21 Javascript Getter Setter Best Practice
@Getter @Setter @Builder @NoArgsConstructor @AllArgsConstructor @ToString @EqualsAndHashCode public class PricingAndCosting { //variables and constants } is it better practice to just do the following to get the same functionality: @Entity @Data public class PricingAndCosting { //variables and constants } Pre-C# 6. I'd use the last of these, for a trivial property. Note that I'd call this a public property as both the getters and setters are public.. Immutability is a bit of a pain with automatically implemented properties - you can't write an auto-property which only has a getter; the closest you can come is:
How Can We Generate Getters And Setters In Visual Studio
typescript extension getter; typescript getter/setter best practice; ... get a span inside a div with div id javascript; ets2 iInvalid floating point value '&7fc00000' battle cats challenge battle; ... getter and setter in typescript 'mat-form-field' is not a known element:
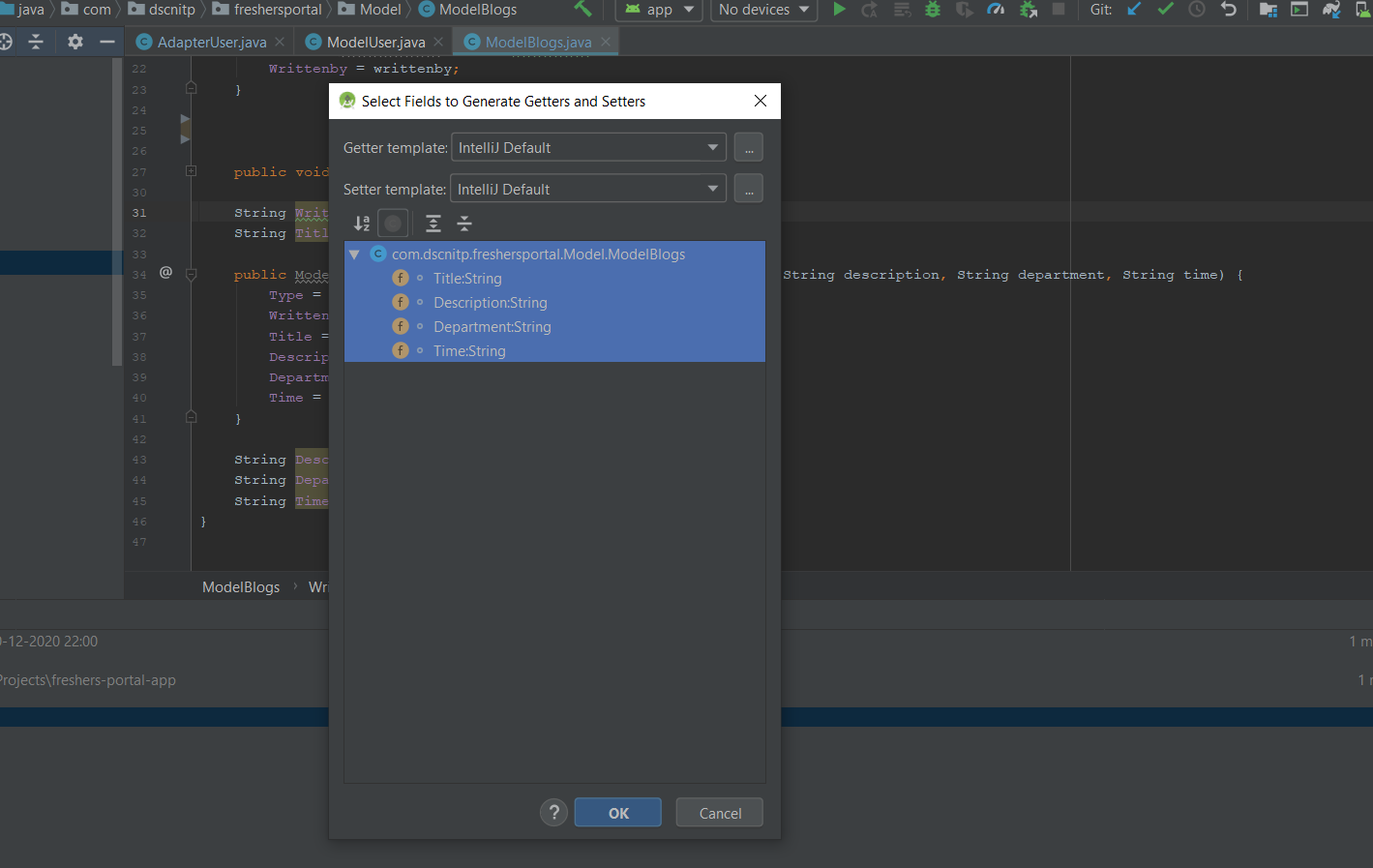
Javascript getter setter best practice. May 15, 2020 - Of course, you can safely use getters/setters in the environment, which guarantees static type check, but this article is about vanilla JavaScript. This article is my opinion why I think this feature shouldn’t arrive to the vanilla JavaScript in the first place. Nov 01, 2019 - In this post, we take a closer look at getter and setter methods in Java, common mistakes, and best practices for combating these frequent misconceptions. 8/8/2006 · Implementing getters / setters as part of the API (eg. vor VO, DTO) should be allowed practice because it still encapsulates a bit ==> it hides direct access to data, but still allows to modify and fetch data for an object at finer granularity, and the developer of the class that contains getter/setter still has the possibilities to eg. validate input data and react with exceptions before actually setting.
