31 How To Validate Password Using Javascript
A password is considered valid if all the following constraints are satisfied: It contains at least 8 characters and at most 20 characters. It contains at least one digit. It contains at least one upper case alphabet. Password Validation in React And Javascript. Here, we are going to validate the password on 5 different conditions to make it strong. Lower case letter. Upper case letter. A Number. A special character. Minimum 8 in length. I am going to use minimal code without bothering about its design and all. My aim to share the core working concept.
Password Validation Using Regular Expressions And Html5
Simple Password Validation using Vanilla Javascript and no jquery required....
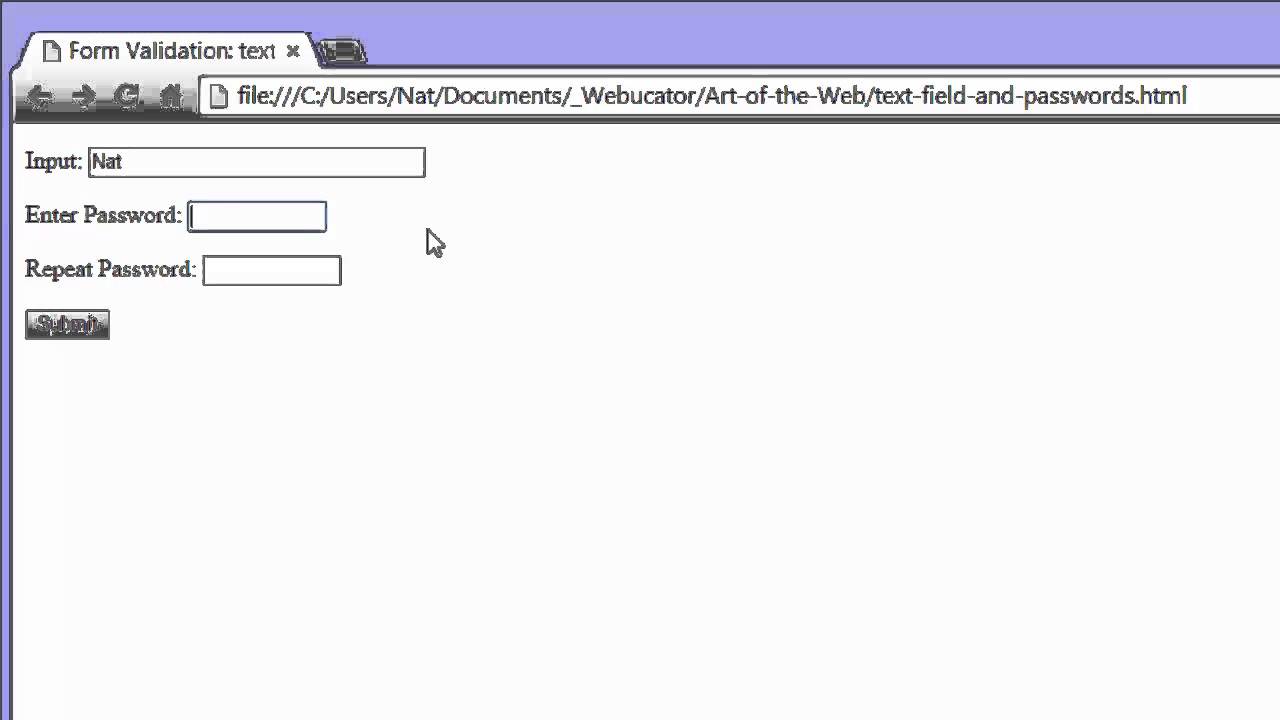
How to validate password using javascript. AboutPressCopyrightContact usCreatorsAdvertiseDevelopersTermsPrivacyPolicy & SafetyHow YouTube worksTest new features · © 2021 Google LLC 12 Oct 2014 — At least one uppercase letter · At least one lowercase letter · At least one digit · At least one special symbol · should be more than 4 character.5 answers · 15 votes: You don't need some big monster single regular expression, which would probably involve arcana ... First, call each individual function to validate username, email, password, and confirm password fields. Second, use the && operator to determine if the form is valid. The form is valid only if all fields are valid. Finally, submit data to the server if the form is valid specified the isFormValid flag.
