20 Javascript Map Contains Key
今までMapオブジェクトをそんなに使ったことがないと気づいたので、調べてみました。 まとめます。 Mapオブジェクトとは ES2015(ES6)から導入された、キーと値の組み合わせを保持することができるオブジェクト。 以下のよう... The Map JavaScript Data Structure Discover the Map data structure introduced in ES6 to associate data with keys. Before its introduction, people generally used objects as maps, by associating some object or value to a specific key value ... Check if a map contains an item by key. Use the has() method: const hasColor = m. has ('color ...
Object Keys Function In Javascript The Complete Guide
The keys () method returns a new Array Iterator object that contains the keys for each index in the array.
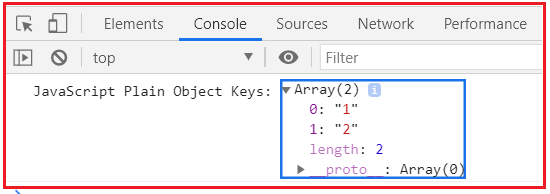
Javascript map contains key. Returns true if this map contains a mapping for the specified key. We can see that this method is a pretty good candidate for doing what we want. Let's create a very simple map and verify its contents with containsKey: @Test public void whenKeyIsPresent_thenContainsKeyReturnsTrue() { Map<String, String> map = Collections.singletonMap ( "key ... How to add JavaScript to html How ... Anonymous Functions Implementing JavaScript Stack Using Array JavaScript classList JavaScript Code Editors JavaScript let keyword Random String Generator using JavaScript JavaScript Queue Event Bubbling and Capturing in JavaScript How to select ... The key of the element to test for presence in the Map object. Return value true if an element with the specified key exists in the Map object; otherwise false .
A map is a collection of key/value pairs similar to an array, but with custom keys instead of a numerical index. Maps are part of the ES6+ specification and needs the added compiler flag -target es6 or greater. Map objects need to be instantiated with the new keyword. To add a single value to a map, we use the .set() method. The optional equals and hash override the contentEquals and contentHash properties that operate on the keys of the map to determine whether keys are equivalent and where to store them. The optional getDefault function overrides the map’s own getDefault method, which is called by get(key) ... 31/12/2018 · The java.util.Map.containsKey() method is used to check whether a particular key is being mapped into the Map or not. It takes the key element as a parameter and returns True if that element is mapped in the map. Syntax: boolean containsKey(key_element) Parameters: The method takes just one parameter key_element that refers to the key whose ...
6/7/2017 · @LoserCoder Yes, create a toString() method for your class that generates the same strings for instances with the same values, and then just use those strings as keys in an object (that way you don't even have to use Map). This is simply how Maps and object comparisons work in JS - objects are compared by reference. – sbking Jul 7 '17 at 16:01 May 31, 2020 - Given a JavaScript object, you can check if a property key exists inside its properties using the in operator. Say you have a car object: const car = { color: 'blue' } We can check if the color property exists using this statement, that results to true: 'color' in car We can use this in a ... Map.prototype.keys () The keys () method returns a new Iterator object that contains the keys for each element in the Map object in insertion order.
The map will be used in a highly concurrent context, but if you have really great ideas based around a standard HashMap please suggest. The implementation should have no external dependencies, that is use only standard Java. So far I came up with this fairly straightforward implementation. There are mainly two methods to check the existence of a key in JavaScript Object. The first one is using "in operator" and the second one is using "hasOwnProperty () method". Method 1: Using 'in' operator: The in operator returns a boolean value if the specified property is in the object. Determine whether map m contains an entry for key k · No security, no password. Other people might choose the same nickname
Make a program that filters a list of strings and returns a list with only your friends name in it.javascript · The value associated with each key will be an array consisting of all the elements that resulted in that return value when passed into the callback. Mar 27, 2020 - Hashtables are often coveted in algorithm optimization for their O(1) constant time lookup. While JavaScript doesn’t have a native Hashtable class, it does have native Objects and Hashmaps(Map) that… By definition, a Map object holds key-value pairs where values of any type can be used as either keys or values. In addition, a Map object remembers the original insertion order of the keys. To create a new Map, you use the following syntax: let map = new Map ([iterable]);
When to Use Map. Summing up, Maps are similar to Objects in that they hold key/value pairs, but Maps have several advantages over objects: Size - Maps have a size property, whereas Objects do not have a built-in way to retrieve their size.; Iteration - Maps are directly iterable, whereas Objects are not.; Flexibility - Maps can have any data type (primitive or Object) as the key to a value ... Aug 16, 2019 - The has() function of the map object accepts a key in string format and returns a boolean value of true if the specified key exists. Otherwise, the function returns false. Map is a data structure in JavaScript that allows the storage of [key, value] pairs where any value can be used as either ... If a Map contains arbitrary (JSON-compatible) data, we can convert it to JSON by encoding it as an Array of key-value pairs (2-element Arrays). Let’s examine first how to achieve that encoding. 19.2.8.1 Converting Maps to and from Arrays of pairs #
A Map 's keys can be any value (including functions, objects, or any primitive). The keys of an Object must be either a String or a Symbol . Key Order. The keys in Map are ordered in a simple, straightforward way: A Map object iterates entries, keys, and values in the order of entry insertion. Although the keys of an ordinary Object are ordered ... In JavaScript, objects are applied for storing keyed collections of various data and more complicated entities. JavaScript objects are included in all aspects of the language, so you need to learn them as soon as you start to study it. Objects are created with figure brackets {…} and should have a list of properties. JavaScript Map Object. Map in JavaScript is a collection of keyed data elements, just like an Object. But the main difference is that the Map allows keys of any type. A Map object iterates its items in insertion order and using the for…of loop, and it returns an array of [key, value] for each iteration. JavaScript Object is similar to Map ...
