20 Nested Object In Javascript
Feb 21, 2018 - JavaScript is amazing, we all know that already. But a few things in JavaScript are really weird and they make us scratch our heads a lot. One of those things is the confrontation with this error when you try to access a nested object, When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ...
Using Es6 To Destructure Deeply Nested Objects In Javascript
Jan 14, 2021 - A module to filter and diff complex nested objects having circular references.
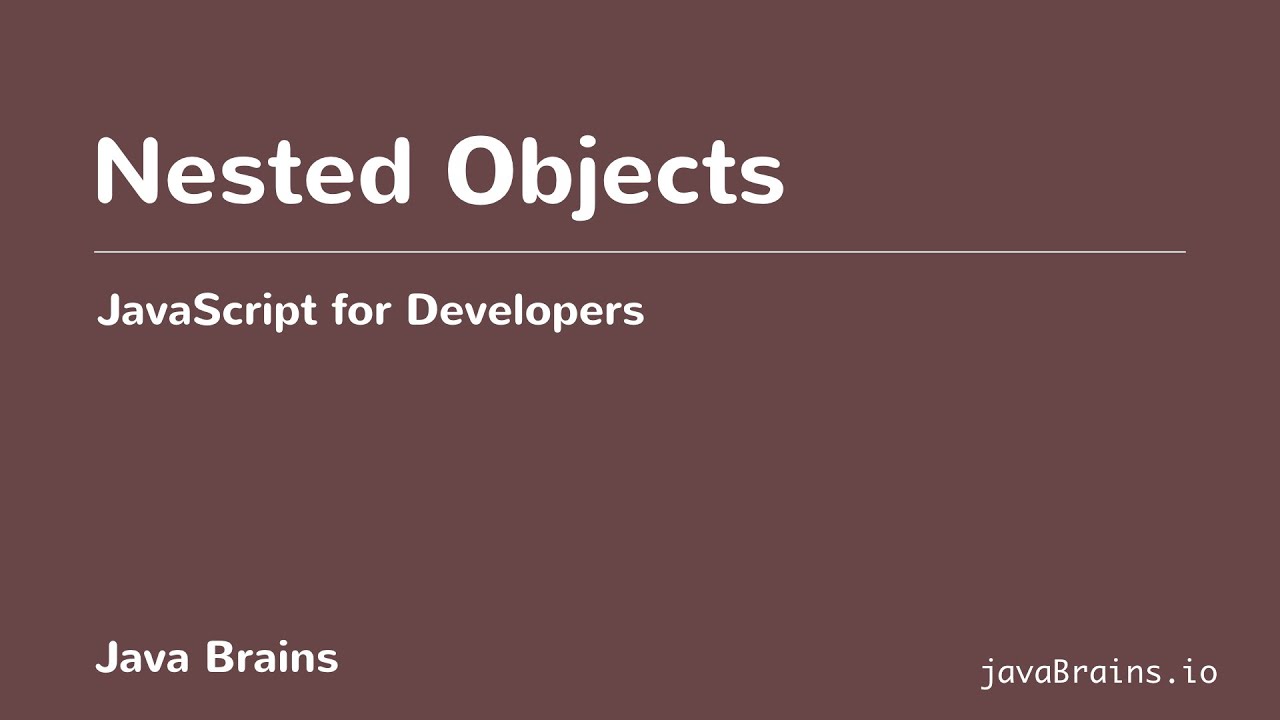
Nested object in javascript. Aug 11, 2019 - Hi FCC, I’ve been struggling with this problem for a day or two and I have been able to get close but not the full solution that I am looking for. Say I have two arrays like so, const arr1 = ['a','b']; const arr2 = ['a… Jul 20, 2021 - The flat() method creates a new array with all sub-array elements concatenated into it recursively up to the specified depth. prefix is the property name of the nested object. current is the current value of the flattened object. In the function body, we check if obj is an object and that obj isn't null. If they're both true, then we know obj is an object.
