29 Javascript Log Function Name
The anonymous function name is 'myFunctionVar', because myFunctionVar variable name is used to infer the function name. When the expression has the name specified, this is a named function expression. It has some additional properties compared to simple function expression: A named function is created, i.e. name property holds the function name 3/1/2018 · You can’t achieve this within the JS interpreter the way you have described. There is no way to get the name of a variable inside of your log function. You can only do it inside of the caller of your log function, by converting the calling function to a string and parsing the code and grabbing the variable name passed to your log function.
How To Get Currently Running Function Name Using Javascript
Finally, inside the for..of cycle, you can check which book price is 0, and log the name to console if that's the case. 4. Summary. The keys, values, and entries are 3 common lists to extract from a JavaScript object for further processing. JavaScript provides the necessary utility function to access these lists:
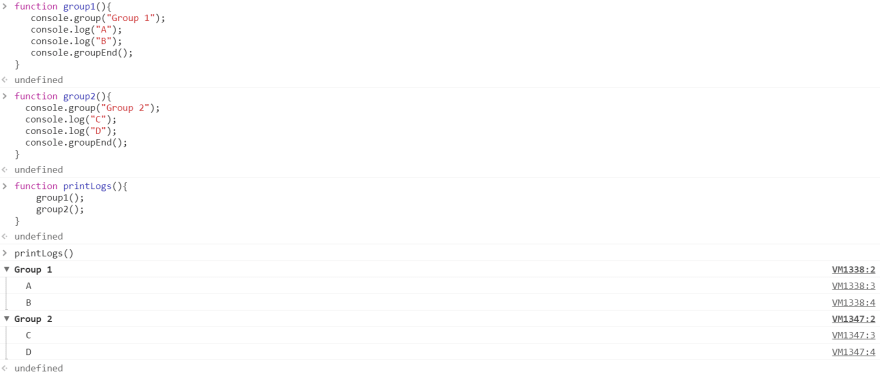
Javascript log function name. Update 2015-12-26: Sections for two caveats: "the name of a function is always assigned at creation" and "minification" The name property of a function contains its name: > function foo() {} > foo.name 'foo' This property is useful for debugging (its value shows up in stack traces) and some metaprogramming tasks (picking a function by name etc.). 26/2/2020 · Write a JavaScript function to get the function name. Sample Solution: HTML Code: <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>JavaScript function to get the function name.</title> </head> <body> </body> </html> JavaScript Code: function abc() { console.log( arguments.callee.name ); } abc(); Output: abc Proxy Method to log Function calls. There is a new way using Proxy to achieve this functionality in JS. assume that we want to have a console.log whenever a function of a specific class is called:. class TestClass { a() { this.aa = 1; } b() { this.bb = 1; } } const foo = new TestClass() foo.a() // nothing get logged
Definition and Usage. The console.log () method writes a message to the console. The console is useful for testing purposes. Tip: When testing this method, be sure to have the console view visible (press F12 to view the console). Functions are one of the fundamental building blocks in JavaScript. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output. var functionLogger = {}; functionLogger.log = true;//Set this to false to disable logging /** * Gets a function that when called will log information about itself if logging is turned on. * * @param func The function to add logging to. * @param name The name of the function.
Using Math.log () with a different base. The following function returns the logarithm of y with base x (ie. log x y. \log_x y ): function getBaseLog(x, y) { return Math.log( y) / Math.log( x); } If you run getBaseLog (10, 1000) it returns 2.9999999999999996 due to floating-point rounding, which is very close to the actual answer of 3. JavaScript can "display" data in different ways: Writing into an HTML element, using innerHTML. Writing into the HTML output using document.write (). Writing into an alert box, using window.alert (). Writing into the browser console, using console.log (). So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String, you can just type the unqualified String.The corollary, in non-strict mode, is that assignment to unqualified identifiers will, if there is no variable of the same name declared in the scope chain, assume you want to create a property with that name on the global object.
It is better to write a descriptive name for your function. For example, if a function is used to add two numbers, you could name the function add or addNumbers. The body of function is written within {}. For example, // declaring a function named greet() function greet() { console.log("Hello there"); } Object.getOwnPropertyNames() returns an array whose elements are strings corresponding to the enumerable and non-enumerable properties found directly in a given object obj.The ordering of the enumerable properties in the array is consistent with the ordering exposed by a for...in loop (or by Object.keys()) over the properties of the object.According to ES6, the integer keys of the object (both ... 16/4/2019 · Here, the Function object is replaced by the name of the function of which we want to know the parent function name. Let’s take a look at an example: <script >. // Child function. function Foo () {. // This will print 'Bar'. console.log (Foo.caller.name); } // Parent function.
