27 Javascript Sum Of Object Values
Javascript sum array. To find the Javascript sum array of two numbers, use array.reduce () method. The reduce () method reduces an array to a single value. The reduce () function executes the provided function for each value of the array (from left-to-right). The return value of a method is stored in an accumulator (result/total). javascript sum array of objects; javascript array unique values; duplicate an array in javascript n times; remove null from array javascript; javascript filter null values from array; js map value in range; array contains case insensitive javascript; merge sort javascript; javascript group by property array of objects; javascript group by on ...
Javascript Basic Compute The Sum Of Two Parts And Store Into
Here will take e.g. sum of array values using for loop, sum of array object values using javaScript reduce () method, javascript reduce array of objects by key, javascript reduce array values using javascript map () method. If you should also read this javascript array posts: Convert Array to Comma Separated String javaScript
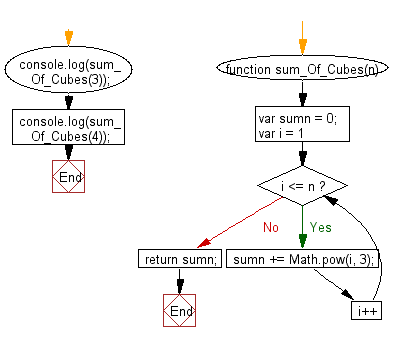
Javascript sum of object values. Given a list of daily temperatures T, return a list such that, for each day in the input, tells you how many days you would have to wait until a warmer temperature. If there is no future day for which this is possible, put 0 instead javascript Morioh is the place to create a Great Personal Brand, connect with Developers around the World and Grow your Career! Nov 21, 2020 - Suppose we have an array of objects that contains the data about some students and their marks like this −const arr = [ { subject: 'Maths', marks ...
8/4/2020 · Sum values of an object with for..in in Javascript Another interesting way to sum object values in Javascript is with for..in loop, which iterates over properties of an object. We can sum up the values with for..in by creating a variable total and adding the next value within the for..in loop to the total variable. You'll notice that the values of the second object equal 15, as well as all of the values of the properties named "b", so it is not entirely clear what you're after here. Answer 3 The method to get a single value back from an array of values is Array#reduce, you give it an accumulator function and an optional starting value and it applies it to ... Aug 29, 2019 - Using Array.reduce to sum a property in an array of objects - reduce-example.js
To calculate the sum of values of a Map<Object, Integer> data structure, first we create a stream from the values of that Map. Next we apply one of the methods we used previously. For instance, by using IntStream.sum (): Integer sum = map.values () .stream () .mapToInt (Integer::valueOf) .sum (); 6. Sum from array values with similar key in JavaScript Javascript Web Development Object Oriented Programming Let's say, here is an array that contains some data about the stocks sold and purchased by some company over a period of time. Once we've "found" the elements, we'll find the sum of those elements, and display the sum in the alert box. Finally, we take that sum and put it in the bottom row of the table. Step 1: a JavaScript stub function that runs when the page loads. In this step, we just add a stub function that will run as soon as the page loads.
This post will discuss how to find the sum of all the values of an array in JavaScript. 1. Using Array.prototype.reduce () function. The reduce () method can be used to execute a reducer function on each array element. To calculate the sum of all the values of an array, the reducer function should add the current element value to the already ... Jun 13, 2021 - In this article, we will learn better and efficient ways to sum the values of an array of objects in JavaScript. In our examples, we will sum the Amount for all objects in the following array. Sum of values in an object array To sum up the values contained in an array of objects, you must supply an initialValue , so that each item passes through your function. let initialValue = 0 let sum = [ { x : 1 } , { x : 2 } , { x : 3 } ] . reduce ( function ( accumulator , currentValue ) { return accumulator + currentValue . x } , initialValue ...
JavaScript provides 8 mathematical constants that can be accessed as Math properties: Example. Math.E // returns Euler's number. Math.PI // returns PI. Math.SQRT2 // returns the square root of 2. Math.SQRT1_2 // returns the square root of 1/2. Math.LN2 // returns the natural logarithm of 2. Math.LN10 // returns the natural logarithm of 10. Javascript Object.values() Javascript Object.values() is a built-in function that returns the array of the given Object's enumerable property values. The Object values() takes an object as an argument of which enumerable own property values are to be returned and return the array containing all the enumerable property values of the given Object. Get code examples like"javascript object array sum of values in object". Write more code and save time using our ready-made code examples.
arr = [{x:1}, {x:3}] arr.reduce((accumulator, current) => accumulator + current.x, 0); Sum object properties. importance: 5. We have an object storing salaries of our team: let salaries = { John: 100, Ann: 160, Pete: 130 } Write the code to sum all salaries and store in the variable sum. Should be 390 in the example above. If salaries is empty, then the result must be 0. let salaries = { John: 100, Ann: 160, Pete: 130 }; let sum ... totalValue is the sum accumulator as before. currentValue is the current element of the array. Now, this element is an object. So the only thing we have to do different in this example is to add the totalValue with the x property of the current value.