Now, when you have finished learning how to validate a sample registration form using JavaScript, let us take you to the another way doing the same thing. But this time, the instead of on submitting the form, validations are on field level , i.e. whenever you move from one field to another. Password validation is crucial as it allows the user to create a strong password and avoids any potential password cracks. Using JavaScript, one can add interactivity and hence notify the user until the password has all the required characters in any sequence. Apr 16, 2019 - The task is to validate the password using HTML and JavaScript. ... At least 1 uppercase character. At least 1 lowercase character. At least 1 digit. At least 1 special character. Minimum 8 characters. ... Writing code in comment? Please use ide.geeksforgeeks , generate link and share the ...
Validating email and password - JavaScript. Javascript Web Development Front End Technology Object Oriented Programming. Suppose, we have this dummy array that contains the login info of two of many users of a social networking platform −. const array = [ { email: 'usman@gmail ', password: '123' }, { email: 'ali@gmail ', password: '123 3 weeks ago - The pattern attribute, when specified, is a regular expression that the input's value must match in order for the value to pass constraint validation. It must be a valid JavaScript regular expression, as used by the RegExp type, and as documented in our guide on regular expressions; the 'u' ... Use the password input type to prevent passwords appearing on screen; Decide what constitutes a 'strong' password for your system and enforce it: server-side for spambots and users with JavaScript disabled; using JavaScript for browsers that don't support HTML5 validation; using HTML5 for a more user-friendly experience;
CSS Web Development Front End Technology Javascript. To create a password validation form with CSS and JavaScript, the code is as follows −. Using JavaScript, you can validate name, password, email, date, mobile numbers, and more fields. Keep reading on How to create a modal popup using JavaScript in ASP.Net, Credit Card Authorization Form Template. Html Form Validation using JavaScript Code. The following example will demonstrate how to validate username, email, and password.
Here we will see how to create strong password validation using JavaScript. For strong validation i mentioned here the condition is at least one capital letter and one small letter and one number and one special character then it shows the strong password and also i mentioned here the minimum character length should be 8 character using html5 ... JavaScript provides facility to validate the form on the client-side so data processing will be faster than server-side validation. Most of the web developers prefer JavaScript form validation. Through JavaScript, we can validate name, password, email, date, mobile numbers and more fields. In this tutorial, we will be building a password validator using JavaScript. The validator will check the password as the user types and compare it to the list of requirements. It will also provide a visual representation of which requirements have already been met.
One of the most popular ways of validating email in JavaScript is by using regular expressions. JavaScript uses regular expressions to describe a pattern of characters. email.js. This file contains the JavaScript code that we need for email validation. We are using regular expressions to validate the email at the client-side of a web application. This JavaScript tutorial will show you how to validate the strength of password entered by the user for your application. The strength of a password is a function of length, complexity, and unpredictability. JavaScript Form Validation. HTML form validation can be done by JavaScript. If a form field (fname) is empty, this function alerts a message, and returns false, to prevent the form from being submitted:
This tutorial is about JavaScript form validation with limit login attempts. In which validation function comes into act to authenticate username and password. I am trying to build a simple login form with the predefined username and Password and I want to validate user input matches with username & password that I specified. If the user enters wrong username or password I want to display an alert message. You can achieve each of the individual requirements easily enough (e.g. minimum 8 characters:. {8,} will match 8 or more characters). To combine them you can use "positive lookahead" to apply multiple sub-expressions to the same content. Something like (?=.*\d.*). {8,} to match one (or more) digits with lookahead, and 8 or more characters.
