23 Stream To File Javascript
javascript does not have access to the file system. if you have a html 5 browser, javascript can access local storage (a sandbox filesystem) and read/write files. local storage is only accessible to javascript on pages hosted by site (same as a cookie which is also local storage). Writable streams → To create a stream of data for writing (say, writing a large amount of data to a file). Duplex streams → To create a stream that is both readable and writable at the same time....
Debug User Defined Functions In Azure Stream Analytics
To write to a file using streams, you need to create a new writable stream. You can then write data to the stream at intervals, all at once, or according to data availability from a server or other process, then close the stream for good once all the data packets have been written. Here is a code example of how we do this:
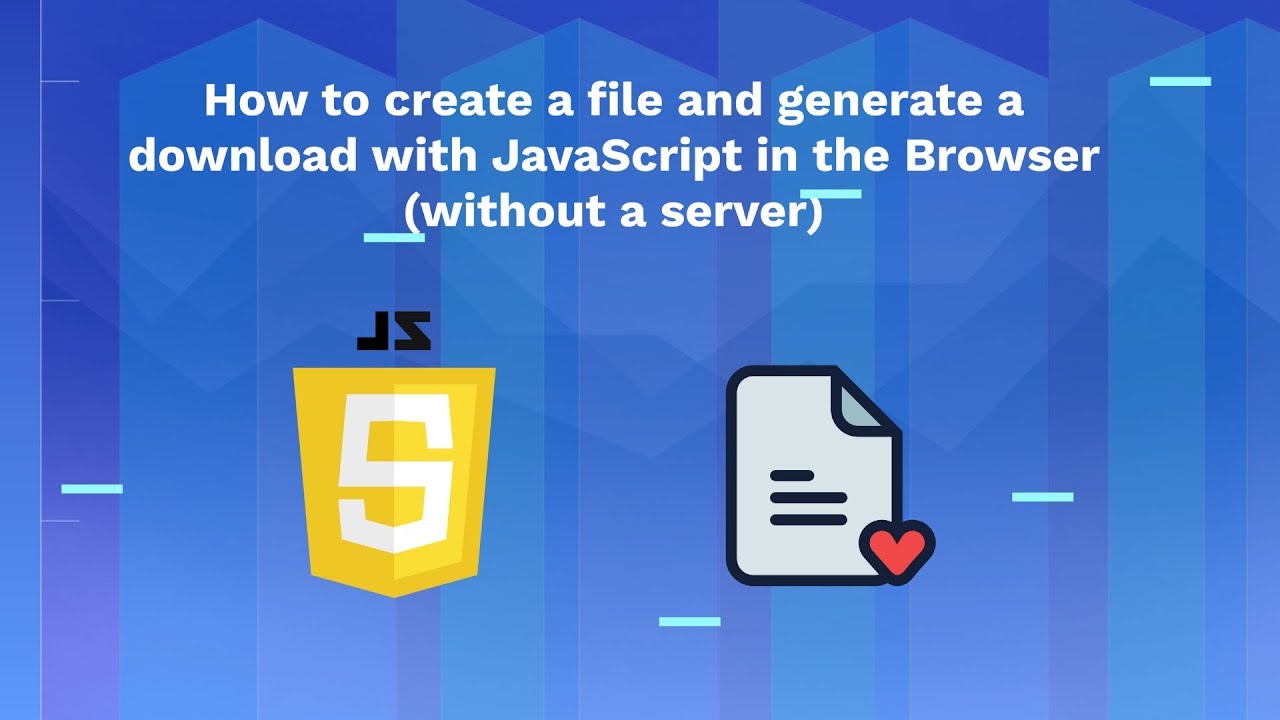
Stream to file javascript. Aug 02, 2019 - Rather than immediately displaying the file, the file is piped through a read stream into a write stream and then finally displayed in the browser. ... Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), ... Feb 09, 2021 - The stream-file package is meant to be used in web client code via a package bundler such as browserify or webpack. javascript- working with stream and large files. assumingthat we working with a server and we recived 50 request simutensly and each request size is 20mb. //data get the current "pice of the file" source.on ('data', function (chunk) { }); //end will start after the all file passed through the data event source.on ('end', function ...
