27 Javascript Form Onsubmit Example
13/12/2012 · I believe the above example is similar to what you need. We use document.getElementById to grab a reference to your form. Then set the onsubmit property to the code you want executed before the form is submitted. Remember to put this inside the window onload event if this JavaScript is executed before the page is rendered to ensure the form is built. onFocus: thisevent is raised when a select, text or textarea items is selected on an HTML form. onSelect: this event, as its name implies, is raised when some tex in a text box or text area is selected. onSubmit: this is an important event that is raised when the submit button on an HTML form is clicked to submit the form data to the web server.
Submit And Validate Html Form Using Javascript
XMLHttpRequest is the safest and most reliable way to make HTTP requests. To send form data with XMLHttpRequest, prepare the data by URL-encoding it, and obey the specifics of form data requests. Let's look at an example: And now the JavaScript: const btn = document.querySelector('button'); function sendData( data ) { console.log( 'Sending data ...
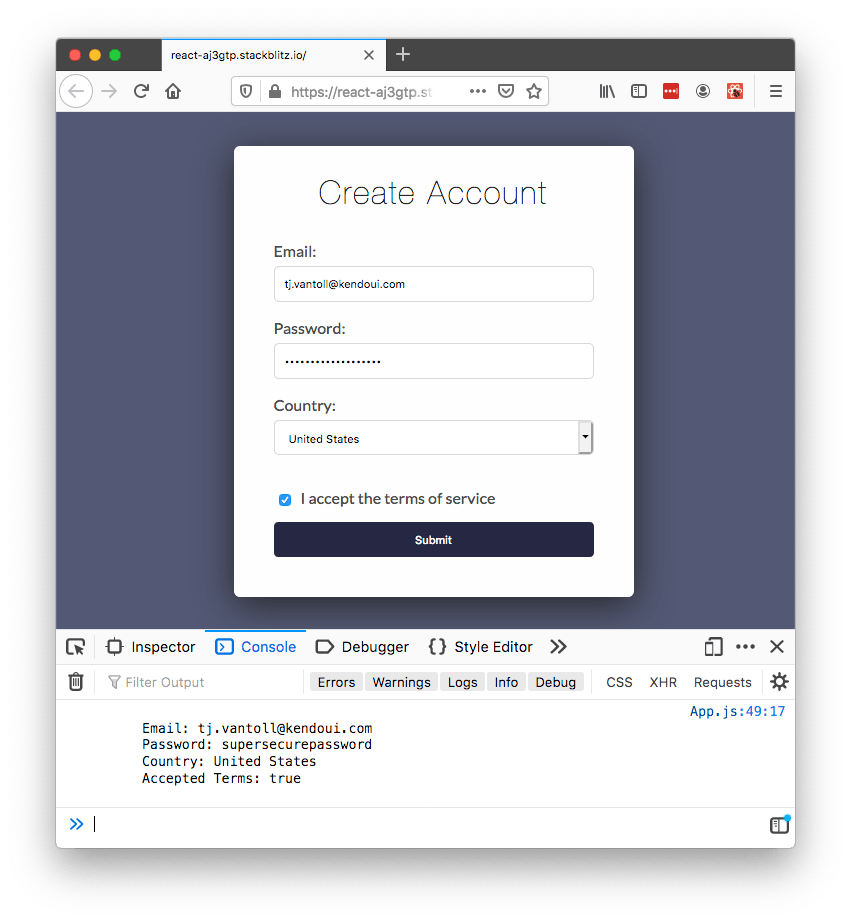
Javascript form onsubmit example. 31/7/2014 · When a user clicks on submit button of a form, JavaScript onsubmit event will call a function. Invoking JavaScript function on form submission: <form action="#" method="post" onsubmit="return ValidationEvent ()"> In our example, we call ValidationEvent () function on form submission. 15/11/2019 · You can see in JavaScript form submit example, as you entered an email with the incorrect format (without @ or . or improper letters) and press the button, an alert will be shown by JS function. Similarly, you can validate other form fields like select dropdowns, radio buttons, check boxes and any other required task by using onsubmit JavaScript event. JavaScript Form Validation Example. In this example, we are going to validate the name and password. The name can't be empty and password can't be less than 6 characters long. Here, we are validating the form on form submit. The user will not be forwarded to the next page until given values are correct.
If I do this I can prevent default on form submit just fine: document.getElementById('my-form').onsubmit(function(e) { e.preventDefault(); // do something }); But since I am organizing my code in a modular way I am handling events like this: How to Validate a Form with JavaScript. To validate a form with javascript, you will have to configure the following two simple steps - 1. Create an HTML Form. You will learn to validate form based on id of its input fields. So, I have created an HTML form with the following input fields that have its own type & id attribute. The onsubmit event in HTML DOM occurs after submission of a form. The form tag supports this event. Supported HTML Tags: <form> Syntax: In HTML: <element onsubmit="Script"> In JavaScript: object.onsubmit = function(){myScript}; In JavaScript, using the addEventListener() method: object.addEventListener("submit", myScript); Example: Using JavaScript
Step 1. Type GMAIL in text box −. After clicking the search button, the output is as follows −. Step 2. On typing another value, except GMAIL, you will get an alert message. Let's first type the value and press enter −. After clicking the search button, you will get an alert message as in the below output −. AmitDiwan. For example,if the ID of your form is 'form_id', the JavaScript code for the submit call is document.getElementById ("form_id").submit ();// Form submission onclick form submit by class For example,if the class of your form is 'form_class', the JavaScript code for the submit call is The purpose of a form validation script is to return a boolean value ( true or false) to the onsubmit event handler. A value of true means that form will be submitted while a false value will block the form from being submitting. The focus () command is used to set the focus to the problem element.
