30 Javascript Parameter By Reference
24/6/2019 · When a variable’s reference (address) and not its value is passed to a function’s parameter, any changes made to the parameter will update the original variable reference. This is known as call by... The arguments object is a local variable available within all non-arrow functions. You can refer to a function's arguments inside that function by using its arguments object. It has entries for each argument the function was called with, with the first entry's index at 0.. For example, if a function is passed 3 arguments, you can access them as follows:
Mastering This In Javascript Callbacks And Bind Apply
JavaScript arguments are passed by value: The function only gets to know the values, not the argument's locations. If a function changes an argument's value, it does not change the parameter's original value. Changes to arguments are not visible (reflected) outside the function. ... In JavaScript, object references ...
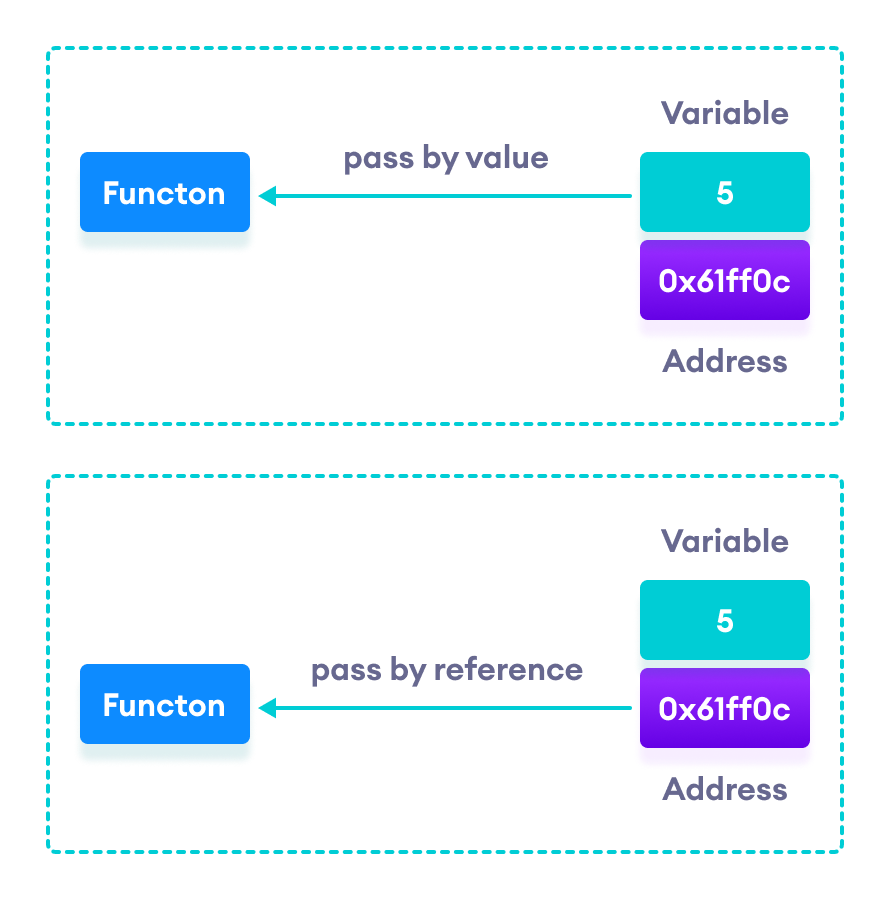
Javascript parameter by reference. Summary. Objects are assigned and copied by reference. In other words, a variable stores not the "object value", but a "reference" (address in memory) for the value. So copying such a variable or passing it as a function argument copies that reference, not the object itself. All operations via copied references (like adding/removing ... Call by Value in JavaScript. The concept of Call by Value is, when we want to pass any parameter as a function argument then there are two types of arguments. An argument that is passed when the function is called an Actual Argument and the argument that takes the value in the caller's function is called a Formal Argument . Another possibility is to hand in optional parameters as named parameters, as properties of an object literal (see Named Parameters). Simulating Pass-by-Reference Parameters In JavaScript, you cannot pass parameters by reference; that is, if you pass a variable to a function, its value is copied and handed to the function (pass by value).
