23 What Does Do In Javascript
Nov 04, 2011 - In JavaScript, logical operators are used for boolean logic where a boolean value may be returned depending on the outcome of an expression. With the || (OR) operator, since values don't need to be explicitly true or false (they can be truthy or falsy), the operator can return non-boolean results ... Javascript Web Development Front End Technology. The Modulus operator (%) outputs the remainder of an integer division. Example. You can try to run the following code to learn how to work with Modulus (%) operator.
What The Hell Does Console Error Bind Console Do
Updated July 03, 2019 The dollar sign ($) and the underscore (_) characters are JavaScript identifiers, which just means that they identify an object in the same way a name would. The objects they identify include things such as variables, functions, properties, events, and objects.
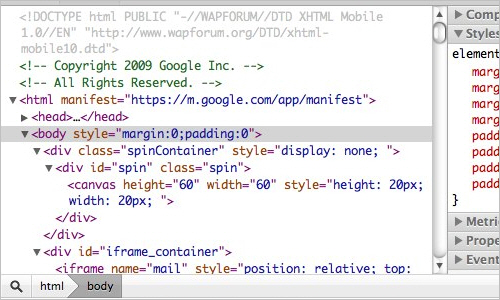
What does do in javascript. JavaScript allows you to take ordinary web elements and make them interactive. It can be used alongside your HTML and CSS and is an extremely important tool for any web developer. To complete the example above, JavaScript code would be used to make your login button perform the required actions (logging a user in when it is clicked). As JavaScript developers, we often have "use strict" - strict mode enabled in our projects. But do we know what does that expression does?. Let's find out. What is a strict mode? JavaScript's strict mode makes JS be executed in strict mode, which helps identify common mistakes in coding practices which might lead to potential bugs. Syntax "use ... 1 week ago - The standard arithmetic operators ... as they do in most other programming languages when used with floating point numbers (in particular, note that division by zero produces Infinity). For example: ... In addition to the standard arithmetic operations (+, -, *, /), JavaScript provides the ...
In classical programming, the logical OR is meant to manipulate boolean values only. If any of its arguments are true, it returns true, otherwise it returns false. In JavaScript, the operator is a little bit trickier and more powerful. But first, let's see what happens with boolean values. Ah, that little dot! Every line of JavaScript you write contains something like foo.bar, foo.bar(), or foo.bar = baz. But do you know what it does? Two concepts interact subtly here: prototypical inheritance and accessor properties (getters/setters). There are some weird corner cases, and I bet you don't know all of them! In this post series, I explain the behavior of foo.bar, foo.bar(), and ... Java Script is a Dynamic Scripting language used along with HTML. It is an interpreted language that is used to add dynamics content in web pages. There are a number of events that will trigger the execution of JavaScript. The JavaScript client-si...
16/1/2012 · The remainder operator returns the remainder left over when one operand is divided by a second operand. It always takes the sign of the dividend, not the divisor. It uses a built-in modulo function to produce the result, which is the integer remainder of … Aug 02, 2018 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers. Below, is my attempt to explain what do the three dots do in JavaScript. Hopefully, this removes the fog around the concept, for people who will find this article in the future and have the same question. Updates. Added more explicit usage of Array.prototype.slice.call(arguments). Thanks @gabriel for commenting and providing feedback.
3 weeks ago - JavaScript uses the double ampersand (&&) to represent the logical AND operator. ... If a can be converted to true, the && operator returns the b; otherwise, it returns the a. In fact, this rule is applied to boolean values. Apr 30, 2015 - What is the purpose of template literals (backticks) following a function in ES6? ... JavaScript property access: dot notation vs. brackets? ?., ?.[], ?.() — Question mark, dot: optional chaining operator ... {...props} — Javascript Property with three dots (…), What does the '…rest' ... Observables represent a progressive way of handling events, async activity, and multiple values in JavaScript. Observables are really just functions that throw values. Objects called observers define callback functions for next (), error (), and complete (). These observer objects are then passed as arguments to the observable function.
Let's explore what the return keyword in JavaScript does. Posted on March 31, 2021. The return keyword in JavaScript is a keyword to build a statement that ends JavaScript code execution. For example, suppose you have the following code that runs two console logs: Mathematical operations are among the most fundamental and universal features of any programming language. In JavaScript, numbers are used frequently for common tasks such as finding browser window size dimensions, getting the final price of a monetary transaction, and calculating the distance between elements in a website document. A short guide on what a colon does in JavaScript language. In the above example, the colon symbol is used to delimit the properties of the car object. The property brand has the value "Toyota" while the property color has the value "red", and both used a colon to separate between the key and the value.. The following assignment expressions also do the same thing as the above:
A JavaScript function is a block of code designed to perform a particular task. A JavaScript function is executed when "something" invokes it (calls it). The JavaScript includes operators as in other languages. An operator performs some operation on single or multiple operands (data value) and produces a result. The tilde in JavaScript is actually surprisingly useful for shorthand determining the truthiness of a value.
Aug 05, 2015 - I am looking at these lines of code from here: if (callback) callback(sig || graph); I have never see vertical "or" bars in a javascript method call. What do they mean? Do they pass t... In the living world of object-oriented programming we already know the importance of classes and objects but unlike other programming languages, JavaScript does not have the traditional classes as seen in other languages. But JavaScript has objects and constructors which work mostly in the same way to perform the same kind of operations. Jan 28, 2021 - I've noticed that if you do something in JS like below, no error will be thrown. let num = 0; if(n... Tagged with javascript, help, beginners, explainlikeimfive.
