31 For In Javascript Index
13/7/2016 · One can access the index Array.prototype.map()via the second argument of the callback function. Here is an example: const array = [1, 2, 3, 4];const map = array.map((x, index) => { console.log(index); return x + index;});console.log(map); Other arguments of Array.prototype.map(): The findIndex () method returns the index of the first array element that passes a test (provided by a function). The method executes the function once for each element present in the array:
Using Javascript To Debug Fusion Lucidworks
JavaScript indexOf () method used to find the occurrence of an item in an array. Method name indicates index means sequence values position. So, the indexOf () method always used with an array. indexOf () position always starts with 0 and ends with N-1. N is an array length.
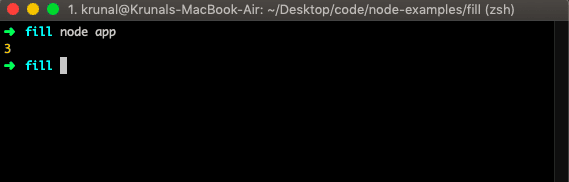
For in javascript index. Below examples illustrate the Array findIndex () function in JavaScript: Example 1: In this example the method findIndex () finds all the indices that contain odd numbers. Since no odd numbers are present therefore it returns -1. function isOdd (element, index, array) { return (element%2 == 1); } print ( [4, 6, 8, 12].findIndex (isOdd)); Conceptually, arrays in JavaScript contain array.length elements, starting with array up until array [array.length - 1]. An array element with index i is defined to be part of the array if i is between 0 and array.length - 1 inclusive. If i is not in this range it's not in the array. Definition and Usage The indexOf () method returns the position of the first occurrence of a specified value in a string. indexOf () returns -1 if the value is not found. indexOf () is case sensitive.
array.forEach(callback( currentVal [, index [, array]])[, thisVal]) The callback function accepts between one and three arguments: currentVal — The value of the current element in the loop. index — The array index of the current element. array — The array object the forEach () loop was called upon. Only the first argument is required. If you need the index of the found element in the array, use findIndex().; If you need to find the index of a value, use Array.prototype.indexOf(). (It's similar to findIndex(), but checks each element for equality with the value instead of using a testing function.); If you need to find if a value exists in an array, use Array.prototype.includes(). 24/2/2009 · Append multiple elements at a specific index // Append from index number 1 arrName.splice(1, 0, 'newElemenet1', 'newElemenet2', 'newElemenet3'); // 1: index number from where append start, // 0: number of element to remove, //newElemenet1,2,3: new elements
Definition and Usage The lastIndexOf () method returns the position of the last occurrence of a specified value in a string. lastIndexOf () searches the string from the end to the beginning, but returns the index s from the beginning, starting at position 0. lastIndexOf () returns -1 if the value is not found. In JavaScript, objects use named indexes. Arrays are a special kind of objects, with numbered indexes. When to Use Arrays. When to use Objects. JavaScript. The arr.filter () method is used to create a new array from a given array consisting of only those elements from the given array which satisfy a condition set by the argument method. Parameters: This method accepts five parameter as mentioned above and described below: callback: This parameter holds the function to be called for ...
At the implementation level, JavaScript's arrays actually store their elements as standard object properties, using the array index as the property name. The length property is special. It always returns the index of the last element plus one. (In the example below, 'Dusty' is indexed at 30, so cats.length returns 30 + 1). 4/3/2020 · When looping through an array in JavaScript, it may be useful sometimes to get the index of every item in the array. This is possible with .forEach method, let's take an example to illustrate it: const apps = ["Calculator", "Messaging", "Clock"]; apps. forEach ((app, index) => {console. log (app, index);}); The output of this code is: "Calculator" 0 "Messaging" 1 Each character in a JavaScript string can be accessed by an index number, and all strings have methods and properties available to them. In this tutorial, we will learn the difference between string primitives and the String object, how strings are indexed, how to access characters in a string, and common properties and methods used on strings.
for…initerates over property names, not values, and does so in an unspecified order(yes, even after ES6). You shouldn't use it to iterate over arrays. For them, there's ES5's forEachmethod that passes both the value and the index to the function you give it: var myArray = [123, 15, 187, 32]; Javascript - Use Variable as Array Index. Related. 7626. How do JavaScript closures work? 6728. How do I remove a property from a JavaScript object? 4435. How do I check if an array includes a value in JavaScript? 3489. How to insert an item into an array at a specific index (JavaScript) 5724. In this tutorial, we will learn about the JavaScript Array indexOf () method with the help of examples. The indexOf () method returns the first index of occurance of an array element, or -1 if it is not found.