so instead {…} you just create an empty object and then assign stuff to it, and use another if statement to check if there's a next property, case in which you'd assign a recursive call object to the terms sub-object. So you kinda need a nested if statement: the outer one to check if any of the lists have any elements left and the inner ... JSON Object Literals. JSON object literals are surrounded by curly braces {}. JSON object literals contains key/value pairs. Keys and values are separated by a colon. Each key/value pair is separated by a comma. It is a common mistake to call a JSON object literal "a JSON object". JSON cannot be an object. tldr; safely access nested objects in JavaScript in a super cool way. JavaScript is amazing, we all know that already. But a few things in JavaScript are really weird and they make us scratch our heads a lot. One of those things is the confrontation with this error when you try to access a nested object,
10/11/2017 · The function nest() takes two parameters 1) el and 2) parent. The first time nest is called we pass a (first element of the array) and root to the function. Nest() creates a new object called node. Node then gets added to the parent parameter. Parent corresponds to root the first time the function is called. Then node is returned. Note: JavaScript is flexible enough that we can mix the objects and arrays together. ... What if we have criteria for finding an element that we know is in a nested data structure? Let's implement a simple find function that takes two arguments: an array (which can contain sub-arrays) and a ... Apr 13, 2018 - In the previous knowledge bit, we have demonstrated the difference in between in arrays and in an object. Now in this knowledge bit, we are going to learn the concept of the nested array object in javaScript. We will learn to create an object inside of the array, and an array inside of the object.
14/12/2014 · This works fine and returns an object from a nested "structure" by Id. I would like to know if you could suggest a better approach, possible a faster one. ... Data item prototype in a Javascript data structure where object instance functions see own data. 2. The "Nesting Objects" Lesson is part of the full, JavaScript: From Fundamentals to Functional JS course featured in this preview video. Here's what you'd learn in this lesson: Filter nested object in Javascript. Posted on June 7, 2021 | by Prashant Yadav. Posted in Interview, Javascript. Create a function in javascript which will take a nested object and a filter function as input and return the filtered object. Example
Jul 15, 2017 - Hello! So for the Javascript challenges, I am stuck in the “Accessing Nested Objects” part. The sub-properties of objects can be accessed by chaining together the dot or bracket notation. Here is a nested object: v… As you can see in the above example, the nested object for address is different across the two variables, but its nested object for country is still pointing to the same reference as in our original state variable. We could fix this by going down further, but at this point it may be easier to reach for a library to help us, such as Immer. How to safely work with nested objects in JavaScript. Posted by banerjeer2611. Why do we need to be careful when working with nested objects? If you have worked with APIs before, you have most likely work with deeply nested objects. Consider the following object.
5/11/2020 · In the above example, only “JavaScript” is the name of the course with price “1500”. Approach 2: This approach uses some () method to filter the nested objects. The some () method tests whether at least one element in the array passes the test implemented by the provided function. It returns a Boolean value. Sep 10, 2019 - If you’re working with Javascript, chances are you might have encountered a situation where you have had to access a deeply nested object. If everything goes well, you get your data without any… Nested Properties of an Object in JavaScript. So far, we have seen different ways of accessing the properties i.e.: Dot notation: objectName.property; //person.firstName. Bracket notation: objectName [“property”] //person [“firstName”] Computed property: objectName [expression] //z=”age”; person [z]; The expression needs to calculate to a property ...
This is a short post intended to show the many different ways on how to safely access deeply nested values in JavaScript. The following examples all do the same thing and while they may vary in… Accessing and Setting values in nested objects. Using the dot notation is a great way to access values in nested objects. However, when dynamically setting values in a nested object (when you don't know exactly what the name of the key is going to be), you have to use the bracket notation. Let's take a look at a quick example. May 27, 2021 - Manipulating Nested Object Values With JavaScript and Ruby ... As a developer, you often need to manipulate objects, and programming languages like Ruby and JavaScript can help you a lot in this process, but sometimes you can run into problems that are not that easy to solve.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. 27/6/2019 · Javascript Object Oriented Programming Front End Technology. Accessing nested json objects is just like accessing nested arrays. Nested objects are the objects that are inside an another object. In the following example 'vehicles' is a object which is inside a main object called 'person'. Using dot notation the nested objects' property (car) is ... Aug 31, 2020 - Knowing all the tricks in the JavaScript book is nearly impossible. I’m living proof of that. As I was recently scrolling through some JavaScript documentation to read up on some topic I stumbled…
I truelly hope this brief insight on how I handle deep nested properties in Javascript Objects (and so JSON too) will show you that factoring your code is great and taking a little bit more time ... Parsing Nested JSON Data in JavaScript. JSON objects and arrays can also be nested. A JSON object can arbitrarily contains other JSON objects, arrays, nested arrays, arrays of JSON objects, and so on. The following example will show you how to parse a nested JSON object and extract all the values in JavaScript. Object destructuring is a very cool feature of ES6. Some of us are tired of using dot notation for accessing object properties. Object destructuring made the code cleaner and more readable. But in some cases, if we dont know how to use it properly we may run into unexpected issues: First of all try to restructure the name from userObj.
