35 Javascript Document Createelement Div
Apr 22, 2017 - I am trying to create a <div> dynamically, with an appended <div> inside. I have this so far which works: var iDiv = document.createElement('div'); iDiv.id = 'block'; iDiv.className = 'block'; document.getElementsByTagName('body')[0].appendChild(iDiv); With document.createElement () method you can create a specified HTML element dynamically in JavaScript. Once created, you can insert (or add) the element to your web page, or add it to a pre-defined element or a dynamically created element. In fact, you can create an entire form dynamically using this method.
Document Createelement Code Example
The document.createElement('style') method is used to create the style element using JavaScript. The steps to create style element are listed below: Steps: Create a style element using the following syntax Syntax: document.createElement('style'); Apply the CSS styles. Append this style element to the head element. Syntax:
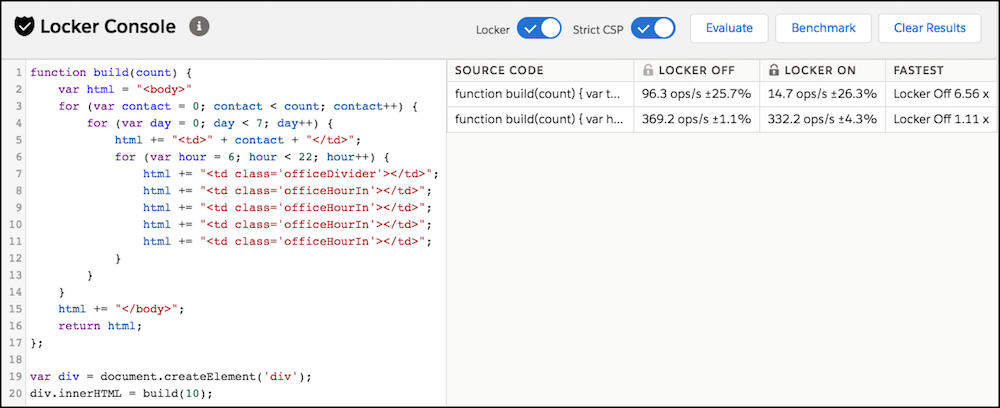
Javascript document createelement div. Create a <p> element and append it to a <div> element: var para = document.createElement("P"); // Create a <p> element. para.innerHTML = "This is a paragraph."; // Insert text. document.getElementById("myDIV").appendChild(para); // Append <p> to <div> with id="myDIV". Try it … Aug 27, 2011 - How can I use JavaScript to create and style (and append to the page) a div, with content? I know it's possible, but how? Yesterday, we looked at how to get an element's CSS attributes with vanilla JavaScript. One thing I neglected to mention: getComputedStyle() can only be used to get values, not set them. Today, let's look at how to set CSS with vanilla JS. Approach 1: Inline Styles The easiest way to set an element's style with JavaScript is using the style property.
17/3/2019 · let div = document.createElement('div');div.classList.add('test');let text = document.createTextNode('Test');div.appendChild(text);document.body.appendChild(div) .test { color: green;} You can also do by className. 29/8/2021 · One way to add a div element to the body element is to set the document.body.innerHTML property. For instance, we can write: document.body.innerHTML += '<div>hello world</div>'; We assign the innerHTML to a string with the code for a div element. Therefore, we’ll see the div rendered in the body. Call the document.createElement Method. Another way to add a div to the body element is to … In an HTML document, the document.createElement () method creates the HTML element specified by tagName, or an HTMLUnknownElement if tagName isn't recognized. Note: In a XUL document, it creates the specified XUL element. In other documents, it creates an element with a null namespace URI.
Oftentimes, we want to add a div element to the body or document of the web page with JavaScript. In this article, we'll look at how to add a div element to the body or document with JavaScript. Set the document.body.innerHTML Property. One way to add a div element to the body element is to set the document.body.innerHTML property. Definition and Usage The appendChild () method appends a node as the last child of a node. Tip: If you want to create a new paragraph, with text, remember to create the text as a Text node which you append to the paragraph, then append the paragraph to the document. Mar 26, 2021 - After the page is loaded such a call erases the document. Mostly seen in old scripts. ... We have an empty DOM element elem and a string text. Which of these 3 commands will do exactly the same? ... Answer: 1 and 3. Both commands result in adding the text “as text” into the elem. ... <div ...
En un documento HTML, el método Document.createElement() crea un elemento HTML especificado por su tagName, o un HTMLUnknownElement (en-US) si su tagName no se reconoce. En un documento XUL, crea el elemento XUL especificado. En otros documentos, crea un elemento con un namespace URI null. To add an element to a div, you create an element and append it to the div using the appendChild () method: let div = document .createElement ( 'div' ); div.id = 'content' ; div.className = 'note' ; // create a new heading and add it to the div let h2 = document .createElement ( 'h2' ); h2.textContent = 'Add h2 element to the div' ; div ... Uwagi. Jeżeli znane są atrybuty o domyślnych wartościach, zostaną automatycznie utworzone i przypisane do elementu węzły tychże atrybutów. Aby utworzyć element o uściślonej nazwie i URI przestrzeni nazw, użyj metody createElementNS.
document. body. onload = addElement; function addElement {// 新しい div 要素を作成します var newDiv = document. createElement ("div"); // いくつかの内容を与えます var newContent = document. createTextNode ("Hi there and greetings!" Creates an element with the specified tag name. After the element is created, use the appendChild or insertBefore method to insert it into a document. If you want to create a CommentNode or TextNode, use the createComment and createTextNode methods. How to createElement('a') and add innerText Can someone show me how to create an 'a' element and append it to a div tag thanks erik body onload=s. I'll cover the following topics in the code samples below: JavaScript, CreateElement, MyLink, Body Onload, and Div Tag.
