25 Javascript Get Set Function
The JavaScript addEventListener() method allows you to set up functions to be called when a specified event happens, such as when a user clicks a button. This tutorial shows you how you can implement addEventListener() in your code. Understanding Events and Event Handlers Events are actions that happen when the 23/8/2021 · Set.prototype.size – It returns the number of elements in the Set. Methods: Set.prototype.add() – It adds the new element with a specified value at the end of the Set object. Syntax: set1.add(val); Parameter: val - It is a value to be added to the set. Returns: The set object. Example:
Javascript Set How To Use Sets In Javascript
Description In JavaScript, a setter can be used to execute a function whenever a specified property is attempted to be changed. Setters are most often used in conjunction with getters to create a type of pseudo-property. It is not possible to simultaneously have a setter on a property that holds an actual value.
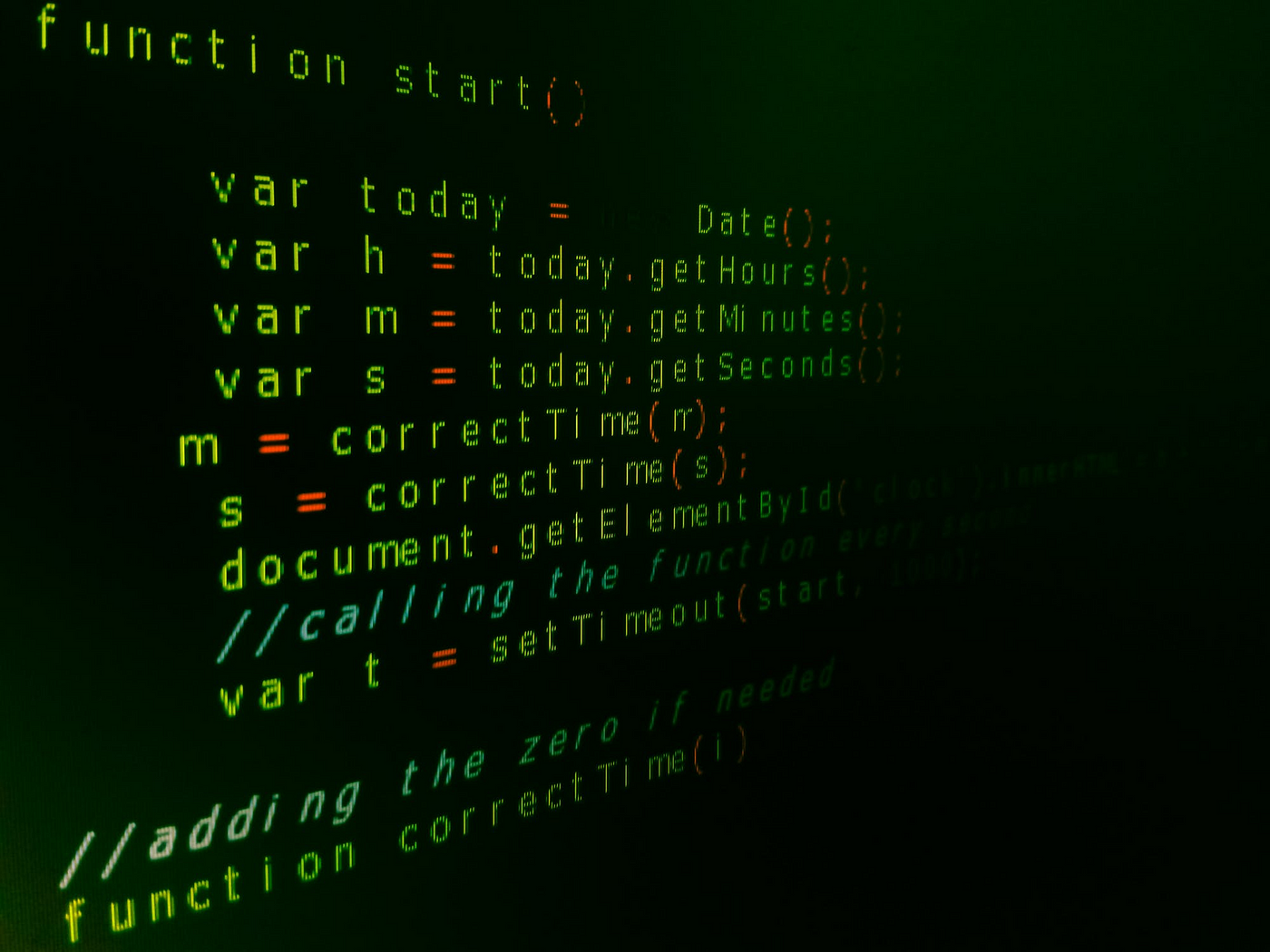
Javascript get set function. Get and Set ES6 classes brings a new syntax for getters and setters on object properties Get and set allows us to run code on the reading or writing of a property. ES5 had getters and setters as well but was not widely used because of older IE browsers. ES5 getters and setters did not have as nice of a syntax that ES6 brings us. A Set is a special type collection - "set of values" (without keys), where each value may occur only once. Its main methods are: new Set (iterable) - creates the set, and if an iterable object is provided (usually an array), copies values from it into the set. set.add (value) - adds a value, returns the set itself. JavaScript interval to be set use setInterval () function. It could be defined simply as a method which allows you to invoke functions in set intervals. The JS setInterval () function is similar to the setTimeout method. How are they different?