Dec 17, 2020 - An arrow function expression is a compact alternative to a traditional function expression, but is limited and can't be used in all situations. You can specify that you want to pass-by-reference however by putting an ampersand character between the data type and the parameter name in the parameter list in the function definition. Conclusion: Function parameters and arguments in JavaScript. Function parameters and arguments can be confusing. I hope that this post made it easier for you to understand what parameters and arguments are and how they differ. I also hope that this post helped you learn how to use arguments object and rest and default parameters.
7/8/2021 · a copy reference to obj1 and obj2 is passed to the function, pointing to the same address of the “original” objects in memory (call by sharing) 3. With the obj1Param = obj2Param assignment in the function, both obj1Param and obj2Param to the original obj2 object in memory, so when changing its property obj2Param.value = 'changed' it will also be visible outside of the function scope, after it is … In JavaScript all arguments are passed by value. When a function assigns a new value to an argument variable, that change will not be visible to the caller: var obj = {a: 2}; function myfunc(arg){ arg = {a: 5}; // Note the assignment is to the parameter variable itself } myfunc(obj); console.log(obj.a); // 2 Function arguments are passed either by-value or by-sharing, but never ever by reference in JavaScript! Call-by-Value. Primitive types are passed by-value: var ...13 answers · Top answer: Primitives are passed by value, and Objects are passed by "copy of a reference". Specifically, ...
Jul 20, 2021 - See also the exhaustive reference chapter about JavaScript functions to get to know the details. ... A function definition (also called a function declaration, or function statement) consists of the function keyword, followed by: The name of the function. A list of parameters to the function, ... The words "value" and "reference" refer specifically to the parameter as it appears in the function call expression. JavaScript always evaluates each expression ...32 answers · 1,754 votes: It's interesting in JavaScript. Consider this example: function changeStuff(a, b, c) { ... When you are passing something by reference, you are passing something that points to something else, not a copy of the object. So since JavaScript passes objects by reference, when you change a property of that object within the function, the change will be reflected in the outer scope: var myName = {}; function myNameIs (aName) {
Now, on to functions and passing parameters.... When you call a function, and pass a parameter, what you are essentially doing is an "assignment" to a new ...32 answers · 1,752 votes: It's interesting in JavaScript. Consider this example: function changeStuff(a, b, c) { ... JavaScript pass by value or pass by reference In JavaScript, all function arguments are always passed by value. It means that JavaScript copies the values of the passing variables into arguments inside of the function. Any changes that you make to the arguments inside the function does not affect the passing variables outside of the function. 1 week ago - The rest parameter syntax allows a function to accept an indefinite number of arguments as an array, providing a way to represent variadic functions in JavaScript.
Using URL parameters is probably the easiest way of passing variables from one webpage to the other. In this article I'm going to present how to get a URL parameter with JavaScript. The image above presents how will the variables passed in the link. Passing Arguments By Reference. In JavaScript, everything is passed by value, but when we pass a variable that refers to an object (including arrays), the "value" is a reference to the object, and changing a property of an object referenced by a variable does change the underlying object. Consider this function: There are some instances that address is passed instead of arguments to call a function. At that time, changing the value inside the function affect the variable passed from outside the function. This is called a pass by reference. In javascript mostly arrays and objects follow pass by reference.
There are two ways that a function argument, or parameter, can be passed to it: by value or by reference. In the first instance, a copy of the variable is made when the function receives it. Hence, any changes made to the passed variable within the function end when the function returns or exits for whatever reason. There is no "pass by reference" available in JavaScript. You can pass an object (which is to say, you can pass-by-value a reference to an object) and then have a function modify the object contents: function alterObject(obj) { obj.foo = "goodbye"; } var myObj = { foo: "hello world" }; alterObject(myObj); alert(myObj.foo); // "goodbye" instead of "hello world" Passing by value and passing by reference in javascript. Till now in this article we learnt about how placeholders hold static data types by value and non-static data types by reference and also how mutation works for these two. We also saw how non-static data types get mutated even inside other functions.
