31 Javascript Push Object Into Array With Key
4/8/2021 · In this article, let’s have a look at how to push object into array with key through example. The example is as follows: <!DOCTYPE html> <html> <body> <h2> JavaScript Array Methods </h2> <h2> push () </h2> <p> The push () method appends a new element to an array. </p> <button onclick="myFunction ()"> Try it </button> <p id="demo"></p> <script> ... Answer 2. You want to split your string, not iterate over it. Then you want to iterate over array of splitted elements and for every one of them you want to create an object and push it into the array. var reportColsShort = 'col1,col2,col3,col4,col5'; var aoCols = []; reportColsShort.split(',').forEach(item => { aoCols.push( { 'mData': item ...
Javascript Pushing An Object In An Array Is What By
1 week ago - If you want to add an object to the array, you must pass an object as an argument and ensure that keys are the same. So, this is How to push both key and value into an Array in Javascript.
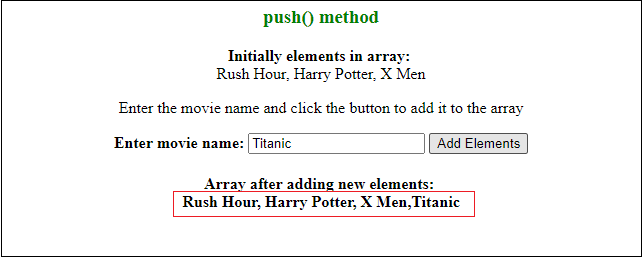
Javascript push object into array with key. ES6: Using Object.keys() to convert Object to array in JavaScript. ECMAScript 2015 or ES6 introduced Object.keys() method to fetch the key data (property)of an object. In the below example, we will fetch the property names of the object "Book". In this way, we can convert an object to an array. In this article, I would like to discuss some common ways of adding an element to a JavaScript array. The Push Method. The first and probably the most common JavaScript array method you will encounter is push(). The push() method is used for adding an element to the end of an array. jquery push array with key, create array with key and value in jquery, javascript array push key value pair dynamically, array push with specific key javascript, javascript array push dynamic key value
In order to push an array into the object in JavaScript, we need to utilize the push () function. With the help of Array push function this task is so much easy to achieve. push () function: The array push () function adds one or more values to the end of the array and returns the new length. This method changes the length of the array. Method 3: unshift () The unshift () function is one more built-in array method of JavaScript. It is used to add the objects or elements in the array. Unlike the push () method, it adds the elements at the beginning of the array. " Note that you can add any number of elements in an array using the unshift () method." How to group an array of objects by key in JavaScript Javascript Web Development Front End Technology Object Oriented Programming Suppose, we have an array of objects containing data about some cars like this −
Oct 27, 2020 - Instead, we store the collection on the object itself and use call on Array.prototype.push to trick the method into thinking we are dealing with an array—and it just works, thanks to the way JavaScript allows us to establish the execution context in any way we want. Feb 10, 2021 - You may have heard conflicting information about associative arrays in JavaScript. This post will clarify that mystery for you, and explain some odd behavior that you have have experienced. JavaScript - Convert an array to key value pair. ... Use the Array.prototype.reduce() method to construct an object from the array. Example. Following is the code − ... How to convert an array into JavaScript string? Print pair with maximum AND value in an array in C Program.
Using Object.fromEntries() In ES2019+, you can achieve that by using the Object.fromEntries() method in the following way: // ES2019+ const obj = Object.fromEntries(pairs); console.log(obj); // {foo: 'bar', baz: 'qux'} Using a for Loop. To create an object from an array of key/value pairs, you can simply use a for loop in the following way: 17/8/2019 · If you want to add the single item into the arryNum array. So you can use the push() method of javaScript like below: var arrNum = [ "one", "two", "three", "four" ]; arrNum.push("five"); console.log( arrNum ); The result of the above example is: ["one", "two", "three", "four", "five"] How to add the multiple items of the array? If you want to add multiple items and elements into a given array. How to transpose a javascript object into a key/value array . Given a JavaScript object, how can I convert it into an array of objects (each with key, value)? ... result. push ({key:k, value:data[k]}); Answered By: Gene R. Answer #7: Or go wild and make the key and value keys customizable:
3 weeks ago - A protip by steveniseki about jquery and javascript. In the above program, the splice () method is used to add an object to an array. The splice () method adds and/or removes an item. The first argument represents the index where you want to insert an item. The second argument represents the number of items to be removed (here, 0). The third argument represents the element that you want to add to ... No need to create an array, do the comparison and push yourself. In the same way when checking for the values, Object.keys can be used with array.every to iterate through your constraints and see if each of the current item's keys match the constraint. Also, I wouldn't call it source. It's not a source of anything.