The forEach() function of the Set object accepts the name of a particular function and runs that function for every value in the current set. For Example, if you have written a function to print a value, if you use forEach() function it prints the value of every element in the set. 35 Javascript Get Set Function Written By Roger B Welker. Sunday, August 22, 2021 Add Comment Edit. Javascript get set function. Advanced Functionality With Functions In Javascript Dev. What Is Web Resource How To Use Javascript How To Use Javascript Function Dynamics Crm. If you have ever been tasked with writing a find function in plain JavaScript, you would probably have used a for loop. In ES6, a new array function named find() was introduced. Longhand:
8 rows · It returns an object of Set iterator that contains an array of [value, value] for each element. forEach() It executes the specified function once for each value. has() It indicates whether the Set object contains the specified value element. values() It returns an object of Set iterator that contains the values for each element. Function Basics. Level Set! If you are coming from a programming background, you all know what declaring a function means, right? It looks something like this in JavaScript. Accessor properties are represented by "getter" and "setter" methods. In an object literal they are denoted by get and set: let obj = { get propName() { }, set propName(value) { } }; The getter works when obj.propName is read, the setter - when it is assigned.
The so-called getter and setter methods represent accessor properties. They are specified by get and set inside an object literal as shown below: let obj = { get propName () { // getter, the code executed when obj.propName id getting }, set propName (value) { // setter, the code executed when obj.propName = value is setting } }; In JavaScript, this can be accomplished with the use of a getter. It is not possible to simultaneously have a getter bound to a property and have that property actually hold a value, although it is possible to use a getter and a setter in conjunction to create a type of pseudo-property. Note the following when working with the get syntax: In this post, we will cover how to get and set values for fields on Microsoft Dynamics 365/CRM forms. Dynamics 365 has the following types of fields (or datatypes): Single Line of Text, Option Set, MultiSelect Option Set, Two Options, Image, Whole Number, Floating Point Number, Floating Point Number, Decimal Number, Currency, Multiple Lines of Text, Date and Time, Lookup and Customer.
ECMAScript 5 (ES5 2009) introduced Getter and Setters. Getters and setters allow you to define Object Accessors (Computed Properties). JavaScript Getter (The get Keyword) This example uses a lang property to get the value of the language property. By default, the lifetime of a cookie is the current browser session, which means it is lost when the user exits the browser. For a cookie to persist beyond the current browser session, you will need to specify its lifetime (in seconds) with a max-age attribute. This attribute determine how long a cookie can be remain on the user's system before it is deleted, e.g., following cookie will live ... The then() function will include code that handles the response received from the Random User API server: fetch(url, fetchData) .then(function() { // Handle response you get from the server }); To create an object and use the fetch() function , there is also another option.
