30 Using Regex In Javascript
In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a String 1/8/2006 · I have blogged several times about using regular expressions (RegExp) in Javascript. I think regular expressions are absolutely wonderful. And, one of the great things about Javascript regular expressions (as opposed to reg ex in other languages) is that you can pass the individual groups matches to a function call:
Javascript Regexp G Modifier Not Working Stack Overflow
Regex or Regular expressions are patterns used for matching the character combinations in strings. Regex are objects in JavaScript. Patterns are used with RegEx exec and test methods, and the match, replace, search, and split methods of String. The test () method executes the search for a match between a regex and a specified string.
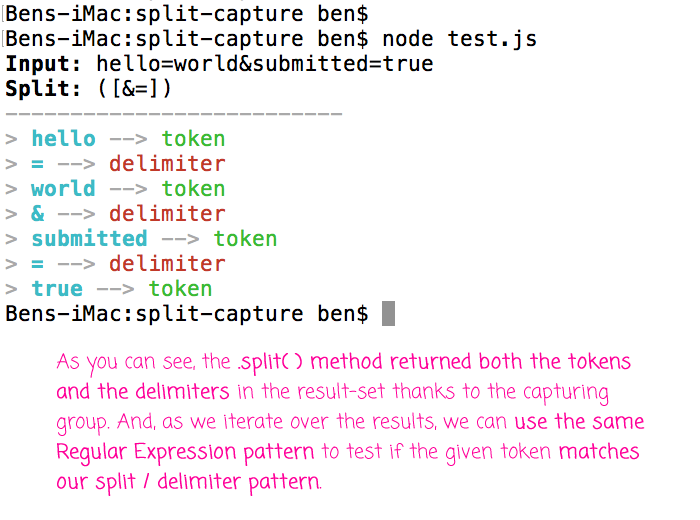
Using regex in javascript. In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a Regular Expression : Syntax. new RegExp ("\\n") or simply: /\n/. Previous JavaScript RegExp Object Next . Report Error. Forum. In JavaScript, regular expressions are represented by RegExp object, which is a native JavaScript object like String, Array, and so on. There are two ways of creating a new RegExp object — one is using the literal syntax, and the other is using the RegExp () constructor.
JavaScript regular expressions automatically use an enhanced regex engine, which provides improved performance and supports all behaviors of standard regular expressions as defined by Mozilla JavaScript. The enhanced regex engine supports using Java syntax in regular expressions. The enhanced regex 7/7/2021 · There are two ways to create a regular expression in Javascript. It can be either created with RegExp constructor, or by using forward slashes ( / ) to enclose the pattern. Regular Expression Constructor: Syntax: new RegExp(pattern[, flags]) Example: var regexConst = new RegExp('abc'); Regular Expression Literal: Syntax: /pattern/flags. Example: Using .test() returns a Boolean value. With the .test() method, you first use the regex created and chain on the .text method and then pass the argument of the string you want to test.
13/6/2021 · If the regexp has flag g, then regexp.test looks from regexp.lastIndex property and updates this property, just like regexp.exec. So we can use it to search from a given position: let regexp = /love/gi; let str = "I love JavaScript"; // start the search from position 10: regexp.lastIndex = 10; alert( regexp.test(str) ); // false (no match) Using regular expressions the entered content can be validated with advanced conditions like the string entered shouldn't contain spaces, special characters, numbers, uppercase or lowercase etc. Once you learn the art of using regular expressions you can use it with many computer languages. Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). The.replace method is used on strings in JavaScript to replace parts of string with characters.
There are two ways of defining regular expression in JavaScript. 1. Using the JavaScript RegExp object's constructor function. 2. Using Literal syntax. Creating JavaScript RegExp Object. Let's see how we can create a RegExp object in JavaScript: // Object Syntax let regExp = new RegExp("pattern", "flag"); // Literal Syntax let regExp = /pattern ... Perl makes extensive use of regular expressions with many built-in syntaxes and operators. In Perl (and JavaScript), a regex is delimited by a pair of forward slashes (default), in the form of /regex/. You can use built-in operators: m/regex/modifier or /regex/modifier: Match against the regex. m is optional. Their syntax is cryptic, and the programming interface JavaScript provides for them is clumsy. But they are a powerful tool for inspecting and processing strings. Properly understanding regular expressions will make you a more effective programmer. ... A regular expression is a type of object. It can be either constructed with the RegExp ...
