26 Javascript Function To Variable
JavaScript libraries such as YUI have already learned that the variable order is a common nuisance and an opportunity to introduce errors, so they've come up with a solution: pass a single object to the function. It ends up looking something like this: A function is just a declaration until it is explicitly evaluated. The declaration can be assigned to a variable, which can then be passed as a parameter to another function. Thus, a JavaScript...
How To Check If A Variable In Javascript Is Undefined Or Null
In JavaScript, you can declare variables by using the keywords var, const, or let. In this article, you'll learn why we use variables, how to use them, and the differences between const, let and var. What are variables used for in JavaScript? In the context of coding, data is information that we use in our computer programs.
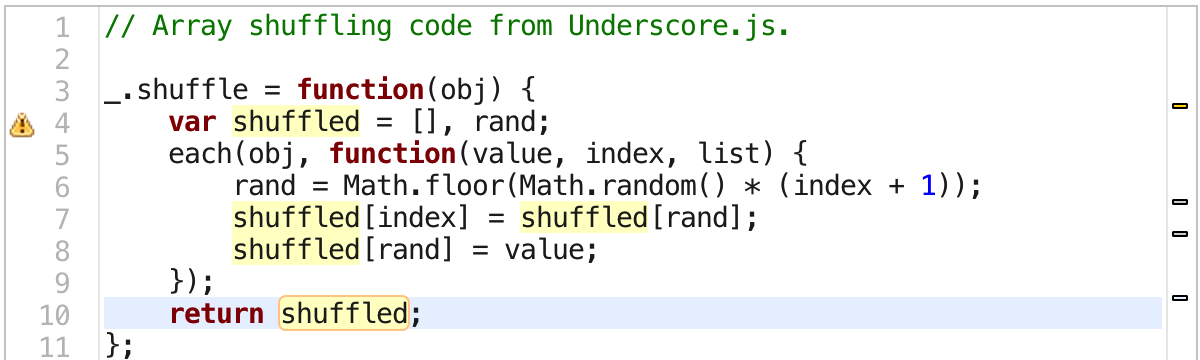
Javascript function to variable. Variables in JavaScript are containers that hold reusable data. It is the basic unit of storage in a program. The value stored in a variable can be changed during program execution. A variable is only a name given to a memory location, all the operations done on the variable effects that memory location. Variable Scope in Javascript. Scope of a variable is the part of the program from where the variable may directly be accessible. In JavaScript, there are two types of scopes: Global Scope - Scope outside the outermost function attached to Window. Local Scope - Inside the function being executed. Let's look at the code below. JavaScript allows for the nesting of functions and grants the inner function full access to all the variables and functions defined inside the outer function (and all other variables and functions that the outer function has access to).
In JavaScript, you can do the same thing with functions: // Example 1 var hashAlgorithm = 'sha1'; var hash; if ( hashAlgorithm === 'sha1' ) hash = function (value) { /*...*/ }; else if ( hashAlgorithm === 'md5' ) hash = function (value) { /*...*/ }; hash ('Fred'); In the example above, hash is a normal variable. Variables created outside of functions are global variables, and the code in all functions have access to all global variables. If you forget to code the var keyword in a variable declaration, the JavaScript engine assumes that the variable is global. This can cause debugging problems. In JavaScript, it's possible to declare variables in a single statement. let x = 5, y = 6, z = 7; If you use a variable without initializing it, it will have an undefined value. let x; // x is the name of the variable console.log (x); // undefined. Here x is the variable name and since it does not contain any value, it will be undefined.
The parameters, in a function call, are the function's arguments. JavaScript arguments are passed by value: The function only gets to know the values, not the argument's locations. If a function changes an argument's value, it does not change the parameter's original value. Changes to arguments are not visible (reflected) outside the function. Output: //no output is produced by this code// Here we made a new variable function printFunc2 which refers to the function printFunc() of object geeks.Here the binding of this is lost, so no output is produced. To make sure that any binding of this is not to be lost, we are using Bind() method. By using bind() method we can set the context of this to a particular object. When the function is called in lines (*) and (**), the given values are copied to local variables from and text.Then the function uses them. Here's one more example: we have a variable from and pass it to the function. Please note: the function changes from, but the change is not seen outside, because a function always gets a copy of the value:
When the function completes (finishes running), it returns a value, which is a new string with the replacement made. In the code above, the result of this return value is saved in the variable newString. If you look at the replace () function MDN reference page, you'll see a section called return value. Hoisting is JavaScript's default behavior of moving declarations to the top of the current scope. Hoisting applies to variable declarations and to function declarations. Because of this, JavaScript functions can be called before they are declared: myFunction (5); The declaration begins with the function keyword, followed by the name of the function. Function names follow the same rules as variables — they can contain letters, numbers, underscores and dollar signs, and are frequently written in camel case. The name is followed by a set of parentheses, which can be used for optional parameters.