Found a solution for saving the file, and it if possible in Scenario 1. In Octet-stream you will be receiving the response as "Encoded Binary Data". Your need to convert the response data into "Decoded Base64 String". Finally save it as PDF. This is the function that is responsible for actually generating the next value. In the case of the natural numbers, it would be a function that adds 1 to its argument. Now we can convert our two streams to use our new stream function: stream-usage.js link. const nats = stream(n => n + 1, 1); Note that in this example, the input stream has known and pre-determined data - such as a file on disk or an in-memory stream. Because of this, we don't need to do any bounds checking and we can - if memory allows - simply read it and write it in one go.
A File object in JavaScript references an actual file in the local filesystem. This File object inherits all properties and methods from the Blob class. Although the File objects and Blob objects are different, they expose same methods and properties. There is no way to create a File object, some JavaScript API return references File objects. The readable.pipe() method in a Readable Stream is used to attach a Writable stream to the readable stream so that it consequently switches into flowing mode and then pushes all the data that it has to the attached Writable.. Syntax: readable.pipe( destination, options ) Parameters: This method accept two parameters as mentioned above and described below: Therefore, the automatic download of file has been difficult to achieve in the latest years, but now with the introduction of HTML5, this task has become easier to achieve. In this article we are going to show you a couple of tricks to generate and download directly a file using pure Javascript. Self-implemented download function
The flags you'll likely use are. r+ open the file for reading and writing; w+ open the file for reading and writing, positioning the stream at the beginning of the file. The file is created if it does not exist; a open the file for writing, positioning the stream at the end of the file. The file is created if it does not exist; a+ open the file for reading and writing, positioning the stream ... Streaming is transmitting the data from server to the viewer's computer Concept of Buffer and Streaming in Node.js Buffer and Stream in Node.js are actually similar to the general concepts When reading a file, Node.js allocates memory as much as the size of a file and saves the file data into the memory If you want to append repeatedly to the same file, for example writing in a log file, do not use these methods. Instead, you should use the fs.createWriteStream() method that creates a writable stream and reuses the file handle to append new data.
Instead, we'll send the form to PHP through JavaScript. Create a file called upload.js. First, let's define two variables - the URL where we want to send the data, and the DOM element for the form. upload.js // Define processing URL and form element const url = 'process.php' const form = document. querySelector ('form') Upload a File in JavaScript. Sep 2, 2020 HTML has a file input tag that lets users select one or more files to upload. For example, below is HTML that defines a file input. <input type= "file" /> Given an <input type="File">, you can access the selected file as a blob by accessing input.files[0]: How to stream files to and from the computer in browser JavaScript? I am working on a web app (pure HTML/Javascript, no libraries) that does byte-level processing of a file (Huffman Encoding demo).
Nov 22, 2019 - Streams are one of the fundamental concepts that power Node.js applications. Check out this blog post to understand them! Sep 06, 2020 - There is an objectMode flag that we can set to have the stream accept any JavaScript object. Here’s a simple example to demonstrate that. The following combination of transform streams makes for a feature to map a string of comma-separated values into a JavaScript object. .text () transforms the file into a stream and then into a string..stream () returns a ReadableStream..arrayBuffer () returns an ArrayBuffer that contains the blob's data in binary form..slice () allows you to get slices of the file.
Small JS library to abstract streaming of large files via XHR w/ Range-based chunking - GitHub - brion/stream-file: Small JS library to abstract streaming of large files via XHR w/ Range-based chun... Node.js | Stream readable.read () Method. The readable.read () method is an inbuilt application programming interface of Stream module which is used to read the data out of the internal buffer. It returns data as a buffer object if no encoding is being specified or if the stream is working in object mode. Azure code sample libraries. To view the complete JavaScript sample libraries, go to: Azure Blob code samples. Azure Data Lake code samples. Azure Files code samples. Azure Queue code samples. You can browse and clone the GitHub repository for each library.
It provides sync and async functions to read and write files on the file system. Let us look at the exmaples of reading and writing files using the fs module on node.js Let us create a js file named main.js having the following code − Aug 22, 2017 - I ran the code multiple times and still got the same result. Why is this? My guess is that it has something to do with the way the createReadStream splits the file into chunks. If anyone has a better idea, then let me know! ... JavaScript developer & data visualiser. The Javascript Web API MediaDevices provides access to connected media devices such as microphones and cameras. ... (stream); — this is where we ... Encode An mp3 File Using ffmpeg.
