29 Find Data Type Javascript
1 week ago - The following table summarizes the possible return values of typeof. For more information about types and primitives, see also the JavaScript data structure page. Note: ECMAScript 2019 and older permitted implementations to have typeof return any implementation-defined string value for non-callable ... Feb 01, 2018 - How to better check data types in javascript. Lots of code examples on how and workarounds for bugs with javascripts typeof operator.
How To Check If A Variable Is An Array In Javascript
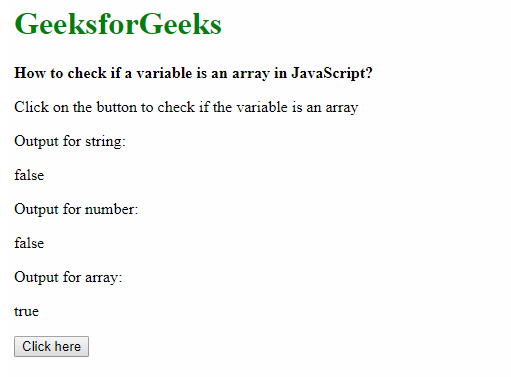
Find data type javascript. Use typeof to Check JavaScript Data Types at Runtime JavaScript is a dynamically typed language. In other words, the data type of JavaScript variables can change over the course of a program's execution. In short, the answer in typeof. In JavaScript, the typeof operator returns the data type of its operand in the form of a string. The operand can be any object, function, or variable. By specification, object property keys may be either of string type, or of symbol type. Not numbers, not booleans, only strings or symbols, these two types. Till now we've been using only strings. Now let's see the benefits that symbols can give us. Symbols. A "symbol" represents a unique identifier.
Jul 24, 2019 - JavaScript (JS) is a lightweight, interpreted, or just-in-time compiled programming language with first-class functions. The Concept of Data Types. In programming, data types is an important concept. To be able to operate on variables, it is important to know something about the type. Without data types, a computer cannot safely solve this: Code language: JavaScript (javascript) In this example, we called the filter () method of the cities array object and passed into a function that tests each element. Inside the function, we checked if the population of the each city in the array is greater than 3 million. If it is the case, the function returns true; Otherwise, it returns false ...
Jun 06, 2020 - JavaScript has a few built-in types, including numbers, strings, booleans, objects. Using the typeof operator we can check what is the type of a value assigned to a variable. For example: typeof 'test' Note that it’s not a function, it’s an operator, so parentheses are not required. In JavaScript, the data types of variable are not specified with declaration of variables. In fact, a variable does not have a fixed data type. The same JavaScript variables can be used to store any type of data at any time. JavaScript assigns to the variable, the data type of the value that you assign. Now you can import this Sys code in a Sys.js file. then you can use this Sys object functions to find out the type of JavaScript Objects . you can also check is Object is Defined or type is Function or the Object is Empty... etc. Sys.isObject; Sys.isArguments; Sys.isFunction; Sys.isString; Sys.isArray; Sys.isUndefined; Sys.isDate; Sys.isNumber; Sys.isRegExp
JavaScript provides different data types to hold different types of values. There are two types of data types in JavaScript. Primitive data type; Non-primitive (reference) data type; JavaScript is a dynamic type language, means you don't need to specify type of the variable because it is dynamically used by JavaScript engine. You need to use ... Just like in selenium web driver, javascript also provides some methods to find the elements: getElementById. getElementsByName. getElementsByClass. getElementsByTagName. Here if you observe carefully, we have only one single method (getElementById) which returns the webelement and the rest are returning the list of webelements. The find () method returns the value of the first element in the provided array that satisfies the provided testing function. If no values satisfy the testing function, undefined is returned. If you need the index of the found element in the array, use findIndex (). If you need to find the index of a value, use Array.prototype.indexOf ().
Below you can find the Javascript cheat sheet in .pdf as well as in the text. JavaScript Cheat Sheet. Download Link; JavaScript Basics. Let's start off with the basics - how to include JavaScript in a website. ... Data Types. Variables can contain different types of values and data types. Below is the list of primitive data types: null. undefined. number. boolean. string. symbol. The symbol is the data type that exists only in the ES6 or in simple terms we can say that symbol is available from the ES6. Also, one complex data type is also existing in JavaScript and that complex data type is object. The arr.find () method is used to get the value of the first element in the array that satisfies the provided condition. It checks all the elements of the array and whichever the first element satisfies the condition is going to print.
Find the type of a variable - null, string, array etc. Javascript provides 2 operators to check the type of a given value : typeof : This checks whether the value is one of the primitive data types. It will return a string specifying the type — "undefined" / "string" / "number" / "boolean" / "object" etc. instanceof : This checks the "kind ... To select the multiple elements with the same data attribute name, we need to use the document.querySelectorAll () method by passing a [data-attribute] as an argument. Example: const elements = document.querySelectorAll(" [data-id]"); console.log(elements); In the above example, the querySeletorAll () method returns the multiple elements in an ... Feb 26, 2020 - JavaScript exercises, practice and solution: Write a JavaScript function which accepts an argument and returns the type.
