29 Await Function In Javascript
Async/Await Basics in JavaScript There are two parts to using async/await in your code. First of all, we have the async keyword, which you put in front of a function declaration to turn it into an async function. An async function is a function that knows to expect the possibility that you'll use the await keyword to invoke asynchronous code. JavaScript Await function is used to wait for the promise. It could only be used inside the async block. It instructs the code to wait until the promise returns a response. It only delays the async block.
How To Run Async Javascript Functions In Sequence Or Parallel
Everything is synchronous until a Javascript asynchronous function is executed. In using async-await await is asynchronous and everything after await is placed in event queue. Similar to.then (). To better explain take this example:
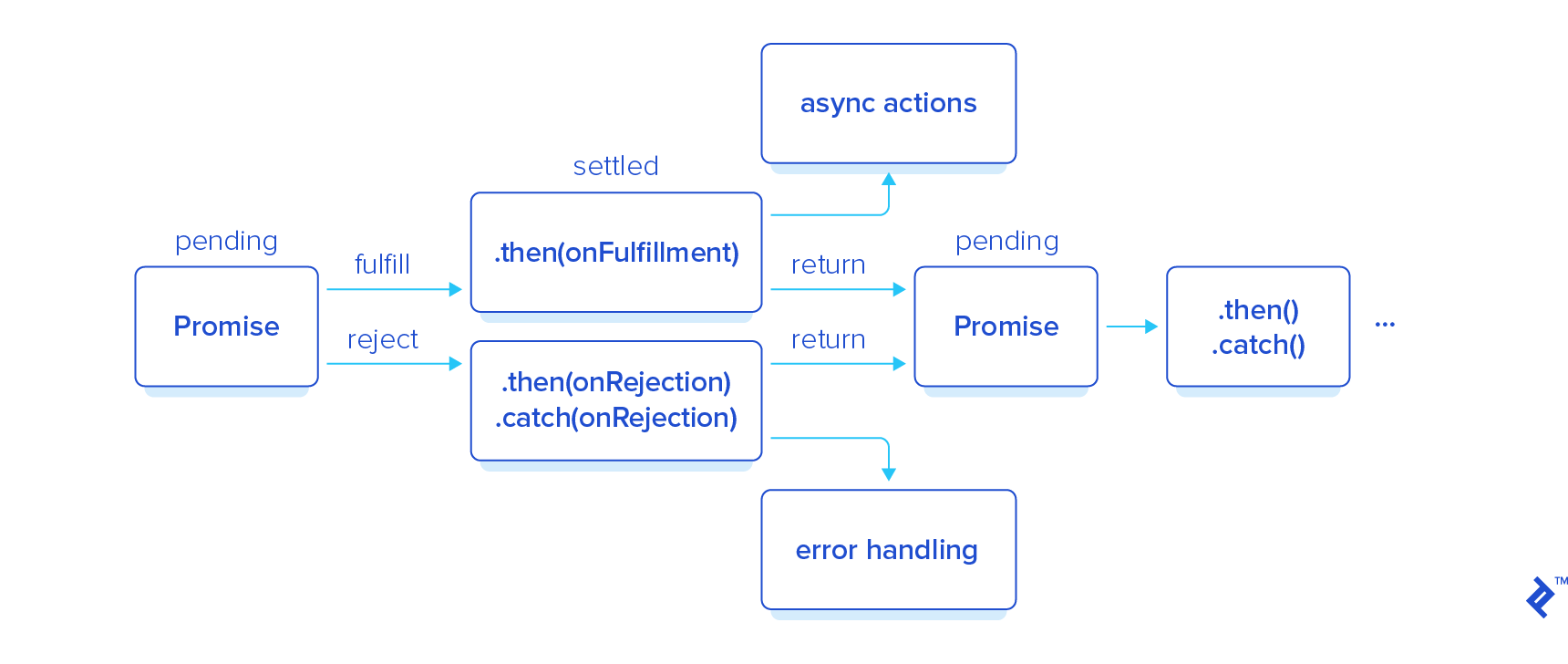
Await function in javascript. Nice post! Thanks for sharing. Just my 2 cents, in the function ParallelMapFlow the async await code in the map is not necessary: should be this let results = jobs.map(job => doJob(job,job)); instead of let results = jobs.map(async (job) => await doJob(job,job)); And, in the function ParallelPromiseAllFlow, the await in line number 7 the same ... The await keyword waits for the script until the function settles its promise and returns the result. We can use the await keyword only inside the async functions. It will pause the " async " function and wait for the Promise to resolve before moving on. Its syntax looks like below: Async/Await is the extension of promises which we get as a support in the language. You can refer Promises in Javascript to know more about it.
The await keyword tells JavaScript to pause the execution of the async function in which it is. This function is then paused until a promise, that follows this keyword, settles and returns some result. So, it is this await keyword what moves the executed code the siding until it is finished. list.map () returns a list of promises, so in result we'll get the value when everything we ran is resolved. Remember, we must wrap any code that calls await in an async function. See the promises article for more on promises, and the async/await guide. The keyword 'async' before a function makes the function return a promise, always. And the keyword await is used inside async functions, which makes the program wait until the Promise resolves.
