33 Javascript Print To Console
JavaScript JavaScript Reference ... open() print() prompt() resizeBy() ... The console.log() method writes a message to the console. The console is useful for testing purposes. Tip: When testing this method, be sure to have the console view visible (press F12 to view the console). Browser Support. Oct 13, 2017 - learning JS, first time using netbeans.My code can compile, but i don't know how to print to the console screen. I've tried the system.out.println function, but that still doesn't work. What am I d...
Snippet Console Log Return Different Value Than Browser
Hi, today I would like to show you how to print json to console using JavaScript. Note: using JSON.stringify(userObj) we convert object to JSON and print to con...
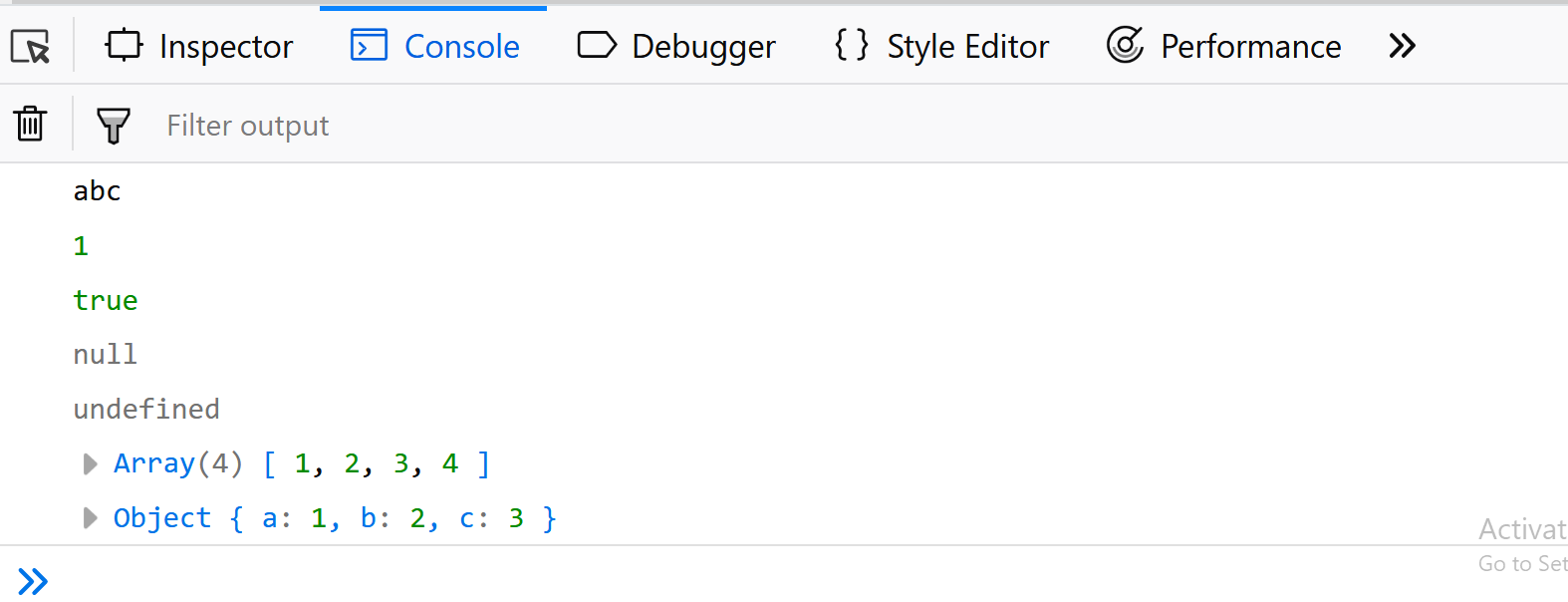
Javascript print to console. In this tutorial, you will learn the really simple function to use JavaScript to print to the console of your web browser.Don't forget to subscribe to the Ju... Color the output. You can color the output of your text in the console by using escape sequences. An escape sequence is a set of characters that identifies a color. Example: console.log('\x1b [33m%s\x1b [0m', 'hi!') You can try that in the Node.js REPL, and it will print hi! in yellow. However, this is the low-level way to do this. This method does not display anything unless used in the inspector. Stops the current JavaScript CPU profiling session if one has been started and prints the report to the Profiles panel of the inspector. See console.profile() for an example.
