21 Random Number Generator In Javascript With Range
Dec 28, 2020 - Math.random() is a function that returns a pseudo-random floating numbers between 0 and 1 (0 is inclusive, 1 is exclusive) We usually multiply the result with other numbers to scale the randomized value. However, there.... Random Number Generator. Its the core of all randomness. Pick a number or generate a whole sequence of numbers within a minimum and maximum value (inclusive) while including or suppress duplicates. Your device is used to quickly generate these numbers, completely random and unique to you every time.
Generate A Random Number In A Range In Javascript
Jun 04, 2017 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers.
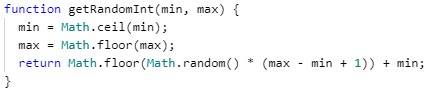
Random number generator in javascript with range. We have used the Math.random() method to generate the random values and Math.floor method to round a number downward to its nearest integer. You have to pass the min and max values in the parameter of the function to generate a random number between those values. 2. Get a random number between 0 to n number. Use the below method to get a random ... Generating a random number within a range You may have often wondered how to generate a random number from within a certain range. This is exactly what we will look at in this recipe; we will obtain a random number that resides in an interval between a minimum (min) and maximum (max) value. 5/10/2009 · Question : Function to Generate Random Whole Numbers in JavaScript within a Range of 5 to 25. General Overview : (i) First convert it to the range - starting from 0. (ii) Then convert it to your desired range ( which then will be very easy to complete). So basically, if you want to generate Random Whole Numbers from 5 to 25 then :
Math.random () in JavaScript generates a floating-point (decimal) random number between 0 and 1 (inclusive of 0, but not 1). Let's check this out by calling: console .log ( Math .random ()) This will output a floating-point number similar to: 0.9261766792243478. Jul 09, 2020 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Learn to Code — For Free
JavaScript: get a random number from a specific range - random.js The random () method returns a random number from 0 (inclusive) up to but not including 1 (exclusive). The Math.random() function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately uniform distribution over that range — which you can then scale to your desired range. The implementation selects the initial seed to the random number generation algorithm; it cannot be chosen or reset by the user.
A Proper Random Function. As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes. This JavaScript function always returns a random number between min (included) and max (excluded): This article describes how to generate a random number using JavaScript. Method 1: Using Math.random () function: The Math.random () function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive). This random number can then be scaled according to the desired range. Here we are going to generate the random numbers, to do this we have Math.random () method. It will generate the floating-point, pseudo-random number in the range from 0 inclusive up to but not including 1. Note: Random number generated by Math.random () function not cryptographically secure. To generate secure random number click here.
node js function pick a random number in range; javascript random number generator from 1 to 6; random number javascript between 1 and 6; randomnise setinterval; random integer num javascript; javascript randon between; javascript random number between 10-100; js random between 1 and 9.999.999; javascript generate numbers in range; random ... Generate Random Numbers Within a Range using JavaScript Tweet: A formula for generating a random number within a given range using JavaScript is as follows: Math.floor(Math.random() * (UpperRange - LowerRange + 1)) + LowerRange; So to generate a random number between 25 and 75, the equation would be . A mathematically correct random number generator library for JavaScript. - GitHub - ckknight/random-js: A mathematically correct random number generator library for JavaScript.
Let us see some of the examples to generate random numbers: 1. Use of Math.random () function. We have the Math. random () function in JavaScript to deal with the random numbers. This number always return less than 1 as a result. This will always give the result in the form of a decimal point. 2. Sep 25, 2017 - In this article, we will be generating a random integer between given two numbers using JavaScript. We will be generating a random number in a given range using JavaScript Math Object. We often need to generate a random number within a specified range in realtime applications. The logic dealt ... May 17, 2020 - Generate a random number in a range. The way we all wish Math.random worked.
Task: Generate a random number within the following range (30-75) in TestComplete using a scripting language of your choice. This is a solution created for [TechCorner Challenge #9] The following Vb Script code demonstrates how to generate random integers that fall within a specified range from 30 to 75. 1/4/2021 · In this article, I’ll talk about how to use Math.random() to generate a random number between two numbers. “The Math.random() function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately uniform distribution over that range — which you can then scale to your desired range.” Math.random () = 0.8244326990411024. (myMax - myMin + 1) = 10 - 1 + 1 -> 10. a * b = 8.244326990411024. c + myMin = 9.244326990411024. Math.floor (9.244326990411024) = 9. randomRange should use both myMax and myMin, and return a random number in your range. You cannot pass the test if you are only re-using the function ourRandomRange inside ...