Apr 23, 2019 - Getters and setters exist in most object-oriented programming languages, including JavaScript. They are code constructs that help developers access the properties of objects in a secure way. With getters, you can access (“get”) the values of properties from external code, while setters ... Jan 24, 2021 - ERROR in The Angular Compiler requires TypeScript >=4.0.0 and <4.1.0 but 3.4.5 was found instead · Cannot find name 'require'. Do you need to install type definitions for node? Try `npm i @types/node` and then add `node` to the types field in your tsconfig · ERROR in The Angular Compiler ... In JavaScript, a setter can be used to execute a function whenever a specified property is attempted to be changed. Setters are most often used in conjunction with getters to create a type of pseudo-property. It is not possible to simultaneously have a setter on a property that holds an actual value. Note the following when working with the set ...
Apr 12, 2019 - Getters and setters are functions or methods used to get and set the values of variables. The getter-setter concept is common in computer programming: Oct 07, 2018 - I cant solve this challenge help!!! Use class keyword to create a Thermostat class. The constructor accepts Fahrenheit temperature. Now create getter and setter in the class, to obtain the temperature in Celsius scale. Remember that C = 5/9 * (F - 32) and F = C * 9.0 / 5 + 32, where F is the ... In JavaScript, this can be accomplished with the use of a getter. It is not possible to simultaneously have a getter bound to a property and have that property actually hold a value, although it is possible to use a getter and a setter in conjunction to create a type of pseudo-property. Note the following when working with the get syntax:
C# getter/setter best practices. Getters, setters, and properties best practices. Java vs. C#, Pre-C# 6. I'd use the last of these, for a trivial property. Note that I'd call this a public property as both the getters and setters are public. Regardless of which way you choose in C# the end result is the same. 1. Add a comment. |. 2. This is part opinion, part the requirements of your application. It is certainly not bad to use getters and setters. But I would also use them with discretion and in most cases getters and setters may be unnecessary. In the example code you provide, there is no reason to use a getter and setter. 30/9/2019 · So providing getter and setter is necessary when integrating your code with such frameworks. 3. Naming convention for getter and setter The naming scheme of setter and getter should follow Java bean naming convention as follows: getXXX() and setXXX() where XXX is name of the variable. For example with the following variable name: private String name; then the appropriate setter and getter will be: public void …
Accessors - getters/setters. TypeScript supports getters/setters as a way of intercepting accesses to a member of an object. This gives you a way of having finer-grained control over how a member is accessed on each object. Let's convert a simple class to use get and set. class Employee { private _id: number ; private _fullName: string ... May 04, 2016 - Honest question here) If you work with Java, you'll agree with me this is how getters & setters are used in practice, common enough that we cannot argue it's just "bad programmers" that do it ;) Frameworks like Hibernate make this problem even worse. – Andres F. 29/1/2016 · It is basically a code generation framework that generates the getter and setter methods for you behind the scenes. It also can generate other methods such as toString(), equals(), and hashCode().
JavaScript Getter and Setter. In this tutorial, you will learn about JavaScript getter and setter methods with the help of examples. In JavaScript, there are two kinds of object properties: Data properties; Accessor properties; Data Property. Here's an example of data property that we have been using in the previous tutorials. The above article provides a clear understanding of the use of getters and setters in java. Using getter and setter is considered as best practice in java development. We use getters and setters in our application as they help create secure, roust and maintainable code. Recommended Article. This is a guide to Java Getter Setter. Jul 01, 2018 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers.
14/1/2016 · Yes, a getter or setter can be very useful at times, but they only need to be used when their specific functionality is required - otherwise plain property access without a getter or setter can be just fine. A getter has use when you want to run some code every time a property is requested. In your example, the getter always returns an uppercase version of the name regardless of what the case of the name is, while … Jul 07, 2016 - I don’t think that this concept is wrong in general, but I think it’s not very well suited for JavaScript. It might look like getters and setters are a time saver and simplification of your code, but actually they bring hidden errors which are not obvious from the first look. Sep 25, 2020 - One way to implement getters and setters is to use methods, like it’s done in Java. However, since ES5, we have a much more elegant and practical alternative available by using the get and set syntax. Well, let’s get started! ... In JavaScript, objects are collections of properties and ...
Best practices: Accessors vs. methods. Close. 13. Posted by 3 years ago. Archived. ... Until IDE tooling is consistently able to mark getters/setters as such, I'm not using it in any codebase. 8. Share. Report Save. ... TypeScript is a language for application-scale JavaScript development. TypeScript is a typed superset of JavaScript that ... Getters and Setters in Java Explained. Getters and setters are used to protect your data, particularly when creating classes. For each instance variable, a getter method returns its value while a setter method sets or updates its value. Given this, getters and setters are also known as accessors and mutators, respectively. Getters and Setters are Best Practice In a language like Java, accessor and mutator methods like getValue and setValue are considered a part of life. Tools even offer to automatically generate them for you based on a class' internal properties.