Printing Hello World. In order to print the famous 'Hello World' sentence to the screen, we can make use of different methods that javascript provides. Most common are: console.log () document.write () alert () Each of the above method have different ways of outputting the content. Though 'document.write ()' is used when we want to ... 13 Oct 2017 · 2 answersThat looks like you're confusing Javascript with Java. They're not the same language. NetBeans is a development environment for Java, ... 20 Jul 2021 — The console.log() is a function in JavaScript which is used to print any kind of variables defined before in it or to just print any message ...
// program to write to console // passing number console.log(8); // passing string console.log('hello'); // passing variable const x = 'hello'; console.log(x); // passing function function sayName() { return 'Hello John'; } console.log(sayName()); // passing string and a variable const name = 'John'; console.log('Hello ' + name); // passing object let obj = { name: 'John', age: 28 } console.log(obj); As always, Internet Explorer is the big elephant in rollerskates that stops you just simply using console.log().. jQuery's log can be adapted quite easily, but is a pain having to add it everywhere. One solution if you're using jQuery is to put it into your jQuery file at the end, minified first: Javascript Printing to the Developer Console Web browsers contain a tool called the developer console that displays debugging output from scripting languages like Javascript. Many programming languages, like Java, do something similar by directing output to a programming terminal.
If the JavaScript Console window is closed, you can open it while you're debugging in Visual Studio by choosing Debug > Windows > JavaScript Console. Note If the window is not available during a debugging session, make sure that the debugger type is set to Script in the Debug properties for the project. You should use the console.log () method to print to console JavaScript. The JavaScript console log function is mainly used for code debugging as it makes the JavaScript print the output to the console. To open the browser console, right-click on the page and select Inspect, and then click Console. Nov 12, 2019 - The standard way to print out a message to the JavaScript console of the web browser (or Node application) is using the log method of the console object. It accepts a list of strings and objects and prints them to the console.
28/10/2013 · The console.println()function is particularly useful for monitoring how code is working. Just place a few console.println() statements at critical locations in your script and you can literally watch the progression of code execution in real time. It is up to the developer to decide what information to display. Learn JavaScript - Formatting console output. Advanced styling. When the CSS format specifier (%c) is placed at the left side of the string, the print method will accept a second parameter with CSS rules which allow fine-grained control over the formatting of that string:console.log('%cHello world!', 'color: blue; font-size: xx-large'); I have an HTML code with JavaScript. I set the form to print to a console. My problem is that when it prints multiple selections or check boxes it still prints the comma after the last selection. Example "red, white," I would like the comma after the white not to print. Please advise on how to get rid of the last comma. Thank you in advance
Just open the console, Ctrl+Shift+K or F12, and in the top right you will see a button that says "Switch to multi-line editor mode". Alternatively, you can press Ctrl+B. This gives you a multi-line code editor right inside Firefox. 12/3/2019 · JavaScript Print to console chrome & Other browsers. Use a console.log () method to print message in the console. It is use full for developing purposes. Visible console tab in browser press F12 button. Or you can go through Right click -> inspect element -> console. There are certain situations in which you may need to generate output from your JavaScript code. For example, you might want to see the value of variable, or write a message to browser console to help you debug an issue in your running JavaScript code, and so on.
4/7/2016 · Console printing can be done in order to check or test or debug our code. Tha... In this video we are going to see how to print to the console using javascript. First, open the HTML file that included javascript like this, copy and paste below code to console.html. Then open console.html on the browser (e.g. chrome), right-click on the browser and click inspect. Click console from inspecting tab, you will see console print out of "Hello Javascript"). Jhon's answer does a great job explaining how you can place your data somewhere you can see, but I believe that's not your question. You want to change the ...
A browser's debugging console can be used in order to print simple messages. This debugging or web console can be directly opened in the browser ( F12 key in most browsers - see Remarks below for further information) and the log method of the console Javascript object can be invoked by typing the following: console.log ('My message'); 40 answersIf you want to print the object for debugging purposes, use the code: ... output to dev tools console. alert(str); // Displays output using window.alert(). Use <script> tag inside echo command to print to the console.
Print array of objects into console in javascript An array can contain multiple objects. Each object contain key and value pairs enclosed in {}. There are multiple ways we can display object array Using console.log () console.log () is used in debugging the code. Hello, World! Here, the first line is a comment. console.log ('Hello, World!'); prints the 'Hello, World!' string to the console. 2. Using alert () The alert () method displays an alert box over the current window with the specified message. Apr 06, 2021 - The useful constants that you’re more likely to use are JSON_FORCE_OBJECT and JSON_PRETTY_PRINT. ... Sometimes, you don’t want to install PHP libraries only when you’re absolutely sure you have to. So, you might prefer to console log the PHP variables in the middle of your JavaScript code ...
