23 Password Length Validation Javascript
var length = document.getElementById("length"); // When the user clicks on the password field, show the message box. myInput.onfocus = function() {. document.getElementById("message").style.display = "block"; } // When the user clicks outside of the password … Password Regexp Test (input must contain at least one digit/lowercase/uppercase letter and be at least six characters long) If you want to restrict the password to ONLY letters and numbers (no spaces or other characters) then only a slight change is required. Instead of using. (the wildcard) we use \w:
Solutions For Javascript Maxlength Check Codeproject
validate password length after user leave password field. Ask Question Asked 7 years, 5 months ago. Active 4 years, 6 months ago. Viewed 22k times 1 I want to check if the password length is at least 8 characters or not, when the user leaves the password field or press tab key. ... Browse other questions tagged javascript jquery passwords or ...
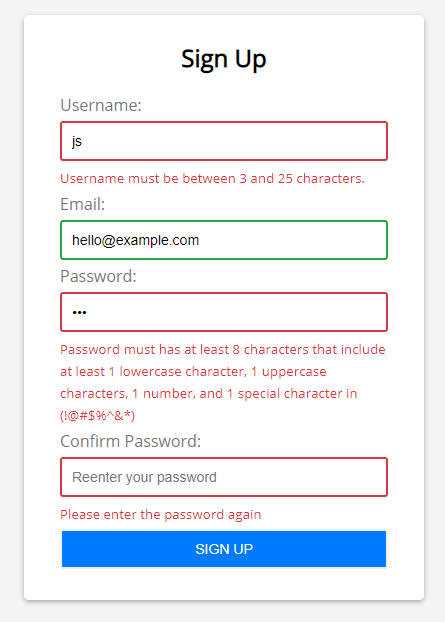
Password length validation javascript. In this tutorial, we are going to validate the name and password. The name can't be empty and password can't be less than 7 characters long.Here, we are vali... 21/4/2013 · You can use a regular expression and check the length. The regular expression is a case insensitive check for one letter and one number in any order. The length must be greater than 3. function testString(s) { var re = /[a-z]\d|\d[a-z]/i; return re.test(s) && s.length > 3; } The password must have a minimum and maximum length. Password validation in JavaScript ensures that these parameters are followed when the user creates a password. The given conditions must be met for the formation of a reasonably strong password. The structure of the password can be validated by regular expression using JavaScript code.
Tutorial: Limit Password Length Using JavaScript with Source Code. In this article we will try to do Limit Password Length using JavaScript. The code can restrict the inputted user password. The trick is you need to compare the length of textbox and the possible maximum length in the if statement. To learn more about this, just follow the steps ... The task is to validate the password using HTML and JavaScript. A password is correct if it contains: At least 1 uppercase character. At least 1 lowercase character. At least 1 digit. At least 1 special characte r. Minimum 8 characters. In this chapter, we will discuss password validation using JavaScript. We need to validate a password every time whenever a user creates an account on any website or app. So, we have to verify a valid password as well as put the confirm password validation. For a valid password, the following parameters must be contained by it to be valid -
This tutorial is about JavaScript form validation with limit login attempts. In which validation function comes into act to authenticate username and password. Steps to Password Validation. Get The Input Elements. The first step will be to grab the input elements and store them into variables. This will allow us to work with the inputs throughout the script. Capturing The Event. We want to check the values of the inputs every time someone releases a key in one of the input boxes. JavaScript provides facility to validate the form on the client-side so data processing will be faster than server-side validation. Most of the web developers prefer JavaScript form validation. Through JavaScript, we can validate name, password, email, date, mobile numbers and more fields. JavaScript Form Validation Example
Program for Setting the password length in JavaScript There are many other methods to validate the length of the password. You can also validate the length using regular expression and JQuery. Hopefully, now, you are able to write a program for validating the password length in javascript. Password Length Validation by Javascript and Custom Validator. Posted on March 1, 2011 Updated on March 1, 2011. I've to validate the minimum length of password to be 6. So i used a Custome Validator and a Javascript method to do so. Hope that helps 🙂. Html Code for Customer Validator: Password validation in JavaScript We'll talk about how to use JavaScript to validate passwords. Every time a user establishes an account on a website or app, we must confirm the password. As a result, we must validate a valid password as well as enable password validation.