The function is called only with one argument: sum(1).While param1 has the value 1, the second parameter param2 is initialized with undefined.. param1 + param2 is evaluated as 1 + undefined, which results in NaN.. If necessary, you can always verify if the parameter is undefined and provide a default value. Let's make the param2 default to 0: In JavaScript, a constructor function is used to create objects. For example, // constructor function function Person () { this.name = 'John', this.age = 23 } // create an object const person = new Person (); In the above example, function Person () is an object constructor function. To create an object from a constructor function, we use the ... JavaScript onload. In JavaScript, this event can apply to launch a particular function when the page is fully displayed. It can also be used to verify the type and version of the visitor's browser. We can check what cookies a page uses by using the onload attribute. In HTML, the onload attribute fires when an object has been loaded.
In JavaScript, accessor properties are methods that get or set the value of an object. For that, we use these two keywords: get - to define a getter method to get the property value set - to define a setter method to set the property value JavaScript functions are a special type of objects, called function objects. A function object includes a string which holds the actual code -- the function body -- of the function. The code is literally just a string. Although not recommended, you can create a new function object by passing the built-in Function constructor a string of code ... Set the value property: textObject.value = text. Property Values: text: It specifies the value of input text field. attributeValue: This parameter is required. It specifies the value of the attribute to add. setAttribute method. This method adds the specified attribute to an element, and set it's specified value.
Set property to default. inherit: Inherit property from its parent. Return Value. As most JavaScript functions, the method used for style display also has a return value. It shows the display type of a specific element. You are now familiar with all the display types. Therefore, it will be easy for you to identify the return value you get when ... In the above example, the Person() function creates a FirstName property by using Object.defineProperties() method. The first argument is this, which binds FirstName property to calling object. Second argument is an object that includes list of properties to be created. We have specified FirstName property with get & set function. A JavaScript function is a block of code designed to perform a particular task. A JavaScript function is executed when "something" invokes it (calls it). Example. function myFunction (p1, p2) {. return p1 * p2; // The function returns the product of p1 and p2. }
9/4/2019 · On this page it is shown that a object can implement get and setters in javascript. But they use a different way to create an object than my code. How can i implement get and setters in the following code: function Settings () { var fullscreen; // get function this.fullScreen = function () { if (fullscreen === undefined) { return false } ... 24/11/2012 · Get/Set local variables of JavaScript function from outside & Dynamic code insertion in JS function. I am working on a JavaScript framework that needs to get list of local function variables and set values of some of them. Unlike Java, JavaScript does not have runtime introspection Date Methods. Javascript provides us with numerous methods to operate on the date object. We can get and set milliseconds, seconds, minutes, hour, day, month or year of date using either local time or Universal (UTC or GMT) time. By default output of date object in javascript is in string format which is as follows:
These methods can be used for getting information from a date object: Method. Description. getFullYear () Get the year as a four digit number (yyyy) getMonth () Get the month as a number (0-11) getDate () Get the day as a number (1-31) JavaScript Global Variable. A JavaScript global variable is declared outside the function or declared with window object. It can be accessed from any function. Let's see the simple example of global variable in JavaScript. Previous: Write a JavaScript function that generates a string id (specified length) of random characters. Next: Write a JavaScript function that accepts two arguments, a string and a letter and the function will count the number of occurrences of the specified letter within the string.
Indefinite Call To Set Function In Javascript Es6 Class
Data Binding Revolutions With Object Observe Html5 Rocks
Using Method Chaining With The Revealing Module Pattern In
1 Set And Get Data In Cookies With Javascript Tutorialswebsite
Javascript Memory Creation And Code Execution Runtime By
Use Es6 Sets To Improve Javascript Performance
36 Javascript Get Set Cookie Modern Javascript Blog
Javascript Function Construction Part 3 By Austin Smith
Javascript Set How To Use Sets In Javascript
Element Getboundingclientrect Web Apis Mdn
Run Javascript In The Console Chrome Developers
Watch Modern Javascript From The Beginning Prime Video
Learn About Properties In Javascript
How To Convert Set To Array In Javascript Geeksforgeeks
Javascript Functions Understanding The Basics By Brandon
Variables Not Being Updated With Get And Set Solved Unity
Function Construction Idioms Function Call
Getters And Setters In Javascript Dev Community
Get And Set Formyoula Field Values Using The Javascript
Run Javascript In The Console Chrome Developers
Using Javascript With Embedded Data And Or Selected Choices
Powerful Js Reduce Function You Can Use It To Solve Any Data
0 Response to "25 Javascript Get Set Function"
Post a Comment