26 Document Ready Function Javascript
If script is the last tag of the body, the DOM would be ready before script tag executes When the DOM is ready, "readyState" will change to "complete" Put everything under 'DOMContentLoaded' event listener Nov 17, 2018 - How to wait for the DOM ready event in plain JavaScript · How to run JavaScript as soon as we can, but not sooner ... I usually don’t use arrow functions inside for the event callback, because we cannot access this. In this case we don’t need so, because this is always document.
Javascript In Question Editor Gets Removed Limesurvey Forums
Here is a native Javascript replacement for jQuery's $ (document).ready () function, which works in all modern browsers. There is a standards based replacement, DOMContentLoaded that is supported by over 98% of browsers, though not IE8. document.addEventListener ("DOMContentLoaded", function (event) { //do work });
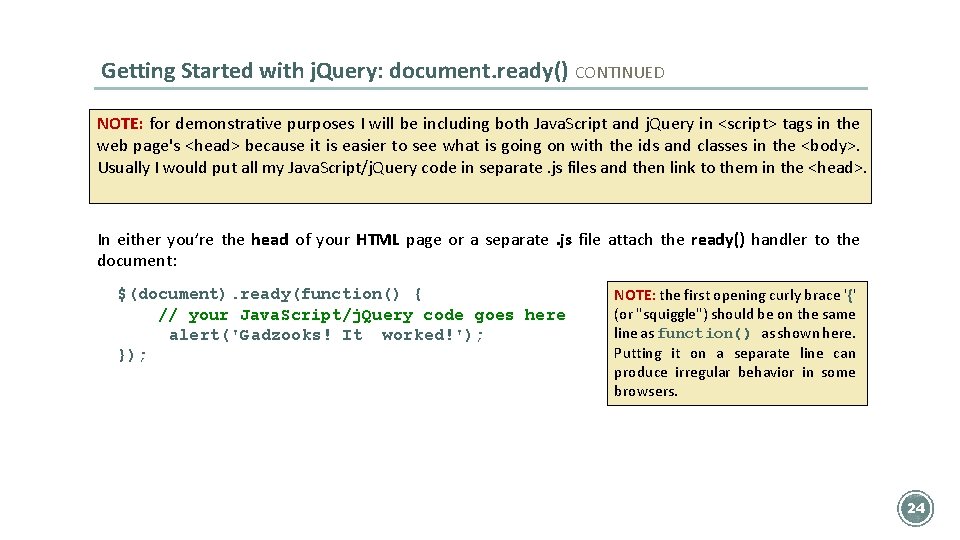
Document ready function javascript. Note: Post jQuery 3.0, this way of calling the ready function is not advisable. Rather you simply call the function as $(function) as the ready method is only called on the current document. $ (document).ready (function () { $ (window).load (function () { // this code will run after all other $ (document).ready () scripts // have completely finished, AND all page elements are fully loaded. feedStaticQuote (); }); the $ () function is the short hand for jquery $ (document).ready () function; so just wrap all the code inside the above function. No need for another document ready function. m4sterbunny May 14, 2018, 2:41pm #3. So you are telling me it works in shorthand- but my longhand version sucks?!
