25 Array Slice Javascript Example
If start > array.length, splice () does not delete anything and starts appending arguments to the end of the array. If start < 0, the index is counted from backward (array.length + start). For example, -1 is the last element. If array.length + start < 0, it will begin from index 0. In the example at first marigold is removed from index 2. Now, the modified array holds rosemary and hibiscus at index 5 and 6. So, removed them using *splice(5,2). Lastly, "sunflower" has been replaced by "tulip". In the process of changing the original array, with the first execution, the value of the indices has been changed and depending on that we executed the next steps.
Javascript Array Slice How To Slice An Array In Javascript
Javascript array slice(): Extracts a section of an array and returns a new array. It extracts the elements from a specified starting index to end index but excludes the element which is at the end Index.
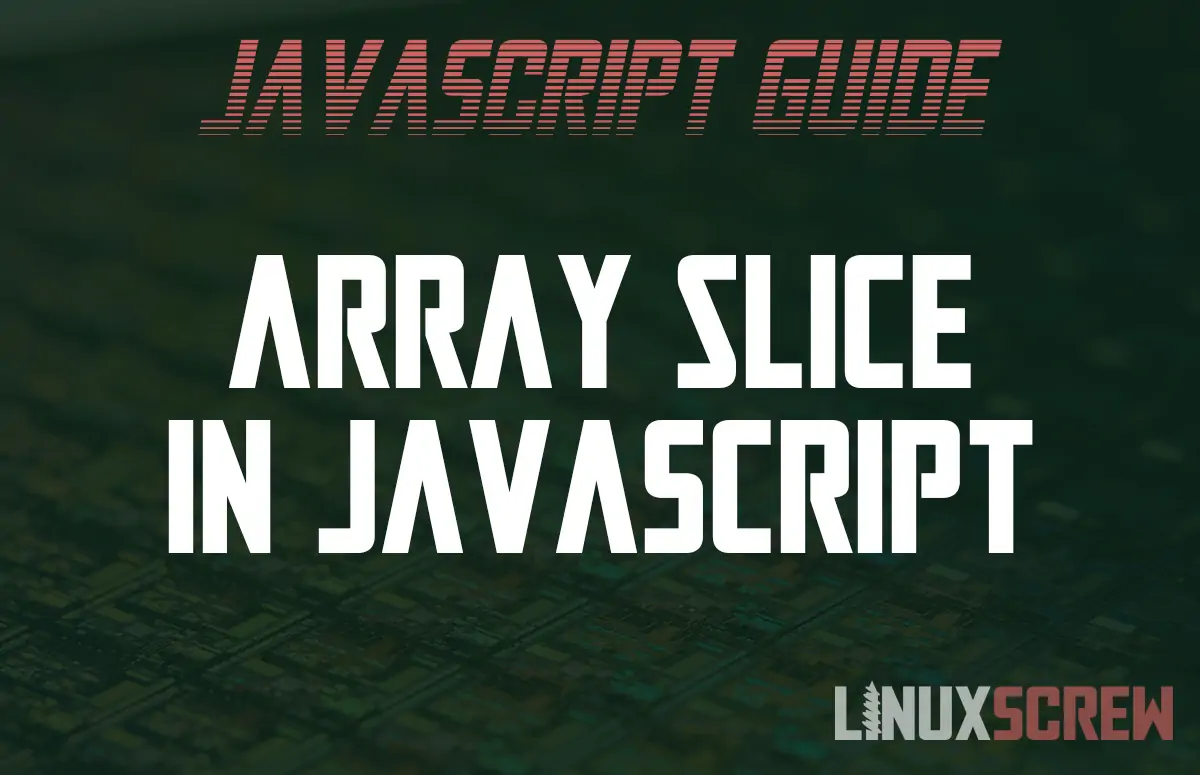
Array slice javascript example. 14/2/2012 · JavaScript array.slice () explained with examples #.slice () is a method on all instances of arrays in JavaScript. It accepts two parameters - start index and end index - both of which are optional, and returns an array of the sliced items. It is a useful function for 'chopping off' items from an array. Use negative numbers to select from the end of an array. If omitted, it acts like "0". end. Optional. An integer that specifies where to end the selection. If omitted, all elements from the start position and to the end of the array will be selected. Use negative numbers to select from the end of an array. Hi, in this tutorial, we are going to talk about splice() array method in javascript ES6 with example. splice() in Javascript. This array method provides the functionality of replacing that is inserting or removing slices to the existing array. The one thing to note about the splice() method is that unlike slice() and concat() methods, the ...
Slice leaves the original array intact and returns a shallow copy of selected items, splice modifies the original array. How Javascript Array Slice Works. Before we dive into some of the more advanced uses, lets look at the basics of the slice method. As shown in the MDN documentation, slice is a method on an array that takes up to 2 arguments ... I will use it below in examples: let array = [1, 2, 3, "hello world", 4.12, true]; This usage is valid in JavaScript. An array with different data types: string, numbers, and a boolean. Slice ( ) The slice( ) method copies a given part of an array and returns that copied part as a new array. It doesn't change the original array. array.slice ... The definition of 'Array.prototype.slice' in that specification. Standard: ECMAScript 2015 (6th Edition, ECMA-262) The definition of 'Array.prototype.slice' in that specification. Standard: ECMAScript (ECMA-262) The definition of 'Array.prototype.slice' in that specification. Living Standard
JavaScript Array splice () method. The JavaScript array splice () method is used to add/remove the elements to/from the existing array. It returns the removed elements from an array. The splice () method also modifies the original array. Code language: JavaScript (javascript) In this example, the arguments of the toArray () function is an array-like object. Inside the toArray () function, we called the slice () method to convert the arguments object into an array. Every argument we pass to the toArray () function will be the elements of the new array. slice () splice () This method is used to get a new array by selecting a sub-array of a given array. This method is used to add/remove an item from the given array. The parameter 's' indicates the starting index and 'e' indicates the ending index. They denote the index of the sub-array to be taken.
