33 How To Handle Javascript Alert In Selenium Webdriver
To handle alert window in Selenium Webdriver we have predefined Interface known as Alert. Check out official docs for Alert in Selenium Webdriver. Alert Interface has some methods- 1- accept ()- Will click on the ok button when an alert comes. ** Selenium Training: https://www.edureka.co/selenium-certification-training **This Edureka 'Alerts in Selenium Webdriver' video helps you understand how to ...
How To Handle Login Pop Up Window Using Selenium Webdriver
We can handle alerts in Selenium webdriver by using the Alert interface. An alert can be of three types - a prompt which allows the user to input text, a normal alert and a confirmation alert.
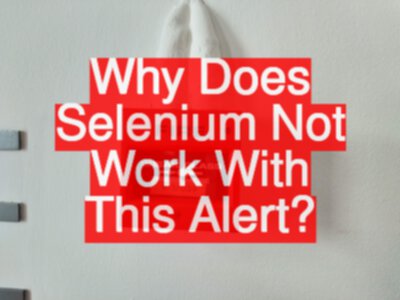
How to handle javascript alert in selenium webdriver. In this video, I have shown how to handle Alerts in Selenium WebDriver.Selenium WebDriver is an open-source tool for automated testing of web apps across man... 18/1/2020 · To solve this, WebDriver provides API to handle JavaScript alert and popup. Let’s check the code first, and then try to understand it. Code Explanation. We can handle the JavaScript popup using the Alert interface. It contains method to switch to the alert box using the statement, Alert alert = driver.switchTo().alert(). 31/5/2021 · Alert Interface Methods Provided By Selenium WebDriver. When an alert gets triggered, the control still remains with the webpage and an alert interface is required to shift the control to the pop-up window. The switch_to method in Python is used for switching to the desired alert window. alert = driver.switch_to.alert. The Java equivalent of the same is below: Alert alert = driver.switchTo().alert(); …
Hello Dimple, the simplest way to handle JavaScript popup/alert using selenium is by using the Alert interface. To access the popup/alert dialog in Selenium Webdriver, use the following line of code: webDriver.switchTo ().alert () The alert interface provides following methods to handle/interact with such Javascript popups/dialogs: JavaScriptExecutor is an interface provided by Selenium Webdriver, which presents a way to execute JavaScript from Webdriver. This interface provides methods to run JavaScript on the selected window or the current page. JavaScriptExecutor is an interface which is available for all the languages that support the Selenium Framework. 28/8/2021 · JavaScriptExecutor provides two methods "executescript" & "executeAsyncScript" to handle. Executed the JavaScript using Selenium Webdriver. Illustrated how to click on an element through JavaScriptExecutor, if selenium fails to click on element due to some issue. Generated the 'Alert' window using JavaScriptExecutor.
Handling alerts is one of the tricky tasks, but Selenium WebDriver provides some of the predefined methods, which makes the handling process so easy. When we are executing our automation script, we are creating an object of browser class, and that driver object has control over all the browser operations even on alert too. 1.Introduction In this article, we're going to illustrate how to handle alert or javascript Popup using selenium WebDriver. 2. Basics Let's get started by understanding what are these alerts or javascript popups. Javascript Alert/popup is a notification/warning or message displayed on the User interface in box format to notify the user about some information or … Selenium webdriver software testing tool has Its own Alert Interface to handle all above different popups. Alert Interface has different methods like accept(), dismiss(), getText(), sendKeys(java.lang.String keysToSend) and we can use all these …
The Alert Interface provides some methods to handle the popups. While running the WebDriver script, the driver control will be on the browser even after the alert generated which means the driver control will be behind the alert pop up. In order to switch the control to alert pop up, we use the following command : WebDriver offers the users with a very efficient way to handle these pop ups using Alert interface. There are the four methods that we would be using along with the Alert interface. 1) void dismiss () - The dismiss () method clicks on the "Cancel" button as soon as the pop up window appears. Inspect element 'Click for JS Alert' Write line of code to find and click on above element and use below code to click on 'Ok' on the java pop up alert box driver.switchTo ().alert ().accept (); Inspect element 'Click for JS Confirm'
Whenever we open an application in Selenium the focus of the web driver remains on the current page. If any action triggers an alert/PopUp Selenium will not be able to perform any operation on the Alert/PopUp until the web driver focus is shifted on it. Once the focus is shifted to the Alert/PopUp we can perform the desired action on it. It is present in the org.openqa.selenium.Alert package. Alert interface gives us following methods to deal with the alert: accept () To accept the alert by clicking on OK button. dismiss () To dismiss the alert by clicking on CANCEL button. getText () To get the text of the alert. 28/8/2021 · How to handle Alert in Selenium WebDriver. Alert interface provides the below few methods which are widely used in Selenium Webdriver. 1) void dismiss () // To click on the 'Cancel' button of the alert. 2) void accept () // To click on the 'OK' button of the alert. 3) String getText () // To capture the alert message.
