35 Javascript Map Promise All
Aug 31, 2020 - In this quick example, we'll learn how to use Promise.all() and map() with Async/Await in JavaScript to impelemt certain scenarios that can't be implemented with for loops with async/await. Example of JavaScript Promises that Rely on Each Other Jun 22, 2020 - In this tutorial, you will learn how to use the Promise.all() method to aggregate results from multiple asynchronous operations.
Why Using Reduce To Sequentially Resolve Promises Works
Aug 15, 2018 - Assuming we have already written the functions to perform the steps, “pasta making code” could be written much succinctly by using Bluebird, a javascript promise library. Bluebird’s map function allows us to make promise calls in an array and, only after all of them are resolved, it returns ...
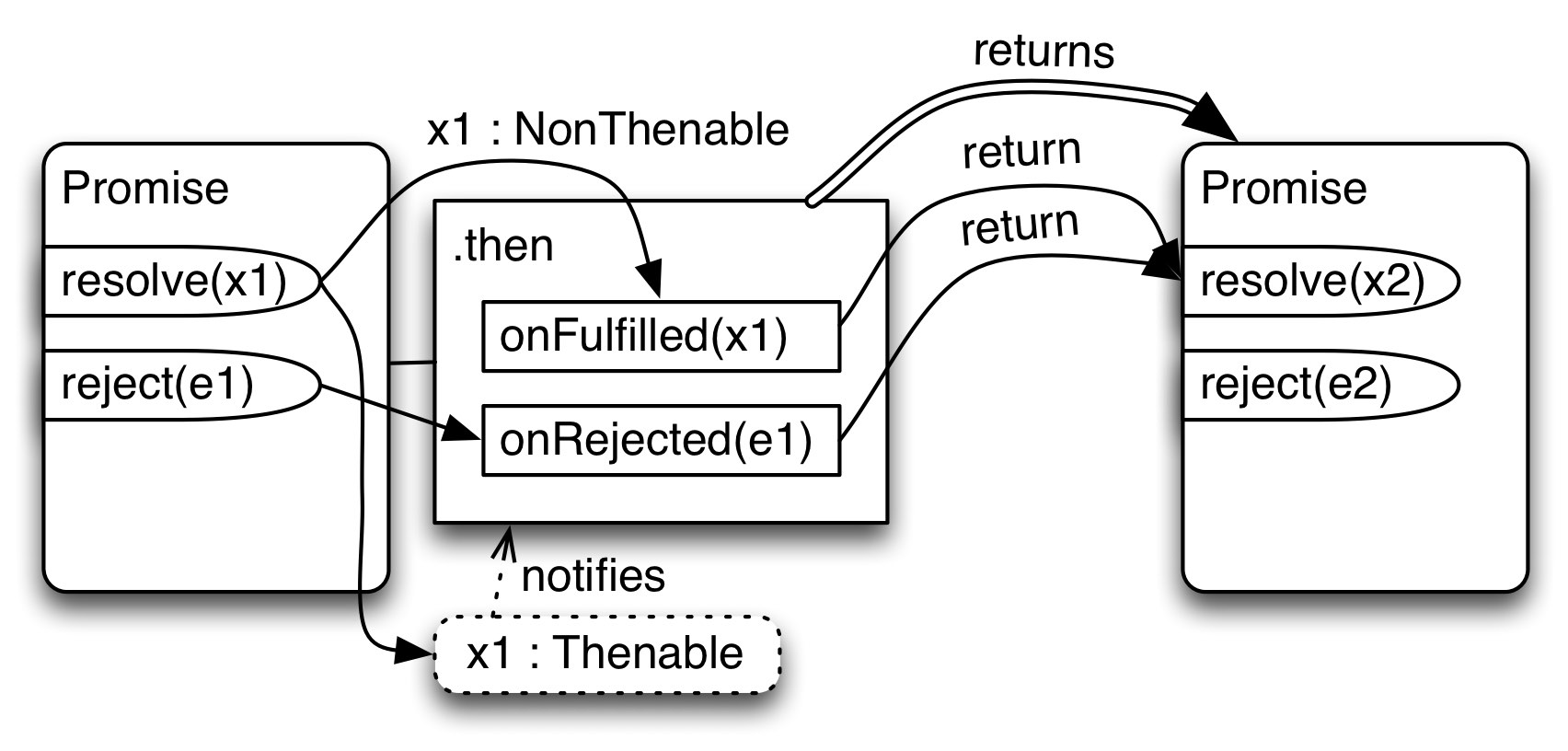
Javascript map promise all. Use Promises to Run Async Operations in Array.map. Iterating through an array in JavaScript with .map() expects a synchronous operation and a return of an (updated) value. Well, you could do a trick and return a promise. The returned promise is a value that satisfies map and ultimately represents an async operation 🤘 The Promise.all() method takes an iterable of promises as an input, and returns a single Promise that resolves to an array of the results of the input promises. This returned promise will resolve when all of the input's promises have resolved, or if the input iterable contains no promises. It rejects immediately upon any of the input promises rejecting or non-promises throwing an error, and ... Given a finite Iterable(arrays are Iterable), or a promise of an Iterable, which produces promises (or a mix of promises and values), iterate over all the values in the Iterable into an array and map the array to another using the given mapper function.. Promises returned by the mapper function are awaited for and the returned promise doesn't fulfill until all mapped promises have fulfilled as ...
