28 Javascript String Remove All Spaces
Nov 13, 2019 - Let "A" be a string. Remove all the whitespaces and find it's length in javascript ... Removing whitespace from strings. js ... // How to create string with multiple spaces in JavaScript var a = 'something' + '\xa0\xa0\xa0\xa0\xa0\xa0\xa0' + 'something'; 26/11/2019 · The first parameter is given a regular expression with a space character (” “) along with the global property. This will select every occurrence of space in the string and it can then be removed by using an empty string in the second parameter. This will remove all the spaces in the original string. Syntax: string.replace(/ /g, "") Example:
How To Remove Whitespaces From A String In Java Selenium
Problem: Remove all whitespace from string javascript. asked Jul 9 Aurelia Maja 84.2k points. javascript. jquery. 0 votes. 1 answer 20 views. 20 views. Remove all instances of character from string javascript. Problem: Hey i want to remove all the instances of character from string javascript. Help me to get this one. asked May 7 Ryan evans 1 ...
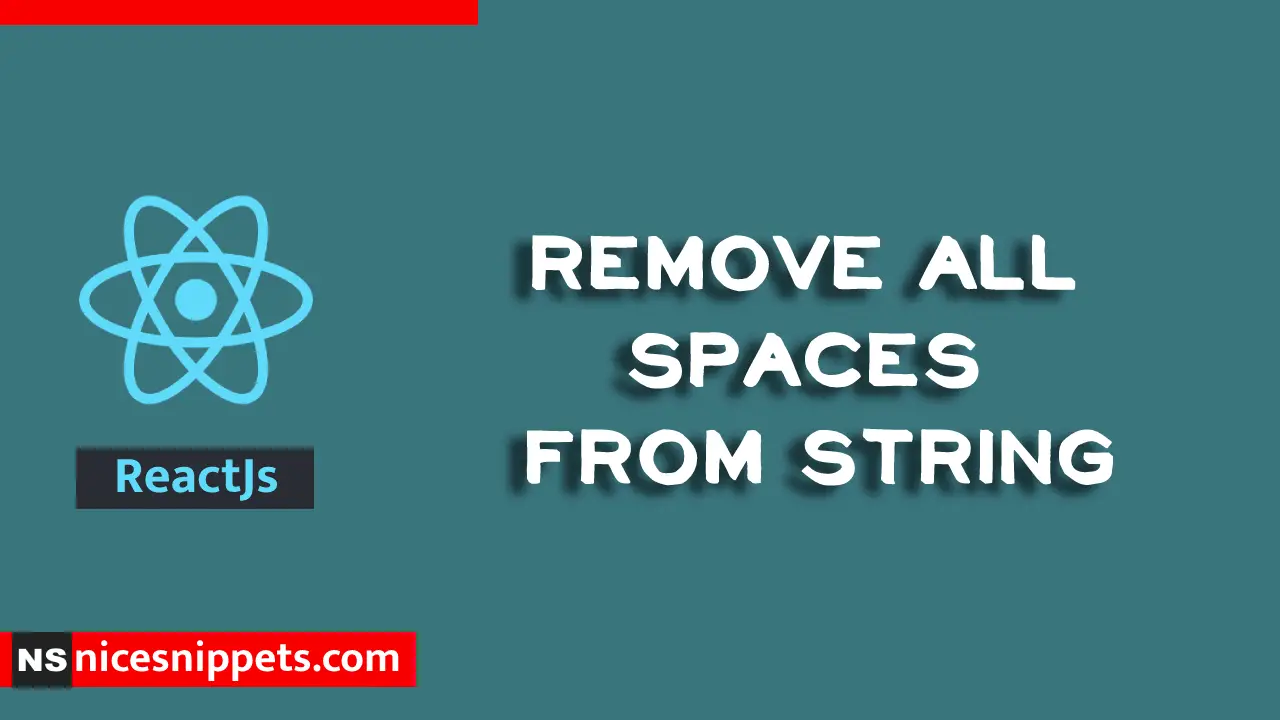
Javascript string remove all spaces. This tool saves your time and helps to remove all Spaces from text data with ease. This tool allows loading the text data URL, which loads text and remove all spaces. Click on the URL button, Enter URL and Submit. Users can also remove backspace from text data File by uploading it. Remove Whitespace Online works well on Windows, MAC, Linux ... String#trim()removes the whitespace at the start and end of the string. If the string contained only whitespace, it would be empty after trimming, and the empty string is falsey in JavaScript. If the string might be nullor undefined, we need to first check if the string itself is falsey before trimming. To remove all spaces in a string with JavaScript, you can use RegEx: const sentence = "This sentence has 6 white space characters." console.log( sentence.replace(/\s/g, "")) The example above only logs out the result, it doesn't save the changes. If you want to permanently remove the white space from your text, you have to create a new ...
