21 Javascript Generate Number Sequence
A.map (Function.call, Number) returns an N -element array, whose index I gets the result of Function.call.call (Number, undefined, I, A) Function.call.call (Number, undefined, I, A) collapses into Number (I), which is naturally I. Result: [0, 1,..., N-1]. For a more thorough explanation, go here. I am quite against writing all functions as arrow functions. The reason being that arrow functions are not bind-able and it refers to the this from the outer scope. I feel that it is designed to prevent us writing var self = this; and refer to self in a callback function. So I tend to write arrow functions only when it is a callback or when it is a one-off function.
Fibonacci Series In Javascript Javatpoint
9/1/2020 · You can also generate random numbers in the given range using JavaScript. Approach 1: Get the minimum and maximum number of n digits in variable min and max respectively. Then generate a random number using Math.random () (value lies between 0 and 1). Multiply the number by (max-min+1), get its floor value and then add min value to it.
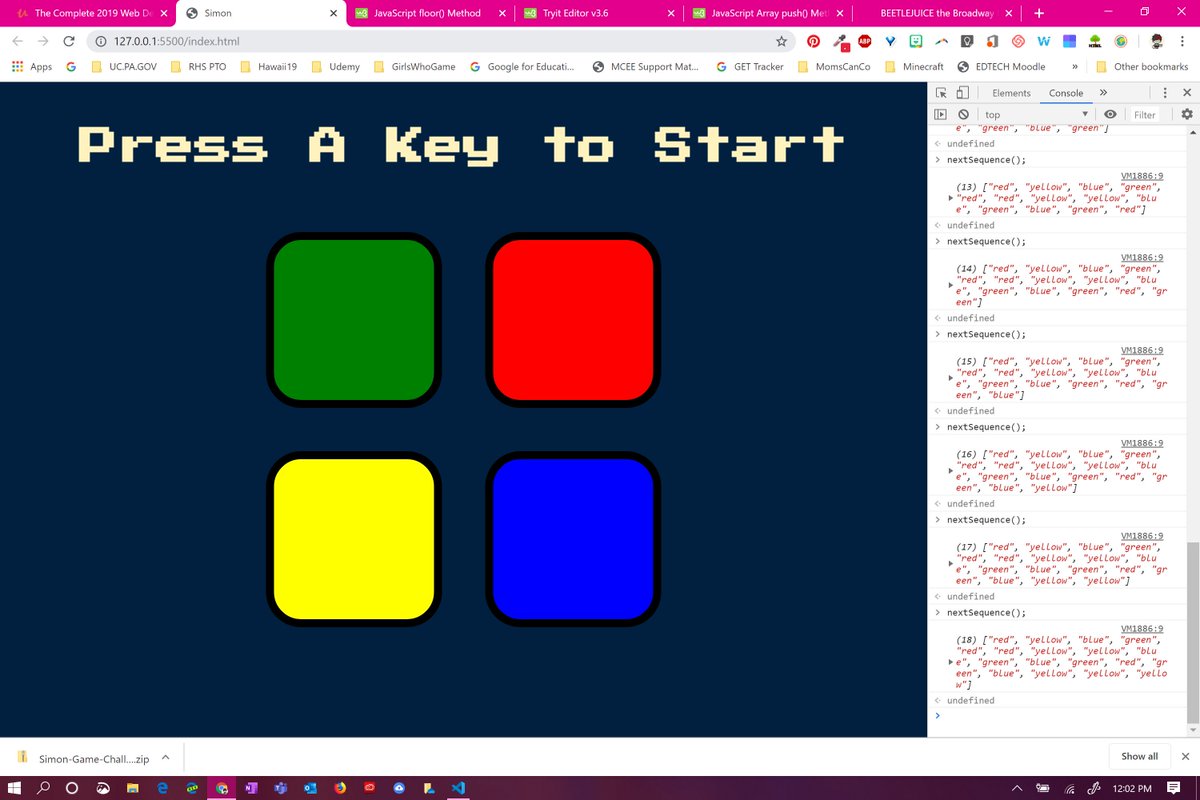
Javascript generate number sequence. Algorithmic random number generation can't exactly be random, per se; which is why they're more aptly called pseudo-random number generators (PRNGs). If you're using math and formulae to create a sequence of numbers, random though they might seem, those numbers will eventually repeat and reveal a non-random pattern. 19/2/2021 · JavaScript generate a number sequence Example HTML example code generate array of sequential numbers in JavaScript. <!DOCTYPE html> <html> <body> <script type="text/javascript"> function range(start, end) { return Array(end - start + 1).fill().map((_, idx) => start + idx) } var seqNumbers = range(9, 18); console.log(seqNumbers); </script> </body> </html> Is there a way to generate sequence of characters or numbers in javascript? For example, I want to create array that contains eight 1s. I can do it with for loop, but wondering whether there is a jQuery library or javascript function that can do it for me?
