32 How To Remove Last Character From String In Javascript
25/4/2020 · Now let’s see how to remove the last character from string using substr function in the below example. function removeLastCharacter() { var str = 'tracedynamics'; str = str.substr(0,str.length-1); console.log(str); } Output: tracedynamic using below JavaScript code, we can also remove whitespace character from a string. In some situations, it may be necessary to delete the last character of a string. In Javascript, there are several ways to do this: Remove the last character using the slice () function. Remove the last character using the substring () function.
3 Different Ways To Remove The Last Character Of A String In
If you wanted to remove say 5 characters from the start, you'd just write substr(5) and you would be left with IsMyString using the above example.. Remove the last character. To remove one character off of the end of a string, we can use the String.slice method. The beautiful thing about slice is it accepts negative numbers, so we don't need to calculate the length like many other ...
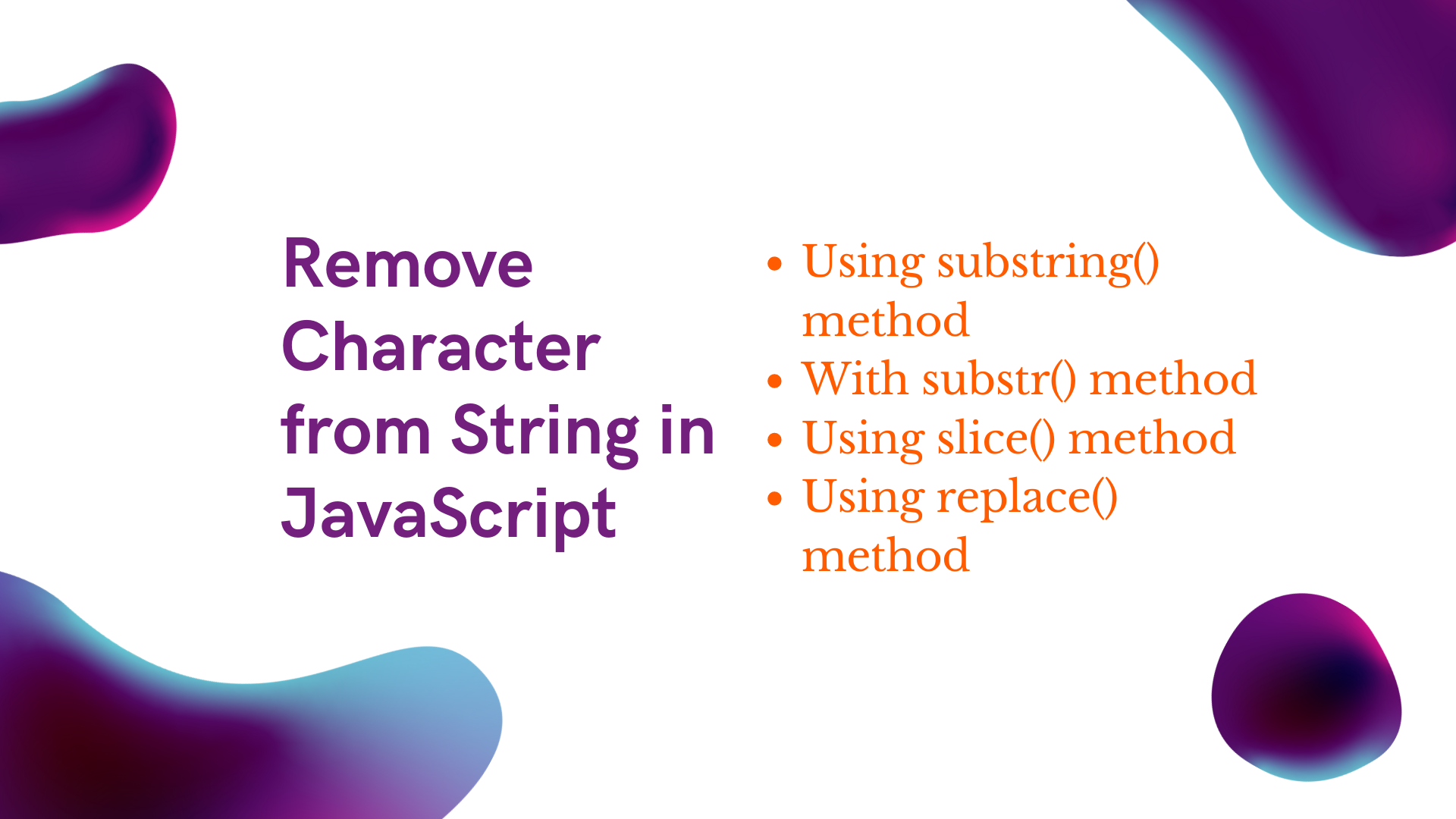
How to remove last character from string in javascript. In this tutorial, you will learn two ways to remove the last character of a JavaScript string. 1. Using String.substring () method. You can get the substring except the last character of the actual string by passing (0, string.length-1) as arguments to the substring () method. 2. Use the JavaScript substring () function to remove the last character from a string in JavaScript. with the using javascript trim string functions to string slice. This function String returns the part of the string between the start part as well as end indexes, or to the end of the string. Removing the last n characters. To remove the last n characters of a string in JavaScript, we can use the built-in slice () method by passing the 0, -n as an arguments. 0 is the start index. -n is the number of characters we need to remove from the end of a string. Here is an example, that removes the last 3 characters from the following string.
In the above code, we first remove the first character from a string using substring () method then we chain it to slice () method to remove the last character. The StringUtils class provides a chop () method to remove the last character from a string. The method parses a parameter of type String. It also accepts null, as a parameter. It returns the string after removing the last character. 15/7/2018 · Use the substring () function to remove the last character from a string in JavaScript. This function returns the part of the string between the start and end indexes, or to the end of the string. Syntax: JavaScript. str.substring (0, str.length - 1);