We split the string into an array, using whitespaces as the breakpoint - split(" "). Then join the array back into a string (which is devoid of whitespaces now) - join(""). An interesting way to remove whitespaces, but has bad performance, and not practical at all. Not recommended. How to remove all line breaks from a string using JavaScript? Line breaks in strings vary from platform to platform, but the most common ones are the following: Windows: \r\n carriage return followed by newline character. Linux: \n just a newline character. Older Macs: \r just a carriage return character. There are several ways in which you can replace all white space using JavaScript. For all the examples we are going to look at, we have used the following string: var str = 'hey there! hello there! hi hello!'; Achieving it with replace () function in JavaScript
JavaScript trim() function is used for removing leading (before) and trailing (after) spaces from a string. This tutorial will help you to remove extra spaces from a string using JavaScript function. Remove Spaces with JavaScript trim() Function. For the example, assign a string with spaces to a variable. Then use JavaScript trim() function to ... May 02, 2020 - In this tutorial, you'll learn how to use the JavaScript trim() method to remove whitespace characters from both ends of a string. Recommended: Please try your approach on {IDE} first, before moving on to the solution. To remove all white spaces from String, use replaceAll () method of String class with two arguments, i.e. replaceAll ("\\s", ""); where \\s is a single space in unicode.
A new string representing str stripped of whitespace from both its beginning and end.. If neither the beginning or end of str has any whitespace, a new string is still returned (essentially a copy of str), with no exception being thrown.. To return a new string with whitespace trimmed from just one end, use trimStart() or trimEnd(). The $.trim() function removes all newlines, spaces (including non-breaking spaces), and tabs from the beginning and end of the supplied string. If these whitespace characters occur in the middle of the string, they are preserved. ... Remove the white spaces at the start and at the end of the string. 1 week ago - The trim() method is a built-in JavaScript method that removes whitespace from both sides of the String. Whitespace, in this context, is all of the whitespace characters, including space, tab, no-break space, and all the line terminator characters, including LF, CR, etc.
Mar 22, 2021 - Let's learn how to strip whitespace from JavaScript strings. .replace() with /\s+/g regexp is changing all groups of white-spaces characters to a single space in the whole string then we .trim() the result to remove all exceeding white-spaces before and after the text. Use a replace () and trim () method to Remove whitespace of string in JavaScript (). The trim () method do not support in browsers, you can remove whitespaces from both sides of a string or between words using replace () method with a regular expression: Methods in JS to remove string whitespace replace () - Used with regular expression.
Sep 02, 2014 - See the Pen Remove Whitespace from Strings by Chris Coyier (@chriscoyier) on CodePen. Note that none of this works with other types of whitespace, for instance   (thin space) or (non-breaking space). Mar 12, 2016 - The REPLACE() only removes the 1st space. ... The g character makes it a "global" match, meaning it repeats the search through the entire string. Read about this, and other RegEx modifiers available in JavaScript here. If you want to match all whitespace, and not just the literal space character, ... The trim method of javascript is used to remove the leading and trailing spaces in a given string. This may be useful in scenarios where visitors are given options to enter text in textboxes or text area and they enter text with leading or trailing spaces or presenting database driven string and ensuring no white spaces are shown etc.
Nov 19, 2020 - It demonstrates multiple ways to remove spaces from a string in JavaScript Jul 02, 2020 - var spacesString= "Do I have spaces?"; var noSpacesString= myString.replace(/ /g,'');// "DoIhavespaces?" ... Let "A" be a string. Remove all the whitespaces and find it's length in javascript The Regex \sis the regex for "whitespace", and gis the "global" flag, meaning match ALL \s(whitespaces). A great explanation for +can be found here. As a side note, you could replace the content between the single quotes to anything you want, so you can replace whitespace with any other string.
GeeksForGeeks. str.trim () method is used to remove the white spaces from both the ends of the given string. Syntax: str.trim () Return value: This method returns a new string, without any of the leading or the trailing white spaces. Examples for the above method are provided below: Example 1: Let "A" be a string. Remove all the whitespaces and find it's length in javascript ... Removing whitespace from strings. js Often JavaScript is used to display string returned from back-end systems. Sometimes, string returned from backend consists of either leading or trailing whitespace and in some case contains whitespaces at both ends So, we need to remove those leading & trailing whitespaces from string returned before displaying to end user/client system
Apr 28, 2021 - This post will discuss how to remove all whitespace characters from a string in JavaScript... The solution should remove all newline characters, tab characters, space characters, or any other whitespace character from the string. 19/8/2021 · The easiest way to remove all spaces from a string in JavaScript is to make use of the String.prototype.replaceAll method to replace all space characters in a string with an empty string. The String.prototype.replaceAll method accepts either a regex or a string, as well as the string you want to replace the original string with. The \s meta character in JavaScript regular expressions matches any whitespace character: spaces, tabs, newlines and Unicode spaces. And the g flag tells JavaScript to replace it multiple times. If you miss it, it will only replace the first occurrence of the white space. Remember that the name value does not change.