Definition and Usage. The includes () method returns true if an array contains a specified element, otherwise false. includes () is case sensitive. May 22, 2017 - Possible Duplicate: How do I check to see if an object has an attribute in Javascript? ... How to check whether this map has a value for a given key (for example 'X')? Does .hasOwnProperty() get into play? The hasOwnProperty () method returns true if the specified property is a direct property of the object — even if the value is null or undefined. The method returns false if the property is inherited, or has not been declared at all. Unlike the in operator, this method does not check for the specified property in the object's prototype chain.
Learn JavaScript - Checking if a key exists in a Map Although map [key] also works, e.g. we can set map [key] = 2, this is treating map as a plain JavaScript object, so it implies all corresponding limitations (only string/symbol keys and so on). So we should use map methods: set, get and so on. Map can also use objects as keys. May 06, 2021 - Learn more about How to Check If a Key Exists in a JavaScript Object? from DevelopIntelligence. Your trusted developer training partner. Get a customized quote today: (877) 629-5631.
Map is a collection of elements where each element is stored as a Key, value pair. Map object can hold both objects and primitive values as either key or value. When we iterate over the map object it returns the key,value pair in the same order as inserted. Map is a data structure in JavaScript which allows storing of [key, value] pairs where any value can be either used as a key or value. The keys and values in the map collection may be of any type and if a value is added to the map collection using a key which already exists in the collection, then the new value replaces the old value. Definition and Usage. The map() method creates a new array with the results of calling a function for every array element.. The map() method calls the provided function once for each element in an array, in order.. map() does not execute the function for empty elements. map() does not change the original array.
The above code returns the list of keys available in the Object obj.Now you need to check if the key that we are looking for exists in the keyList.You can make use of the Array.includes method to check if the key exists in the array. HashMap containsKey () Method in Java. The java.util.HashMap.containsKey () method is used to check whether a particular key is being mapped into the HashMap or not. It takes the key element as a parameter and returns True if that element is mapped in the map. Map in JavaScript Map is a collection of key-value pairs where the key can be of any type. Map remembers the original order in which the elements were added to it, which means data can be retrieved in the same order it was inserted in. In other words, Map has characteristics of both Object and Array:
How to check if a map contains a key in Go? if statements in Go can include both a condition and an initialization statement. First initialization of two variables - "value" which will receive either the value of "china" from the map and "ok" will receive a bool that will be set to true if "china" was actually present in the map. Second ... Transforming objects. Objects lack many methods that exist for arrays, e.g. map, filter and others. If we'd like to apply them, then we can use Object.entries followed by Object.fromEntries:. Use Object.entries(obj) to get an array of key/value pairs from obj.; Use array methods on that array, e.g. map, to transform these key/value pairs. Use Object.fromEntries(array) on the resulting array ... Mar 01, 2020 - How can I test whether a variable has a value in JavaScript · Check If An Array Is Empty Or Null Or Undefined In JavaScript · Use multiple conditional operators in the checkSign function to check if a number is positive, negative or zero. The function should return "positive", "negative" or "zero"
Interactive API reference for the JavaScript Map Object. Maps allow associating keys and values similar to normal Objects except Maps allow any Object to be used as a key instead of just Strings and S Mar 01, 2020 - ExpressionChangedAfterItHasBeenCheckedError: Expression has changed after it was checked. Previous value: 'ngIf: [object Object]'. Current value: 'ngIf: true' · How can I test whether a variable has a value in JavaScript · Use multiple conditional operators in the checkSign function to check ...
Javascript Fundamental Es6 Syntax Convert An Array Of
Adding Details To Markers On Google Maps Api Code Example
Javascript Map Data Structure With Examples Dot Net Tutorials
Java Hashmap Containskey Object Key And Containsvalue
Obiee 12c Simple Google Maps Report Using A Narrative Blank
Javascript Tracking Key Value Pairs Using Hashmaps By
Faster Smoother Web Maps With New Browser Features Maps
How To Check If Array Includes A Value In Javascript
Map In Javascript Geeksforgeeks
Map In Javascript Geeksforgeeks
Javascript Map Array Array Map Method Mdn
How To Use Maps Amp Sets In Javascript By Alex Ritzcovan Itnext
Hash Table Hash Map Data Structure Interview Cake
Checking If A Key Exists In A Javascript Object Stack Overflow
Javascript Map Data Structure With Examples Dot Net Tutorials
How To Use Javascript Collections Map And Set
Among Us Map Guide Skeld Mira Hq Amp Polus Maps Gamewith
Typescript Key Value Pair Internal Working And Advantages
0 Response to "20 Javascript Map Contains Key"
Post a Comment