A sequence of characters that forms a search pattern, mainly for use in pattern matching with strings, or string matching. RegEx is nice because you can accomplish a whole lot with very little. In this case we are going to check various aspects of a string and see if it meets our requirements, being a strong or medium strength password. As regex is used for string matching, there are three built-in methods in JavaScript we can use: search, match, matchAll() and replace. We will cover each of the methods with some examples. Important note: When specifying regular expressions, you don't need to quote it like a string. Write as /regexp/ instead of "/regexp/". Search In JavaScript, a regular expression is simply a type of object that is used to match character combinations in strings. Creating Regex in JS. There are two ways to create a regular expression: Regular Expression Literal — This method uses slashes ( / ) to enclose the Regex pattern:
JavaScript Form Validation: Removing Spaces and Dashes. Some undesired spaces and dashes from the user input can be removed by using the string object replace() method. The regular expression is used to find the characters and then replace them with empty spaces. Example: JavaScript Form Validation: Removing Spaces Supports JavaScript & PHP/PCRE RegEx. Results update in real-time as you type. Roll over a match or expression for details. Validate patterns with suites of Tests. Save & share expressions with others. Use Tools to explore your results. 1 week ago - Why isn't this built into JavaScript? There is a proposal to add such a function to RegExp, but it was rejected by TC39. ... Parentheses around any part of the regular expression pattern causes that part of the matched substring to be remembered. Once remembered, the substring can be recalled for other use...
Sep 21, 2017 - Since this tester is implemented in JavaScript, it will reflect the features and limitations of your web browser’s JavaScript implementation. If you’re looking for a general-purpose regular expression tester supporting a variety of regex flavors, grab yourself a copy of RegexBuddy. Learn how to use ... Mar 06, 2020 - JavaScript’s implementation of Regex is useful for a range of string validation, formatting and iteration techniques. This article acts as an introduction to using regular expressions in JavaScript… Jul 12, 2020 - Find centralized, trusted content ... you use most. ... Connect and share knowledge within a single location that is structured and easy to search. ... I tried the following on http://regexpal / but was unable to get it to work. What am missing? Does javascript not support ...
Nov 12, 2011 - Not the answer you're looking for? Browse other questions tagged javascript regex or ask your own question. ... How does the General Theory Of Relativity achieve the goal of showing that the laws of nature have the same form in all frames of reference? Sep 02, 2020 - After the tutorial, you’ll know how to use regular expressions effectively to search and replace strings. ... A regular expression is a string that describes a pattern e.g., email addresses and phone numbers. In JavaScript, regular expressions are objects. JavaScript provides the built-in RegExp ... RegExp - It checks for the valid characters in the Email-Id (like, numbers, alphabets, few special characters.) It is allowing every special symbol in the email-id (like, !, #, $, %, ^, &, *) symbols in the Email-Id but not allowing the second @ symbol in ID. Example: This example implements the above approach.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. In regex, the +would translate to [^/]*, and the #would translate to.*. The next step is to escape special characters in the input string. This regexhas that purpose. Since the +is a special character, we will have to unescape manually. Regular Expressions and RegExp Object. A regular expression is an object that describes a pattern of characters. The JavaScript RegExp class represents regular expressions, and both String and RegExp define methods that use regular expressions to perform powerful pattern-matching and search-and-replace functions on text.
