32 If Function Returns False Javascript
Write a JavaScript function which accepts an argument and returns the type. Go to the editor Note : There are six possible values that typeof returns: object, boolean, function, number, string, and undefined. Click me to see the solution. 10. Write a JavaScript function which returns the n rows by n columns identity matrix. Go to the editor Return true or false from the javascript function. The regex is correctly detecting a valid/invalid list of zip codes. console.log ('zip code: ' + listOfZipCodes); if (ValidateZipCodeString (listOfZipCodes)) { $tr.find ('label#lblCoverageEditError').text ('There is invalid text in the list of zip codes.
according to the JavaScript documentation ( This is a snippet from the docs ), the comparison algorithm works in the following matter: The comparison x === y, where x and y are values, produces true or false. Such a comparison is performed as follows: If Type(x) is Number, then If x is NaN, return false. If y is NaN, return false.
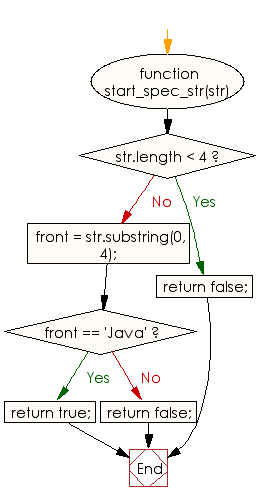
If function returns false javascript. Combine the basics of your declared functions with parameters being passed in and return statements sending values back. The first return statement immediately stops execution of our function and causes our function to return true. The code on line three: return false; is never executed. Rule #3 The return statement returns a value to the function caller. The return statement stops the execution of a function and returns a value from that function. Read our JavaScript Tutorial to learn all you need to know about functions. Start with the introduction chapter about JavaScript Functions and JavaScript Scope. For more detailed information, see our Function Section on Function Definitions ...
The strict equality operators (=== and !==) use the Strict Equality Comparison Algorithm to compare two operands.If the operands are of different types, return false.; If both operands are objects, return true only if they refer to the same object.; If both operands are null or both operands are undefined, return true.; If either operand is NaN, return false. In the code above, if checkAge (age) returns false, then showMovie won't proceed to the alert. A function with an empty return or without it returns undefined If a function does not return a value, it is the same as if it returns undefined: function doNothing() { } alert(doNothing() === undefined); also, getelementsbyname is depreciated. you should use getElementById("fileSelect") and ID your form appropriately.
A close look at the return not in function SyntaxError within JavaScript, including sample code snippets illustrating how these errors might occur. The isNaN () function determines whether a value is an illegal number (Not-a-Number). This function returns true if the value equates to NaN. Otherwise it returns false. This function is different from the Number specific Number.isNaN () method. These were some of the basic implementations of return false statement in JavaScript. However, there exist some more interesting implementations. Web Developers use 'return false' in different ways. During form submission, if a particular entry is unfilled, return false is used to prevent the submission of the form.
From what I understand you want to see if termos() returns true or false and in this case, when it returns false you want to go through a block of code. You can use falsy / truthy evaluations (http://11heavens /falsy-and-truthy-in-javascript) So you can just say: if ( termos() ) { // when its true } else { // when its false } この記事では「 【JavaScript入門】returnの使い方と戻り値・falseのまとめ! 」といった内容について、誰でも理解できるように解説します。この記事を読めば、あなたの悩みが解決するだけじゃなく、新たな気付きも発見できることでしょう。お悩みの方はぜひご一読ください。 Explanation: In general, the "return" statement is the last statement in the body of function if the function is return-type. Whenever the return statement gets encountered in the definition of the function, the execution of the function will stop, and it returns the stored value to the statement where the function call has been made.
If expr1 can be converted to true, returns expr1; else, returns expr2. If a value can be converted to true, the value is so-called truthy. If a value can be converted to false, the value is so-called falsy. Examples of expressions that can be converted to false are: null; NaN; 0; empty string ("" or '' or ``); undefined. The isFinite () function determines whether a number is a finite, legal number. This function returns false if the value is +infinity, -infinity, or NaN (Not-a-Number), otherwise it returns true. 7/8/2019 · Depending upon boolean(true or false) value If a form field (fname) is empty, then function alerts a message, and returns false, to prevent the form from being submitted. In order to avoid bubbling (which makes an event to propagate from child element to a parent element)developers have started to use return false statement to stop such propagation.