While the document ready is a jQuery event which means that it is only available in the jQuery library, the window.onload is a pure JavaScript event, and therefore, available in most browsers and libraries. The other main difference is apparent from their definitions. The window.onload event waits for the content unlike $ (document).ready (). Calling $ (document).ready () multiple times. The answer is that yes, it can be called multiple times. For example, you might have several Javascript library files and each of them may need to do something after the DOM has loaded. You could then easily put a $ (document).ready () into each of them and they will all be run when the DOM's loaded. 12/3/2016 · The anonymous function passed as parameter in ready() function is executed once as soon as the document is ready. But what if you are not using JQuery in your project? Here is a little JS tip to detect the ready state of document in pure JavaScript. You can detect if document is ready in three ways, sorted by preference. 1. Listening for DOMContentLoaded
16/1/2018 · Javascript Web Development Front End Technology. In jQuery, if you want an event to work on your page, you should call it inside the $ (document).ready () function. Everything inside it will load as soon as the DOM is loaded and before the page contents are loaded. $ (document).ready (function () { alert (“Document loaded successful!"); This function is the equivalent of jQuery's $(document).ready() method: document.addEventListener('DOMContentLoaded', function(){ // do something}); However, in contrast to jQuery, this code will only run properly in modern browsers (IE > 8) and it won't in case the document is already rendered at the time this script gets inserted (e.g. via Ajax). Instead, create a function with a custom name, and push the custom name into the _spBodyOnLoadFunctionNames array. It's wonderful and allows things to load in their proper order. So - with examples, instead of: $ (document).ready (function () { // My custom functionality }); 1.
The ready event occurs when the DOM (document object model) has been loaded. Because this event occurs after the document is ready, it is a good place to have all other jQuery events and functions. Like in the example above. The ready () method specifies what happens when a ready event occurs. Tip: The ready () method should not be used together ... Yes you can have 2 Document Ready Function in your jQuery code but they serve no purpose at all. You just need one document Ready Function and put all you jQuery codes there. The Document Ready Function can have 2 types of syntaxes: 1. First one [... 27/3/2012 · Here is a full substitute for jQuery's .ready() written in plain javascript: (function(funcName, baseObj) { // The public function name defaults to window.docReady // but you can pass in your own object and own function name and those will be used // if you want to put them in a different namespace funcName = funcName || "docReady"; baseObj = baseObj || window; var readyList = []; var readyFired = false; var readyEventHandlersInstalled = false; // call this when the document …
Mar 21, 2021 - The Javascript/jQuery code inside the $(document).ready() function will load after the DOM is loaded, yet before the page contents load. This is important for “events” to work correctly. Using $(document).ready(), your events can happen before the window loads. handler. Type: Function () A function to execute after the DOM is ready. The .ready () method offers a way to run JavaScript code as soon as the page's Document Object Model (DOM) becomes safe to manipulate. This will often be a good time to perform tasks that are needed before the user views or interacts with the page, for example to add event ... Sep 27, 2014 - You call jQuery's $ function, passing to it the document object. The $ function returns an enhanced version of the document object. This enhanced object has a ready() function you can call, to which you pass a JavaScript function. Once the DOM is ready, the JavaScript function is executed.
Jun 20, 2018 - You can present this same function differently, by passing another function instead of the anonymous one. First you have to write the function and then use it by the $(document).ready() method. The code would look like this: $ (document).ready (); is the same as any other function. it fires once the document is ready - ie loaded. the question is about what happens when multiple $ (document).ready ()'s are fired not when you fire the same function within multiple $ (document).ready ()'s The readyState of a document can be one of following: The document is still loading. The document has finished loading and the document has been parsed but sub-resources such as scripts, images, stylesheets and frames are still loading. The document and all sub-resources have finished loading. The state indicates that the load event is about to ...
Saya diberitahu untuk menggunakan document.ready ketika saya pertama kali mulai menggunakan Javascript / jQuery tetapi saya tidak pernah benar-benar tahu mengapa. Mungkinkah seseorang memberikan beberapa pedoman dasar tentang kapan masuk akal untuk membungkus kode javascript / jquery di dalam jQuery document... JavaScript HTML DOM Document Previous Next The HTML DOM document object is the owner of all other objects in your web page. ... document.getElementById(id).onclick = function(){code} Adding event handler code to an onclick event: Finding HTML Objects. Most JavaScript programmers are familiar with jQuery's document ready function. There is no doubt that it is a very helpful way to wrap your JavaScript with a cross-browser compatible function that will not run until the document has achieved a "ready" state. While the syntax is fairly simple, there are two slightly less verbose ...