JavaScript data types. Before looking at type-checking with typeof, it is important to have a glance at the JavaScript data types. Although this article does not go into details about the JavaScript data types, you can glean a thing or two as you progress. Prior to ES6, JavaScript had six data types. In the ES6 specification, the Symbol type ... Apr 28, 2021 - typeof is a JavaScript keyword that will return the type of a variable when you call it. You can use this to validate function parameters or check if variables are defined. There are other uses as well. The typeof operator is useful because it is an easy way to check T he primitive data type symbol is a unique identifier, useful for creating keys on objects in JavaScript. "A symbol value may be used as an identifier for object properties; this is the data type's only purpose." — MDN web docs. As one would expect, the typeof Symbol() is indeed "symbol":
Nov 20, 2016 - As you know, we can create an object of any function using the new keyword. Sometimes you want to know the type of an object to perform some action on it. Use the typeof operator to get the type of an object or variable in JavaScript. ... The typeof operator also returns the object type created ... Javascript function to check for all letters in a field. To get a string contains only letters (both uppercase or lowercase) we use a regular expression (/^ [A-Za-z]+$/) which allows only letters. Next the match () method of string object is used to match the said regular expression against the input value. Here is the complete web document. Summary. There are 8 basic data types in JavaScript. number for numbers of any kind: integer or floating-point, integers are limited by ± (2 53 -1). bigint is for integer numbers of arbitrary length. string for strings. A string may have zero or more characters, there's no separate single-character type.
In JavaScript, different data types require distinct forms of checking. Strings, numbers, booleans and functions can be easily checked by using the typeof operator. For null and undefined, you can use a simple comparison with the strict equality operator. Arrays can be recognized by using the Array.isArray static method Object: It is the most important data-type and forms the building blocks for modern JavaScript. We will learn about these data types in detail in further articles. Variables in JavaScript: Variables in JavaScript are containers that hold reusable data. It is the basic unit of storage in a program. The above query will match documents where the field value is any of the listed types. The types specified in the array can be either numeric or string aliases. See Querying by Multiple Data Type for an example.. Available Types describes the BSON types and their corresponding numeric and string aliases.
The find () method returns the value of the array element that passes a test (provided by a function). The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, find () returns the value of that array element (and does not check the remaining values) 10/11/2020 · JavaScript Data Types. Let's take a quick look at JavaScript data types before we dig into the typeof operator. In JavaScript, there are seven primitive types. A primitive is anything that is not an object. They are: String; Number; BigInt; Symbol; Boolean; undefined; null; Everything else is an object – even including array and function. Delphi answers related to "how to find a data type in javascript". get current date + 1 js. javascript 1 + "1". javascript add minutes to date. javascript all type of data. javascript clone date. javascript convert minus to plus. javascript datum addieren. javascript diffence between a++ and ++a.
May 02, 2017 - But on could add those to the type_of_map as needed. I hope that helps! ... "use strict"; /** * * @author xgqfrms * @license MIT * @copyright xgqfrms * @created 2020-06-09 * @modified * * @description js data type checker * @augments * @example * @link * */ const dataTypeChecker = (data, debug ... The typeof operator is used to get the data type (returns a string) of its operand. The operand can be either a literal or a data structure such as a variable, a function, or an object. The operator returns the data type. Syntax. typeof operand or typeof (operand) The constructor property returns the constructor function for all JavaScript variables. Which function is used to add functionality to a custom component? ... how to Create a class called Person which defines the generic data and functionality of a human with multiple interests in javascript
You will learn more about the functions in JavaScript functions chapter. ... The typeof operator can be used to find out what type of data a variable or operand contains. It can be used with or without parentheses (typeof(x) or typeof x).
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
Confusion In Regards To Javascript Data Type And Types Of
Javascript Data Types Grab Complete Knowledge About Data
Referenceerror Can T Find Variable Stack Overflow
Enhanced Searching Using Find Amp Findindex In Javascript In
Javascript Journey Hour 1 Javascript Has Typed Values
How To Check Data Types In Javascript Using Typeof Code Leaks
Javascript Array Indexof And Lastindexof Locating An Element
Comparing Varchar Max Vs Varchar N Data Types In Sql Server
If I Cant Read It Your Doing It Wrong This Is Why
How To Check Data Types In Javascript Using Typeof By Dr
Javascript Data Types Grab Complete Knowledge About Data
Javascript Data Types Dev Community
Javascript Datatypes Primitive Vs None Primitive Kamp Blog
How To Check Data Types In Javascript Using Typeof Code Leaks
How To Avoid Javascript Type Conversions
How To Work With Date And Time In Javascript Using Date
Indexed Collections Javascript Mdn
Javascript Data Types Examples Tuts Make
Programminghunk Data Types In Javascript
Various Kinds Of Data Types In Javascript And Their Utility
Javascript In Lt Head Gt Or Lt Body Gt Prezentaciya Onlajn
Data Tyes Amp Primitive Types Javascript
The Pandas Dataframe Make Working With Data Delightful
Plotly Javascript Graphing Library Javascript Plotly
0 Response to "29 Find Data Type Javascript"
Post a Comment