25 How To Compare Dates In Javascript
Please enable JavaScript and reload the page. ... A common task to do as a frontend developer is to process Date objects, and frequently that means to compare them. Today, I am going to show you the best way to compare dates and some tips that will help you to get the results you expected. Compare two dates in JavaScript with various methods At first, we gonna start with the easiest method. Comparing two dates in JavaScript can be said like this: Compare two dates in terms of which is greater and which is smaller.
Moment Js Difference Between Two Dates Code Example
In this article, we're going to have a look at how to compare two dates in JavaScript. Quick solution: Copy. xxxxxxxxxx. 1. var date1 = new Date(2020, 10, 15); 2. var date2 = new Date(2020, 10, 15); 3.
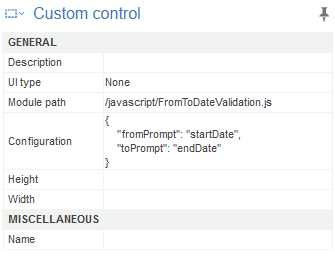
How to compare dates in javascript. var date1 = new Date('December ... //date 1 is newer } ... using javascript when i ' m iterate localstorage current input value my DOM its Add multiple value or perivous value of localstorage ? ... Install and run react js project... ... Error: Node Sass version 5.0.0 is incompatible with ^4.0.0. ... Access to XMLHttpRequest ... 1 week ago - Working with dates is something developers encounter very often. From creating to storing and processing dates, date manipulation in Javascript has many use cases in application development. In today’s tutorial, we thought to focus on one of them: comparing dates. Jan 02, 2021 - How to compare dates in Javascript. Compare dates with vanilla JS.
Feb 13, 2018 - To compare two dates with JavaScript, create two dates object and get the recent date. You can try to run the following code to compare two dates.Example Live ... Comparing date with getTime () A better approach to make comparison between dates is to use getTime () function. This function lets converting date into numeric value to directly compare them. Example1: Comparing current date and time with a given date and time. Checks if a date is the same as another date. Use Date.prototype.toISOString () and strict equality checking (===) to check if the first date is the same as the second one.
Comparing Two Dates in JavaScript We can use comparison operators like < and > two compare two Date objects, and under the hood, their time counters are effectively compared. You're effectively comparing two integer counters: Comparing dates with date-fns. So far, we have only relied on Javascript's native date object to compare dates. Even though it provided sufficient methods to execute basic comparisons, we had to use several lines of code to implement something more advanced, like comparing dates without time. Today, We want to share with you compare two dates in javascript.In this post we will show you convert the Date into a numeric value by using getTime() function, hear for By converting using js the given dates into numeric value we can directly compare we will give you demo and example for implement.In this post, we will learn about Calculating ...
28/1/2009 · The easiest way to compare dates in javascript is to first convert it to a Date object and then compare these date-objects. Below you find an object with three functions: dates pare(a,b) Returns a number:-1 if a < b; 0 if a = b; 1 if a > b; NaN if a or b is an illegal date; dates.inRange (d,start,end) Returns a boolean or NaN: Jan 28, 2020 - How to compare dates in JavaScript natively using the Date Object, without using any third-party libraries. 21/8/2021 · In this guide, I’m going to explain the simplest and most functional method to compare dates in JavaScript. Simplest Method to Compare Dates. In order to compare dates, you have to convert the data type values to numerical values. You can do that using the built in method getTime (). getTime Method Definition.
23/10/2020 · JavaScript Compare Two Dates With Comparison Operators We can directly compare two dates in JavaScript with comparison operators like < , <= , > and >= . var date1 = new Date('2020-10-23'); var date2 = new Date('2020-10-22'); console.log(date1 > date2); console.log(date1 >= date2); console.log(date1 < date2); console.log(date1 <= date2); In the above example, first we are constructing the dates with delimiter -then we are comparing the first date with second date, if both dates are equal it returns true else it returns false if it is not equal.. Second way using toDateString() method. Similarly, we can also compare two dates by using the toDateString() method which returns the date in English format "Mon Dec 16 2019". Nov 05, 2020 - Compare two string dates in JavaScript, ... two dates in javascript dd mm yyyy, compare date with current date in javascript, javascript compare date strings, javascript compare dates without time, how to check two dates are equal in javascript, angular 2 compare date with current ...
The following describes how to compare two dates: I have two dates. Date one is 15-01-2010 and another date is 15-01-2011. I compare these two dates using the following JavaScript code. <script type="text/javascript"language="javascript">. function CompareDate () {. var dateOne = new Date (2010, 00, 15); var dateTwo = new Date (2011, 00, 15); To compare the values of two dates, we need to check the first date is greater, less or equal than the second date. Using the Date object we can compare dates in JavaScript. Different ways to compare two dates in JavaScript. Compare two dates without time; Compare dates with time; Compare dates Using getTime() function; 1. Compare two dates ... 12/8/2021 · Comparing dates is something that can be a little confusing in JavaScript because you can’t just compare two Date objects because they are objects and as well all know {} !== {}. There are many different scenarios to comparing dates in JS, such as if you want to compare whole dates, days, hours, months, years, with or without time, the current date, and so on.