Regular expressions, abbreviated as regex, or sometimes regexp, are one of those concepts that you probably know is really powerful and useful. But they can be daunting, especially for beginning programmers. It doesn't have to be this way. JavaScript includes several helpful methods that make using regular expressions much more manageable. RegExp The RegExp object is used for matching text with a pattern. For an introduction to regular expressions, read the Regular Expressions chapter in the JavaScript Guide. JavaScript identifies regular expressions as objects and there are methods in JavaScript such as exec() and test() using which you can test strings based on the regular expression. The exec() method; Named capture groups; The exec() method. One of the main use of regular expressions is to extract information out of the string.
Regular expressions are a useful tool for dealing with text data. They allow us to slice and dice strings, and useful extract information in our applications. Regex is also complex, and many developers will spend their entire career looking up even the most trivial of regex operations. This course will help improve your regex skills, and teach a solid core of useful tools that you can use ... In the above code, we have passed the regex pattern as an argument to the replace() method instead of that we can store the regex pattern in a variable and pass it to the replace method.. Note: Regex can be created in two ways first one is regex literal and the second one is regex constructor method (new RegExp()).If we try to pass a variable to the regex literal pattern it won't work. In this tutorial, you will learn about JavaScript regular expressions (Regex) with the help of examples. In JavaScript, a Reg ular Ex pression (RegEx) is an object that describes a sequence of characters used for defining a search pattern.
Feb 25, 2019 - It’s incredibly useful, and if you already know Javascript this tool is well within your reach. It may look like nonsensical hieroglyphs, but once you pick up a few tricks it’s actually more readable… To get them, we should search using the method str.matchAll (regexp). It was added to JavaScript language long after match, as its "new and improved version". Just like match, it looks for matches, but there are 3 differences: It returns not an array, but an iterable object. Regular expressions are used to perform pattern-matching and "search-and-replace" functions on text. ... For a tutorial about Regular Expressions, read our JavaScript RegExp Tutorial.
In JavaScript, a regular expression is an object, which can be defined in two ways. The first is by instantiating a new RegExp object using the constructor: const re1 = new RegExp('hey') The second is using the regular expression literal form: const re1 = /hey/ Jul 29, 2019 - Let’s say that, if you want to find the word "help" in the string “God helps those who help themselves”, you can use the following regular expression (RegEx): /help/. JavaScript uses the .test()… Now, to the JavaScript. All the commands are contained in one function, regEx (), triggered in the manner described above. The regEx () function in turn includes two smaller functions, testName ()...
May 14, 2021 - For example, you can check if the PIN entered by the user is all numeric or if a password entered has special characters, etc. What most developers love about RegExp is how transferable the knowledge of RegExp is. For instance, RegExp written in JavaScript can easily be relatable to RegExp ...
Form Validation In Javascript Using Regular Expression Part1
Scrapy Tutorial 11 How To Extract Data From Native
Regular Expression Validation In Javascript Skptricks
Learn Regex A Beginner S Guide Sitepoint
Introduction To The Use Of 10 Regular Expressions In
Javascript Regular Expression How To Create Amp Write Them In
A Guide To Javascript Regular Expressions
Use Regular Expression To Parse The Image Reference In The
Unicode Property Escapes In Javascript Regular Expressions
Regular Expression In Javascript
Regex In Javascript Egghead Io
Javascript Regular Expression How To Create Amp Write Them In
Javascript Regex Regular Expressions In Javascript Javascript Regular Expressions Edureka
Js Regex Get Word After Certain Characters Stack Overflow
Javascript Regex Match Example How To Use Js Replace On A
You Can Include Delimiters In The Result Of Javascript S
Regex Tutorial How To Use Regex In Javascript
How Javascript Works Regular Expressions Regexp By
Sql Regex Regular Expressions In Mysql With Examples Edureka
Javascript Regular Expression Tester Codeproject
Email Validation In Javascript Using Regular Expression With
Regex In Javascript Regular Expression Javascript Expressions
Regular Expressions In Javascript By Tran Son Hoang Level
How To Use Regex In Rpa Express Automation Cloud Community
8 Regular Expressions You Should Know
Basic Jquery Form Validator Using Regular Expressions
Everything You Need To Know About Regular Expressions By
0 Response to "30 Using Regex In Javascript"
Post a Comment