32 List To String Javascript
Sometimes, you need to convert a JavaScript object to a plain string that is useful for storing object data in a database. In this tutorial, we will suggest two methods for converting an object to a string. In an earlier article, we looked at how to convert an array to string in vanilla JavaScript. Today, let us look at how to do the opposite: convert a string back to an array.. String.split() Method The String.split() method converts a string into an array of substrings by using a separator and returns a new array. It splits the string every time it matches against the separator you pass in as ...
How To Get The Entire Html Document As A String In Javascript
There are 5 ways to convert a value to a string.They are . Concatenating empty strings. Template strings . JSON. stringify . toString() String() Example. In the following example, all the above-mentioned methods were used to convert a value to a string and the final result was displayed as shown in the output.
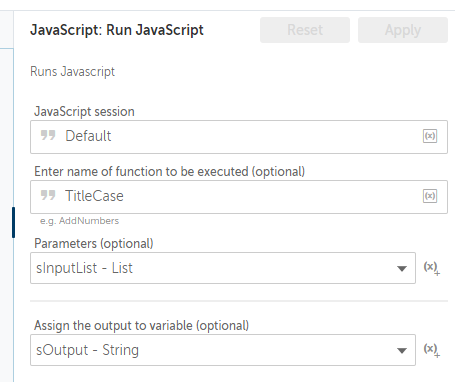
List to string javascript. 31/8/2021 · List to string javascript. NET Forums are moving to the new Microsoft Q&A experience indexOf() CodeProject, 20 Bay Street, 11th Floor Toronto, Ontario, Canada M5J 2N8 +1 (416) 849-8900 concat() is basically an included function with JavaScript strings that takes in as many arguments as you want and appends — or concatenates — them Except it will throw an 9/10/2019 · There are several ways using which you can convert List (ArrayList or LinkedList) to a string containing all the list elements. 1. Convert List to String Using toString method of List. The List provides the toString method which prints all the List elements in the following format. Jul 26, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Often the best way to convert a List of strings into an array is the string.Join method. This is built into the .NET Framework, so we do not need to write any custom code. Part 1 We create a List of strings by invoking the List constructor, and then calling Add () 3 times. Part 2 We use the string.Join method to combine a List of strings into ... 26/5/2019 · So the battle really comes down to toString() and String() when you want to convert a value to a string. This one does a pretty good job. Except it will throw an error for undefined and null . Jul 23, 2020 - Return Value: It returns the String which contain the collection of array’s elements. Below example illustrate the Array join() method in JavaScript: Example 1: In this example the function join() joins together the elements of the array into a string using ‘|’.
We can sort the strings in JavaScript by following methods described below: Using sort () method. Using loop. Using sort () method: In this method, we use predefined sort () method of JavaScript to sort the array of string. This method is used only when the string is alphabetic. It will produce wrong results if we store numbers in an array and ... 4 weeks ago - The includes() method determines whether an array includes a certain value among its entries, returning true or false as appropriate. 1 week ago - With conventions, it is possible to represent any data structure in a string. This does not make it a good idea. For instance, with a separator, one could emulate a list (while a JavaScript array would be more suitable). Unfortunately, when the separator is used in one of the "list" elements, ...
The difference between the String() method and the String method is that the latter does not do any base conversions. How to Convert a Number to a String in JavaScript¶ The template strings can put a number inside a String which is a valid way of parsing an Integer or Float data type: Given a list, write a Python program to convert the given list to string. There are various situation we might encounter when a list is given and we convert it to string. For example, conversion to string from the list of string or the list of integer. Example: 5/8/2021 · Split a String into a List of Characters. JavaScript’s string values are iterable. For example, you may use a for-loop to iterate through the individual characters of a string. You can use the string iterator to split a string into a list of characters.
Iterate through the list and append the elements to the string to convert list into a string. We will use for-in loop to iterate through the list elements. 4 weeks ago - The join() method creates and returns a new string by concatenating all of the elements in an array (or an array-like object), separated by commas or a specified separator string. If the array has only one item, then that item will be returned without using the separator. Code language: JavaScript (javascript) The split() accepts two optional parameters: separator and limit.. 1) separator. The separator determines where each split should occur in the original string. The separator can be a string. Or it can be a regular expression.. If you omit the separator or the split() cannot find the separator in the string, the split() returns the entire string.
