34 Declare Global Variable In Javascript
My code is in JavaScript. It is a controller for my component. I want to declare a global variable but am I declaring it correctly? Simplified code: ({ x: 10, /* Global variable */ y: 15 /* Global variable */ function1 : function(..) Like other programming languages, JavaScript also has local and global variables. But the declaration and scope of a variable in JavaScript is different from others programming languages. In this article, I am going to explain the difference between local and global variables in JavaScript.
Using Variables Postman Learning Center
Local variable is declared inside a function whereas Global variable is declared outside the function. Local variables are created when the function has started execution and is lost when the function terminates, on the other hand, Global variable is created as execution starts and is lost when the program ends.
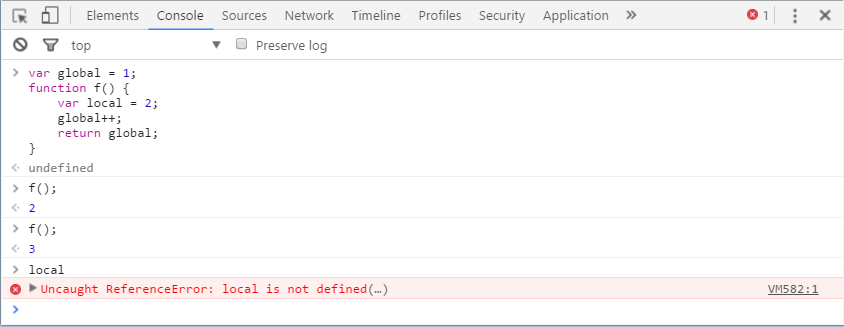
Declare global variable in javascript. If you forget to code the var keyword in a variable declaration, the JavaScript engine assumes that the variable is global. This can cause debugging problems. In general, it's better to pass local variables from one function to another as parameters than it is to use global variables. This results in declaring a global variable as property of the global object. It is not a recommended way, source of bugs and you should avoid declaring variables without the keyword var. With let keyword. ECMAScript 2015 introduced block-scoped variables. To declare block-scope local variable use let keyword instead of var: let size = 12; Global Variable. A global variable is one that can be accessed, whether it is retrieving the value of, or assigning a value to it, anywhere in an application. Global variables are used for variables that will need to be accessed throughout an application, like totals, file paths, etc. As a rule of thumb, it is best to avoid using too many ...
The use of the varkeyword can easily lead to declaring global varsthat were intended to be local or vice versa. This sort of variable scoping is a point of confusion for a lot of Javascript developers. So as a general rule, I make sure all variable declarations are preceded with the keyword varor the prefix window. Global Variables in JavaScript Explained. Global variables are declared outside of a function for accessibility throughout the program, while local variables are stored within a function using var for use only within that function's scope. If you declare a variable without using var, even if it's inside a function, it will still be seen as ... 25/5/2020 · To declare a global variable, you can use the var at global scope like this: Watch a video course JavaScript - The Complete Guide (Beginner + Advanced) let yourGlobalVariable = "global value" ; //global variable function displayGlobalVal ( ) { console .log(yourGlobalVariable); //global variable , result is "global value" } displayGlobalVal();
JavaScript variables have only two scopes. Global Variables − A global variable has global scope which means it can be defined anywhere in your JavaScript code. Local Variables − A local variable will be visible only within a function where it is defined. Function parameters are always local to that function. Declaring Variable: A variable can be either declared as a global or local variable. Variables can be declared by var, let, and const keywords. Before ES6 there is only a var keyword available to declare a JavaScript variable. Global Variables are the variables that can be accessed from anywhere in the program. Static variable in JavaScript: We used the static keyword to make a variable static just like the constant variable is defined using the const keyword. It is set at the run time and such type of variable works as a global variable. We can use the static variable anywhere. The value of the static variable can be reassigned, unlike the constant ...
Let's declare a variable, age, and use the assignment operator (the equals sign) to assign our value, 4, to this variable. We'll use the var keyword. var age = 4. Variables are how programmers give a name to a value so that we can reuse it, update it, or simply keep track of it. Variables can be used to store any JavaScript type. Summary: in this tutorial, you will learn how to use the JavaScript let keyword to declare block-scoped variables. Introduction to the JavaScript let keyword. In ES5, when you declare a variable using the var keyword, the scope of the variable is either global or local. If you declare a variable outside of a function, the scope of the variable ... A variable declared outside a function scope is a global variable. In this sense, the variable is in a global context. Also remember, assigning a value to an undeclared variable makes it a global variable by default.