Sep 18, 2020 - We are required to write a JavaScript function that takes in one such array and combines the value property of all those objects that have similar value for the firstName property. You can use the reduce () method to find the sum of an array of numbers. The reduce () method executes the specified reducer function on each member of the array resulting in a single output value as in the following example: The 0 in is the default value. If default value is not supplied, the first element in the array will be used. It can be as simple as that: const sumValues = obj => Object.values(obj).reduce((a, b) => a + b); Quoting MDN: The Object.values() method returns an array of a given object's own enumerable property values, in the same order as that provided by a for...in loop (the difference being that a for-in loop enumerates properties in the prototype chain as well).
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: Jan 18, 2021 - When you call the reduce method ... first object as the initial value. Perform multiple operations before returning the data · If the cart has quantity data, you can multiply the quantity by price before you add to the sum to the accumulator: let cart = [ { name: "JavaScript book", quantity: ... Sum values of objects in array. Ask Question Asked 5 years, 6 months ago. Active 1 year, 6 months ago. Viewed 50k times ... How to Solve this code in javascript? Combining of different objects with same value into single array-3. Add together all objects in an array of objects. 0.
Iterate over each object's (basket's) key-value pairs using Object.entries() method. Check if the result object has a property with the name of the key. If yes (true), add to the previous value of the property the value of the key, e.g. if there is a property "apples" with value "4" in the result object, then add more apples from the ... If we need to sum a simple array, we can use the reduce method, that executes a reducer function (that you provide) on each member of the array resulting in a single output value. For example: let sum = [1, 2, 3].reduce ( (a, b) => a + b, 0); console.log (sum); // expected output: 6. But if we have an objects array like the following: The reduce() method applies a function ... value of the array (from left-to-right) to reduce it to a single value. ... Note that this code uses some ECMAScript features which are not supported by some older browsers (like IE). You might need to use Babel to compile your code. ... function sum( obj ) { var ...
Oct 09, 2020 - arr = [{x:1}, {x:3}] arr.reduce((accumulator, current) => accumulator + current.x, 0); arr = [{x:1}, {x:3}] arr.reduce((accumulator, current) => accumulator + current.x, 0); Home » JavaScript » Sum array of objects values by multiple keys in array. Search for: Search for: JavaScript September 17, 2020. Sum array of objects values by multiple keys in array. Suppose I have:
Sep 25, 2019 - In ultra modern javascript you could use Object.values (only available using the Babel transpiler or Typescript I think). const sumObj = (o) => Object.values(o).reduce((sum, n) => sum + n, 0) But rn the best approach is the for-in loop which gives you a list of “keys” (props) so that you ... 1/7/2021 · We’ll create an object with numeric values. And then sum the values in the object using a syntax. So to begin, create an object with numeric values like this: const speed = { bike : 60, car : 300, tricycle: 100, motorBike: 200, superBike :500, } Next, to view this object, we log it to the console like this: console.log (speed); 26/2/2020 · JavaScript Code: function sum(input){ if ( toString.call( input) !== " [object Array]") return false; var total = 0; for(var i =0; i < input.length; i ++) { if(isNaN( input [ i])){ continue; } total += Number( input [ i]); } return …
Object.values() returns an array whose elements are the enumerable property values found on the object. The ordering of the properties is the same as that given by looping over the property values of the object manually. Oct 09, 2020 - arr = [{x:1}, {x:3}] arr.reduce((accumulator, current) => accumulator + current.x, 0); In javascript, we can calculate the sum of the elements of the array by using the Array.prototype.reduce () method. The reduced method for arrays in javascript helps us specify a function called reducer function that gets called for each of the elements of an array for which we are calling that function.
Object.values(hero) returns the values of hero: ['Batman', 'Gotham']. 2.1 Values in practice: calculate properties sum. books is an object that holds the prices of some books. The property key is the book name, while the value is the book price. How would you determine the sum of all books from the object? Plain objects also support similar methods, but the syntax is a bit different. Object.keys, values, entries. For plain objects, the following methods are available: Object.keys(obj) - returns an array of keys. Object.values(obj) - returns an array of values. Object.entries(obj) - returns an array of [key, value] pairs.
Sum Object Property And Store In Another Property In Javascript Javascript Tutorial Javascript
Javascript How Group And Sum Values From Multidimensional
Javascript Basic Compute The Sum Of Cubes Of All Integer
Array Prototype Reduce Javascript Mdn
Javascript Group By Sum Array Reduce Code Example
Javascript Sum Array Object Values Examples
Javascript Algorithm Sum All Numbers In A Range By Erica N
Javascript Array Reduce How To Create Reducer Function
Javascript Array Reduce Amp Reduceright Reducing An Array Into
How To Obtain The Sum Of Integers Between 2 Indexes In The
Javascript Array Map Return Object Code Example
Add Two Numbers In C Using Method
Hacks For Creating Javascript Arrays
Javascript Array Compute The Sum And Product Of An Array Of
How To Access Object S Keys Values And Entries In Javascript
Implementing Custom Javascript Actions For Scriptless Testing
Javascript Lesson 27 Add Methods To Javascript Object
Javascript Sum All Numbers In A Range And Diff Two Arrays
Javascript Quiz Find Sum Of Object And Variable Value Js
How To Group An Array Of Objects Through A Key Using Array
Rounding And Truncating Numbers In Javascript Pawelgrzybek Com
Kent C Dodds No Twitter Here 39 S What Mdn Has To Say About
Javascript Sum Array Calculate The Sum Of The Array Elements
Javascript Math Calculate The Sum Of Values In An Array
0 Response to "27 Javascript Sum Of Object Values"
Post a Comment