Most programming languages (including JavaScript) compare dates as numbers, so a later date is a larger number, and an earlier date is a smaller number. They usually can combine dates and times, as well, treating each date + time combination as a number, so 3:31 AM on January 17, 1832 is a smaller number than 7:15 PM on that same date. In JavaScript we can compare two dates by converting them to a numeric value to match the times, for this we use the getTime () function. By converting the specified dates into numeric values, we can compare them directly. We can use the relational operators below to compare two dates: < > <= >= As you have seen above, date and date1 variables are initialized at different time and that's why getTime() is returning different values for both. This is a numeric value and if we want to compare two Date, we can simply do it by comparing the values returning by the getTime() method. Javascript program :
JavaScript Date Output. By default, JavaScript will use the browser's time zone and display a date as a full text string: You will learn much more about how to display dates, later in this tutorial. Creating Date Objects. Date objects are created with the new Date() constructor. Complete JavaScript Date Reference. For a complete reference, go to our Complete JavaScript Date Reference. The reference contains descriptions and examples of all Date properties and methods. Test Yourself With Exercises. Exercise: Use the correct Date method to get the month (0-11) out of a date object. 1. You can set header to get formatted value if you are using Web API, "GET" request. 2. when you get the response then extract date value as "var crmDateValue= new Date (results.value ["new_datetime"])". 3. get current date value as "var today=new Date ();"
This is a short and simple guide on how to compare two dates in JavaScript. This is useful if you need to figure out which date is greater or which date occurred first. Firstly, let's create two JavaScript Date objects: //Example date one. var dateOne = new Date ('2019-01-01'); //Example date two. var dateTwo = new Date ('2012-03-02'); As you ... Sep 01, 2020 - Let's say we start with a date String and we want to calculate the difference in days to the current... Tagged with javascript, webdev, beginners. Mar 18, 2020 - Although neither == nor === can ... and > work fine for comparing dates: d1 < d2; // false d1 < d3; // false d2 < d1; // true · So to check if date a is before date b, you can just check a < b. Another neat trick: you can subtract dates in JavaScript....
You can compare two dates with JavaScript, you compare them using the >, <, <= or >= operators. This article will help you to resolve the following problems: Compare two dates with time. Compare today's date with any other date. JavaScript has a built-in Date Object we can use to access methods able to assist us in doing logic based on timestamps. To set this up, instantiate a new Date by coding the following: const date = new Date ( "09-20-2020" ); //2020-09-20T07:00:00.000Z. If you were to input console.log (date), the time (the substring after the T) will differ ... Create a JavaScript Function with the name age () this function will take your date of birth as parameters and return your age in years. ... how to write a program that displays a message “It’s Fun day” if it's Saturday or Sunday today in javascript
14/2/2021 · var date1 = new Date(2021, 02, 14); var date2 = new Date(2020, 02, 14); In order to compare dates, you can use the getTime() method and a strict equality comparison === . Note: The getTime method functions by returning the number of milliseconds between the midnight of 1/1/1970 and the supplied date. Have a couple of dates in Javascript that you need to compare? There are a few common ways to compare dates in Javascript: Parse the date strings into date objects and compare them directly using operators. var dateA = new Date ("1 Feb 2003"); Jun 10, 2020 - Create a JavaScript Function with the name age () this function will take your date of birth as parameters and return your age in years. ... javaScript Age in Dog years //write a function that takes your age and returns it to you in dog years - they say that 1 human year is equal to seven dog ...
1/1/2013 · You can use this to get the comparison: if (new Date(startDate) > new Date(endDate)) Using new Date(str) parses the value and converts it to a Date object. JavaScript's dates can be compared using the same comparison operators the rest of the data types use: >, <, <=, >=, ==, !=, ===, !==. If you have two dates A and B, then A < B if A is further back into the past than B. But it sounds like what you're having trouble with is turning a string into a date. In JavaScript, we can compare two dates by converting them into numeric value to corresponding to its time. First, we can convert the Date into a numeric value by using getTime () function. By converting the given dates into numeric value we can directly compare them. Example-1:
Create a JavaScript Function with the name age () this function will take your date of birth as parameters and return your age in years. ... javaScript Age in Dog years //write a function that takes your age and returns it to you in dog years - they say that 1 human year is equal to seven dog ... Javascript Object Oriented Programming Front End Technology Dates can be compared easily in javascript. The dates can belong to any frame i.e past, present and future. Past dates can be compared to future or future dates can be compared to the present. The new Date () gives the current date and time. You can then directly compare these two dates using comparison operators >, <, <=, or >=. However, to use comparison operators like ==, !=, ===, or !==, you have to use date.getTime (). The getTime () method returns the number of milliseconds from midnight of January 1, 1970 (EcmaScript epoch) to ...
How To Check If One Date Is Between Two Dates In Javascript
Custom Javascript In Cognos Analytics Date Prompts And
How To Compare Two Dates In Javascript Atomized Objects
Date Manipulation In Javascript A Complete Guide
You Can Compare Dates In Javascript By Filip Vitas Medium
How To Compare Dates In Php Simple Examples
Compare Dates In Javascript Javascript Helperbyte
How To Manipulate Date And Time In Javascript
Calculate Age Using Javascript Javatpoint
Date Functions In Tableau Time To Manipulate Date Values
Javascript Date And Time In Detail By Javascript Jeep
Javascript Date Difference Javatpoint
Eca 225 Applied Online Programming Javascript Dates Eca
How To Compare Two Dates In Javascript
How To Calculate Minutes Between Two Dates In Javascript
How To Compare Dates In Javascript Code Example
Sql Date Format Overview Datediff Sql Function Dateadd Sql
Javascript Date Comparison Quick And Simple Udemy Blog
How To Compare Two Dates In Javascript
Selenium Tips Comparing Some Dates
Everything You Need To Know About Date In Javascript Css Tricks
Github Guardian Compare Dates Simple Modular Utilities To
3 Ways To Compare Dates In Javascript Simple Examples
How To Work With Date And Time In Javascript Using Date
0 Response to "25 How To Compare Dates In Javascript"
Post a Comment