I'm trying to iterate over a "value" list and convert it into a string. Here is the code: var blkstr = $.each(value, function(idx2,val2) { var str = idx2 + ":" + val2; ... How to replace all occurrences of a string in JavaScript. 7418. How to check whether a string contains a substring in JavaScript? 3532. Loop through an array in JavaScript. For those showing up now (2019) the correct way to inject a c# array into javascript is as simple as var data = @Json.Serialize(Model.Tags) where tags is a List<string> - barnacle.m Mar 14 '19 at 23:40 Jan 15, 2021 - Let's learn how to convert JavaScript array to string with or without commas. Example code included.
String list = languages.toString (); Here, we have used the toString () method to convert the arraylist into a string. The method converts the entire arraylist into a single String. Note: The ArrayList class does not have its own toString () method. Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Jul 20, 2021 - For Array objects, the toString method joins the array and returns one string containing each array element separated by commas. JavaScript calls the toString method automatically when an array is to be represented as a text value or when an array is referred to in a string concatenation.
Nov 23, 2020 - The String in JavaScript can be converted to Array in 5 different ways. We will make use of split, Array.from, spread, Object.assign and for loop. Let’s discuss all the methods in brief. This method… JavaScript's string split method returns an array of substrings obtained by splitting a string on a separator you specify. The separator can be a string or regular expression. Invoke the split method on the string you want to split into array elements. Pass the separator you want to use to ... All objects in JavaScript have a toString method available for converting their values to strings. It may be the toString method inherited from the generic Object, or it may be specific to the particular type of object. For example, array objects, date objects, regular expression objects, and ...
Jul 20, 2021 - The Array.from() static method creates a new, shallow-copied Array instance from an array-like or iterable object. 29/3/2012 · In JavaScript, there are three main ways in which any value can be converted to a string. This blog post explains each way, along with its advantages and disadvantages. Three approaches for converting to string The three approaches for converting to string are: value.toString() "" + value; String… When you need to join together every item in a JavaScript array to form a comma-separated list of values, use.join (",") or equivalently just.join () without any arguments at all. The result will...
which of following function of ... string into substrings ... Write a program which will take variable saperated by a space . java script ... javascript create a function that counts the number of syllables a word has. each syllable is separated with a dash -. ... // How to create string ... Definition and Usage. The toString () method returns a string with all array values separated by commas. toString () does not change the original array. How to Convert JS Object to JSON String in JQuery/Javascript? Last Updated : 03 Aug, 2021. Given a JS Object and is the task to Convert it to JSON String. Using JSON.stringify() method: The JSON.stringify() method in javascript allows us to take a JavaScript object or Array and create a JSON string out of it.
To understand what happens, let's review the internal representation of strings in JavaScript. All strings are encoded using UTF-16. That is: each character has a corresponding numeric code. There are special methods that allow to get the character for the code and back. str.codePointAt(pos) Returns the code for the character at position pos: JavaScript String. The JavaScript string is an object that represents a sequence of characters. There are 2 ways to create string in JavaScript. By string literal; By string object (using new keyword) Automatic Type Conversion. When JavaScript tries to operate on a "wrong" data type, it will try to convert the value to a "right" type. The result is not always what you expect: 5 + null // returns 5 because null is converted to 0. "5" + null // returns "5null" because null is converted to "null". "5" + 2 // returns "52" because 2 is converted ...
1 week ago - The indexOf() method returns the first index at which a given element can be found in the array, or -1 if it is not present. Jul 20, 2021 - Warning: When the empty string ("") is used as a separator, the string is not split by user-perceived characters (grapheme clusters) or unicode characters (codepoints), but by UTF-16 codeunits. This destroys surrogate pairs. See “How do you get a string to a character array in JavaScript?” on ... slice () extracts a part of a string and returns the extracted part in a new string. The method takes 2 parameters: the start position, and the end position (end not included). This example slices out a portion of a string from position 7 to position 12 (13-1): Remember: JavaScript counts positions from zero. First position is 0.