Selenium provides an Alert interface, which is used to handle JavaScript alert. The basic syntax is: Alert alert = driver.switchTo ().alert (); Methods provided by Alert interface: accept (); //To select Accept/OK from the prompt. dismiss (); // To select Dismiss/Cancel option from the prompt. In this section, you will learn how to handle alerts in Selenium WebDriver. Selenium WebDriver provides three methods to accept and reject the Alert depending on the Alert types. 1. void dismiss () This method is used to click on the 'Cancel' button of the alert. To use JavaScriptExecutor in Selenium scripts there is no need to install an addon or plugin. The only step we need to take is to import org.openqa.selenium.JavascriptExecutor in the Selenium script. JavascriptExecutor in Selenium enables the WebDriver to interact with HTML DOM within the browser.
The following methods are useful to handle alerts in selenium: 1. Void dismiss (): This method is used when the 'Cancel' button is clicked in the alert box. 2. Void accept (): This method is used to click on the 'OK' button of the alert. 3. A web alert is generally a javascript based pop-up that appears on the screen. It commonly have a text message and two buttons which could be Yes or No, Accept or Dismiss, Ok or Cancel. So there aren't much operations we can perform on an alert. Below are the commands used to handle web alerts: alert.accept () 1/11/2017 · You need to use Alert interface to handle it in selenium webdriver. Javascript code to create a confirm popup: Java code: Output: Alert text is:I am a confirm popup. You can inspect me. You need to use alert interface to handle me. Alert closed successfully afetr accept. You pressed OK! Alert closed successfully after dismiss. You pressed Cancel!
19/1/2014 · Working with Alerts using Selenium Webdriver: The below is the sample code for alerts, please copy and make an html file and pass it to the webdriver. <html> <head> <title> Selenium Easy Alerts Sample </title> </head> <body> <h2> Alert Box Example </h2> <fieldset> <legend> Alert Box </legend><p> Click the button to display an alert box. </p> ... Selenium WebDriver gives multiple APIs to handle pop ups or alerts with the help of Alert interface. dismiss () This will cancel the button for alert. accept () This will accept the button for alert. getText () This will extract the text on alert. sendKeys () This will enter text on the alert box. Handling JavaScript Alert in Selenium WebDriver is big question if you have just started learning Selenium WebDriver. Because we always get multiple pop-ups and alert and some time we get information about validity of input and some time these pop-ups speaks about error, and also asks about user response.
You need to do some action such as accept or dismiss the alert box to resume your task on the browser. To handle alerts popupswe need to do switch to the alert window and call Selenium WebDriver Alert API methods. There are two types of alerts. So, for handling the Alerts using Selenium WebDriver, the focus need to be shifted to the child windows opened by the Alerts. To switch the control from the parent window to the Alert window, the Selenium WebDriver provides the following command: driver.switchTo ().alert (); 7/1/2015 · I am not sure whether selenium webdriver can handle Javascript alert/pop-up window. I have a scenario like 1. User uploads a xls file and click on upload button 2. Alert/Pop-up window will be displayed . Click "OK" on window. Am able to automate the above scenario but the Alert/pop-up window is displayed while running the scripts.
How to Handle Web Alerts using Selenium Webdriver? Selenium WebDriver provides below methods to accept ,reject , retrieve text and typing the text in the input box for the Alert . 1. void dismiss() It is used to click on the 'Cancel' button of the alert. Syntax: driver.switchTo().alert().dismiss(); 2. void accept()
How To Handle Alert In Selenium Webdriver Scientech Easy
Webdriver Handling Alerts Javatpoint
How To Click On A Browser Alert Using Ruby And Selenium
How To Handle Javascript Alerts Popups In Selenium Webdriver
How To Handle Javascript Alert Message Popup In Selenium Web
Selenium Alert Amp Popup Window Handling How To Handle
How To Handle Alerts And Pop Ups In Selenium Edureka
How To Handle Alerts Popups In Selenium Webdriver Selenium
How To Handle Alerts In Selenium Webdriver Qa Tech Hub
Easy Approach To Handle Authentication Window In Selenium
Selenium Alert Amp Popup Window Handling How To Handle
How To Handle Javascript Alert In Selenium Webdriver Using
Popup Handling In Selenium Appening
Handling Window Authentication In Chrome Using Selenium
How To Handle Alerts Popups In Selenium Webdriver Selenium
Javascript Error Handling With Javascriptexecutor In Selenium
Handling Javascript Alerts When Leaving A Page With Webdriver
Handling Javascript Alert And Popup In Selenium The Testers
Popup Handling In Selenium Appening
Handle Alert Amp Popup Boxes Using Selenium With Python
How To Handle Alert In Selenium Webdriver Scientech Easy
Use Of Switch To Commands For Handling Javascript Pop Ups And
Js Alert Popup Auth Pop Up And File Upload Pop Up Handling
Handle Pop Up Dialog Alert In Selenium Webdriver
How To Handle Browser Closing Popup Software Quality
Js Alert Popup Auth Pop Up And File Upload Pop Up Handling
How To Handle Javascript Alert In Selenium Webdriver Using
Handling Javascript Alert In Puppeteer Tools4testing
Alert Amp Popup Window Handling In Selenium Webdriver Besant
Why Does Selenium Not Work With This Alert Eviltester Com
Selenium C Tutorial Handling Alert Windows
0 Response to "33 How To Handle Javascript Alert In Selenium Webdriver"
Post a Comment