21 How To Write Data To A Text File In Javascript
I need to save same data to a .txt file (using javascript). Please see this example of clicking in an image and get the coordinates ( Click Here ). The coordinates appear on the bottom of the image. Thanks for the link! I'll check it out today. I have a wpf application that has the option to create a 'print out' form in excel. Each page already has an excel template to sort of mirror the wpf page, but I would want to check and make sure the data from the excel print form matches the data that was just entered into the form.
Reading Uploaded Text File Contents In Html Stack Overflow
writeFile(Path, Data, Callback) It has three parameters path, data, callback. Path: The path is the location of Text File. If the file is to be generated in the same folder as that of the program, then provide the name of the file only.
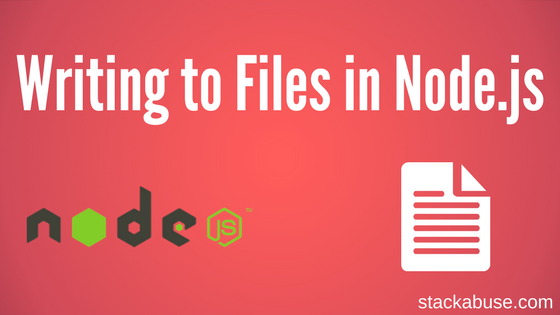
How to write data to a text file in javascript. A web form usually has many different elements, mostly input fields. You can extract data from these elements and save it in a database like SQL Server, or simply convert it into a JSON file. You can even save your form data in a text file. Let's see the example first. Definition and Usage. The write () method writes HTML expressions or JavaScript code to a document. The write () method is mostly used for testing: If it is used after an HTML document is fully loaded, it will delete all existing HTML. Note: When this method is not used for testing, it is often used to write some text to an output stream opened ... In this Tutorial. Quick Sample Code. Demo — Reading a Local Text File. How is File Reading Done ? Step 1 — Allow User to Choose the File. Step 2 — Read File Metadata (Name, Type & Size) using Properties of File Object. Step 3 — Read File Contents using FileReader Object. Other FAQs on Reading a File with Javascript.
This page discusses - JavaScript write to text file. The Active object takes three parts: servername - the name of the application providing the object. It is required. typename - the type or class of the object to create. location - the name of the network server where the object is to be created.. Here, we have taken servername as 'Scripting' and typename as 'FileSystemObject': Due to security restrictions, client-side Javascript cannot directly access the file system. That is, no direct writing and loading of files on the user's computer. But this is the roundabout way of doing things - Create a BLOB (binary) object to contain all the data, then set a download link to it. 3) UPLOAD BLOB TO SERVER THE JAVASCRIPT Example: In this example we will just create a text area where the text will appear from the text file that has been used as an input in the index.html. The JavaScript code will be able to extract the text from any text file and display it in script.js. index.html:
It is basically a JavaScript program (fs.js) where function for writing operations is written. Import fs-module in the program and use functions to write text to files in the system. The following function will create a new file with a given name if there isn't one, else it will rewrite the file erasing all the previous data in it. Using a library. Make libraries, not the war. FileSaver.js implements the saveAs() FileSaver interface in browsers that do not natively support it.. If you need to save really large files bigger then the blob's size limitation or don't have enough RAM, then have a look at the more advanced StreamSaver.js that can save data directly to the hard drive asynchronously with the power of the new ... Let's keep the following html [code]<form onSubmit="WriteToFile(this)"> <label>Type your first name:</label> <input type="text" name="FirstName" id="firstName" size ...
Creating CSV Files in Javascript. To create a CSV file, we need a data source that creates a CSV file. The data source that we encounter most often is an array of data types. Besides that, there is also a collection of objects in an array. See the following code to create a CSV file in Javascript. I have some problem to write date to a file Test.txt by using JavaScript. I have find answer in good too but I am still can't solve it. This is my code <script type="text/javascript"> funct... Read Write to file with javascript. GitHub Gist: instantly share code, notes, and snippets.