Javascript remove last N characters from a string using slice () Javascript's slice (startIndex, endIndex) returns a new String object part of the original string. The slice method will not modify the original string. The startIndex is the first argument that specifies from where the extraction should begin. 3 Methods to Remove Last Character from a String in JavaScript JavaScript Provides a wide range of string handling functions. There are three straightforward functions substring (), slice (), or substr () to do this task. 1. The last character could be removed by specifying the ending index to be one less than the length of the string. This extracts the string from the beginning of the string to the second to last character. Syntax: // Removing the first character string.splice(1); // Removing the last character string.splice(0, string.length - 1); Example:
Solution: I will show you several method and with their syntax to remove last character from string in JavaScript. Method 1: Using substring function How can you remove the last character from a string? The simplest solution is to use the slice() method of the string, passing 2 parameters. THe first is 0, the starting point. The second is the number of items to remove. Passing a negative number will remove starting from the end. This is the solution: const text = 'abcdef' const editedText = text.slice(0, -1) //'abcde' Note that the slice ... Use charAt: The charAt () method returns the character at the specified index in a string. You can use this method in conjunction with the length property of a string to get the last character in that string.
Answer:- Use the replace () function to remove the specific character from a string in JavaScript. The following example will show you how to remove a specific character from string javascript. 1. 2. 3. var string = 'hello javascript'; var test = string.replace (/^hello+/i, ''); The simplest way to remove the last character from a string with JavaScript is to use the slice () method. To do that, we'll need to add two parameters inside the slice () method: The starting point (0) The number of items to remove (1) Here's an example where we correct a misspelled company name: const companyName = "Netflixx" const ... Javascript remove last character from string if exists / if slash. The below section will delete the last character only if a specific character exists in the string at the end. In the example below, we check for slash ('/') and deleting it off if found in the end.
