25 Ajax Callback Function Javascript
Using a Callback Function. A callback function is a function passed as a parameter to another function. If you have more than one AJAX task in a website, you should create one function for executing the XMLHttpRequest object, and one callback function for each AJAX task. The function call should contain the URL and what function to call when ... 16. You can't return the result of your ajax request since the request is asynchronous (and synchronous ajax requests are a terrible idea). Your best bet will be to pass your own callback into f1. var f1 = function (arg, callback) { $.ajax ( { success: function (data) { callback (data); } }); } Then you'd call f1 like this:
How To Redirect Ajax Callback To My Callback Function
Apr 12, 2017 - On the other hand, after looking ... the ajax function, and using done() would be a lot simpler, like so -> JSBIN – adeneo Jan 6 '13 at 18:47 · @adeneo So that will only run on success? – Brett Jan 6 '13 at 18:53 ... Not the answer you're looking for? Browse other questions tagged javascript jquery ajax callback or ask your ...
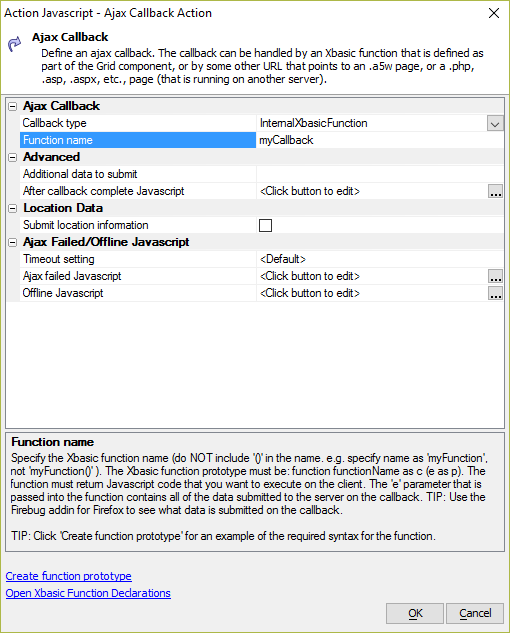
Ajax callback function javascript. By default ajax() method performs http GET request if option parameter does not include method option. The second parameter is options parameter in JSON format where we have specified callback function that will be executed when request succeeds. You can configure other options as mentioned ... jQuery Overview jQuery Selectors jQuery Events jQuery Effects jQuery HTML/CSS jQuery Traversing jQuery AJAX jQuery Misc jQuery Properties ... A callback function is executed after the current effect is 100% finished. ... JavaScript statements are executed line by line. Of course, as I said, it's unpredictable when the callbacks will be executed and depends on multiple factors the JavaScript interpreter used, the function invoking the callbacks and it's input data. An example is an HTTP request with a success callback that won't be executed before the server sends a response, which could be any time interval ...
Aug 16, 2011 - The jsonp and jsonpCallback properties of the settings passed to $.ajax() can be used to specify, respectively, the name of the query string parameter and the name of the JSONP callback function. The server should return valid JavaScript that passes the JSON response into the callback function. Each Javascript callback function is dispatched directly from core Ajax Load More or one of the various add-ons. To utilize the following callback functions simply copy and paste a code example below into your sites JavaScript file. Change. Complete. Destroyed. But it is also good to know how to send AJAX request with plain Javascript. Use the XMLHttpRequest object to communicate with the server. In this tutorial, I show how you can send GET and POST AJAX requests with JavaScript and handle the request with PHP.
Ajax Callback Function. The application process Ajax callback function gets the CLOB data from a table named cmr_clob. It converts the CLOB to a BLOB and invokes the browser to download the BLOB. This download is caught by the fetch in the JavaScript above. Jul 03, 2020 - Understanding how callback functions ... of JavaScript design patterns. I hope this summary of callbacks helps you to understand this concept better. If you have anything technical to add, feel free to post a comment below. ... When I need to wait on multiple animates’ calbacks or ajax requests, inside the function that calls ... There are many JavaScript functions and libraries that can make an Ajax callback; some rely on browser-specific features, and some go to great lengths to insulate the caller from the vagaries of different browsers. In Alpha Anywhere, we have simplified this process by creating a JavaScript function called a5.ajax.callback ().
Dec 02, 2014 - Thanks for writing this, It’s really going to help with all those nested ajax callbacks! I feel like I can actually write a few normal functions now and get some re-usability in my javascript! Callbacks are fundamental to asynchronous coding in JavaScript. In this tutorial we delve into callbacks and look at several examples to help you understand ... The server should return valid JavaScript that passes the JSON response into the callback function. $.ajax() will execute the returned JavaScript, calling the JSONP callback function, before passing the JSON object contained in the response to the $.ajax() success handler. For more information on JSONP, see the original post detailing its use.