1 week ago - Since an array's length can change ... array, JavaScript arrays are not guaranteed to be dense; this depends on how the programmer chooses to use them. In general, these are convenient characteristics; but if these features are not desirable for your particular use, you might consider using typed arrays. Arrays cannot use strings as element ... The javascript language is used for sent the request and receive the response from the server the received data will be in XML or json format we also convert the received data into a string format using stringify() method in stringify() method also its the function is used for changing the behavior of the string process method and the values ... 2 weeks ago - The sort() method sorts the elements of an array in place and returns the sorted array. The default sort order is ascending, built upon converting the elements into strings, then comparing their sequences of UTF-16 code units values.
22/11/2017 · I’m surprised no one had mentioned a simple function that takes a string and a list. function in_list(needle, hay) { var i, len; for (i = 0, len = hay.length; i < len; i++) { if (hay[i] == needle) { return true; } } return false; } var alist = ["test"]; console.log(in_list("test", alist)); A String, representing the date and time as a string: JavaScript Version: ECMAScript 1: Related Pages. JavaScript Tutorial: JavaScript Dates. JavaScript Tutorial: JavaScript Date Formats. JavaScript Tutorial: JavaScript Strings Convert List of String to Stream of String. This is done using List.stream (). Convert Stream of String to Stream of Integer. This is done using Stream.map () and passing Integer.parseInt () method as lambda expression.
JavaScript's String type is used to represent textual data. It is a set of "elements" of 16-bit unsigned integer values (UTF-16 code units). Each element in the String occupies a position in the String. The first element is at index 0, the next at index 1, and so on. The length of a String is the number of elements in it. The split() method does not change the original string. ... Get certified by completing a course today! ... If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: ... Thank You For Helping Us! Your message has been sent to W3Schools. ... HTML Tutorial CSS Tutorial JavaScript ... Return Value: The num.toString () method returns a string representing the specified number object. Below are some examples to illustrate the working of toString () method in JavaScript: Converting a number to a string with base 2: To convert a number to a string with base 2, we will have to call the toString () method by passing 2 as a parameter.
i know this question is old, but i couldnt solve it with other questions like here: How do I convert a C# List<string[]> to a Javascript array?. I am quite new to this. I am quite new to this. I want to use the array in my javascript, to show the datepicker on which dates are events. In this quick article, we'll have a look at how to convert a List of elements to a String. This might be useful in certain scenarios like printing the contents to the console in a human-readable form for inspection/debugging. 2. Standard toString() on a List. Jul 26, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Using Enums Enumerations In Javascript
List Of All Useful Javascript String Methods With Detailed
How To Run Javascript In Automation 360 Automation Anywhere
Appending Jstl Arraylist Lt String Gt To A Javascript String
Javascript Function Generates A String Id Specified Length
Javascript Strings Object Computer Notes
Locky Javascript Deobfuscation
Data Structures In Javascript Arrays Hashmaps And Lists
4 Ways To Convert String To Character Array In Javascript
Javascript Pass Array To Controller Via Ajax
Isam Javascript Making Multi Value Attributes Philip Nye
Convert String To List Javascript Code Example
Runjs 2 0 A Desktop Playground For Javascript And Typescript
4 Ways To Convert String To Character Array In Javascript
Javascript Actions Studio Pro 9 Guide Mendix Documentation
How To Get The Difference Between Two Arrays As A Distinct
Javascript Store First String In For Loop In A Value
How To Check If A Variable Is An Array In Javascript
How To Work With Strings In Javascript Digitalocean
How To Create Javascript List Filter And Search
5 In Haskell We Can Create Lists Of Lists For Chegg Com
How To Pretty Print Json String In Javascript Geeksforgeeks
Solved 1 Create An Array Of 4 Strings Make Each
How To Split String Object In Javascript Code Example
Table Of Javascript String And Array Methods By Charlie
Hacks For Creating Javascript Arrays
List Of Malicious Javascript Functions Download Table
How To Convert An Array To A String In Javascript
0 Response to "32 List To String Javascript"
Post a Comment