How to delete last symbol in JS string using slice#. const base = 'javascript, remove last char!' const s = base. slice ( 0, -1 ); console. log ( s ); // javascript, remove last char. The function slice returns a part of the string. We pass in two values, start and end. Eventually slice returns us a substring of base between start and end. To remove the last character, we have passed the first argument as 0 and the second as string length - 1. Removing a specific character using substr() Javascript string substring() is an inbuilt function that returns a part of the string between the start and end indexes or to the end of a string. There are several ways to do that in JavaScript using the substring (), slice (), or substr () method. 1. Using substring () method. The substring () method returns the part of the string between the specified indexes. You can use it as follows to remove the last character from a string: 1. 2.
20/8/2021 · The most common way to trim the last character is by using the JavaScript slice method. This method can take up to two indexes as parameters and get the string between these two values. To keep the whole string and remove the last character, you can set the first parameter to 0 and pass the string length - 1 as the second parameter. Use the substring () string function to remove the last character from a string in Java Script source code. This javascriptstring function simple returns the section of the string between the start and end indexes, or to the get the data string end of the string. Read Also: Vuejs Autocomplete Input Tags component 3 Methods To Remove The Last Character From A String In Javascript. As I have said there are many ways of removing a string in javascript we will see the best 3 methods we will use the following methods for string removal in javascript. Using substring() method; Using slice() method; Using substr() method
How to Remove the Last Word from the String Using JavaScript Example 1: Remove the Last Character of a String Using substring () Method In this example, we used the substring () method to remove the last character of a string. The substring () method returns the part of the string between the specified indexes. Javascript provides a wide array of string-handling functions. Removing the last character from a string is a simple task in Javascript. There are two very straightforward ways to go about this ... # Splitting the String and Popping or Slicing the Last Character We can split the string at each character to get an array of all characters in the string. If we simply pop the last character from that array of characters and then join all the characters back into a string, we get the same string but without the last character.
Use the substring () Function to Remove the Last Character From a JavaScript String We use the substring () method to return the part of a string between two indexes. If only one index is provided, it is treated as the starting index, and the function returns the substring from starting index to the last index of the string. You can, in fact, remove the last arr.length - 2 items of an array using arr.length = 2, which if the array length was 5, would remove the last 3 items. Sadly, this does not work for strings, but we can use split() to split the string, and then join() to join the string after we've made any modifications.
Ruby String Exercises Remove Last Specified Characters From
Everything You Need To Know About Regular Expressions By
Remove Last Character From String In Javascript Pakainfo
How To Remove Last Character From String In Javascript
Javascript Chop Slice Trim Off Last Character In String
How To Remove The Last Character From String In Javascript
Visual Basic String Trim Method Trimstart Trimend Tutlane
Remove Last Character From String Javascript Js Tricks
How To Remove Last Character From String Javascript Nawazify
How To Remove A Character From String In Javascript
Formula To Delete The First Or Last Word From A Cell In Excel
Remove Leading And Trailing Whitespace From Javascript String
How To Remove Character From String Using Javascript Codekila
Mysql Remove Characters From String Thispointer Com
Remove Last Character From String In Javascript
Javascript Remove Characters From Beginning Of String Code
Javascript Remove Character From String The Complete
Java Program To Print First And Last Character In A String
Remove Last Character From String In Abap
4 Ways To Remove Character From String In Javascript
Javascript Remove Character From String The Complete
Javarevisited How To Remove First And Last Character Of
Add Remove Replace String In C
Remove All Whitespace From A String In Javascript Clue Mediator
Java67 How To Get First And Last Character Of String In Java
Javascript Tutorial Remove First And Last Character
How To Remove The Last Character In A String With Javascript
Javascript Remove Last Character Design Corral
How To Remove Last Character From String Jquery
Get First Names From A Name String By Removing Last Name
How To Remove First And Last Character S From A String In
0 Response to "32 How To Remove Last Character From String In Javascript"
Post a Comment