May 22, 2017 - I am trying to validate the password using regular expression. The password is getting updated if we have all the characters as alphabets. Where am i going wrong ? is the regular expression right ? JavaScript to Validate Email and Password Fields on Form Submit Event. The idea behind JavaScript form validation is to provide a method to check the user entered information before they can even submit it. JavaScript also lets you display helpful alerts to inform the user what information they have entered incorrectly and how they can fix it. Sep 21, 2018 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Note: We use the pattern attribute (with a regular expression) inside the password field to set a restriction for submitting the form: it must contain 8 or more characters that are of at least one number, and one uppercase and lowercase letter. The Password strength validation in JavaScript and jQuery will be performed using Regular Expressions (Regex). TAGs: JavaScript, jQuery, Regular Expressions Here Mudassar Ahmed Khan has explained how to implement Password Strength validation for Password TextBox with examples in JavaScript and jQuery. Basically, the JavaScript will do all the heavy lifting and the AngularJS code will be responsible for binding it all to the screen. To show the password strength to the user, we're going to use a rectangle that contains a color. We'll say that a red rectangle holds a weak password, orange medium, and green a strong password.
In this, I have validate form by using Regular Expression in JQuery & used Javascript to show & hide password, where when user type password & want to see that then user can click on eye icon to see their password & to hide it again user will again on same eye icon to hide it. Confirm password with form validation in JavaScript Code examples Form validation is a process to validate that user is submitting the data according to correct format or not. Most of the time it happens that user enter the password during registration and he thinks that he enter the password as he thinks but unfortunately due to the wrong ... JavaScript Password Validation - For any password related form we must need to validate password field like password must be 10 character and use numeric and character combination.
24 Jan 2021 — This tutorial will give readers a detailed guide on how to build a password strength checker using regular expressions in JavaScript. Feb 26, 2020 - You can create a password in different ways, it's structure may be simple, reasonable or strong. Here we validate various type of password structure through JavaScript codes and regular expression. Check a password between 7 to 16 characters which contain only characters, numeric digits and ... To create this program Confirm Password Validation in HTML & JavaScript. First, you need to create two Files one HTML File and another one is CSS File. After creating these files just paste the following codes into your file. First, create an HTML file with the name of index.html and paste the given codes in your HTML file.
May 25, 2017 - Find centralized, trusted content and collaborate around the technologies you use most. ... Connect and share knowledge within a single location that is structured and easy to search. ... I need to add some further validation to this password code. I created a if statement where if any of the ... The Button has been assigned a JavaScript OnClick event handler. When the Button is clicked, the Validate JavaScript function gets executed. Inside the Validate JavaScript function, the values of the Password and the Confirm Password TextBoxes are fetched and are compared. We'll look at validating the password using vanilla JavaScript and also doing it with jQuery. Vanilla Javascript (no framework used) Using the onkeyup event, we can check what the user has entered in both fields and confirm that they have typed the same thing twice and visually confirm to the user that there is no problem with his password.
JS this Keyword JS Debugging JS ... String trim() JavaScript timer Remove elements from array JavaScript localStorage JavaScript offsetHeight Confirm password validation Static vs Const How to Convert Comma Separated String into an Array in JavaScript Calculate age using JavaScript ...
Python Program To Take User Input And Check Validity Of A
How To Create A Password Validation Form
Confirm Password Validation In Javascript Javatpoint
Regular Expression With Jquery Validation Tricks Of It
Form Validation Lt Javascript The Art Of Web
Confirm Password Validation In Javascript Javatpoint
Form Validation Using Jquery Geeksforgeeks
Javascript A Sample Registration Form Validation W3resource
Validate Html Forms With Javascript And Html
How To Validate If Input In Input Field Has Uppercase Letters
How To Create Login Form With Javascript Validation In Html
Bootstrap 4 Validation With Password Confirmation And Submit
Password Strength Verification With Jquery Webdesigner
Confirm Password Validation Example In Html5 Xpertphp
How To Validate Password In Html Form Using Javascript
Password Compare Validation In Html5 Using Javascript In Asp
Password Validation Using Regular Expressions And Html5
Form Validation Using Javascript Form Validation By
Form Field Validation Without Javascript By Fionna Chan
Password And Confirm Password Validation In Html Css Amp Javascript
Javascript Form Validation With Example Codingstatus
Javascript Password Validation Lets Solve
Simple Form Validation In Reactjs Example Skptricks
How To Validate Login Form With React Js By Html Hints Medium
How To Do Simple Form Validation In Reactjs Learnetto
Javascript Client Side Form Validation
Spring Custom Password Validation
Vue Js Email And Password Validation Css Codelab
0 Response to "31 How To Validate Password Using Javascript"
Post a Comment