Ajax is a very well known method for loading the content of a Web page without manually refreshing it. But the letter "A" in Ajax means asynchronous, meaning that you need to have a callback function that will return the results. You can always use a synchronous call, but that will freeze your page and maybe turn off some of your users. JavaScript Callbacks. A callback is a function passed as an argument to another function. Using a callback, you could call the calculator function ( myCalculator ) with a callback, and let the calculator function run the callback after the calculation is finished: Example. function myDisplayer (some) {. This is an interesting and useful part about callback functions - Depending on which callback function we pass in, we can change how the "main function" processes the results. 4) AJAX CALLS Lastly, some of you sharp code ninjas should have realized.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Ajax Interview Questions 1. XHR. XMLHttpRequest is an object s uch as (a native component in most other browsers, an ActiveX object in Microsoft Internet Explorer) that permits a web page to make ... Jan 08, 2015 - I want to use jQuery ajax to retrieve data from a server. I want to put the success callback function definition outside the .ajax() block like the following. So do I need to declare the variable
Nov 09, 2018 - Ajax is the backbone of Javascript application. It’s used heavily with SPA(Single Page Application). It’s used to communicate with the server. In this blog, I will cover the following topics AJAX requests run asynchronously — that means that the $.ajax method returns before the request is finished, and therefore before the success callback runs. That means that this function's return statement runs before the request is complete. This means the getSomeData function below will ... Example. Request the file cd_catalog.xml and parse the response: const xmlDoc = xhttp.responseXML; const x = xmlDoc.getElementsByTagName("ARTIST"); let txt = ""; for (let i = 0; i < x.length; i++) {. txt += x [i].childNodes[0].nodeValue + "<br>"; } document.getElementById("demo").innerHTML = txt;
Jika function pada umumnya di eksekusi berurutan dari atas ke bawah maka callback di eksekusi pada point tertentu, itu sebabnya di sebut callback. Callback disebut juga dengan high-order function. In the event editor with Action Javascript mode selected, click the Add button to add a new action. Select the Ajax Callback action and click OK. Define the name for the callback function that will be called. In the image below, a new function will be created that performs the callback called myCallback. For future reference: You need to store the callback function (rssToTarget) within the success property of the object literal you are passing to $.ajax(), so jQuery can call that function once the AJAX request is completed.By adding (elem) to the end of the function name, you are mistakenly invoking rssToTarget and storing its return value within success.
Javascript callback functions with ajax. Ask Question Asked 10 years, 6 months ago. Active 9 years, 9 months ago. Viewed 37k times 8 1. I am writing a generic function that will be reused in multiple places in my script. The function uses ajax (using jQuery library) so I want to somehow pass in a function (or lines of code) into this function ... How to Use the Callback Function in Ajax. Webucator provides instructor-led training to students throughout the US and Canada. We have trained over 90,000 students from over 16,000 organizations on technologies such as Microsoft ASP.NET, Microsoft Office, Azure, Windows, Java, Adobe, Python, SQL, JavaScript... I want to use jQuery ajax to retrieve data from a server. I want to put the success callback function definition outside the .ajax() block like the following. So do I need to declare the variable dataFromServer like the following so that I will be able to use the returned data from the success callback?. I've seen most people define the success callback inside the .ajax() block.
The ajax () method is used to perform an AJAX (asynchronous HTTP) request. All jQuery AJAX methods use the ajax () method. This method is mostly used for requests where the other methods cannot be used. What is a callback function? Simply put, a callback function is a function that passed as an argument of another function.Later on, it will be involved inside the outer function to complete some kind of action. A higher-order function is a function that takes a function as its argument, or returns a function as a result.. In JavaScript, functions are first-class objects which means functions ... A callback function is a function passed into another function as an argument, which is then invoked inside the outer function to complete some kind of routine or action. Here is a quick example:
May 05, 2020 - Learn how to use $.ajax(), the most powerful jQuery Ajax function, to perform asynchronous HTTP requests. The event object, XMLHttpRequest, and settings used for that request are passed as arguments to the callback. ... Following is a simple example a simple showing the usage of this method. ... <html> <head> <title>The jQuery Example</title> <script type = "text/javascript" src = "https://ajax.goo... Callback functions are used to handle responses from the server in Ajax. A callback function can be either a named function or an anonymous function. Follow the steps below to create and use the different kinds of callback functions. To write a callback function as an anonymous function:
In Ajax functions many callback functions exist. For example, when the request is raised, one function will raise one event when the request is successfully finished, again another function will be fired. It just calls the A5.ajax.callback () function (which is defined in the Alpha Anywhere Javascript libraries). This function takes two arguments: the name of the.a5w page that will handle the callback, and any data that you want to submit to the callback page. The second argument is in the form of a query string. As of now, the loadScript function doesn't provide a way to track the load completion. The script loads and eventually runs, that's all. But we'd like to know when it happens, to use new functions and variables from that script. Let's add a callback function as a second argument to loadScript that should execute when the script loads:
You can save a reference of a function in a variable when using JavaScript. Then you can use them as arguments of another function to execute later. This is our "callback". One example would be: Ajax callbacks always start in JavaScript. The actual mechanism for making an Ajax callback is not really important. There are many JavaScript functions and libraries that can make an Ajax callback; some rely on browser-specific features, and some go to great lengths to insulate the caller from the vagaries of different browsers.
When To Ajax Callback Vrs When To Dynamic Action
Getting To Know Javascript Callbacks Create Callback
Javascript Wait Until Ajax Done Code Example
Ajax Asynchronous Java Script And Xml By Kranthi
How To Make A Wordpress Ajax Call Step By Step Example
Ajax Success Callback Not Getting Called On 200 Network
Ajax Programming In Application Express Are You Still Using
Drupal Get Form On Ajax Callback Returns This Javascript
Getting Started With Jquery Ajax Back To Basics
Jquery Tutorial 2 Ways Ajax Return Outside Of Ajax Object
Jquery When Executing Callback Function Based On Objects
How To Use Ajax Api In Drupal 8 Opensense Labs
Building Ajax Request And Caching The Response With Url
Ajax Callback Action Javascript
Example Of Vanilla Javascript Fetch Post Api In Laravel 5
Ajax Callback Action Javascript
Jquery Ajax Function Clientserver
Understand Jquery Ajax Function Callback Function Of Jquery
Ajax Load Posts On Wordpress Revamped Made By Denis
How Ajax Works Difference Between Angular Js And Jquery
Asynchronous Javascript Async Await Tutorial Toptal
Javascript From Callbacks To Async Await
0 Response to "25 Ajax Callback Function Javascript"
Post a Comment