It provides sync and async functions to read and write files on the file system. Let us look at the exmaples of reading and writing files using the fs module on node.js Let us create a js file named main.js having the following code − Write To a File. In the following example, we use the FileWriter class together with its write() method to write some text to the file we created in the example above. Note that when you are done writing to the file, you should close it with the close() method: Given a text file, write a JavaScript program to extract the contents of that file. There is a built-in Module or in-built library in NodeJs which handles all the reading operations called fs (File-System). It is basically a JavaScript program (fs.js) where function for reading operations is written. Import fs-module in the program and use ...
However, it is not possible for browser-based Javascript to WRITE the file system of local computer without disabling some security settings because it is regarded as a security threat for any website to change your local file system arbitrarily. The text file (here is only a basic exemple) will be generated by an Excel extract. Therefore, I have no possibility to transform it into a javascript script assigning data to variables. Regards. Jean-Marie I assume that you have access to the Excel file. If so, why not simply make a VB macro saving the text file in any format you decide? There is an ArrayBuffer present which represents the data of the file. FileReader.readAsBinaryString(): This method also reads the contents of specified file in javascript. The result of this file contains string as the raw data. FileReader.readAsText(): This method will read the contents of javascript file. It contains the content as a text ...
JavaScript can "display" data in different ways: Writing into an HTML element, using innerHTML. Writing into the HTML output using document.write (). Writing into an alert box, using window.alert (). Download JavaScript Data as Files on the Client Side February 09, 2019. When building websites or web apps, creating a "Download as file" link is quite useful. For example if you want to allow user to export some data as JSON, CSV or plain text files so they can open them in external programs or load them back later. Create a text area to enter the text data. Create an anchor tag <;a> using the createElement property and assign download and href attributes to it.; Use the encodeURIComponent to encode the text and append it to URI as its component. This will help us to replace certain special characters with a combination of escape sequences.
Quick explanation: I need to run this script for all docs in the collection and check if the data fields are data objects or null, then perform a correction (or do nothing in case of null) and append the _ids and the data itself to a txt file. If there is an easier way to do it, I will apreciate it very much! A JavaScript function that fire on the button click event. Create a Blob constructor, pass the data in it to be to save and mention the type of data. And finally, call the saveAs (Blob object, "your-file-name.text") function of FileSaver.js library. Note: To create and save data into a text file, I have used a third party FileSaver.js library. The fs module includes a high-level writeFile method that can write data to files asynchronously. This means, like with a lot of operations in Node.js, the I/O is non-blocking, and emits an event when the operation finishes. We can write a callback function to run when the writeFile returns.. Here is a simple code example of writing some song lyrics to a file:
In my previous article, I wrote about reading and writing different types of files in Java. In this quick article, you'll learn how to read and write text files in Java. Reading Text Files. Java provides multiple APIs to read a text file. The result attribute contains a URL representing the file's data. FileReader.readAsText(): Reads the contents of the specified input file. The result attribute contains the contents of the file as a text string. This method can take encoding version as the second argument(if required). The default encoding is UTF-8. Writing files using JavaScript and built-in extensions is straightforward: open the file for writing, write to a file and close a file. 1.
Using The File System Module In Node Js With Examples
Python Write To File Open Read Append And Other File
Reading Csv File Using Javascript And Html5
Looping Through A Data File In The Postman Collection Runner
Reading A Text File Using Javascript James D Mccaffrey
Python File Handling How To Create Text File Read Write
Node Js Fs Writefilesync Method Geeksforgeeks
Write And Read Data In Cypressio
Saving Text Json And Csv To A File In Python Geeksforgeeks
Javascript Write To File Code Example
Read Locally Txt File Use Fetch Method In Javascript By
How To Read Local Json File In React Js By Rajdeep Singh
The Ultimate Guide To Web Scraping With Node Js
Get Data From Text File Javascript Code Example
Javascript Project How To Create A To Do List Using
What Is Javascript Learn Web Development Mdn
How To Write In A Text File Using Javascript Code Example
Python Reading A Text File 101 Computing
Using Node Js To Read Really Really Large Datasets Amp Files
0 Response to "21 How To Write Data To A Text File In Javascript"
Post a Comment