25 Javascript Documentation Optional Parameter
This can be seen as a call by reference: although a function cannot change the caller's object by assigning a new value to it, it could mutate the caller's object. As primitive valued arguments, like numbers or strings, are immutable, there is no way for a function to mutate them: In JavaScript, the pattern for named parameters shown here is sometimes called options or option object (e.g., by the jQuery documentation).
Aug 30, 2018 - explains how to use optional arguments with your functions.
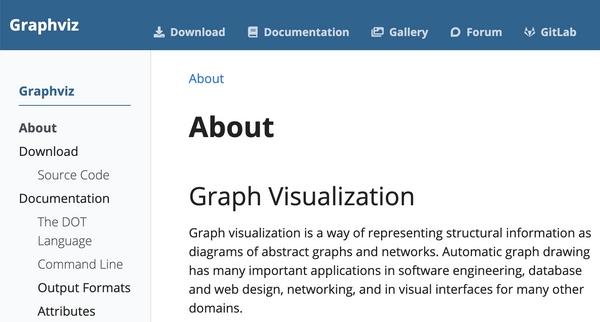
Javascript documentation optional parameter. May 28, 2021 - Let’s look at some more examples to learn some available options for setting default values of the function parameters. ... The following createDiv() function creates a new <div> element in the document with a specific height, width, and border-style: The syntax (a: string) => void means "a function with one parameter, named a, of type string, that doesn't have a return value".Just like with function declarations, if a parameter type isn't specified, it's implicitly any.. Note that the parameter name is required.The function type (string) => void means "a function with a parameter named string of type any"! For functions and methods, you can document parameters, return values, and exceptions they may throw: ... Describes the parameter whose name is paramName. Type and description are optional. Here are some examples:
Jul 28, 2019 - TypeScript is a superset of JavaScript that compiles to clean JavaScript output. - JSDoc support in JavaScript · microsoft/TypeScript Wiki May 28, 2020 - There are a few subtleties, which are all far better documented at MDN's JavaScript Default Parameters docs. The rest of the blogpost is obsolete, but kept below for posterity. ... I've been reading a lot of JavaScript code lately, and it's been a joy. I'm picking up on some common JavaScript idioms too, and I thought I'd share. ... Optional ... May 31, 2019 - JavaScript optional parameters allows Java developers to declare optional parameters. This article describes different ways to pass optional parameters in JavaScript.
The arguments object is a local variable available within all non-arrow functions. You can refer to a function's arguments inside that function by using its arguments object. It has entries for each argument the function was called with, with the first entry's index at 0.. For example, if a function is passed 3 arguments, you can access them as follows: Aug 02, 2020 - Warning: This page has been moved herePlease do not edit this page, use edit on the new page. WordPress follows the JSDoc 3 standard for inline JavaScript documentation. What Should … Nov 24, 2016 - While separate parameters are a good starting point, we run into trouble as soon as the function has optional parameters. For functions with a single optional parameter, the solution is simple:
2/3/2021 · In the example the optional parameter is ‘b’: The optional parameter is Logically OR with the default value within the body of the function and should always come at the end of the parameter list. Pre ES2015, Functions that accept configurations or options with a lot of optional parameters Functions that change often because it doesn't require changing every call site, only the ones that use the specific parameter. The next time you run into one of the earlier stated problems or one of these scenarios, consider reaching for named parameters. function documentation Any function can be declared with function name return types and accepted input types. @param tag provides parameters for a javascript function. This includes parameter type in open and close braces and Description of a parameter Type of the accepted parameter are string or Object or namespace pointed to code.
The optional chaining operator provides a way to simplify accessing values through connected objects when it's possible that a reference or function may be undefined or null. For example, consider an object obj which has a nested structure. @param uses the same type syntax as @type, but adds a parameter name. The parameter may also be declared optional by surrounding the name with square brackets: // Parameters may be declared in a variety of syntactic forms Optional parameter used to set the page item's display value, in the case where the return value is different. For example for the item type "Popup LOV", with the attribute "Input Field" = "Not Enterable, Show Display Value and Store Return Value", this value sets the "Input Field".
An API key is passed as the key parameter in the URL that is used to load the Maps JavaScript API. Here are a few options to check if you are using an API key: Use the Google Maps Platform API Checker Chrome extension. This allows you to determine if your website is properly implementing Google's licensed Maps APIs. Apr 26, 2017 - It's pretty common to see JavaScript libraries to do a bunch of checks on optional inputs before the function actually starts. A container object which may or may not contain a non-null value. If a value is present, isPresent() will return true and get() will return the value. Additional methods that depend on the presence or absence of a contained value are provided, such as orElse() (return a default value if value not present) and ifPresent() (execute a block of code if the value is present).
Another possibility is to hand in optional parameters as named parameters, as properties of an object literal (see Named Parameters). Simulating Pass-by-Reference Parameters In JavaScript, you cannot pass parameters by reference; that is, if you pass a variable to a function, its value is copied and handed to the function (pass by value). 36 Javascript Documentation Optional Parameter Written By Roger B Welker. Saturday, August 14, 2021 Add Comment Edit. Javascript documentation optional parameter. Jsdoc Javascript Documentation Tutorials With Examples. Invoke Javascript. Documentation Anime Js. Refactoring Javascript Webstorm. Apr 03, 2015 - Blog posts about programming, mostly JS and frontend, but a few other topics as well.
typescript optional parametertypescript optional parameter or default valuetypescript omit optional parameterstypescriptparameters All parameters of a function are required by default. The parameters of a function should match the type specified by the function signature as well. From the documentation for addEventListener I see the following pattern: target.addEventListener (type, listener [, useCapture]); Now I understand that useCapture is an optional parameter. Why does the [ then start before the comma (,) and not immediately after the comma that follows the listener parameter? There are three main differences between rest parameters and the arguments object: The arguments object is not a real array, while rest parameters are Array instances, meaning methods like sort, map, forEach or pop can be applied on it directly; ; The arguments object has additional functionality specific to itself (like the callee property).
