31 Try Catch Javascript Node
Node.js is asynchronous unless the programmer goes out of their way to make the code synchronous. In most of the Node core APIs, try/catch cannot be used to properly handle exceptions. This is because errors arising in an asynchronous operation in JavaScript may not be scoped within the try/catch block where the operation was begun. Aug 19, 2018 - The variation on async/await without try-catch blocks in Javascript. Consider:
Perfomatix Nodejs Coding Standards
JavaScript's try-catch hid my bugs! Zubin Pratap ... So I put a few try-catch handlers inside the various methods being called, ... ('This function is not contained inside a try-catch, so will crash the node program.') } callAll() You'll note that the console.log statement never runs here because the program crashes when routeHandler() finishes ...
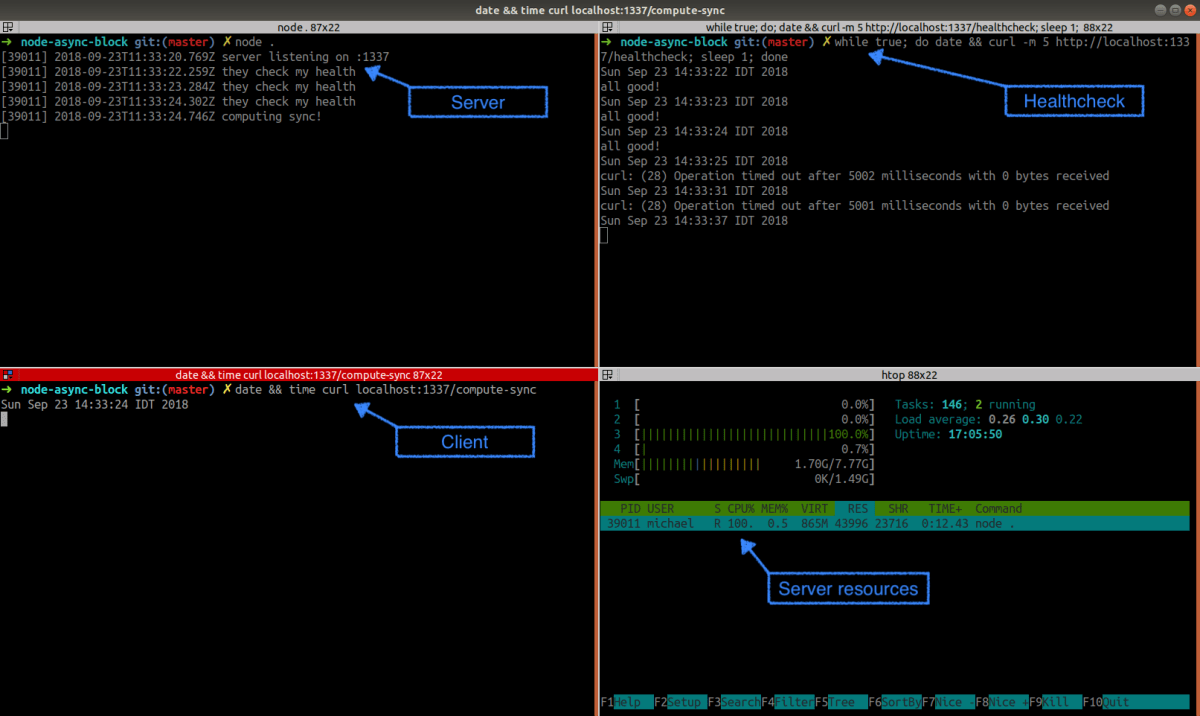
Try catch javascript node. Ideas, insights, and perspectives from inside the world of enterprise software development. Blog posts on design, software development, business and technology. Nov 15, 2011 - As such, it is best to either use domains or to return errors to avoid (1) uncaught exceptions in asynchronous code (2) the try catch catching execution that you don't want it to. In languages that allow for proper threading instead of JavaScript's asynchronous event-machine style, this is ... Node.JS and MongoDB. ... try...catch block is for handling exceptions, remember exception means the thrown error not the error.
An exception handler is a try / catch statement. Any exception raised in the lines of code included in the try block is handled in the corresponding catch block: try { } catch (e) {} e in this example is the exception value. You can add multiple handlers, that can catch different kinds of errors. The throw statement throws a user-defined exception. Execution of the current function will stop (the statements after throw won't be executed), and control will be passed to the first catch block in the call stack. If no catch block exists among caller functions, the program will terminate. 2 weeks ago - Unfortunately, neither JavaScript nor TypeScript support this. That's what this module adds. ... To use a simple try / catch, use the tryCatch function and pass a potentially failing function as well as a function that acts as a catch clause. In that function, you may return a value that is ...