A function is a parametric block of code defined once and called multiple times later. In JavaScript a function is composed and influenced by many components: JavaScript code that forms the function body The list of parameters Each keyword holds some specific reason or feature in JavaScript. Basically we can declare variables in three different ways by using var, let and const keyword. Each keyword is used in some specific conditions. var: This keyword is used to declare variable globally. If you used this keyword to declare variable then the variable can accessible ... A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2,...)
In programming, just like in algebra, we use variables (like price1) to hold values. In programming, just like in algebra, we use variables in expressions (total = price1 + price2). From the example above, you can calculate the total to be 11. JavaScript variables are containers for storing data values. How to use static variables in a Javascript function. There may be times when a static variable is needed in a Javascript function; static variables maintain their value between function calls and are tidier than using a global variable because they cannot be modified outside of the function. The arguments object is a local variable available within all non-arrow functions. You can refer to a function's arguments inside that function by using its arguments object. It has entries for each argument the function was called with, with the first entry's index at 0.. For example, if a function is passed 3 arguments, you can access them as follows:
The return statement stops the execution of a function and returns a value from that function. Read our JavaScript Tutorial to learn all you need to know about functions. Start with the introduction chapter about JavaScript Functions and JavaScript Scope. For more detailed information, see our Function Section on Function Definitions ... When you assign a function object to another variable JavaScript does not create a new copy of the function. Instead it makes the new variable reference the same function object as original. It is just that two variables having different names are accessing the same underlying function object. Then the variable a is accessed inside a function and the value changes to 3. Hence, the value of a changes after changing it inside the function. Note: It is a good practice to avoid using global variables because the value of a global variable can change in different areas in the program. It can introduce unknown results in the program.
JavaScript variables have only two scopes. Global Variables − A global variable has global scope which means it can be defined anywhere in your JavaScript code. Local Variables − A local variable will be visible only within a function where it is defined. Function parameters are always local to that function. Enter the first variable: 4 Enter the second variable: 2 The value of a after swapping: 2 The value of b after swapping: 4. Here, We created a temp variable to store the value of a temporarily. We assigned the value of b to a. The value of temp is assigned to b; As a result, the value of the variables are swapped. 15/4/2016 · To assign a function to a variable you have to use just the name, such as: var x = a; or pass the name to a function f: f(a) As a counter-example you invoke it in this next line of code and pass to g not the function be the result of its execution: g(a())
In JavaScript, a variable can be declared either in the global scope or the local scope. Global Variables. Variables declared out of any function are called global variables. They can be accessed anywhere in the JavaScript code, even inside any function. Local Variables. Variables declared inside the function are called local variables to that ... One last point: you also need to avoid using JavaScript reserved words as your variable names — by this, we mean the words that make up the actual syntax of JavaScript! So, you can't use words like var, function, let, and for as variable names. Browsers recognize them as different code items, and so you'll get errors.
Javascript Variable Scope And Highlight Ariya Io
We Need To Minimize The Use Of Global Variables In Javascript
A Simple Explanation Of Javascript Closures
How To Define Global Variable In A Javascript Function
Variable And Function Hoisting In Javascript By Erin Walker
Javascript Basics Let Variable And Arrow Function Definition
Execution Context And Activation Object In Details
Anonymous Functions Assigned To References Show Up Well
Javascript Variables Lifecycle Why Let Is Not Hoisted
How Javascript Variable Scoping Is Just Like Multiple Levels
Let Your Javascript Variables Be Constant By Jakub Janczyk
Declaring Variables In Es6 Javascript By Yash Agrawal
This Keyword In Javascript As Of Most Used Keyword Of
Binding A Name For Javascript Function Using Variable Name
Javascript Global Variable Code Bridge Plus
How To Declare A Variable Function From Libraries In
Javascript Function And Function Expressions With Examples
What Are Closures In Javascript Dzone Web Dev
Basic Javascript Local Scope And Functions Global Variable
Gtmtips Create Utility Variables By Returning Functions
How To Add Insert Javascript Variable To Button Html Tag In
0 Response to "26 Javascript Function To Variable"
Post a Comment