As a result, you are returning an array of promises. To summarize: the map method is a synchronous operation, but we are trying to do something asynchronous here, which combined with async/await leads to an array of promises instead of values. Using Promise.all with an Asynchronous Map Method The solution here is to use Promise.all, like so: 31/8/2020 · The Promise.all() method takes an iterable of promises as an input, and returns a single Promise that resolves to an array of the results of the input promises. This returned promise will resolve when all of the input's promises have resolved, or if the input iterable contains no promises. 4/11/2019 · If you don't await for the fetch, you get a bunch of rejected promises, and errors telling you to handle your promise rejections. But recall, a Promise.all() takes an array of promises and wraps them into a single promise. So we wrap our map function. And we already know some nice syntax for dealing with a single promise. We can await it.
11/10/2019 · The Promise.all() method is actually a promise that takes an array of promises(an iterable) as an input. It returns a single Promise that resolves when all of the promises passed as an iterable, which have resolved or when the iterable contains no promises. In simple way, if any of the passed-in promises reject, the Promise.all() method asynchronously rejects the value of the promise that ... The main thing to notice is the use of Promise.all(), which resolves when all its promises are resolved.. list.map() returns a list of promises, so in result we'll get the value when everything we ran is resolved. Remember, we must wrap any code that calls await in an async function.. See the promises article for more on promises, and the async/await guide. Jun 18, 2019 - Promises in JavaScript are one of the powerful APIs that help us to do Async operations. Promise.all takes Async operations to the next new level as it helps you to aggregate a group of promises. In other words, I can say that it helps you to do concurrent operations
The Promise.all method takes asynchronous operations to a whole new level and helps us to aggregate and perform a group of promises in JavaScript. Promise.all is just a promise that receives an array of promises as an input. It gets resolved when all the promises get resolved or gets rejected if one of the promises gets rejected. p-all - Run promise-returning & async functions concurrently with optional limited concurrency. p-filter - Filter promises concurrently. p-times - Run promise-returning & async functions a specific number of times concurrently. p-props - Like Promise.all () but for Map and Object. p-map-series - Map over promises serially. Known Number of Promises. The ES6 way of doing things async way is .then() and .catch().. According to JavaScript Promise spec, every promise is thenable.. BTW, thenable just means a function which exposes a then method. If you have ever dealt with Promises, you will generally deal with .then and .catch methods.. Now, that the context is set, we can come back to our example from above.
Promise.all takes an array of promises (it technically can be any iterable, but is usually an array) and returns a new promise.. The new promise resolves when all listed promises are resolved, and the array of their results becomes its result. For instance, the Promise.all below settles after 3 seconds, and then its result is an array [1, 2, 3]: Promise.all([Promise1, Promise2, Promise3]) .then(result) => { console.log(result) }) .catch(error => console.log(`Error in promises ${error}`)) 5/4/2019 · All you need to know about Promise.all. Promises in JavaScript are one of the powerful APIs that help us to do Async operations. Promise.all takes Async operations to the next new level as it helps you to aggregate a group of promises. In other words, I can say that it helps you to do concurrent operations (sometimes for free).
17/8/2021 · 35 Javascript Map Promise All Written By Leah J Stevenson. Tuesday, August 17, 2021 Add Comment Edit. Javascript map promise all. The Javascript Promise Tutorial Adrian Mejia Blog. Javascript Async Await Explained In 10 Minutes Tutorialzine. Dealing With Multiple Promises In Javascript By Edvinas. JavaScript adds asynchronous operations to the event loop, which operates by using a queue to handle events. The event loop itself is non-blocking, and uses callback functions to execute the ... 10/5/2019 · JavaScript promises are one of the most popular ways of writing asynchronous functions that return a single value on completion or failure of the operation.. What is Promise.all()? The Promise.all() is a static method (part of Promise API) that executes many promises in parallel, and waits until all of them are settled. It takes an array of promises as an input (an iterable) and returns a ...
I can use Promise.all() ... first, then all the second, then all the third functions. const runAsyncFunctions = async => {const users = await getUsers Promise. all (users. map (async (user) => {const userId ... a software engineer, writer, and open-sourcerer. I publish articles and tutorials about modern JavaScript, design, and programming. Get ... It works like this: the .map() will convert each array item to a promise, so we'll end up with an array of promises to resolve. There are two ways of doing this: Promise.all() throws if the map function throws Promise.allSettled() runs the map function for the whole array even if sometimes it throws Therefore, the output of the .allSettled() is an array of objects that tells whether the ... With Promises we can simply map over the array of messages, return the Promise in the map function and pass the resulting array into the built-in function Promise.all(). This will return a new Promise that resolves as soon as all calls succeed, or rejects once one of them fails.