Apr 20, 2018 - In most languages it works the following way: If the first value is false, it checks the second value. If it’s true, it returns true and if it’s false, it returns false. If the first value is true, it always returns true, no matter what the second value is. ... JavaScript is a bit different, ... JavaScript is a scripting language that enables you to create dynamically updating content, control multimedia, animate images, and pretty much everything else. (Okay, not everything, but it is amazing what you can achieve with a few lines of JavaScript code.) The three layers build on top of one another nicely. JavaScript is a text-based programming language used both on the client-side and server-side that allows you to make web pages interactive. Where HTML and CSS are languages that give structure and style to web pages, JavaScript gives web pages interactive elements that engage a user.
3 days ago - What is = in JavaScript? Equal to (=) is an assignment operator, which sets the variable on the left of the = to the value of the expression that is on its right. This operator assigns lvalue to rvalu So, a Javascript framework is a framework written in JavaScript for use in a JavaScript runtime environment. There are lots of JavaScript frameworks out there for different purposes, such as creating user interfaces, building mobile apps, and so much more. To understand what this truly means in JavaScript, let's take a look at a very similar concept in the English Language: Polysemy. Let's consider the word "run". Run is a single word which could mean many different things depending on the context. "I will run home" - means to move quickly
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Jul 20, 2021 - This chapter documents all the JavaScript language operators, expressions and keywords. ... For an alphabetical listing see the sidebar on the left. ... Basic keywords and general expressions in JavaScript. In Discuss you will find 5,000+ discussions and 48,000 answers. We know that entrepreneurs genuinely want to help one another, which is why we created a place where all entrepreneurs can ask questions about everything from common issues to highly technical and industry-specific queries.
[The] "question mark" or "conditional" operator in JavaScript is a ternary operator that has three operands. — GeeksForGeeks. It has many names: question mark, question — colon, ternary operator. While the syntax is pretty straight forward, describing the JavaScript question mark ? aloud can be difficult due to its terminology. What can in-browser JavaScript do? Modern JavaScript is a "safe" programming language. It does not provide low-level access to memory or CPU, because it was initially created for browsers which do not require it. The map () method creates a new array with the results of calling a function for every array element. The map () method calls the provided function once for each element in an array, in order. map () does not execute the function for empty elements. map () does not change the original array.
NULL in Javascript. A variable is a storage location that contains a value. A null represents nothing . Its not an actual value. It means that the storage location does not contain anything (but null itself is just a special kind of value). Using JavaScript, you can enhance a normal input box to do that. You can use JavaScript to animate elements on a page — for example to show and hide information, or highlight specific sections of a page — this can make for a more usable, richer user experience. Jan 01, 2021 - Compares two expressions and returns true if one or both evaluate to true. Returns false only if both expressions are false. The following list shows all possible combinati…
JavaScript allows multiple white spaces and line breaks in a variable declaration. Multiple variables can be defined in a single line separated by a comma. JavaScript is a loosely-typed language, so a variable can store any type value. Here, prototype comes into picture. First of all, JavaScript engine checks whether toString() method is attached to studObj? (It is possible to attach a new function to a instance in JavaScript). If it does not find there then it uses studObj's __proto__ link which points to the prototype object of Student function. If it still cannot find it ... JavaScript is a traditional language that can be used to create dynamic and interactive websites. jQuery is a JavaScript library that simplifies how you interact with the DOM.
Aug 19, 2018 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers. Jul 26, 2018 - A double negation using !! will gives us a Boolean to tell whether it's found or not found ... Bitwise not operates by reversing all the bits in the operand. ... Not the answer you're looking for? Browse other questions tagged javascript bitwise-operators or ask your own question. ... How does !!~ ... Concat () The concat () method provided by javascript helps in concatenation of two or more strings (String concat ()) or is used to merge two or more arrays. In case of arrays,this method does not change the existing arrays but instead returns a new array. let arr = [1,2,3];
How does JavaScript Work? JavaScript is a client-side scripting language and one of the most efficient, commonly used scripting languages. The term .client-side scripting language means that it runs at the client-side( or on the client machine) inside the web-browsers, ... 5/9/2018 · JavaScript is a programing language. And the thing that makes it unique is that it actually runs in your browser, as opposed to on the server. It can provide interactivity, real-time content updates, and quite a few animation options. JavaScript is an exceptionally powerful language.
Handle Javascript Events Like A Pro By Divyesh Javascript
Understand The Impact Of Javascript On Seo
Deobfuscating The Javascript Code Of A Phishing Website
What Does Javascript Do 10 Things To Learn On The Way To
Can Someone Explain This Piece Of Javascript Code Stack
What Does A Node Js Developer Do
Neptune Javascript Editor Does Not Work Blog Neptune
What Is A Function In Javascript And How Do We Use It
What Does Static Public Do Express Js Faq Codecademy
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
Custom Form Javascript Doesn T Load When Js Aggregation Is
Javascript Quiz What Does This Function Do Tutorialzine
35 Useful Coding Tools And Javascript Libraries For Web
What Is Javascript What Does It Do
Github Markknol Console Log Viewer Displays Logs And
Javascript Reference Error For Objects Declared In Another
Log Messages In The Console Chrome Developers
Javascript Running Order Question Learn Mozilla Discourse
Delay Javascript Execution Wp Rocket Knowledge Base
Intellicode For Typescript Javascript Typescript
Function Hoisting In Javascript Ilovecoding
Javascript Quiz What Does This Function Do Tutorialzine
0 Response to "23 What Does Do In Javascript"
Post a Comment