Types of Form Validation in Javascript. As stated earlier, form validation can be done in two ways. Lets have a look at both of them. onchange() Validation. onchange() validation is useful when you want to validate each of the input fields as soon as a user enters some value into it. Both examples above are great examples of onchange vaidation ... JavaScript provides the form object that contains the submit() method. Use the 'id' of the form to get the form object. For example, if the name of your form is 'myform', the JavaScript code for the submit call is: //form.html <!DOCTYPE html> Javascript Onsubmit Event Example Javascript Onsubmit Event Example Name : Email : Gender : Male Female Contact No. : All type of validation will execute on OnSubmit Event. //js/onsubmit_event.js // Below Function Executes On Form Submit function ValidationEvent() { // Storing Field Values In Variables var name ...
Get code examples like"javascript onsubmit form validation". Write more code and save time using our ready-made code examples. The onSubmit event handler is used to execute specified JavaScript code whenever the user submits a form, and as such, is included within the HTML <FORM> tag. The onSubmit event handler uses the following properties of the Event object: type - indicates the type of event. target - indicates the target object to which the event was sent. There are two main ways to submit a form: The first - to click <input type="submit"> or <input type="image">. The second - press Enter on an input field. Both actions lead to submit event on the form. The handler can check the data, and if there are errors, show them and call event.preventDefault (), then the form won't be sent to the server.
Basic Form Validation. First let us see how to do a basic form validation. In the above form, we are calling validate() to validate data when onsubmit event is occurring. The following code shows the implementation of this validate() function. <script type = "text/javascript"> <!-- // Form validation code will come here. The server reads form data and the file, as if it were a regular form submission. Summary. FormData objects are used to capture HTML form and submit it using fetch or another network method. We can either create new FormData(form) from an HTML form, or create an object without a form at all, and then append fields with methods: formData.append ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... The onsubmit attribute fires when a form is submitted. Browser Support. Event Attribute; onsubmit: Yes: Yes: Yes: Yes: Yes: Syntax <form onsubmit="script"> Attribute Values ...
12/8/2015 · JavaScript onsubmit Event Example. Updated on August 12, 2015 Kisan Patel. If we want to execute a javascript function when the html form is being submitted, we need to use javascript onsubmit event. <!--. HTML --> <form action="" method="post" onsubmit="return TestFunction ()"> Password: <input type="password" name="txtPassword" ... GlobalEventHandlers.onsubmit. The onsubmit property of the GlobalEventHandlers mixin is an event handler that processes submit events. The submit event fires when the user submits a form. Redirect to URL in new tab Method 2 #. The down side of option 1 is that browser pop-up blockers can prevent your redirect window from opening. Option 2 provides another way to open new page/tab on submit without triggering pop-up blockers. To do this, you'll need to go to your form settings -> Customize HTML and add the following to the submit ...
The onsubmit event is performed the triggers is based upon the submit function whenever the client user request in the form-wise data is submitted to the server or the client request is supposed to be cancelled or aborted the submission of the datas in JavaScript. The method form.onsubmit () allowed to be initiated in the form datas which is to ... Finally, the form tag includes an onsubmit attribute to call our JavaScript validation function, validate_form(), when the "Send Details" button is pressed. The return allows us to return the value true or false from our function to the browser, where true means "carry on and send the form to the server", and false means "don't send ... sample-registration-form-validation.js is the external JavaScript file which contains the JavaScript ocde used to validate the form. js-form-validation.css is the stylesheet containing styles for the form. Notice that for validation, the JavaScript function containing the code to validate is called on the onSubmit event of the form.
When you submit the form, the submit event is fired before the request is sent to the server. This gives you a chance to validate the form data. If the form data is invalid, you can stop submitting the form. To attach an event listener to the submit event, you use the addEventListener() method of the form element as follows: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... Example. Execute a JavaScript when a form is submitted: <form onsubmit="myFunction()"> Enter name: <input type="text"> Sample code for javascript form submit with validations
<form method="post" action="javascript" enctype="text/plain" onsubmit="return valForm(this);"> And added this at the top of the page, a simple validation function:
Javascript Form Submission How To Submit A Form In
How To Handle Forms In React The Alternative Approach Dev
Javascript Field Level Form Validation W3resource
How To Invoke Customvalidator Function And Page Validation
Adding A New Todo Form Vue Events Methods And Models
Why Use A Third Party Form Validation Library Css Tricks
Demystifying Enter Key Submission For React Forms
Form Validation Lt Javascript The Art Of Web
Reactjs A Quick Tutorial To Build Dynamic Json Based Form
How To Build Forms With React The Easy Way
React Hook Form Onsubmit Code Example
Events In Javascript Part Three
Display Form Results With Javascript Mvcode
Need Help With Setting Getting Cookie For Form Chegg Com
How To Create A Working Contact Form In Next Js By Chad
Handling A Form In React Programming With Mosh
Difference Between Onsubmit And Onclick Events In Javascript
How To Use React Hook Form For Dead Simple Forms By Reed
Form Verification Example Javascript The Definitive Guide
Javascript Onsubmit How Onsubmit Event Work In Javascript
Javascript Onsubmit Event Tutorial For Beginners How To Handle Forms In Javascript
12 Javascript Tutorial Form Level Events Onsubmit And Onreset
Form Onsubmit Not Working Issue 388 Reactstrap
Handling React Forms And Validation With Formik And Yup By
Javascript Form Validation Script More Features Javascript
Form Validation With Javascript
0 Response to "27 Javascript Form Onsubmit Example"
Post a Comment