Any use of the JavaScript throw mechanism will raise an exception that must be handled using try…catch or the Node.js process will exit immediately. Use of synchronous functions that report operational errors (row 2) is very rare in Node.js except for user input validation. However, with the release of Node.js version 8 people are starting to promisify these asynchronous functions and using await inside of a try/catch. Programmer errors (row 3) should never happen except in development. And we can use a regular try..catch instead of .catch. That's usually (but not always) more convenient. That's usually (but not always) more convenient. But at the top level of the code, when we're outside any async function, we're syntactically unable to use await , so it's a normal practice to add .then/catch to handle the final ...
One of the best features of async/await syntax is that standard try-catch coding style is possible, just like you were writing synchronous code. const myFunc = async (req, res) => { try { const result = await somePromise(); } catch (err) { // handle errors here } }); So if any exceptions are thrown down that call stack, the catch block will trigger. Since both the try block and the catch block invoke the callback, any exceptions in callback will result in the callback being invoked twice. Here is how I would change that third-party code. In JavaScript, we use the try...catch statement to capture unexpected errors and exceptions. How does try...catch work? try ... Node.js, Spring Boot, core Java, RESTful APIs, and all things web development. The newsletter is sent every week and includes early access to clear, concise, and easy-to-follow tutorials, and other stuff I think you'd ...
I'm just happy that this approach works in JavaScript and Node.js as well as other programming languages, like ColdFusion. Tweet This Provocative thoughts by @BenNadel - Rethrowing Errors In JavaScript And Node.js Woot woot — you rock the party that rocks the body! The try/catch/finally statement handles some or all of the errors that may occur in a block of code, while still running code. Errors can be coding errors made by the programmer, errors due to wrong input, and other unforeseeable things. The try statement allows you to define a block of code to be tested for errors while it is being executed. Try catches are usually used within functions but don't have to be. With Node.js you can import/require modules so some scripts (modules) may be short and not even have functions. I think you're over complicating what they are asking. Just a basic try catch as is. Below is the answer if you are unsure.
Developers working with Node.js sometimes find themselves writing not-so-clean code while handling all sorts of errors. This article will introduce you to error-handling in Node.js and demonstrate some of the best techniques for the job. "Try / Catch" statements are everywhere… and sometimes they are even nested or chained. This leads to such example: async function anyFunction() ... Executing and Saving JavaScript in a web browser / Node environment. Bankole Samuel. leaft: Day 4-6, Sept 17th, 18th, + 21st. Home › javascript node js try catch › try catch javascript node › try catch node js express. 34 Try Catch Javascript Node Written By Leah J Stevenson. Sunday, August 29, 2021 Add Comment Edit. Try catch javascript node. The Catch Method Javascript Promises Mastering The.
Javascript generates an object containing the details about it, which is what the (error) is above; this object is then passed (or "thrown") as an argument to catch. For all built-in or generic... Sep 07, 2018 - In Node.js, we don’t throw strings, we just throw Error objects. ... An error object is an object that is either an instance of the Error object, or extends the Error class, provided in the Error core module: ... An exception handler is a try/catch statement. Mar 30, 2021 - This daisy chain is called the call stack (don’t worry—we’ll get to the call stack soon). The nearest catch that JavaScript finds is where the thrown exception will emerge. If no try/catch is found, the exception throws, and the Node.js process will exit, causing the server to restart.
Like any kind of apps, there are difficult issues to solve when we write Node apps. In this article, we'll look at some solutions to common problems when writing Node apps. Getting Line Number in... An alternative way to use async/await without try/catch blocks in Node JS. ... To get something like the code above, our starting point is to remind some concepts in JavaScript: async methods and its try/catch blocks work in the same way as then/catch from promises pattern. It's just a sugar syntax. Jan 06, 2016 - A domain-based asynchronous try/catch ... for node.js optimized for V8. WARNING: trycatch replaces the built-in global Error object. ... Visit http://localhost:8000 and get your 500. ... See the /test and examples directories for more use cases. ... trycatch effectively wraps all application callbacks in try/catch blocks, preventing ...