Promise Object Properties. A JavaScript Promise object can be: Pending; Fulfilled; Rejected; The Promise object supports two properties: state and result. While a Promise object is "pending" (working), the result is undefined. When a Promise object is "fulfilled", the result is a value. When a Promise object is "rejected", the result is an ... All you have to do is use the callback function as an argument to util.promisify, and store it an a variable. In my case, that's getChuckNorrisFact. Then you use that variable as a function that you can use like a promise with the .then() and the .catch() methods. Solution 2 (involved): Turn the Callback into a Promise Definition and Usage. The map() method creates a new array with the results of calling a function for every array element.. The map() method calls the provided function once for each element in an array, in order.. map() does not execute the function for empty elements. map() does not change the original array.
Running Promises in a loop sequentially, one by one. Promises are perhaps the most complicated and frustrating thing in the Javascript world (not accounting for Javascript itself). While the ... Introduction. Javascript Promises can be challenging to understand. Therefore, I would like to write down the way I understand promises. Understanding Promises. A Promise in short: "Imagine you are a kid.Your mom promises you that she'll get you a new phone next week.". You don't know if you will get that phone until next week. Your mom can really buy you a brand new phone, or she ... How to use `Array#map` within `Promise.all` in JavaScript. Ask Question Asked 4 years, 4 months ago. Active 4 years, 4 months ago. Viewed 23k times 22 1. I am trying to create a Promise.all with an array of items. So if I create it like this it works fine ... Promise.all(items.map(...)) instead of.
Nov 09, 2020 - A good handle on .map and Promises will serve you well with your JavaScript development. All of the above applies to TypeScript, flow and is the same no matter if you're in Node or the web, using react, vue or whatever. This is a vanilla JavaScript problem with a vanilla JavaScript solution. The methods promise.then(), promise.catch(), and promise.finally() are used to associate further action with a promise that becomes settled.. The .then() method takes up to two arguments; the first argument is a callback function for the resolved case of the promise, and the second argument is a callback function for the rejected case. Each .then() returns a newly generated promise object ... I want to use Map function to make a DB query (that uses promises) about all of them and attach the results of the query to each object. [obj1, obj2].map(function(obj){ db.query('obj1.id').then(function(results){ obj1.rows = results return obj1 }) }) Of course this doesn't work and the output array is [undefined, undefined]
Feb 17, 2018 - Promise.all + map. Contribute to asfktz/promise-all-map development by creating an account on GitHub. To run the JavaScript promises in parallel, you can make use of async/await. Let's have a look at how asyc/await executes the promises in parallel. As seen in the above code, instead of awaiting for the getPhotos promise to resolve, you straight away called the getPosts method. Now we await the promises running in parallel to finish. Controlled concurrency map map e1 e1 e2 e2 e3 e3 e4 e4. I couldn't come up with a simple implementation for this, but fortunately, the Bluebird library provides one out of the box. This makes it straightforward, just import the library and use the Promise.map function that has support for the concurrency option.
The Promise.all() is useful when you want to aggregate the results from multiple asynchronous operations. JavaScript Promise.all() examples. Let's take some examples to understand how the Promise.all() works. 1) Resolved promises example. The following promises resolve to 10, 20, and 30 after 1, 2, and 3 seconds. The order map calls the mapper function on the array elements is not specified, there is no guarantee on the order in which it'll execute the maper on the elements. For order guarantee in sequential execution - see Promise.mapSeries. Please enable JavaScript to view the comments powered by Disqus.
How To Use Async Await To Write Better Javascript Code
Menu Documentation Features Pricing Support Search Search
Dealing With Multiple Promises In Javascript By Edvinas
Javascript Async And Await In Loops Zell Liew
Promise All La Gi Promise All Javascript Giup Toi Xá» Ly
Promise All And Map With Async Await By Example
Swaggerhub For Vs Code How A Developer S Need Became A Cool
Promise All Function In Javascript The Complete Guide
Deeply Understanding Javascript Async And Await With Examples
Add A Map To Your Website Javascript
Javascript Promise And Promise Chaining
Go Js On Twitter Async Predicate Logic In Parallel Yay
Composing The Results Of Nested Asynchronous Calls In
Dealing With Multiple Promises In Javascript By Edvinas
Deeply Understanding Javascript Async And Await With Examples
Promise Chaining Is Dead Long Live Async Await Logrocket Blog
25 Promises For Asynchronous Programming
Async Javascript History Patterns And Gotchas Speaker Deck
Implement Your Own Bluebird Style Promise Map Better
Using Async Await With A Foreach Loop Stack Overflow
Performance Tuning Of Node Js Application With Parallel
Intro To Asynchronous Javascript
Await Array Prototype Map With Es6 By Remy Villulles
How To Run A Rest Api Query For Each Item In An Array And
Using Geofences For Location Based Notifications And Events
Promise Chaining In Javascript
Promise Async Await Va Map Reduce Huy S Blog
How To Use Async Functions With Array Map In Javascript
Introduction To Javascript Iterables Iterators And
How To Learn Javascript Promises And Async Await In 20 Minutes
Use Promise All To Stop Async Await From Blocking Execution In Js
Javascript Notes Leesei S Notebook
0 Response to "35 Javascript Map Promise All"
Post a Comment