Random Sequence Generator. This form allows you to generate randomized sequences of integers. The randomness comes from atmospheric noise, which for many purposes is better than the pseudo-random number algorithms typically used in computer programs. Note: A randomized sequence does not contain duplicates (the numbers are like raffle tickets ... mysequence. A nodejs sequence generator which generate unique sequential numbers as user-assigned ids for your records/documents. There are four reasons to use mysequence to generate sequences as ids/keys for your records/documents by application itself.. Independent: Our data model and persistence layer can be independent of any RDB & noSQL DB such as MySQL auto increment, MongoDB ObjectId ...
The most common iterator in JavaScript is the Array iterator, which returns each value in the associated array in sequence. While it is easy to imagine that all iterators could be expressed as arrays, this is not true. Arrays must be allocated in their entirety, but iterators are consumed only as necessary. To generate a member of the sequence from the previous member, we read off the digits of the previous member, counting the number of digits in groups of the same digit. For instance, the next number to 1211 is −. 111221. Because if we read the digit of 1211 louder it will be −. One one, one two, two one which gives us 111221. Most people want to use a random number because they think it will solve the problem of different people using the same form. Well it doesn't. You can't even guarentee that it's unique. If you want different people to fill out the form and generate Invoice numbers, then you need a common source for the numbers. For example a local DB, or even a ...
In JavaScript, you can use the Math. random () function to generate a pseudo-random floating number between 0 (inclusive) and 1 (exclusive). If you want to get a random number between 0 and 20, just multiply the results of Math.random () by 20: To generate a random whole number, you can use the following Math methods along with Math.random (): Generate a Sequence of Numbers or Characters in JavaScript. To generate a sequence of numbers or characters in JavaScript, we can create our own function. For instance, we write: A good generator will produce a very long sequence of apparently random numbers. The length of this sequence is referred to as the period of the generator (less commonly, the cycle). A properly configured LCG should have a period equal to its modulus, in this case, 25.
Number sequences are used to generate readable, unique identifiers for master data records and transaction records that require identifiers. A master data record or transaction record that requires an identifier is referred to as a reference. Before you can create new records for a reference, you must set up a number sequence and associate it ... A Proper Random Function. As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes. This JavaScript function always returns a random number between min (included) and max (excluded): 22/9/2017 · Select 'javascript' tool and then 'document javascript' or while in 'prepare form'tool press 'shift+d'. add new script and replace any code already in when you open window with this code: var num = this.getField("Text1"); num.value = Number(num.value)+1; num.defaultValue = num.value;
I was using JavaScript to augment the creation of a list item and document in SharePoint. The customer wanted to have a unique sequence number (record identifier) that was a combination of the year and a sequence number. (yy-s). This means that I needed to have SharePoint manage the sequence number as a list item. 24/5/2017 · If you want to produce a sequence of equal numbers, this is an elegant function to do it (solution similar to other answer): seq = (n, value) => Array(n).fill(value) If you want to produce a sequence of consecutive numbers, beginning with 0, this a nice oneliner: With just three lines of code, we could generate a maximum of 8nnumbers for an n-digit number after which the sequence repeats itself. There is a pitfall though. Some seeds can cause the algorithm to have a shorter cycle like the seed 25, which causes the algorithm to repeat 25 indefinitely. 2.