25/8/2021 · Javascript getter setter best practice. How To Create Constructor Getter Setter Methods And New Best Practice For Error Handling In Modern Javascript Prog Getter And Setter Stack Overflow Generate Rename And Delete Getters Setters Instantly In Javascript Es6 Classes Objects In Programming Languages Getters Setters Evil Period C# getter/setter best practices. Getters, setters, and properties best practices. Java vs. C#, Pre-C# 6. I'd use the last of these, for a trivial property. Note that I'd call this a public property as both the getters and setters are public. Regardless of which way you choose in C# the end result is the same. (As for Javascript, Lists or Objects are still mutable but cannot be redefined). C++ getter/setter best practice. Conventions for accessor methods (getters and setters) in C++ , Don't use accessors at all. We can just make the respective member variables public. Use Java-style get/set methods.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. 4/7/2019 · When you pass a plain JavaScript object to a Vue instance as its data option, Vue will walk through all of its properties and convert them to getter/setters using Object.defineProperty. The getter/setters are invisible to the user, but under the hood they enable Vue to perform dependency-tracking and change-notification when properties are accessed or modified. TypeScript Getters Setters with TypeScript Accessor Tutorial. Posted by robert | Filed under TypeScript. Update: New and better Boilerplate that uses ES6 Modules in TypeScript check it out.. In this TypeScript tutorial I will be going over TypeScript Getters and Setters which are called TypeScript Accessor.. To get TypeScript Accessor's working we need to let the compiler know we want to ...
To avoid repeating the check, you can use setters and getters. The getters and setters allow you to control the access to the properties of a class. For each property: A getter method returns the value of the property's value. A getter is also called an accessor. A setter method updates the property's value. A setter is also known as a mutator. Get and Set. You learned from the previous chapter that private variables can only be accessed within the same class (an outside class has no access to it). However, it is possible to access them if we provide public get and set methods.. The get method returns the variable value, and the set method sets the value.. Syntax for both is that they start with either get or set, followed by the ... Typescript getter/setter best practice. TypeScript & JavaScript Getters and Setters: Are they useless , He frequently publishes articles about Domain-Driven Design, software design and Advanced TypeScript & Node.js best practices for large-scale applications. TypeScript supports getters/setters as a way of intercepting accesses to a member of ...
C# getter/setter best practices. Getters, setters, and properties best practices. Java vs. C#, Pre-C# 6. I'd use the last of these, for a trivial property. Note that I'd call this a public property as both the getters and setters are public. Regardless of which way you choose in C# the end result is the same. Getters and setters (also known as accessors) were introduced to JavaScript when ECMAScript 5 (2009) was released. The thing is, there's a lot of confusion about their utility and why you would ever even want to use them. I came across this reddit thread where the discussion was about if they were an anti-pattern. That's why it has become good practice to have getter and setter methods for a variable and never allow accessing a variable directly! Look at the following code: 1. class YourClass {2.
Dec 23, 2013 - These two keywords define accessor functions: a getter and a setter for the fullName property. When the property is accessed, the return value from the getter is used. When a value is set, the setter is called and passed the value that was set. It's up to you what you do with that value, but ... Dec 08, 2017 - Let's make getters and setters our last, rather than first, resort. Aug 07, 2013 - I'd be grateful for any comments on whether the code is written in accordance to JS best practices and whatnot. More specifically, I didn't implement getters or setters per se, is my approach still sound?
Aug 28, 2015 - Hi guys, Let's say we have an object as follows: We can see that with this object, we have two areas that can have possible side effects. When we … When this happens, the getter is triggered. JavaScript calls the getter, but the getter is then told to do the exact same thing: get the property that it is meant to handle. The function always calls itself, creating infinite recursive calls. In case 2.2 The same idea applies to the setter. //case2.1.
How Should I Set Up Getter And Setter Which Is Key Value
Best Practices For Java Getter And Setter Dzone Java
Getter And Setter In Java Basics Common Mistakes And Best
Watch Javascript Essentials Prime Video
How To Create Constructor Getter Setter Methods And New
Typescript Amp Javascript Getters And Setters Are They Useless
Getter Setter In Typescript Without Class Stack Overflow
Building A Project With Javascript Objects Dev Community
Javascript Getters And Setters Mosh
Display Attributes Getter Setter In Classes And Display The
Copying Properties From One Object To Another Including
Best Practices For Writing Custom Actions In Mendix By
What Are Getters And Setters In Javascript Dhananjay Kumar
Javascript Es6 Classes Objects In Programming Languages
Inheritance Setter And Getter In Java Code Example
How Can We Generate Getters And Setters In Visual Studio
Cs2 Discussion Features Getters And Setters Issue 4915
Javascript Class Getters And Setters
Getters And Setters Used In Javascript Objects How Dev
Property Getter And Setter Kotlin
0 Response to "21 Javascript Getter Setter Best Practice"
Post a Comment