Async await function and operators work on promises. Async/await functions help us to write completely synchronous-looking code while performing async tasks behind the scenes. For example, let us say we have a asynchronous function that returns a promise −. We want to use this in a function, but we need to wait for the return value. The await keyword is for making the JavaScript function execution wait until a promise is settled (either resolved or rejected) and value/error is returned/thrown. As the fetchUserDetails async function returns a promise, let us handle it using the await keyword. const user = await fetchUserDetails (); console.log (user) Every async function myFunction(){ <your code here> } declaration automatically wraps the whole async function's code (ie. <your code here>) in a new Promise, and turns any return x within the code into resolve(x). But you still need to await outside of it (ie. let y = await myFunction()) or it won't actually wait. The debugging around this ...
javascript - Call async/await functions in parallel - Stack Overflow As far as I understand, in ES7/ES2016 putting multiple await's in code will work similar to chaining.then() with promises, meaning that they will execute one after the other rather than in paralle... If await gets a non-promise object with .then, it calls that method providing the built-in functions resolve and reject as arguments (just as it does for a regular Promise executor). Then await waits until one of them is called (in the example above it happens in the line (*)) and then proceeds with the result. The run() function shouldn't be responsible for handling every possible error, you should instead do run().catch(handleError). Looking to become fluent in async/await? My new ebook, Mastering Async/Await, is designed to give you an integrated understanding of async/await fundamentals and how async/await fits in the JavaScript ecosystem in a few ...
Our function has an async keyword on its definition (which says that this function will be an Async function, of course). It also has an await keyword, which we use to "wait for" a Promise. The await operator is used to wait for a Promise. It can only be used inside an async function within regular JavaScript code; however it can be used on its own with JavaScript modules. As you can see, the callback is called but we are not waiting for it to be done before going to the next entry of the array. We can solve this by creating our own asyncForEach () method: async function asyncForEach (array, callback) {. for (let index = 0; index < array.length; index++) {. await callback (array [index], index, array);
First, let's go through what the Aysnc/Await keywords actually mean as it relates to JavaScript. The Async keyword is short for 'Asynchronous' and in always positioned before a function. This is done to indicate an Aync function and more importantly, that the function being specified as Async will always return a promise. Since JavaScript is based on asynchronous programming techniques, there are multiple approaches such as callbacks, promises and async/await enabling you to put your functions executions in sequence. So that a code-block or a function won't be executed before another specific function finishes. async function foo() {const result1 = await new Promise((resolve) => setTimeout(() => resolve('1'))) console.log(result1); //Output is 1} Output of above functions is 1, pretty straight forward and self explanatory, Where await will block the main thread until the promise is resolved. Now let's check an interesting version of above code snippet.
await is a new operator used to wait for a promise to resolve or reject. It can only be used inside an async function. The power of async functions becomes more evident when there are multiple steps involved: Await Syntax The keyword await before a function makes the function wait for a promise: let value = await promise; The await keyword can only be used inside an async function. JavaScript await Keyword. The await keyword is used inside the async function to wait for the asynchronous operation. The syntax to use await is: let result = await promise; The use of await pauses the async function until the promise returns a result (resolve or reject) value. For example,
The await keyword causes the JavaScript runtime to pause your code on this line, not allowing further code to execute in the meantime until the async function call has returned its result — very useful if subsequent code relies on that result! Once that's complete, your code continues to execute starting on the next line. JavaScript is a synchronous language, yet sometimes asynchronous behavior is required. For example, you may need to use an asynchronous function while waiting for data to be fetched from an API, or while waiting for a site's images to load. JavaScript - Async and Await Function A async keyword before a function makes the function async function. It is a special syntax to work with promises in a more comfortable way. It is very easy to understand and use.
The keyword Await makes JavaScript wait until the promise returns a result. It has to be noted that it only makes the async function block wait and not the whole program execution. The code block below shows the use of Async Await together. async function firstAsync () {. let promise = new Promise ( (res, rej) => {.
Async Constructor Functions In Typescript Stack Overflow
5 Ways To Make Http Requests In Node Js Using Async Await
Asynchronous Javascript With Promises Amp Async Await In
Async Await The Hero Javascript Deserved
Async Await Vs Promises A Guide And Cheat Sheet By Kait
Asynchronous Programming Eloquent Javascript
Comparing Callbacks Promises And Async Await In Typescript
Async Await What You Should Know Updated Codeproject
Asynchronous Javascript Async Await Tutorial Toptal
Don T Make That Function Async Dev Community
Microsoft Azure S Durable Functions For Serverless Javascript
Async Await In Node Js How To Master It Risingstack
Asynchronous Javascript With Promises Amp Async Await In
Faster Async Functions And Promises V8
Async Await Javascript Stack Overflow Code Example
Calling An Async Function From Another Async Function Js Code
Es7 Async Await In Node Js With Babel Js
Javascript Async Await 101 Hakaselogs
How To Throw Errors From Async Functions In Javascript
Asynchronous Adventures In Javascript Async Await By
Deeply Understanding Javascript Async And Await With Examples
Callback Hell Promises And Async Await
Javascript Asynchronous Method Comparison Callbacks
Faster Async Functions And Promises V8
Understanding Async Await In Javascript By Gemma Stiles
How To Use Async Await To Write Better Javascript Code
How To Rewrite A Callback Function In Promise Form And Async
Be Careful Of Passing An Async Function As A Parameter In
0 Response to "29 Await Function In Javascript"
Post a Comment