Node.js - Streams, Streams are objects that let you read data from a source or write data to a destination in continuous fashion. In Node.js, there are four types of streams − All streams created by Node.js APIs operate exclusively on strings and Buffer (or Uint8Array) objects. It is possible, however, for stream implementations to work with other types of JavaScript values (with the exception of null, which serves a special purpose within streams). Such streams ... const stream = new ReadableStream({ start(controller) { }, pull(controller) { }, cancel() { }, type, autoAllocateChunkSize }, { highWaterMark, size() }); The constructor takes two objects as parameters. The first object is required, and creates a model in JavaScript of the underlying source the data is being read from.
Now we can start working on the upload_file () function that will do most of the heavy lifting. First we grab a chunk of the selected file using the JavaScript slice () method: function upload_file( start ) { var next_slice = start + slice_size + 1 ; var blob = file.slice ( start, next_slice ); } We'll also need to add a function within the ... public void CopyStream(Stream stream, string destPath) { using (var fileStream = new FileStream(destPath, FileMode.Create, FileAccess.Write)) { stream.CopyTo(fileStream); } } For more solutions see: How do I save a stream to a file in C#? 1 week ago - But this has never been available to JavaScript before. Previously, if we wanted to process a resource of some kind (be it a video, or a text file, etc.), we'd have to download the entire file, wait for it to be deserialized into a suitable format, then process the whole lot after it is fully received. With Streams ...
Jun 03, 2014 - Streams in Node.js are an important and versatile Tool. Unix Pipes were an example for Streams. At the beginning they were just representations of byte sequences or strings, built to improve the efficiency of the internals of Node.js, especially the parsers. But streams were always sequences ... Streams-powered Node.js APIs. Due to their advantages, many Node.js core modules provide native stream handling capabilities, most notably: process.stdin returns a stream connected to stdin; process.stdout returns a stream connected to stdout; process.stderr returns a stream connected to stderr; fs.createReadStream() creates a readable stream to a file fs.createWriteStream() creates a writable ... This continues until the end of the file when end is fired like before. Producing a readable stream. To use streams with the file system or from http, you can use the core fs and http methods to construct a stream, but how would you create your own stream and fill it with data? This might be data from a database or from any number of sources.
Node.js makes use of the 'fs' library to create readable and writable streams to files. These streams can be used to read and write data from files. Pipes can be used to connect multiple streams together. One of the most common example is to pipe the read and write stream together for the transfer of data from one file to the other. Jul 24, 2019 - As mentioned in the post on buffering, the request object passed to the request listener is a readable stream. To validate the file size, I create a FileValidator class based on the Transform class and then pipe the request stream into a new instance of the FileValidator class. To save a file with JavaScript, send the contents of the file to the server, prepare the file, and stream it back to the client with a content-disposition header of attachment; filename="file.txt". This header causes the file to be downloaded and saved the user's pc rather than displayed in the browser.
Welcome to a tutorial on how to create and save files in Javascript. Well, this will be kind of a complicated process for beginners. To keep things simple - Saving files on server-side NodeJS is a breeze, but it is impossible to directly save files on the client-side because of security restrictions. May 23, 2019 - Everything about Node.js Stream API - a great way of handling data - in a nutshell! Node.js File System data streaming. Readable & writable streams.
Event Stream Vulnerability Explained Zach Schneider
Streaming Over Http With Javascript Ajax Video Player
Headless Chrome And File Protocol Scheme Security By Anand
Understanding Streams In Node Js Nodesource
Javascript Readablestream To String Javascript Convert
Bitmovin Docs Encoding Node Js Javascript Sdk Getting Started
Github Mrhery H Js Upload Large File On Php Stream Upload
Convert File To String Javascript Code Example
How To Use Node Js Streams For Fileupload Dev Community
Ts File What It Is Amp How To Open One
Node Js Streams Everything You Need To Know
Node Js File Streams Explained
Write Byte Array To A File Javascript Stack Overflow
Understanding Streams In Node Js Nodesource
Force Javascript To Convert Stream Into File Returned From
Download Javascript Data As Files On The Client Side
How To Create A File And Generate A Download With Javascript
Chapter 7 Dealing With Huge Data Files Data Wrangling With
Node Js Streams Tutorial Filestream Pipes
What Is The Output Of A Piped File Stream Stack Overflow
Express Js App Converts Text To Speech With Cognitive
0 Response to "23 Stream To File Javascript"
Post a Comment