We store this input in password variable and check it's length. Since many password checkers have length constraint, we take 8 here. If the length is greater than 8, then we make the length parameter true in strengthValue. Then we move on to check the string for strength metrics. JavaScript code: For strong validation i mentioned here the condition is at least one capital letter and one small letter and one number and one special character then it shows the strong password and also i mentioned here the minimum character length should be 8 character using html5 pattern. For example a userid (length between 6 to 10 character) or password (length between 8 to 14 characters). You can write a JavaScript form validation script where the required field (s) in the HTML form accepts the restricted number of characters. Javascript function to restrict length of user input
In this guide we will walk through creating a custom react hook to validate passwords. Validating length, uppercase, lowercase, number, special character and matches a second password. This JavaScript tutorial will show you how to validate the strength of password entered by the user for your application. The strength of a password is a function of length, complexity, and unpredictability. It is very important for you to keep your user information safe. Password Validation in React And Javascript. Here, we are going to validate the password on 5 different conditions to make it strong. Lower case letter. Upper case letter. A Number. A special character. Minimum 8 in length. I am going to use minimal code without bothering about its design and all. My aim to share the core working concept.
Using java script How to validate the password in asp c# ... This is what I did some time back. A simple point based approach. JavaScript. ... (password.length < 8) { // this is a very weak password txtPassword.style.color = " Red"; txtPassword.innerHTML = " Too short"; return; } ... The password string will be evaluated after each key press so the user can quickly see the result. Let's create an event listener to capture keyboard events inside the password field. Add the following code to the JavaScript file. password.addEventListener('keyup', function (){ }); If no maxlength is specified, or an invalid value is specified, the password field has no maximum length. This value must also be greater than or equal to the value of minlength. The input will fail constraint validation if the length of the text entered into the field is greater than maxlength UTF-16 code units long.
26/2/2020 · Here we validate various type of password structure through JavaScript codes and regular expression. Check a password between 7 to 16 characters which contain only characters, numeric digit s and underscore and first character must be a letter. Check a password between 6 to 20 characters which contain at least one numeric digit, one uppercase and one lowercase letter. Since I've seen tons of password validation help requests on regexadvice (where I hang out from time to time), I've written up a more general-purpose JavaScript password validation function. It's reasonably straightforward, and covers the validation requirements I've most frequently encountered. Plus, if it doesn't handle your exact needs, its functionality can be augmented by… Basically, the JavaScript will do all the heavy lifting and the AngularJS code will be responsible for binding it all to the screen. To show the password strength to the user, we're going to use a rectangle that contains a color. We'll say that a red rectangle holds a weak password, orange medium, and green a strong password.
On the client side, validation can be done in two ways: Using HTML5 functionality; Using JavaScript; How to set up validation with HTML5 functionality. HTML5 provides a bunch of attributes to help validate data. Here are some common validation cases: Making fields required using required; Constraining the length of data: minlength, maxlength ... Implement Password Strength validation using JavaScript The following HTML Markup consists of an HTML Input TextBox and an HTML SPAN element. The HTML Input TextBox has been assigned a JavaScript OnKeyUp event handler which calls the CheckPasswordStrength JavaScript function. JavaScript password validation. We are going to discuses about JavaScript password special characters validation. In this example we have created one "Text Filed" ," Password Filed" and "Button". We have used JavaScript password validation and check for an alphabet , number special characters. If you will not enter special characters like ...
Example JavaScript solution, with x out of y validation This next example enforces a minimum and maximum password length (8-32 characters), and additionally requires that at least three of the following four character types are present: One or more uppercase letters. One or more lowercase letters.
Magento 2 How To Change Password Length Validation Newbedev
Forums Password Length Minimum Length Js Client Validation
Confirm Password Validation In Javascript Javatpoint
Validate If A Password Meets Certain Requirements
Instant Form Field Validation With React S Controlled Inputs
Master Javascript Form Validation By Building A Form From Scratch
Password Strength Verification With Jquery Webdesigner
Using Array Reduce To Streamline Your Javascript Object
Username And Password Validation In React Example
Data Validation How To Check User Input On Html Forms With
Password Strength Verification With Jquery Webdesigner
Form Validation Using Custom React Hooks Upmostly
Form Validation With Javascript
Bootstrap 4 Form Validation With Validator Js Example
Validate Email And Password Using Jquery May 2020
Set Up Data Validation Studio Pro 9 How To S Mendix
Is It Safe That Everybody Can See My Javascript Validation
Bootstrap 4 Validation With Password Confirmation And Submit
Form Validation Using Javascript Form Validation By
How To Perform Email Validation In Javascript Edureka
0 Response to "23 Password Length Validation Javascript"
Post a Comment