In JavaScript, you can use the Math. random () function to generate a pseudo-random floating number between 0 (inclusive) and 1 (exclusive). If you want to get a random number between 0 and 20, just multiply the results of Math.random () by 20: To generate a random whole number, you can use the following Math methods along with Math.random (): Generating Javascript Random Numbers More Easily. Math.random() is a useful function, but on its own it doesn't give programmers an easy way to generate pseudo-random numbers for specific conditions. There may be a need to generate random numbers in a specific range that doesn't start with 0, for example. Generating n random numbers between a range - JavaScript. Javascript Web Development Front End Technology Object Oriented Programming. ... Generate n random numbers between a range and pick the greatest in JavaScript; Generating random numbers in Java;
JavaScript fundamental (ES6 Syntax): Exercise-33 with Solution. Write a JavaScript program to get a random number in the specified range. Use Math.random() to generate a random value, map it to the desired range using multiplication. Sample Solution: JavaScript Code: Random Integer Between X and Y (Exclusive Y) Let's say you want to generate an integer in a range between X and Y. For example, a number larger than 1024, but no bigger than 49151. In this lesson we use the JavaScript pre-defined function Math.random () to generate a pseudo-random number. "Pseudo" means "not genuine" or "a sham". Math.random uses an algorithim to simulate randomness. Because an algorithim is used, there is no way to produce actual randomness. Random means "chosen without method or conscious decision".
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. This post covers ways you can generate floats, integers and big integers (BigInts) within a certain range (min, max) using pure javascript (no dependencies). Floats Generate random float between 0 and 1 Generate random number using JavaScript Example: Here we have create a function getRandomNumber () which accepts two parameters start, end ie. range of number you want between. The above function will generate a random number between start (including) and end (including). So you can add any range that you wish to generate number between.
Algorithm to Generate Random Number in a Specified Range in JavaScript. Generate a number between the range 0 and 1 and store it in a variable named rand. Calculate the total range range as max-min+1. Multiply range by rand and add it to min to get the random number inside the specified range. A way to randomly generate two numbers in a range. A lot of times you will need to create a random number in JavaScript within a given range. In fact, let's say you need to randomly calculate a basketball result, you know that score will be between 70 and 130 (for example ;)). So how do you generate numbers from a range. how to do a random number generator in js. C1 = Math.floor (Math.random () * 100 + 1); random number between 0 and 2 javascript. javascript random number between 1 and 300. random number in jquery. generate random number between 1 and 100 javas. generate random number between 1 and 100 javascript. rand function js.
Aug 28, 2018 - A short guide to .ceil, .floor, and .round. “Using Math.random() in JavaScript” is published by Joe Cardillo. 2. Generating Random Numbers in a Range. 2.1. Math.random. Math.random gives a random double value that is greater than or equal to 0.0 and less than 1.0. Let's use the Math.random method to generate a random number in a given range [min, max): public int getRandomNumber(int min, int max) { return ( int) ( (Math.random () * (max - min)) + min); } function myRandomIntByMax(n){return Math.floor(Math.random() * n) + 1} myRandomIntByMax(15) // 14. Alright. We've got a general-purpose random number generator, and we know how to adjust the range, now comes the hard part. What I want is to be able to ask for some quantity of unique random numbers. So I want to be able to ask for, say, six ...
This method will behave in the following ways: maxExcusive is exclusive, so for example Random.Range(0, 10) will return a value between 0 and 9, each with approximately equal probability.; If minInclusive and maxExclusive are equal, then the "exclusive rule" is ignored and minInclusive will be returned.; If minInclusive is greater than maxExclusive, then the numbers are automatically swapped. Nov 19, 2020 - Whether for performance testing or any other purpose, it is sometimes useful to generate pseudorandom numbers. Dr. Derek Austin 🥳 ... “The Math.random() function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately ... Apr 01, 2018 - For many situations ranging from coin toss operations to procedural animations, you will want to work with numbers whose values are a bit unpredictable. You will want to work with numbers that are random. Generating a random number is easy. It is built-in to JavaScript and exposed via the infamous ...
Jul 21, 2020 - Use Javascript to return a random number between 0 and 100. ... Math.Random() returns a value between 0 and 1. ... Generate random whole numbers in a range JS.
How To Generate Random Whole Numbers Within A Range Using
Generate Random Numbers In Javascript Using Crypto Without
How To Generate Gaussian Distributed Numbers Alan Zucconi
Basic Javascript 105 111 Generate Random Whole Numbers Within A Range Freecodecamp
React Native Generate Random Number Between 1 To 100 In
Visual Basic Net Tutorial 39 How To Make A Random Number Generator Within Range In Vb Net
Random Number In Javascript Practical Example
Distinct Think Time Setting For Virtual User Pacing Web
Random Number Generator In Javascript Top 6 Examples To Learn
How To Generate Random Numbers With Decimals In Excel
How To Generate 3 Random Whole Numbers Within A Given Range
Javascript Lesson 5 Generating Random Numbers
Random Number Generator Rng Randomdraws Com
Easy Programming Beginner C Tutorial Random Number Generator 11
How To Implement A Random Number Generator In Php Edureka
Weighted Random Algorithms For Sampling From Discrete
Random Number Between Range In Javascript Red Stapler
React Native Generate Random Number Between 1 To 100 In
Generate Useful Random Numbers In Javascript Javascript
Working With Random In Python Generate A Number Float In
0 Response to "21 Random Number Generator In Javascript With Range"
Post a Comment