So, to replicate the $(document).ready() function in plain Javascript (i.e. not using jQuery), you can use one of the following. DOMContentLoaded. DOMContentLoaded is an event fired when the DOM has finished loading, but doesn't wait for stylesheets or images to load. The ready () function in jQuery executes the code only when the DOM (Document object model) is fully loaded. It is an inbuilt function in jQuery. It can fire before loading of all images, etc. but only when the DOM is ready. The code inserted between $ (document).ready () is executed only when the page is ready for JavaScript code to execute. $(document).ready() The document ready event fired when the HTML document is loaded and the DOM is ready, even if all the graphics haven't loaded yet. If you want to hook up your events for certain elements before the window loads, then $(document).ready is the right place. Code: $(document).ready(function() { // document is loaded and DOM is ...
Using vanilla JavaScript with no jQuery, the simplest way to check if the document is 'ready' to be manipulated is using the DOMContentLoaded event: document .addEventListener ( 'DOMContentLoaded', function () { // do something here ... }, false ); You can also use a named function instead of an anonymous function: Dec 14, 2017 - The ready() method is used to make a function available after the document is loaded. Whatever code you write inside the $(document ).ready() method will run once the page DOM is ready to execute JavaScript code. You can utilize the JavaScript Window's DOMContentLoaded event to construct $ (document).ready () equivalent without jQuery. This event fires when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading.
Yes! Very simply, jQuery calls your $ (document).ready () function once the DOM has fully loaded. But it's nothing fancy: it's approximately equal to putting your JavaScript code at the absolute bottom of the page. It's nice because it makes our code portable: it will work no matter where it lives. 16/1/2017 · jQuery detects this state of readiness for you. Code included inside $ (document).ready () will only run once the page Document Object Model (DOM) is ready for JavaScript code to execute. Code included inside $ (window).on ("load", function () {... }) will run once the entire page (images or iframes), not just the DOM, is ready. 1 20/10/2016 · Before the release of version 3, there were several ways you could call the ready method: on the document element: $(document).ready(handler); on an empty element: $().ready(handler);
Jul 23, 2019 - In jQuery, the code: $(function() { ... }) is a valid and recommended shortcut for the code: $(document).ready(function() { ... }) ... Which jQuery function is used to prevent code from running, before the document is finished loading? * 1 point $(document).load() $(document).ready() ... Why document.ready() is used in jQuery $( document ).ready() waits for the HTML Document content to be loaded fully and become ready, after rendering all the elements into the window object or in a nutshell, completes the loading of the body. Our addTwoNumbers() function above, for example, is fully described by the JSDoc heading. But for slightly bigger functions, it's useful to add one-line (or sometimes multi-line) comments within to describe what's happening. This may seem overdone. I've had some more pretentious engineers tell me I over-document.
Mar 24, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Solved Using A User Macro Multiple Times On A Page
Undefined Issue In Jquery Ajax Javascript Sitepoint
Conditional Statements Quiz Finishing Last Lesson Find Id
Document Ready Doesn T Seem To Work Properly With User
Jquery Document Ready Alternatives Ppt Download
Unclear Where Document Ready Goes Jquery Forum
Window Onload Vs Document Ready Stack Overflow
Jquery Document Ready Function Skillsugar
Call Jquery Function From Another Js File Es6 Code Example
Sql Server Net And C Video Tutorial What Is Document
I Need Js Code Document Ready Function Chegg Com
Google Pie Chart In Sharepoint Online
What S Wrong With Jquery Document Ready Function Working With
Jquery Exception With Document Ready Object Doesn T
Conditional Statements Quiz Finishing Last Lesson Find Id
Document Ready Function Executed Every Time On Onclick
Jquery Tutorial In Urdu Hindi 4 Jquery Document Ready Function
Solved Portals Javascript Changes You Made May Not Be Sav
Cannot Load Method Unresolved Function Or Method Datatable
0 Response to "26 Document Ready Function Javascript"
Post a Comment