18/1/2021 · In JavaScript array and Object follows pass by reference property. In Pass by reference, parameters passed as an arguments does not create its own copy, it refers to the original value so changes made inside function affect the original value. let us take an example to understand better. 17/9/2019 · Javascript Front End Technology Object Oriented Programming. Javascript is a pass by value language. But for objects, the value is their reference. So for example, if you pass an int to a function as parameter and increment its value in the function, … Aug 07, 2017 - Coming from Ruby when I first dove into Javascript the idea of passing by reference versus value threw me for a loop.
In JavaScript, all objects interact by reference. If an object is stored in a variable and that variable is made equal to another variable then both of them occupy the same location in memory. Changes in one object variable affect the other object variable. Quick Tip: Get URL Parameters with JavaScript. By ... If you need a refresher, check out the MDN JavaScript reference. How the Function Works. Overall, the function takes a URL's query string ... Javascript has 3 data types that are passed by reference: Array, Function, and Object. These are all technically Objects, so we'll refer to them collectively as Objects.
Pass By Reference¶ In pass by reference, a function may be called by directly passing the reference/address of the variable as an argument. If you change the argument inside the function, it will affect the variable passed from outside the function. In JavaScript, objects and arrays are passed by reference. Here is an example: In Pass by Reference, Function is called by directly passing the reference/address of the variable as the argument. Changing the argument inside the function affect the variable passed from outside... Nov 28, 2012 - There are two ways that a function argument, or parameter, can be passed to it: by value or by reference. In the first instance, a copy of the variable is made when the function receives it. Hence, any changes made to the passed variable within the function end when the function returns or ...
Here, lion and tiger are reference types, their values are stored in the Heap and they are pushed to the stack. Their values in stack hold the memory address of the location in Heap. Activation Record, Parameter Passing. We have seen the memory model of a JS program. Now, let's see what happens when a function is called in JavaScript. Aug 01, 2020 - Passing parameters by value and by reference to the function in the JavaScript | standard ES5 ... Strings, numbers, flags are passed to the function by value. That is, when passing the parameter to the function if the parameter (string, number, flag) this option creates a copy inside the function ... If you don't know the method of the object being passed in then you need to pass both the method and the object as parameters and use the call method. call is part of the JavaScript specification and allows a function to run in the context of another object. As a result, the this keyword will reference the right object: the object we passed in.
The join () function converts the array to a string after the function call. This increased the code but due to lack of dereferencing operator in JavaScript, it remains the easiest way to pass a string by reference. Complete example to pass a string by reference In JavaScript, function parameters default to undefined. However, it's often useful to set a different default value. This is where default parameters can help. In the past, the general strategy for setting defaults was to test parameter values in the function body and assign a value if they are undefined.
Calling A Dynamics 365 Action From Javascript Using Xrm
Javascript Actions Studio Pro 9 Guide Mendix Documentation
Does Java Pass By Reference Or Pass By Value Infoworld
Pass By Value Vs Pass By Reference In Javascript By
An Illustrated Guide To Parameter Passing In Javascript
Calling A Dynamics 365 Action From Javascript Using Xrm
How To Pass An Array Of Characters As A Parameter To A
Pass By Value Vs Pass By Reference
Call Action In Crm 2015 Easily From Javascript Library
Add A Parameter To Reference Popup Url Now Platform
Calling A Dynamics 365 Action From Javascript Using Xrm
Javascript Interview Questions And Answers 2021 Interviewbit
Objects Javascript Codecademy Forums
Passing By Reference Vs By Value Javascript Tutorial
How To Call A Global Action In Javascript With One Entity
A Visual Guide To References In Javascript
Passed By Reference Vs Value In Javascript Dev Community
Javascript Pass By Value And Pass By Reference In Javascript
How Do I Pass A Variable By Reference Stack Overflow
Javascript Passing By Value Vs Reference Explained In Plain
Modifying Variables That Are Passed By Reference In
Support Rest Parameter Syntax Like Javascript Issue 893
Pass By Value Vs By Reference In Javascript Danielle Developer
Working With Javascript In Visual Studio Code
How Do I Pass A Variable By Reference Stack Overflow
The Difference Between Values And References In Javascript
C Call By Reference Using Pointers With Examples
Is Javascript Pass By Reference Aleksandr Hovhannisyan
0 Response to "30 Javascript Parameter By Reference"
Post a Comment