32 How To Find Index Of Element In Array Javascript
If you need the index of the found element in the array, use findIndex (). If you need to find the index of a value, use Array.prototype.indexOf (). (It's similar to findIndex (), but checks each element for equality with the value instead of using a testing function.) The Array findIndex () method returns the index of first matched element in array that satisfies a condition. This method also takes a function argument which returns true or false.
Java Exercises Find The Index Of An Array Element W3resource
The callback is a function to execute on each element of the array. It accepts 3 parameters: element is the current element of an array. index the index of the current element of an array. array the array that the findIndex() was called upon. 2) thisArg. The thisArg parameter is an optional object to be used this when executing the callback.
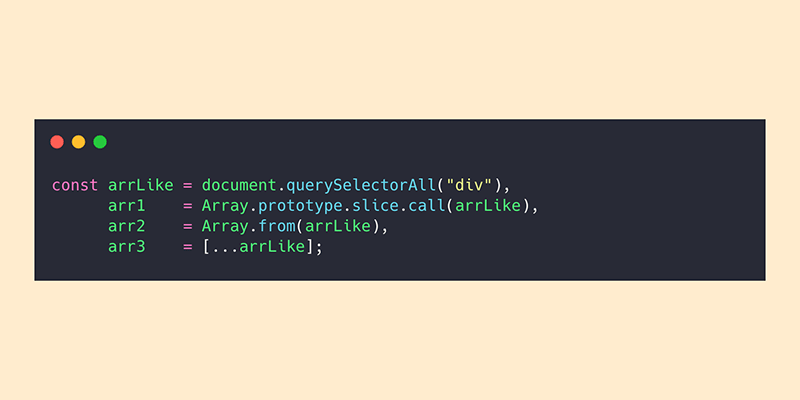
How to find index of element in array javascript. The.indexOf()methodhas an optional second parameter that specifies the index to start searching from, so you can call it in a loop to find all instances of a particular value: function getAllIndexes(arr, val) { var indexes = [], i = -1; while ((i = arr.indexOf(val, i+1)) != -1){ Find Index of Element in Java Array. You can find the index of an element in an array in many ways like using a looping statement and finding a match, or by using ArrayUtils from commons library. In this tutorial, we will go through each of these process and provide example for each one of them for finding index of an element in an array. The findIndex () method executes the callbackFn function once for every index in the array until it finds the one where callbackFn returns a truthy value. If such an element is found, findIndex () immediately returns the element's index. If callbackFn never returns a truthy value (or the array's length is 0), findIndex () returns -1.
Introduction to the JavaScript array indexOf () method To find the position of an element in an array, you use the indexOf () method. This method returns the index of the first occurrence the element that you want to find, or -1 if the element is not found. The following illustrates the syntax of the indexOf () method. The findIndex () method returns the index of the first array element that passes a test (provided by a function). The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, findIndex () returns the index of that array element (and does not check the remaining ... 11/10/2019 · Approach 2: Visit the array elements one by one using reduce() method and if the array elements matches with the given element then push the index into the array. After visiting each element of the array, return the array of indexes. Example: This example uses reduce() method to find the index of all occurrence of elements in an array.
The Array.prototype.findIndex () method returns an index in the array if an element in the array satisfies the provided testing function; otherwise, it will return -1, which indicates that no element passed the test. It executes the callback function once for every index in the array until it finds the one where callback returns true. Array.indexOf and Array.findIndex are similar because they both return the index of the first matching element found in our Array, returning us -1 if it's not found. To check if an element exists, we simply need to check if the returned value is -1 or not. In JavaScript, there are multiple ways to check if an array includes an item. You can always use the for loop or Array.indexOf() method, but ES6 has added plenty of more useful methods to search through an array and find what you are looking for with ease.. indexOf() Method The simplest and fastest way to check if an item is present in an array is by using the Array.indexOf() method.
20/1/2017 · I'm developing an Electron App, and for this specific part of the code, it's necessary that I recover the index of the current element (on click). HTML: <div> <input type="checkbox" class="optionBox"> Minimize to tray after 10 seconds <input type="checkbox" class="optionBox"> Always check day transition </div>. JavaScript: Just like in selenium web driver, javascript also provides some methods to find the elements: getElementById. getElementsByName. getElementsByClass. getElementsByTagName. Here if you observe carefully, we have only one single method (getElementById) which returns the webelement and the rest are returning the list of webelements. In the above code, we first find the index of the element we want to remove and then use the splice() method to remove the array element. Use Array.filter() to Remove a Specific Element From JavaScript Array. The filter methods loop through the array and filter out elements satisfying a specific given condition. We can use it to remove the ...
index will be -1 which means the item was not found. Because objects are compared by reference, not by their values (differently for primitive types). The object passed to indexOf is a completely different object than the second item in the array.. You can use the findIndex value like this, which runs a function for each item in the array, which is passed the element, and its index. The indexOf() method searches an array for a specified item and returns its position. The search will start at the specified position (at 0 if no start position is specified), and end the search at the end of the array. indexOf() returns -1 if the item is not found. The pop() and shift() methods change the length of the array.. You can use unshift() method to add a new element to an array.. splice()¶ The Array.prototype.splice() method is used to change the contents of an array by removing or replacing the existing items and/or adding new ones in place. The first argument defines the location at which to begin adding or removing elements.