In real life arrays of objects is a common thing, so the find method is very useful.. Note that in the example we provide to find the function item => item.id == 1 with one argument. That's typical, other arguments of this function are rarely used. Specifies where the function will start the slice. 0 = the first element. If this value is set to a negative number, the function will start slicing that far from the last element. -2 means start at the second last element of the array. Optional. Numeric value. Specifies the length of the returned array. Hi, in this tutorial, we are going to talk about slice() array method in javascript ES6 with the help of example. slice() in Javascript. These array method comes under the extracting category. In the last tutorial, we have discussed various array methods like reduce, filter, map, push, pop, flat, flatMap, and many more.
1. The splice() method returns the removed item(s) in an array and slice() method returns the selected element(s) in an array, as a new array object.. 2. The splice() method changes the original array and slice() method doesn't change the original array.. 3. The splice() method can take n number of arguments:. Argument 1: Index, Required. An integer that specifies at what position to add ... 10/6/2020 · Q: How to remove an element from array javascript? Answer: The splice() method adds/removes items to/from an array, and returns the removed item(s). See below example of remove elements from array in JS. var fruits = ["Banana", "Orange", "Apple", "Mango"]; fruits.splice(0, 1); // Removes the first element of fruits Learn JavaScript Array slice ( ), splice ( ) and split ( ) methods...They are being confused a lot among developers. In this video, you're going to learn wha...
Below examples illustrate the JavaScript Array slice () method: Example 1: In this example the slice () method extracts the entire array from the given string and returns it as the answer, Since no arguments were passed to it. var arr = [23,56,87,32,75,13]; var new_arr = arr.slice (); document.write (arr); document.write (new_arr); Output: Array splice() and slice() methods look similar, but both are different and used for different use cases. These methods are most commonly used array methods. In this tutorial, we will learn both of these methods with different examples for each. splice() method : splice() can modify the array elements or we can say that it is a mutator method. The slice () method shallow copies the elements of the array in the following way: It copies object references to the new array. (For example, a nested array) So if the referenced object is modified, the changes are visible in the returned new array. It copies the value of strings and numbers to the new array.
slice method can also be called to convert Array-like objects/collections to a new Array. You just bind the method to the object. The arguments inside a function is an example of an 'array-like object'. function list() { return Array. prototype.slice.call(arguments) } let list1 = list(1, 2, 3) Array.prototype.slice() The slice() method returns a shallow copy of a portion of an array into a new array object selected from begin to end (end not included). The original array will not be modified. Try with for loop with a increment of 2 in each iteration. An array slice is a method of slicing the given array to obtain part of the array as a new array. In javascript, there is a method known as the slice () method for selecting part of the given elements and return this as a new array object without changing the original array. Thus the new selected elements of the array are copied to a new array.
8/5/2020 · The JavaScript Array slice() method returns the new array of selected items in a bigger array. It doesn’t change the original array. Following is the code for the array slice() method −. Example. … In this tutorial, we are going to learn slice( ), splice( ), & split( ) methods in JavaScript with examples. This methods are mostly used in react and react native application development, so i though to share my knowledge and some real time examples.Slice( ) and splice( ) methods are used for arrays.Split( ) method is used for strings. In this tutorial we're going to learn about the #slice #JavaScript #Array Method and how it can be used to retrieve a portion of an array.It can also be used...
JavaScript Array slice() method. The JavaScript array slice() method extracts the part of the given array and returns it. This method doesn't change the original array. Syntax. The slice() method is represented by the following syntax: The JavaScript array slice() method is used to return a new array containing the copy of the part of the given array. Note: Original array will not be modified. It will return a new array. Syntax: array.slice(start,end) Parameters: start: It is optional parameter and represents the index from where the function starts to fetch the elements. JavaScript get the last n elements of array | slice example code. Posted September 7, 2020 May 15, 2021 by Rohit. How to get the last n elements of array in JavaScript? For an example given array have 10 elements and we want the last 4 elements. Use slice and length methods.
Indexing And Slicing Numpy Arrays
Javascript Write Your Own Wrapper Functions Tkssharma
Array Slice Method In Javascript With Examples
Javascript Array Move An Array Element From One Position To
Splice Slice Amp Split What S The Difference By Maria
Javascript Arraybuffer Slice Property Geeksforgeeks
Understanding The Slice Method In Javascript The Basics The
Javascript Array Splice Delete Insert And Replace
Javascript Array Slice Vs Splice Ariya Io
Javascript Array A Complete Guide For Beginners Dataflair
Array Slice Vs Splice In Javascript Alligator Io
A List Of Javascript Array Methods By Mandeep Kaur Medium
Why Is Array Slice Not Working Js Code Example
Understanding The Slice Method In Javascript The Basics The
7 Distinct Uses Of Javascript Array Slice Outsource It Today
Javascript Splice Slice Split By Jean Pan Medium
What Is The Difference Splice Slice And Split Method In
Mongodb Slice Modifier Geeksforgeeks
A Comprehensive Guide Of Arrays And Slices In Golang And
Javascript 101 Array Slice And Array Splice Methods
Php Array Slice How To Use Array Slice Function
All You Need To Know About Javascript Arrays
0 Response to "25 Array Slice Javascript Example"
Post a Comment