JavaScript programs may generate unexpected results if a programmer accidentally uses an assignment operator (=), instead of a comparison operator (==) in an if statement. This if statement returns false (as expected) because x is not equal to 10: The javascript code that runs within the onclick handler must return true or false (or an expression than evalues to true or false) back to the tag itself - if it returns true, then the HREF of the anchor will be followed like a normal link. If it returns false, then the HREF will be ignored. The isNaN () function to test whether the num value is not a number — if so, it returns true, and if not, it returns false. If the test returns false, the num value is a number. Therefore, a sentence is printed out inside the paragraph element that states the square, cube, and factorial values of the number.
This article is based on Free Code Camp Basic Algorithm Scripting "Check for Palindromes". A palindrome is a word, phrase, number, or other sequence of characters which reads the same backward or forward. The word "palindrome" was first coined by the English playwright Ben Jonson in the 17th century, from the Greek roots palin ("again") and dromos ("way, direction"). — src. false; 0; 0n: 0 as a BigInt '': Empty string; null; undefined; NaN; These 7 values are the only falsy values in JavaScript. Any value that is not falsy is truthy. In particular, a non-null object is always truthy, even if its valueOf() function returns a falsy value. function isFalsy (v) { return!v; } // `false`. JavaScript's forEach() function executes a function on every element in an array. However, since forEach() is a function rather than a loop, using the break statement is a syntax error: ... With every(), return false is equivalent to a break, and return true is equivalent to a continue.
The short answer is that JavaScript interpreter returns undefined when accessing a variable or object property that is not yet initialized. Return Value: The number.isInteger () method returns a boolean value ,i.e. either true or false. It will return true if the passed value is of the type Number and an integer, else it returns false. The below examples illustrates the Number.isInteger () method in JavaScript: Passing a negative number as an argument: If a negative integer value ... counts only exactly the value "false" as preventing Function2): onclick="return Function1 () && Function2 ();" It also cancels the click event if Function1 returns false or it returns a true-equivalent value and Function2 returns false.
Randomly return a true or false from a JavaScript function It's easy using a ternary expression; once you understand how this is done, many other paths to explore open up By: Ajdin Imsirovic 07 January 2021 We'll again use Math.random () for this exercise. The code inside function (index) is a separate function, nested inside imageDuplicate. The return value of that function would be processed by.each () as it sees fit. As such, the only statement which returns from your outer function is your return false. Description. When a return statement is used in a function body, the execution of the function is stopped. If specified, a given value is returned to the function caller. For example, the following function returns the square of its argument, x , where x is a number. If the value is omitted, undefined is returned instead.
For this, JavaScript has a Boolean data type. It can only take the values true or false. The Boolean () Function You can use the Boolean () function to find out if an expression (or a variable) is true:
Refactor This Redundant Await On A Non Promise Sonarlint
How To Write Cleaner If Statements In Javascript Dev Community
Javascript Fundamental Es6 Syntax Return True If The
Problem 1 Amp Amp Lt Html Amp Amp Gt Amp Amp Lt Head Amp Amp Gt Amp Amp Lt
Caution Some Answers Are Case Sensitive Lt Html Gt Chegg Com
When To Use Preventdefault Vs Return False In Javascript
Returning Boolean Values From Functions Freecodecamp Basic Javascript
Please Help Soft Rejected For Proper Event Binding Envato
Introduction To Test Driven Development Ibm Cloud
If Statement Between Two Numbers How To Calculate Step By Step
What Is The Flaw In My Thinking In This Javascript Boolean
When To Use Preventdefault Vs Return False In Javascript
Comparing Values Of Objects In Javascript Dev Community
Javascript Fundamental Es6 Syntax Return True If An Object
Why Is Php Function File Exist Doesn T Return False When
Javascript Basic Check Whether A String Starts With Java
Elseif Javascript Code Example
How To Check Nan In Javascript Weekly Webtips
How To Output A Js File Without Using Html Or A Script Tag
When And Why To Return False In Javascript Geeksforgeeks
How To Call A Function That Return Another Function In
Language Option Supported In Listings Tex Latex Stack
Javascript Function Check A Number Is Prime Or Not W3resource
Debugging Jquery With Chrome S Developer Tools
How To Use The Javascript If Else Statement
Javascript Programming With Visual Studio Code
Compare 2 Objects Js Es6 Code Example
Your Guide To Acing Javascript Interviews Better
Faq Functions Function Expressions 104 By Flodup
0 Response to "32 If Function Returns False Javascript"
Post a Comment