Introduction to JavaScript Nested Array. Nested Array in JavaScript is defined as Array (Outer array) within another array (inner array). An Array can have one or more inner Arrays. These nested array (inner arrays) are under the scope of outer array means we can access these inner array elements based on outer array object name. Home » JavaScript » Flatten nested object/array in javascript. Search for: Search for: JavaScript September 21, 2020. Flatten nested object/array in javascript. I'm new to Javascript and I have nested objects and arrays that I would like to flatten. May 16, 2019 - There is already a question about what you are referring to (and already including most of your solutions): Access Javascript nested objects safely or Accessing nested JavaScript objects with string key. But anyway: "Unfortunately, you cannot access nested arrays with this trick."
Using Object.assign. As you can see, the location property from the update object has completely replaced the previous location object, so the country property is lost. This is because Object.assign does a shallow merge and not a deep merge. A shallow merge means it will merge properties only at the first level and not the nested level. 18/4/2015 · I would like to know the correct way to create a nested object in javascript. I want a base object called "defaultsettings". It should have 2 properties (object type): ajaxsettings and uisettings. I know that i can write something like. var defaultsettings = new Object(); var ajaxsettings = new Object(); defaultsettings.ajaxsettings = ajaxsettings.. etc. This was my nested object, written in traditional JavaScript dot notation syntax. this.props.match.params.username; // what a mouthful! no one wants to write this anymore To give a little more context around it, this object was being taken from the URL string and passed into the HTTP call from the browser's client to the server side to ...
Access the full course here: https://javabrains.io/courses/corejs_jsfordev Objects can be nested within other objects. Here's how. The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables. ... The object and array literal expressions provide an easy way to create ad hoc packages of data. const x = ... Nested object and array destructuring. Nov 22, 2020 - So we must understand them first before going in-depth anywhere else. An object can be created with figure brackets {…} with an optional list of properties. A property is a “key: value” pair, where key is a string (also called a “property name”), and value can be anything.
Jan 28, 2021 - Package to support nested merging of objects & properties, using Object.Assign 19/8/2020 · Recursively list nested object keys JavaScript. Javascript Web Development Object Oriented Programming. Let’s say, we have an object with other objects being its property value, it is nested to 2-3 levels or even more. Here is the sample object −. const people = { Ram: { fullName: 'Ram Kumar', details: { age: 31, isEmployed: true } }, Sourav: { ... dynamicly nested object from another object. trying to figure out how to dynamicly create a new nested object from this one: ... dom-events ecmascript-6 express firebase forms function google-apps-script google-chrome google-cloud-firestore google-sheets html javascript jestjs jquery json mongodb mongoose node.js object php promise python react ...
Use property paths like 'a.b.c' to get a nested value from an object. Even works when keys have dots in them (no other dot-prop library can do this!). ... Safely flatten a nested JavaScript object. How to dynamically create nested object in vanilla JavaScript. { Player1: [ {cardName: "test", balanceNum: null, ability:""} ], Player2: [ {cardName: "test", balanceNum: null, ability:""} ], . . . } At the moment I am currently using this attempt to loop through and create this dynamically. Player gets update through the loop and I want each ...
Assert Portion Of Json With Nested Objects And Arrays Help
Get The Count Of Nested Array Object S Children Property
How To Use Object Destructuring In Javascript
Json Stringify Will Recursively Call Tojson If It Exists
Use These Javascript Features To Make Your Code More
Javascript Flattening A Json Techtutorialsx
Heres How Javascript S Nested Object Destructuring Works
Js Looping And Iteration Traversing Learn Co
How To Use Recursion To Flatten A Javascript Object By
Javascript For Developers 24 Nested Objects
Javascript Fundamental Es6 Syntax Target A Given Value In
Javascript Exercise 15 Nested Objects Notesformsc
How To Set Update Nested Object With Dynamic Keys In Firebase
What Is The Correct Way To Access Nested Objects In Json
How To Loop Through Every Nested Objects In Array Javascript
Updating React Nested State Properties Dev Community
Print Json Object To Nested Html Table Semicolonworld
Javascript Lesson 26 Nested Array Object In Javascript
Visualizing Nested Objects In Kibana Kibana Discuss The
0 Response to "20 Nested Object In Javascript"
Post a Comment