Javascript is a language, This is the most popular language. The function will replace all the special characters with empty where whitespaces, comma, and dots are ignored. Nov 10, 2019 - Learn how to remove space in string.. “Removing space in string in Javascript” is published by Javascript Jeep🚙💨 in Frontend Weekly. Definition and Usage The trim () method removes whitespace from both sides of a string. The trim () method does not change the original string.
JavaScript Program to Remove All Whitespaces From a Text In this example, you will learn to write a JavaScript program that will remove all whitespaces from a text. To understand this example, you should have the knowledge of the following JavaScript programming topics: JavaScript String split () 3 weeks ago - The trim() method removes whitespace from both ends of a string. Whitespace in this context is all the whitespace characters (space, tab, no-break space, etc.) and all the line terminator characters (LF, CR, etc.). JavaScript - Remove first n characters from string; How to remove all whitespace from String in Java? Remove all duplicates from a given string in Python; Remove all duplicates from a given string in C#; Write a Regular Expression to remove all special characters from a JavaScript String? Java Program to remove all white spaces from a String ...
The following Java program removes all space characters from the given string. Please note that this program doesn't work if you want to remove all whitespaces (not just spaces!) from a string. public class RemoveSpacesExample {. public static void main (String [] args) {. String input = "hello world"; String output = removeSpaces (input); Apr 01, 2020 - Today, we’re going to look at a few different ways to remove whitespace from the start or end of a string with vanilla JavaScript. Let’s dig in. The String.trim() method You can call the trim() method on your string to remove whitespace from the beginning and end of it. 23/7/2021 · The trim() method removes whitespace from both ends of a string. javascript remove all spaces. If you want to remove all whitespace from a string and not just the literal space character, use \s instead. You can remove the whitespace in a string by using a string.replace(/\s/g, “”) regular expression.
23/1/2020 · Remove all Whitespace From a String. JavaScript’s string.replace () method supports regular expressions (Regex) to find matches within the string. There’s a dedicated Regex to match any whitespace character: \s. Combine this Regex to match all whitespace appearances in the string to ultimately remove them: In another way, we can use the split () method with the join () method to remove the whitespace from a string in JavaScript. The split () method splits the string using whitespace as a delimiter and returns them in the form of an array. The join () method joins the array of strings with empty strings. On the other hand, if you want to remove all white spaces from string i.e. white space from the beginning, end, and between words then you can use the replaceAll() method of String and pass regular expression \s to find and replace all white spaces including the tab with empty string "". This is the quickest way of removing all white space from String in Java.
Trimming Unnecessary Space From String In Sql Server Tech Funda
Learn Javascript Trim Method To Remove Spaces With 2 Examples
How To Remove All Spaces From A String In Javascript
C Program To Remove Spaces From String
How To Remove Spaces From A String Using Javascript Stack
Remove Spaces In Excel Javatpoint
T Sql To Replace Multiple Spaces With Single Space Sw Class
How To Remove Spaces With Calculate Field In Arcma Esri
Python Remove Spaces From A Given String W3resource
Remove All White Spaces From Text Example Pakainfo
How Can I Strip All Punctuation From A String In Javascript
How To Remove White Spaces From String In Java
How To Replace All Whitespaces In Javascript Dev Community
Visual Basic String Trim Method Trimstart Trimend Tutlane
How To Remove Spaces From A String In Python
Best Way To Remove Line Breaks And Blank Spaces From A String
How To Javascript Replace All Word Space Comma Special
How To Remove White Spaces From A String In Javascript Reactgo
Reactjs Remove All Spaces From String Example
Efficient Way To Trim Space Characters Off All Properties
Python Remove Extra Spaces Between Words Example Code
Remove Spaces From A String In Javascript Delft Stack
Javascript String Trim Remove Whitespace From String
How To Remove Spaces From A String In Javascript Spursclick
Remove All Whitespace From A String In Javascript Clue Mediator
How To Trim String In Javascript Samanthaming Com
Remove Spaces In Excel When Trim Doesn T Work Auditexcel Co Za
0 Response to "28 Javascript String Remove All Spaces"
Post a Comment