To create a true global variable in Node.js, that is shared between multiple files, you should use the global object. // file1.js global.answer = 42; // file2.js global.answer; // 42 With Webpack. Like Node.js, Webpack supports a global object that you should use to declare global variables. That's because Webpack compiles the below JavaScript: Javascript Object Oriented Programming Front End Technology To declare a global variable, use the var at global scope − <script> var myGlobalVariable; function display() { // } </script> For JavaScript function, assign the property to a window − JavaScript global variables work in the whole JavaScript environment. From the first line of code, your global JavaScript variable scope begins and extends to the end of the script. Variables in JavaScript become available from the moment they are declared. They stop when the function ends.
This tutorial introduces how to declare global variables in JavaScript. Variables hold the data and information, which can be edited anytime. In JavaScript, the variables can be declared using keywords such as const, let, and var. The scope of the variable is usually defined by the place of their declaration. In the global context, a variable declared using var is added as a non-configurable property of the global object. This means its property descriptor cannot be changed and it cannot be deleted using delete. All JavaScript variables must be identified with unique names. These unique names are called identifiers. Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume). The general rules for constructing names for variables (unique identifiers) are: Names can contain letters, digits, underscores, and dollar signs.
I have a situation where I want to set a global variable in javascript for every View returned by all my controller methods. My javascript files will be using the global variable for some processing. I have so many controller methods that return a View so I don't want to return it as part of ViewModel or ViewBag for each controller method. To ceate a variable on the document level, that all scripts in the document can access you would declare that variable in a document level script, but outside of any function scope. To create a true global varible, there is a global object in Acrobat's JavaScript implementation. 19/5/2021 · Global variables are declared at the start of the block (top of the program) Var keyword is used to declare variables globally. Global variables can be accessed from any part of the program. Note: If you assign a value to a variable and forgot to declare that variable, it will automatically be considered as a global variable.
We know that what global variables or constants are, these are fields which are accessible application wide. In java, it is done usually done using defining " public static " fields. Here by adding final keyword, we can change the global variable to global constant. If a variable is going to be used throughout the program it is important that you declare the variable in global scope. But, because JavaScript allows re-declaration of same variables, it can easily become a problem if a function is using a variable with same name as global variable in its scope. Normally accessing this variable's value will ... The variables declared without the var keyword becomes global variables, irrespective of where they are declared. Visit Variable Scope in JavaScript to learn about it. It is Not Recommended to declare a variable without var keyword because it can accidentally overwrite an existing global variable.
Let’s see the simple example of global variable in JavaScript. <script>var value=50;//global variablefunction a(){alert(value);}function b(){alert(value);}</script>. Test it Now. Declaring JavaScript global variable … While it is possible to define a global variable by just omitting var (assuming there is no local variable of the same name), doing so generates an implicit global, which is a bad thing to do and would generate an error in strict mode. answered Mar 24 Huzaifa 86.2k Although global variables are accessible in modules, JavaScript modules have to use an indirect way to declare them because each module has its own scope. That means that modules behave as if their...
Variables declared Globally (outside any function) have Global Scope. Global variables can be accessed from anywhere in a JavaScript program. Variables declared with var, let and const are quite similar when declared outside a block. They all have Global Scope:
Window Variable Javascript Declare Global Variables Inside
Javascript Correct Way To Define Global Variables
Global And Local Variables In Javascript Geeksforgeeks
Chapter 16 Variables Scopes Environments And Closures
Chapter 16 Variables Scopes Environments And Closures
Javascript 20 Local And Global Variables
Javascript Scope Of Variables Huey2672 博客园
Resharper Javascript Use Of Implicitly Declared Global
Scope Of Variables In C Geeksforgeeks
Local Vs Global Scope And Let Vs Var In Javascript By
What Is Global Variable Name In Javascript Variable Targeting
Variable Global Variables Or Global Scope In Javascript
Javascript Global Object Contentful
Internet Explorer Global Variable Blow Ups Rick Strahl S
Global Variables In The Javascript Dailyusefulentertaining Com
Using Variables Postman Learning Center
10 Which Best Describes Global Scope In Javascript Chegg Com
Javascript Global Variable Code Bridge Plus
How To Change The Value Of A Global Variable Inside Of A
Using Variables Postman Learning Center
Javascript Function Tutorial Part 3 Functions Global Variable Vs Local Variable
Tools Qa What Are Javascript Variables And How To Define
Define Global Variable In Laravel 5 8 Itsolutionstuff Com
Javascript Variables A To Z Guide For A Newbie In
Scope And Lifetime Of Variables Springerlink
Jquery Global Variable How To Working Of The Jquery Global
Comprehending Variables Statement And Expression In
Differences Between Local Scope And Global Scope Variable In
Declare Global Variable In Javascript Code Example
Variable Global Variables Or Global Scope In Javascript
Global Variables In Javascript Explained
0 Response to "34 Declare Global Variable In Javascript"
Post a Comment