of substrings, and returns the new array. If an empty string ("") is used as the separator, the string is split between each character. The split () method does not change the original string. Example 2: Split Array Using splice () In the above program, the while loop is used with the splice () method to split an array into smaller chunks of an array. The first argument specifies the index where you want to split an item. The second argument (here 2) specifies the number of items to split. The while loop is used to iterate over the ... Arrays are one the most used structures in JavaScript programming, which is why it's important to know its built-in methods like the back of your pocket. In this tutorial, we'll take a look at how to split an array into chunks of n size in JavaScript. Specifically, we'll take a look at two approaches: Using the slice() method and a for loop
Split ( ) Slice ( ) and splice ( ) methods are for arrays. The split ( ) method is used for strings. It divides a string into substrings and returns them as an array. It takes 2 parameters, and both are optional. string.split (separator, limit); Separator: Defines how to split a string… by a comma, character etc. Creating arrays by using split method in a string We have seen how to create string using elements of an array. Now we will see how to create an array from a string by using some delimiter. For this we will use split() method which works on a string and create array out of it. This is one of the method used to create an array.
Parsing A Query String Into An Array With Javascript A
Javascript Split String By Comma Tuts Make
Split String Overview Outsystems
Visual Basic String Split Method Tutlane
Convert String To Array Using Javascript Split Method
How To Split An Array Into Half In Javascript Codingshala
How To Fix This String Into Array Using Split Method
Split The String Into Separate Values General Node Red Forum
Convert Comma Separated String To Array Using Javascript
String Split Method Archives Js Startup
How To Split An Array Into Two In Javascript Split In Half
Splitting An Array Of Objects General Node Red Forum
Split An Array Into Chunks In Javascript Geeksforgeeks
How To Split A String Into An Array In Javascript Howchoo
Javascript Splice Slice Split By Jean Pan Medium
Javascript Split Array And Call It Over And Over Again Ittone
Solved How Do You Split Items Inside An Array Power
Javascript Splice Slice Split By Jean Pan Medium
Splitting Array Into Groups Of 3 In Javascript Stack Overflow
Github Samicey Canbalance This Is An Algorithm Written In
11 Ways To Check For Palindromes In Javascript By Simon
How To Split An Array To Multiple Arrays As Sets Typescript
Javascript Split A String And Convert It Into An Array Of
How To Manipulate A Part Of String Split Trim Substring
Learn Online Code Tutorial Tuts Make
Javascript Typed Arrays Javascript Mdn
Split And Join In Javascript Examples And Use In Array
Javascript Split String By Comma Pakainfo
Sort Arrays With Javascript Methods
0 Response to "32 Split An Array In Javascript"
Post a Comment