2/1/2018 · Function in JavaScript that i will be using is as below: createElement(“div”) createTextNode(outputValues); createTextNode(outputValues); document.body.insertBefore(newDiv, currentDiv); In Order to print out the element contains inside the Element line by line. The First thing i will need to do is to check how many element contains inside the Array. tagName A string that specifies the type of element to be created. The nodeName of the created element is initialized with the value of tagName.Don't use qualified names (like "html:a") with this method. When called on an HTML document, createElement() converts tagName to lower case before creating the element. In Firefox, Opera, and Chrome, createElement(null) works like createElement("null"). This code creates a new <p> element: const para = document. createElement ( "p" ); To add text to the <p> element, you must create a text node first. This code creates a text node: const node = document. createTextNode ( "This is a new paragraph." ); Then you must append the text node to the <p> element: para. appendChild (node);
Here I am creating inside my ul ("list") a new li and a new a at the end. I'm in the process of learning javascript and am happy like a small child couse I just fixed it :) //creates new li and new a var newSocial = document.createElement("li"); var newSocialLink = document.createElement("a"); // ads property to the href attribute I want to create new divs as the page loads. These divs will appear as an ordered group which changes depending upon external data from a JSON file. I will need to do this with a for loop because t... Definition and Usage. The createAttribute() method creates an attribute with the specified name, and returns the attribute as an Attr object. Tip: Use the attribute.value property to set the value of the attribute. Tip: Use the element.setAttributeNode() method to add the newly created attribute to an element. Tip: Often, you will want to use the element.setAttribute() method instead of the ...
Here is a tutorial guide to tell you about complete JavaScript codes to create Contact Form. In this tutorial, we have created a form div with id "form_sample" in our html page. In our js code we have created form elements using .createElement function and appended the elements to html div using the .appendChild function of JavaScript. var ... Creating elements using HTML tags isn’t the only way to do it — in fact it’s possible to create, modify and insert elements from JavaScript. Here’s an example that creates a div, adds some text to it and appends it as the last element in the body: var div = document.createElement('div'); ... On many occasions, we want to create and style div elements in our web app dynamically. In this article, we'll look at how to create and style a div using JavaScript. Using the document.createElement Method. We can create an HTML element using the document.createElement method.
Summary: in this tutorial, you'll learn the difference between the innerHTML and createElement() when it comes to creating new elements in the DOM tree. #1) createElement is more performant Suppose that you have a div element with the class container: You can new elements to the div element by creating an element and appending it: […] DOM example: document.createElement The following example uses document.createElement ('div') to dynamically create a <div> HTML tag and insert into the Document Object Model of an HTML document. After creating it, the new element's innerHTML is then assigned the text to be displayed in the div. var element = document.createElement("elementName"); In the above syntax, elementName is passed as a parameter. elementName specifies the type of the created element. The nodeName of the created element is initialized to the elementName value. The document.createElement() returns the newly created element.
The Document method getElementById () returns an Element object representing the element whose id property matches the specified string. Since element IDs are required to be unique if specified, they're a useful way to get access to a specific element quickly. Save Your Code. If you click the save button, your code will be saved, and you get a URL you can share with others.
What Is Dom Beginner Dev Community
How React Can Make You A Better Javascript Developer Infoworks
Building Chrome Extension Mvp Day 2 Inject And Insert Html
Building A React Esque Component Using Vanilla Javascript
An Introduction To The Javascript Dom
Build A Bouncing Basketball App With Anime Js By Chad
Learn A Simple Way To Dynamically Change The Background Color
Questions Custom Component Disappear On Save Vue Js
Please Read All Of The Instructions Solve Using Chegg Com
4 Ways To Strip Amp Remove Html Tags In Javascript
Vscode Intellisense For Bound Document Createelement
Javascript Createelement Not Working In Chrome Stack Overflow
Lightning Aura Components Developer Guide Benchmark
Javascript Goes To Asynchronous City Eternalcoding
How Dry Impacts Javascript Performance Faster Javascript
Create Ul And Li Elements Dynamically Using Javascript
How To Create Amp Append Html Elements In Javascript
无法获取js动态创建的元素的高度 Segmentfault 思否
How Inflation Works Examine The Following Code Public Class
Javascript May Cause Ui Update
How To Dynamically Create New Elements In Javascript
Dom And Dom Selection Create Elements Programmer Sought
Garbage Collection As A Joint Venture
Dom Xss Bug Affecting Tinder Shopify Yelp And Moresecurity
How To Create An Image Element Dynamically Using Javascript
Address Field With Autocomplete Tutorial Jsfiddle Geoapify
Javascript Trying To Append To Html Not Working Stack Overflow
Any Help Is Greatly Appreciated Code In Html Chegg Com
Appendchild Is Not Working With Textcontent Javascript
Create Element Inside Div Javascript Code Example
How To Combine Multiple Elements And Append The Result Into A
Dynamically Create A Table Button And Div In Javascript
0 Response to "35 Javascript Document Createelement Div"
Post a Comment