The JavaScript destructuring assignment is a shorthand syntax that allows object properties to be extracted into specific variable values. It uses a pair of curly braces ( {}) with property names on the left-hand side of an assignment to extract values from objects. The number of variables can be less than the total properties of an object. Function.name. A Function object's read-only name property indicates the function's name as specified when it was created, or it may be either anonymous or '' (an empty string) for functions created anonymously. Note: In non-standard, pre-ES2015 implementations the configurable attribute was false as well. This has to go in the category of "world's ugliest hacks", but here you go. First up, printing the name of the current function (as in the other answers) seems to have limited use to me, since you kind of already know what the function is!. However, finding out the name of the calling function could be pretty useful for a trace function. This is with a regexp, but using indexOf would be about ...
console.log () The console.log () method outputs a message to the web console. The message may be a single string (with optional substitution values), or it may be any one or more JavaScript objects. Note: This feature is available in Web Workers. The console.log() is a function in JavaScript which is used to print any kind of variables defined before in it or to just print any message that needs to be displayed to the user. Syntax: console.log(A); Parameters: It accepts a parameter which can be an array, an object or any message. These new elements contain the logging messages that will assist you in debugging your script(s). The four functions used to create the log messages are: debug(msg), info(msg), warn(msg), and error(msg). Each function expects a single string that will be appended to the document. Logging levels and classes#section5
calling a function as a function. As you can see, the value of this is the Window object. That is the context in which our nifty little function is executing. You do have the option to force the context of this to be undefined in this case. To do so, you simply add the 'use strict'; directive to your code. JavaScript function basics. A JavaScript (Node.js) function is an exported function that executes when triggered (triggers are configured in function.json). The first argument passed to every function is a context object, which is used for receiving and sending binding data, logging, and communicating with the runtime. Folder structure The Throw Statement. The throw statement is used to generate user-defined exceptions. During runtime, when a throw statement is encountered, execution of the current function will stop and control will be passed to the first catch clause in the call stack. If there is no catch clause, the program will terminate. Check out this example showing how to use a throw statement:
HTML DOM provides the console object in order to use some auxiliary functions related to the browser.console.log() is one of the useful functions where it will simply print given data, integer, variable, string, JSON to the browser console. console.log() Syntax. console.log() function has very simple syntax where it accepts single or multiple parameters to print their data to the browser console. 21/6/2021 · Function naming: A name should clearly describe what the function does. When we see a function call in the code, a good name instantly gives us an understanding what it does and returns. A function is an action, so function names are usually verbal. There exist many well-known function prefixes like create…, show…, get…, check… and so on. Caller Function Name. By the way, if you only want the name of the caller function (in most browsers, but not IE) you can use: arguments.callee.caller.name But note that this name will be the one after the function keyword. I found no way (even on Google Chrome) to get more than that without getting the code of the whole function.
This tutorial on JavaScript console log will teach you different ways of outputting data, such as window.alert(), document.write(), innerHTML, console.log(), and others. Although JavaScript's primary function is modifying the behavior of the browser, these output techniques are widely used to display certain data. Advanced JavaScript Concepts to Know - Async/defer, Polyfills, Debouncing, and Throttling. Storage in JavaScript. Caveat: The focus here will largely be to cover concepts relevant to the interview and not to create a comprehensive booklet for learning the language. Treat this more like a cheatsheet. In our example, we have a login form with two input fields i.e. username and password, As user clicks on login button, JavaScript validation function comes into act. Moreover, we allowed three attempts for user to login, after third attempt all fields get disabled.
If the function f was invoked by the top level code, the value of f.caller is null, otherwise it's the function that called f.It's also null for strict, async function and generator function callers.. This property replaces the obsolete arguments.caller property of the arguments object.. The special property __caller__, which returned the activation object of the caller thus allowing to ... JavaScript Function Syntax. A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2, ...)
Javascript S Scary Side Speaker Deck
Need Help With Js Function Javascript The Freecodecamp Forum
Handy Tips On Using Console Log
Csn On Twitter In Javascript Functions Are Objects
Javascript Design Pattern Singleton Pattern Develop Paper
The 16 Javascript Debugging Tips You Probably Didn T Know
Developer Notes Javascript Abstract Factory Pattern
Calling An Aws Lambda Function From Another Lambda Function
5 Best Practices To Write Quality Arrow Functions
View A Javascript Method S Contents In Chrome Console Stack
How To Find Out The Caller Function In Javascript
First Class Function In Javascript By Javascript Jeep
All You Need To Know About Javascript Functions
Quick Guide To Searching And Filtering Javascript Logs Tektools
Javascript Console Log With Examples Geeksforgeeks
The 16 Javascript Debugging Tips You Probably Didn T Know
Log Messages In The Console Chrome Developers
Variable And Function Promotion In Javascript Variable Name
Return And Console Log Do Not Work As Expected In Codecademy
Es6 Next Generation Javascript
The 16 Javascript Debugging Tips You Probably Didn T Know
How To Create Node Js Azure Functions Azure Lessons
Beyond Console Log Dev Community
Typeerror Console Log Is Not A Function Stack Overflow
Javascript Function Is Also A Constructor Dhananjay Kumar
Javascript Tackling This Object Nested Functions Closures
Javascript Object Creation Five Common Ways
0 Response to "29 Javascript Log Function Name"
Post a Comment