An array starts from index 0,So if we want to add an element as first element of the array , then the index of the element is 0.If we want to add an element to nth position , then the index is (n-1) th index. MDN : The splice() method changes the contents of an array by removing or replacing existing elements and/or adding new elements, in the ... Today's Little Program isn't even a program. It's just a function. The problem statement is as follows: Given a nonempty JavaScript array of numbers, find the index of the smallest value. (If the smallest value appears more than once, then any such index is acceptable.) One solution is simply to do the operation manually, Arrays indexOf method finds the index of the first matching element in the array and returns it. If element is not present then -1 is returned. It searches the element with strict equality ===.
findIndex () method The findIndex () method returns the index of the first element in the array that satisfies the provided testing function. Otherwise, it returns -1, indicating no element passed the test. Recently while working I came across a scenario. I had to find index of a particular item on given condition from a JavaScript object array. In this post we will see how to find index of object from JavaScript array of object. Let us assume we have a JavaScript array as following, Now if we… 27/9/2017 · Finally, we can use Array.find() to pass in our isOdd() function on the arr Array: arr.find(isOdd); // 9. As expected, the function finds the odd number and returns 9. Array.findIndex. You’ll soon see that findIndex() works very similarly to find(). Instead of returning the value, findIndex() returns the index of the first element in the array that satisfies the given test. If the test is not satisfied, -1 is returned; Example #1
The findIndex () executes the testFn on every element in the array until it finds the one where testFn returns a truthy value, which is a value that coerces to true. Once the findIndex () finds such an element, it immediately returns the element's index. JavaScript findIndex () examples Here, the if condition is testing if any object in the array is available with id and name equal to the given object. If found, it returns true and moves inside the block.. Using findIndex() : findIndex() method is used to find the index of first element in the array based on a function parameter. If the element is not found, it returns -1. Returns the first index of the element in the array if it is present at least once. Returns -1 if the element is not found in the array. Note: indexOf () compares searchElement to elements of the Array using strict equality (similar to triple-equals operator or === ).
This post will discuss how to find the index of the first occurrence of a given value in an array in JavaScript. 1. Using Array.prototype.indexOf () function The indexOf () method returns the index of the first occurrence of the given element in an array and returns -1 when it doesn't find a match. 2. Binary search: Binary search can also be used to find the index of the array element in an array. But the binary search can only be used if the array is sorted.Java provides us with an inbuilt function which can be found in the Arrays library of Java which will rreturn the index if the element is present, else it returns -1. The complexity will be O(log n). The indexOf () method returns the first index at which a given element can be found in an array. It returns -1 if the element does not exist in the array. Let's go back to our example. Let's find the index of 3 in the array.
The arr.findIndex () method used to return the index of the first element in a given array that satisfies the provided testing function. Otherwise, -1 is returned. It does not execute the method once it finds an element satisfying the testing method. It does not change the original array.
Picking A Random Item From An Array Kirupa Com
How To Use Map Filter And Reduce In Javascript
Javascript Array Find Tutorial How To Iterate Through
Hacks For Creating Javascript Arrays
Javascript Move Item In Array To Another Index Code Example
Python Find Index Of Value In Array Design Corral
Javascript Array Indexof And Lastindexof Locating An Element
Find Index Of Smallest Element In Array Javascript
Java67 How To Remove A Number From An Integer Array In Java
Javascript Indexof Method Explained With 5 Examples To
Equilibrium Index Of An Array Geeksforgeeks
Javascript Array A Complete Guide For Beginners Dataflair
6 2 Traversing Arrays With For Loops Ap Csawesome
Indexed Collections Javascript Mdn
3 Ways To Use Array Slice In Javascript By Javascript Jeep
Should You Use Includes Or Filter To Check If An Array
How To Find The Index Of All Occurrence Of Elements In An
Js Array From An Array Like Object Dzone Web Dev
Javascript Array Indexof Amp Lastindexof Find Element Position
Javascript Array Indexof How To Find Index Of Array In
A List Of Javascript Array Methods By Mandeep Kaur Medium
Arrays The Java Tutorials Gt Learning The Java Language
Array Index In Java Find Array Indexof An Element In Java
Creating Indexing Modifying Looping Through Javascript
Find Element Index In Array Javascript Code Example
How To Check If Array Includes A Value In Javascript
Find The Index Number Of A Value In A Powershell Array
Hacks For Creating Javascript Arrays
How Can We Refer To The Last Elements Of C Array Int Num
0 Response to "32 How To Find Index Of Element In Array Javascript"
Post a Comment