Sep 10, 2020 - Logging messages to the console is a very basic way to diagnose and troubleshoot minor issues in your code. But, did you know that there is more to console than just log? In this article, I'll show you how to print to the console in JS, as well as all JavaScript: How to loop through all DOM elements on a page and display result on console? Display the dropdown's (select) selected value on console in JavaScript? Get number from user input and display in console with JavaScript; How to read data from PDF file and display on console in Java? Javascript Make your console talk! ... Used to write a message to the console in javaScript.
Today, We want to share with you javascript console write.In this post we will show you how to display javascript variable value in html page?, hear for javascript print variable to console we will give you demo and example for implement.In this post, we will learn about JavaScript String Methods Functions with an example. The Console. # Overview. The Console is a REPL, which stands for Read, Evaluate, Print, and Loop. It reads the JavaScript that you type into it, evaluates your code, prints out the result of your expression, and then loops back to the first step. # Set up DevTools. This tutorial is designed so that you can open up the demo and try all the ... 18/8/2021 · to print to console using javascript A note about 'throw()' mentioned above. It seems that it stops execution of the page completely (I checked in IE8) , so it's not very useful for logging “on going processes” (like to track a certain variable…) How to print to console using javascript ? to print to console using javascript …
28/4/2017 · Well you can achieve this by doing this. Make an empty string variable and then concatenate each array value to it, then print it out. This will work. var lst = [1,2,3,4,5]; var output = ""; for(var i =0; i <= lst.length; i++){ output += lst[i] + " "; } console.log(output); 16 Jul 2021 — objN: A list of JavaScript objects to output. The string representations of each of these objects are appended together in the order listed ... Aug 15, 2018 - How to print to the command line console using Node, from the basic console.log to more complex scenarios
If you're a web developer like me, you probably use console a lot. We use it to debug JavaScript code, to print out the contents of an object etc. I use built-in Chrome tools, which is enough for most of the cases. I also use a Vue Devtools extension, when I build Vue.js apps, and I love it. Print to Console With the console.warn () Method in JavaScript If you want to print warnings to the console, then that you can use this method. There can be cases where you want the user from doing a particular thing; then, you can use the console.warn () method. For example, you create an application where tracks the user's weight. Jul 25, 2018 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers.
Javascript Make your console talk! ... This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... Material-UI: A component is changing the default value state of an uncontrolled Select after being initialized. To suppress this warning opt ... Nov 19, 2020 - Whatever method you use, it is a useful skill to master the JavaScript console in order to speed up your development process. Happy coding! 💻🏆🙌 ... JavaScript offers many ways to inspect the content of a variable. In particular, let's find out how to print the… JSON.stringify function to print out Javascript objects. Another way to print out the contents of a Javascript object is to use the JSON.stringify function. This is a very nice way to print things out because you don't have to use the console, and you don't have to write out a for/in loop just to get properties to print out.
What Went Wrong Troubleshooting Javascript Learn Web
Javascript Console Log Example How To Print To The
Output Function Console Log Javascript From Scratch
Javascript Console Log Function Tutorial With Examples Poftut
Print Variables Using Var In Javascript Stack Overflow
4 Ways To Print In Javascript Wikihow
Log Messages In The Console Chrome Developers
Print To Console In Javascript Delft Stack
Advanced Logging With Javascript Console By Muhammed
Javascript Print To Console Tutorial Different Ways To Output Data To The Console
The Console As A Javascript Environment Microsoft Edge
A Guide To Console Commands Css Tricks
The 16 Javascript Debugging Tips You Probably Didn T Know
Javascript Log To Console Console Log Vs Frameworks
How To Console Log Better In Javascript
How To Write X In Both Python 3 And Javascript Es2015
How To Prompt The User For Input In Javascript Dummies
Console In Javascript Geeksforgeeks
Javascript Array Console Log Output Behaviour Stack Overflow
Handling Common Javascript Problems Learn Web Development Mdn
Print Log To Javascript Console And Commenting Out Codes
Run Javascript In The Console Chrome Developers
How To Print A Multiplication Table In Html Css And
Javascript Print To Console Object Div Page Button
What Is Javascript Console Explained
How To Print Javascript Console With Php Geeksforgeeks
Javascript Console Log With Examples Geeksforgeeks
Using Javascript Console Objects Effectively
How To Log To Console In Php Stackify
Advanced Console Log Tips Amp Tricks By Liad Shiran Nielsen
Javascript Console Log Example How To Print To The
4 Ways To Print In Javascript Wikihow
0 Response to "33 Javascript Print To Console"
Post a Comment