Speaking of JavaScript, we can use a documentation layer called, JSDoc. It's a command line tool and a "documentation language" at the same time. Let's see how it can helps. JavaScript With JSDoc: first steps. JSDoc is a nice "language" for adding documentation to JavaScript. Consider the following function: Jul 11, 2017 - If you can destructure function parameters, and you can assign default values during that destructuring, this means that you can simulate named and optional arguments in JavaScript. What are Optional Parameters? By definition, an Optional Parameter is a handy feature that enables programmers to pass less number of parameters to a function and assign a default value. Firstly, let us first understand what the word Optional Parameter means. Parameters are the names listed in the function definition.
TypeScript 3.0 Release Notes. In the declaration of f2 above, type inference infers types number, [string, boolean] and void for T, U and V respectively.. Note that when a tuple type is inferred from a sequence of parameters and later expanded into a parameter list, as is the case for U, the original parameter names are used in the expansion (however, the names have no semantic meaning and are ... Pass optional parameters in an options object. Alternatively, you can create an object named options that specifies the optional parameters separately from the method call, and then pass the options object as the options argument.. The following example shows one way of creating the options object, where parameter1, value1, and so on, are placeholders for the actual parameter names and values. Apr 08, 2020 - To add JavaScript code inline documentation, follow these guidelines. Some parts of Magento code may not comply with this standard, but we are working to gradually improve this. Following these standard is optional for 3rd-party Magento developers, but will help to create consistent, clean, ...
The following examples show how to indicate that a parameter is optional and has a default value. An optional parameter (using JSDoc syntax) /** * @param {string} [somebody] - Somebody's name. */function sayHello(somebody) { if (!somebody) { somebody = 'John Doe'; } alert('Hello ' + somebody);} Definition and Usage. The setTimeout() method calls a function or evaluates an expression after a specified number of milliseconds. Tip: 1000 ms = 1 second. Tip: The function is only executed once. If you need to repeat execution, use the setInterval() method.. Tip: Use the clearTimeout() method to prevent the function from running. From official documentation: Optional parameter. An optional parameter named foo. @param {number} [foo] // or: @param {number=} foo An optional parameter foo with default value 1. @param {number} [foo=1]
Sep 22, 2019 - How many different types of JS alerts do we have · How to check whether a checkbox is checked in jQuery · Access to XMLHttpRequest at 'http://localhost:5000/mlphoto' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on ... Jun 10, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Jul 22, 2019 - Composing functions together like this is generally easier to use and doesn't require users to remember various options or always be referring to the documentation. ... There are a few drawbacks to using this method that we should address. ... In JavaScript objects are references and the spread ...
This is unless we use optional arguments. In which case, we can specify fewer arguments than a function accepts because some arguments are optional. Python Optional Arguments. A Python optional argument is an argument with a default value. You can specify a default value for an argument using the assignment operator. console.log () The console.log () method outputs a message to the web console. The message may be a single string (with optional substitution values), or it may be any one or more JavaScript objects. Note: This feature is available in Web Workers. The one-page guide to Jsdoc: usage, examples, links, snippets, and more.
Default Parameters. Same as JavaScript ES6 default parameters, TypeScript also supports default function parameter.In TypeScript, that even works for pre ES6 versions. default-params.ts function test(x: number, y: number = 3): void { console.log(`x= ${x}, y=${y}`); } test(2); test(2, 5); The parameters, in a function call, are the function's arguments. JavaScript arguments are passed by value: The function only gets to know the values, not the argument's locations. If a function changes an argument's value, it does not change the parameter's original value. Changes to arguments are not visible (reflected) outside the function. In JavaScript, function parameters default to undefined. However, it's often useful to set a different default value. This is where default parameters can help. In the past, the general strategy for setting defaults was to test parameter values in the function body and assign a value if they are undefined.
Optional Parameters In Javascript
Javascript Function Optional Parameter Code Example
Writing Your Own Aws Systems Manager Documents Aws
Declare Default Value For Jsdoc Code Example
Optional Parameters In Java Common Strategies And Approaches
Understanding Default Parameters In Javascript Digitalocean
Export With Mapping Studio Pro 9 Guide Mendix Documentation
Chapter 11 Building Map Based Applications Xpressworkx
Apache Jmeter User S Manual Functions And Variables
Chapter 29 Jsdoc Generating Api Documentation
Photoshop Cc Javascript Ref 2019 Pages 51 100 Flip Pdf
Here Are The Most Popular Ways To Make An Http Request In
Parameters From Json Openapi 3 0 Schema Not Displaying In
Javascript Rest Parameter Geeksforgeeks
Understanding Args And Kwargs Arguments In Python
Mocha The Fun Simple Flexible Javascript Test Framework
Angularjs 1 X Cheat Sheet By Deleted Download Free From
0 Response to "25 Javascript Documentation Optional Parameter"
Post a Comment