1 week ago - The Object.values() method returns an array of a given object's own enumerable property values, in the same order as that provided by a for...in loop. (The only difference is that a for...in loop enumerates properties in the prototype chain as well.) Jul 10, 2020 - // original object { key1: "a", key2: "b"} var obj = { key1: "a", key2: "b" }; // adding new filed - you can use 2 ways obj.key3 = "c"; // static // or obj["key3"] = "c"; // dynamic - 'key3' can be a variable console.log(obj) // {key1: "a", key2: "b", key3: "c" } Angular 9/8/7 Push Object in Array Example. There are the Following The simple About AngularJS - Push value to array in scope Full Information With Example and source code. As I will cover this Post with live Working example to develop angularjs push array into array, so the how to push multiple values in array in angularjs is used for this ...
ECMAScript 2015 or ES6 introduced Object.keys() method to fetch the key data (property)of an object. In the below example, we will fetch the property names of the object “Book”. In this way, we can convert an object to an array. How to add an object to an array in JavaScript ?, The push() ... 31/1/2017 · You can use the Object.keys method to get an array of the keys, then use the Array#map method to return a new array containing individual objects for each property. This ES6 one-liner should do it: const splitObject = o => Object.keys(o).map(e => ({ [e]: o[e] })); Or in ES5: In this example, you will learn to write a JavaScript program that will add a key/value pair to an object. Tutorials Examples Course Index Explore Programiz ... Append an Object to An Array. Check if An Object is An Array. Empty an Array. Shuffle Deck of Cards. Add Element to Start of an Array.
Create an Array Iterator object, containing the keys of the array: const fruits = ["Banana", "Orange", "Apple", "Mango"]; const keys = fruits.keys(); for (let of keys) {. text += x + "<br>"; } Jul 10, 2017 - You have to create an object. Assign the values to the object. Then push it into the array: Dec 17, 2020 - //Normal Javascript var key = "happyCount"; var obj = {}; obj[key] = someValueArray; myArray.push(obj); //OR //If you're able to use ES6 and Babel, you can use this new feature: { [yourKeyVariable]: someValueArray, }
//Normal Javascript var key = "happyCount"; var obj = {}; obj[key] = someValueArray; myArray.push(obj); //OR //If you're able to use ES6 and Babel, you can use this ... How to add key/value pair to a JavaScript object?,. or you can set an index to contain the data. Loop through the original object. If the index variable equals the position you want to insert the new key/value pair into, push that to the new object. Push the old key/value pairs into the new object. I've just generated array with unique names and empty series array at first (result), this one is pushing all the series objects data into these empty series arrays in result array. It's just going through original array (arr) to pick series object and push it in result series array - names properties has to match hence y.name===x.name
Add dynamic key, value pairs to JavaScript array or hash table , Define key value pair employee object and push it into employees array. In below example we have employee keys say id , name and age with Define Employee Array. Define key value pair employee object and push it into employees array. To save an object with two fields you have to do something like. arr.push({title:title, link:link}); The closest thing to a python tuple would instead be. arr.push([title, link]); Once you have your objects or arrays in the arr array you can get the values either as value.title and value.link or, in case of the pushed array version, as value[0 ... If your array of object is already empty, make sure it has at least one object, or that object in which you are going to push data to. Let's say, our array is myArray[], so this is now empty array, the JS engine does not know what type of data does it have, not string, not object, not number nothing. So, we are going to push an object (maybe ...