In the above code, we first find the index of the element we want to remove and then use the splice() method to remove the array element. Use Array.filter() to Remove a Specific Element From JavaScript Array The findIndex () method executes the callbackFn function once for every index in the array until it finds the one where callbackFn returns a truthy value. If such an element is found, findIndex () immediately returns the element's index. If callbackFn never returns a truthy value (or the array's length is 0), findIndex () returns -1. Returns a new Array Iterator that contains the keys for each index in the array. Array.prototype.lastIndexOf() Returns the last (greatest) index of an element within the array equal to an element, or -1 if none is found. Array.prototype.map() Returns a new array containing the results of calling a function on every element in this array.
IndexedDB is a database that is built into a browser, much more powerful than localStorage. Stores almost any kind of values by keys, multiple key types. Supports transactions for reliability. Supports key range queries, indexes. Can store much bigger volumes of data than localStorage. That power is usually excessive for traditional client ... Ready to try JavaScript? Begin learning here by typing in your first name surrounded by quotation marks, and ending with a semicolon. For example, you could type the name "Jamie"; and then hit enter. This can be achieved using the zIndex concept. JavaScript zIndex is a property used to set or get stack order of positioned element. Based on the zIndex value, elements get positioned on front of another element or at the back of the other element. Greater the zIndex, target element is in front of another element which has lower zIndex relatively.
It is not comprehensive for learning -. all there is for programming JavaScript. The premise is to familiarize yourself with the code if you have never seen it, and serve as a brush up if you have used it. This is my personal library that I am willing to share. Return to Table of Contents. DELAWARE'S MIDDLETOWN ODESSA AND TOWNSEND AREA. JavaScript Map Index. To find index of the current iterable inside the JavaScript map() function, you have to use the callback function's second parameter. An index used inside the JavaScript map() method is to state the position of each item in an array, but it doesn't modify the original array. Syntax The indexOf () method returns the first index at which a given element can be found in the array, or -1 if it is not present.
Update the value of each element to 10 times the original value: const numbers = [65, 44, 12, 4]; numbers.forEach(myFunction) function myFunction (item, index, arr) {. arr [index] = item * 10; } 4/3/2017 · Getting value out of maps that are treated like collections is always something I have to remind myself how to do properly. In this post I look at JavaScript object iteration and picking out values from a JavaScript object by property name or index.
How to get index in a for-of loop in JavaScript. javascript1min read. In this tutorial, we are going to learn about how to get the index in a for-of loop. Normally, if we loop through an array using for-of loop we only get the values instead of index. Get The Current Array Index in JavaScript forEach () JavaScript's forEach () function takes a callback as a parameter, and calls that callback for each element of the array: The first parameter to the callback is the array value. The 2nd parameter is the array index. That's the current position in the array the forEach () loop is at. An array in JavaScript is a type of global object that is used to store data. Arrays consist of an ordered collection or list containing zero or more datatypes, and use numbered indices starting from 0 to access specific items.
The index of the first occurrence of searchValue in the string, or -1 if not found. If an empty string is passed as searchValue, it will match at any index between 0 and str.length. Examples. In JavaScript, the first character in a string has an index of 0, and the index of the last character is str.length - 1.
Javascript Z Index Examples Of Javascript Z Index
Javascript Array Indexof And Lastindexof Locating An Element
Javascript Characters Learn To Play With Characters In Js
How To Obtain The Sum Of Integers Between 2 Indexes In The
How To Get The Index Of An Object In An Array In Javascript
Beautiful Javascript Charts 10x Fast 30 Js Chart Types
Javascript Array Methods Foreach Vs Map Chiamaka Ikeanyi
Fun With Javascript Native Array Functions Modern Web
Creating Indexing Modifying Looping Through Javascript
Javascript Array Methods How To Use Map And Reduce
Javascript Link Seo Test Result
Javascript Basic Check Whether A String Script Presents At
Javascript Get The Index Of An Object By Its Property
How To Get The Index Of An Array That Contains Objects In
Can Google Index Javascript Rendered Links Before Screenshots
How To Replace A Character At A Particular Index In
Javascript Map Index How To Use Index Inside Map
Implementing Our Own Array Map Method In Javascript Dev
Negative Indexes In Javascript Arrays Using Proxies By
Get Loop Counter Index Using For Of Syntax In Javascript
Javascript Programming With Visual Studio Code
Javascript String Indexof How To Get Index Of String
Javascript Fundamental Es6 Syntax Get The Lowest Index At
Get Array Element Value With Index In Javascript
How To Get The Index Of An Item In A Javascript Array
How To Find The Index Of All Occurrence Of Elements In An
How To Use Indexof On Array Of Object In Javascript Code Example
Seo For Progressive Web Apps Pwa And Javascript Sites
What Is Indexof In Javascript Javascript String Indexof
0 Response to "31 For In Javascript Index"
Post a Comment