I have a doubt and I wonder how can I insert a script and javascript that I would generate a 4-digit sequential number in a text field, the numerical sequence should be updated every refresh I give the new form with the button I added to it, numerical sequence from 0001 to 1000 Example 2: Fibonacci Sequence Up to a Certain Number // program to generate fibonacci series up to a certain number // take input from the user const number = parseInt(prompt('Enter a positive number: ')); let n1 = 0, n2 = 1, nextTerm; console.log('Fibonacci Series:'); console.log(n1); // print 0 console.log(n2); // print 1 nextTerm = n1 + n2; while (nextTerm <= number) { // print the next ... 8/8/2019 · replied on August 8, 2019. So, assuming that you are storing the sequence number in a hidden field from a lookup on the form, you can use the following function: function getSequence () {. // Get Year portion. var now = new Date (); //current date.
Create a sequence of number triples. Generate Tuples of Numbers. Create a sequence of number n-tuples. Find the Decimal Expansion of a Number. ... All conversions and calculations are done in your browser using JavaScript. We don't send a single bit about your input data to our servers. There is no server-side processing at all. How to Create Random Numbers & Characters. This page shows you how to create a random sequence of numbers and/or text. The function which generates the random string can be called from any event handler - the example below uses a button. This article describes how to generate a random number using JavaScript. Method 1: Using Math.random () function: The Math.random () function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive). This random number can then be scaled according to the desired range.
How to get sequence number in loops with JavaScript? Javascript Web Development Object Oriented Programming. To get sequence number in loops, use the forEach () loop. Following is the code −. Array.from() lets you create Arrays from: array-like objects (objects with a length property and indexed elements); or ; iterable objects (objects such as Map and Set).; Array.from() has an optional parameter mapFn, which allows you to execute a map() function on each element of the array being created. More clearly, Array.from(obj, mapFn, thisArg) has the same result as Array.from(obj).map ... How to generate the sequential number in Javascript. 731 Views. Hi , ... It is not one way they may even create the record between 2 and 3 after created the 18th record.. ... The number is : last two digit of year+ F or R + (sequence number 001 or 002 or 003 only newly added record fields F1).. I added the code in click event rightnow I am ...
A lot of applications use IDs that are based on a sequence but use an additional prefix. You can easily support these IDs with a custom id generator. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Tutorials References Exercises Videos NEW Menu . ... Return a random number between 0 (inclusive) and 1 (exclusive):
Foundations Of Random Number Generation In Javascript Dzone
Generate A Random Sequence With All Numbers Javascript Code
Quantum Random Number Generators With Entanglement For Public
De Bruijn Sequence Generator For Faster Shift Register Code
Tranziție Antagonist Tip Jquery Generate Number Sequence
Pseudorandom Number Generators Video Khan Academy
Build Machine Learning Model Using Tensorflow Js Python
How To Quickly Create An Array Of Sequential Numbers In
Github Henryleu Mysequence A Javascript Nodejs High
Gamedev Glossary Sequence And Number Generators
Random Number Generator How Do Computers Generate Random
Part 1 Javascript Loops And Document Write An Chegg Com
Generating Fibonacci Sequence With Javascript Datamelo
Vivax Solutions Learn Javascript Promises Interactively
Knives Chau On Twitter Applying Jquery And
Generate Uml Sequence Diagrams With Javascript Miha Jakovac
Javascript Traverse A Tree To Generate The Sequence Of The
0 Response to "21 Javascript Generate Number Sequence"
Post a Comment