Finally with ES2017, it's official now! We have 3 variations to convert an Object to an Array in JavaScript Javascript/Jquery push json objects into array. 433. November 23, 2016, at 1:35 PM ... So I need to push the full json object into the array. But I cannot alert the item.header within the loop, how can I accomplish this? ... Please note that there is only single key with name "companies" which holds an array of objects. Please correct your ... Definition and Usage The push () method adds new items to the end of an array. push () changes the length of the array and returns the new length. Tip: To add items at the beginning of an array, use unshift ().
Use Object.entries(obj) to get an array of key/value pairs from obj. Use array methods on that array, e.g. map, to transform these key/value pairs. Use Object.fromEntries(array) on the resulting array to turn it back into an object. For example, we have an object with prices, and would like to double them: Object.keys() returns an array whose elements are strings corresponding to the enumerable properties found directly upon object. The ordering of the properties is the same as that given by looping over the properties of the object manually. 19/11/2019 · The task is to convert an Object {} to an Array [] of key-value pairs using JavaScript. Introduction: Objects, in JavaScript, is it’s most important data-type and forms the building blocks for modern JavaScript. These objects are quite different from JavaScript’s primitive data-types (Number, String, Boolean, null, undefined and symbol).
This post will show multiple ways to define key value array. Choose one that suits you the best, based on the use case. To give examples, we will be creating an array of students. We will push some student details in it using javascript array push. We will verify these changes by looping over the array again and printing the result. Jan 19, 2021 - var colors= ["red","blue"]; colors.push("yellow"); Method 1: push () method of Array The push () method is used to add one or multiple elements to the end of an array. It returns the new length of the array formed. An object can be inserted by passing the object as a parameter to this method.
How To Sort Array Of Objects In JavaScript; How To Check If Object Is Array In JavaScript; How To Check If Object Has Key In JavaScript; How To Remove An Attribute From An HTML Element; How To Empty An Array In JavaScript; How To Split Number To Individual Digits Using JavaScript; JavaScript : How To Get Last Character Of A String Mar 31, 2020 - All Languages · javascript push object into array · “javascript push object into array” Code Answer’s · js array add element · javascript by Common Mynah on Sep 23 2019 Comment · array.push(element) · javascript append element to array · javascript by Friendly Hawkes on Oct 29 ... 14/4/2019 · Today, We want to share with you Array push key value pair Dynamically in javascript.In this post we will show you javascript multidimensional associative array push, hear for create array with key and value in jquery we will give you demo and example for implement.In this post, we will learn about How To Push Both Key And Value Into An Array In JQuery with an example.
Javascript Array Push Key Value Code Example
Gtm Guide Datalayer Push With Examples Analytics Mania
Object Push In An Array Code Example
How To Manage React State With Arrays
Javascript Array Push Pop Shift And Unshift Methods With
How To Add An Object To An Array In Javascript Geeksforgeeks
How To Group An Array Of Objects Through A Key Using Array
Data Structures In Javascript Arrays Hashmaps And Lists
Insert A New Key In Array Javascript Code Example
Filtering An Array Of Nested Arrays And Objects Using Angular
How To Add Object In Array Using Javascript Javatpoint
Associative Arrays In Javascript Kevin Chisholm Blog
Push Object Key Into Array Javascript
Object Keys Function In Javascript The Complete Guide
Javascript Push Object Into Array If Not Exists
Javascript Array Push Is 945x Faster Than Array Concat
How To Get Keys From An Object As An Array Spursclick
How To Insert An Item Into Array At Specific Index In
Push Object To A Paired Array Dev Community
Converting Object To An Array Samanthaming Com
Javascript Append To Array A Js Guide To The Push Method
How Do I Add Push Items To An Array One At A Time Looping
How To Group An Array Of Associative Arrays By Key In Php
How To Use The Spread Operator In Javascript
How To Push Object Element To An Array In Vuejs Javascript
Javascript Object Dictionary Examples Dot Net Perls
Javascript Array And Object Destructuring With Es6 Weichie
5 Way To Append Item To Array In Javascript Samanthaming Com
Javascript Array Insert How To Add To An Array With The
Dynamic Array In Javascript Using An Array Literal And
0 Response to "31 Javascript Push Object Into Array With Key"
Post a Comment