First, the code in try {...} is executed. If there were no errors, then catch (err) is ignored: the execution reaches the end of try and goes on, skipping catch. If an error occurs, then the try execution is stopped, and control flows to the beginning of catch (err). 하지만 try..catch에서 처리하지 못한 에러를 잡는 것은 아주 중요하기 때문에, 자바스크립트 호스트 환경 대다수는 자체적으로 에러 처리 기능을 제공합니다. Node.js의 process.on("uncaughtException")이 그 예입니다. Node.js try catch - Error Handling | Node.js Tutorial
async & await in Javascript is awesome; it helps remove many issues with callback nesting and various other problems when working with promises. It's not without its drawbacks, though. One issue I find using async and await it can get quite messy with many try/catch blocks in your code. Because of block scoping, code often ends up looking ... As node.js has the same architecture as Javascript, in fact implementing Javascript I don't see any drawbacks to use try catch. – Endre Simo Feb 16 '16 at 9:20 Node.js Try Catch is an Error Handling mechanism. When a piece of code is expected to throw an error and is surrounded with try, any exceptions thrown in the piece of code could be addressed in catch block. If the error is not handled in any way, the program terminates abruptly, which is not a good thing to happen.
JavaScript try and catch The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block. The JavaScript statements try and catch come in pairs: 5/7/2015 · There isn't a JavaScript language construct to force you to catch all async errors. Node has some stuff to help you catch errors - you can read about it here. Here's an example: process.on('uncaughtException', function(err) { console.log('Caught exception: ' + err); }); throw 'An error'; This will catch the error thrown. Apr 04, 2020 - This tutorial shows you how to handle exceptions using JavaScript try catch statement. It also introduces you the finally clause for the cleanup code.
Aug 26, 2011 - docs.nodejitsu is a growing collection of how-to articles for node.js, written by the community and curated by Nodejitsu and friends. These articles range from basic to advanced, and provide relevant code samples and insights into the design and philosophy of node itself. A catch -block contains statements that specify what to do if an exception is thrown in the try -block. If any statement within the try -block (or in a function called from within the try -block) throws an exception, control is immediately shifted to the catch -block. If no exception is thrown in the try -block, the catch -block is skipped. 26/8/2011 · JavaScript's try-catch-finally statement works very similarly to the try-catch-finally encountered in C++ and Java. First, the try block is executed until and unless the code in it throws an exception (whether it is an explicit throw statement, the code has an uncaught native exception, or if the code calls a function that uses throw ).
Async/await without try/catch in JavaScript. When async/await was announced it became a game-changer in JavaScript development. It allows writing code in a synchronous way and we don't need to have chained promise handlers: That how this code can be refactored with the async/await syntax: Now it is easier to follow the code.
Sending Emails In Node Js Write Javascript
Rethrowing Errors In Javascript And Node Js
Introduction To Error Handling In Angular 7 Part 1 Angular
How To Rewrite A Callback Function In Promise Form And Async
A Guide To Proper Error Handling In Javascript Sitepoint
Robust Error Handling In Node Js Speaker Deck
Node Js Event Loop How Even Quick Node Js Async Functions
How To Learn Javascript Promises And Async Await In 20 Minutes
Iterating Over Array In A Try Catch Finally Results In
Fixing Unhandledpromiserejectionwarning In Node Js
Javascript Async Await Serial Parallel And Complex Flow
Javascript Errors Throw And Try To Catch Geeksforgeeks
Node Js Rxjs Engineering Education Enged Program Section
Cannot Install Truffle Node Js And Npm Are Installed
Javascript Try Catch Amp Error Handling Es6 Tutorial 2019
Best Practices For Node Js Error Handling Toptal
Async Await Without Try Catch In Javascript By Dzmitry
Hate Try Catch Error Handling In Node Js Do This Instead
Github Crabdude Trycatch An Asynchronous Domain Based
Catch Node Does It Really Help General Node Red Forum
What Exceptions Cannot Be Caught By Try Catch By Bytefish
Get Ready A New V8 Is Coming Node Js Performance Is
Node Js Error Handling Best Practices Ship With Confidence
Try Catch Finally Throws User Defined Exception In Vb Net
Can I Catch Warnings In Try Catch Block In Javascript Code
A Mostly Complete Guide To Error Handling In Javascript
Try Catch Finally Throws User Defined Exception In Vb Net
Javascript Error And Exceptional Handling With Examples
0 Response to "31 Try Catch Javascript Node"
Post a Comment