24 Javascript For Loop Step
JavaScript Loop Through Select Options - Example. May 8, 2015. March 8, 2017. Haas dom, dropdown, id, iterate, JavaScript, jquery, js, loop, Open Source, Options. Sometimes we need to iterate through all the options of a DropDown list (and perform operations based on each one). Here is how to do it (for a dropdown with the id "dropdownlist") Dealing with arrays is everyday work for every developer. In this article, we are going to see 6 different approaches to how you can iterate through in Javascript. for Loop. The for loop statement has three expressions: Initialization - initialize the loop variable with a value and it is executed once; Condition - defines the loop stop condition
Javascript Foreach Loops Made Easy Career Karma
The For step is executed first. This step allows you to initialize any loop control variables and increment the step counter variable. Secondly, the condition is evaluated. If it is true, the body of the loop is executed. If it is false, the body of the loop does not execute and flow of control jumps to the next statement just after the For Loop.
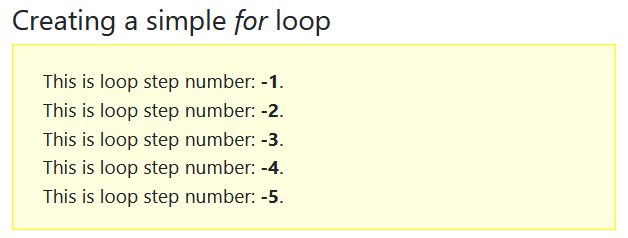
Javascript for loop step. 8/10/2012 · Use the += assignment operator: for (var i = 0; i < myVar.length; i += 3) {. Technically, you can place any expression you'd like in the final expression of the for loop, but it is typically used to update the counter variable. For more information about each step of the for loop… The syntax in a for loop is much more compact. It has the counter, the condition and the increment all in one place. To write a for loop, we use the keyword for, followed by a condition block and then an execution block containing the code we want to have loop. JavaScript provides functionality to perform for and while loops. The following sections describe how to implement loops in your JavaScript. while Loops. The most basic type of looping in JavaScript is the while loop. A while loop tests an expression and continues to execute the code contained in its {} brackets until the expression evaluates ...
The JavaScript forEach method is one of the several ways to loop through arrays. Each method has different features, and it is up to you, depending on what you're doing, to decide which one to use. In this post, we are going to take a closer look at the JavaScript forEach method. Considering that we have the following array below: Nov 02, 2017 - Loops are used in programming to automate repetitive tasks. In this tutorial, we will learn about the for statement, including the for...of and for...in statements, which are essential elements of the JavaScript programming language. The for uses 3 expressions: Initialization - initializes the loop variable with a starting value which can only be executed once. Condition - specifies that the loop should stop looping Final expression - is performed at the end of each loop execution.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. JavaScript provides full control to handle loops and switch statements. There may be a situation when you need to come out of a loop without reaching its bottom. There may also be a situation when you want to skip a part of your code block and start the next iteration of the loop. JavaScript For Loop Explained. There are four important aspects of a JavaScript for loop: The counter variable is something that is created and usually used only in the for loop to count how many times the for loop has looped. i is the normal label for this counter variable and what we will be using. The conditional statement.
The first expression, var i = 0, is the starting point. It creates a variable named i and sets its value to 0. The second expression, i < arguments.length, tells the for loop to continue processing arguments until i is equal to or greater than the value of arguments.length. In other words, the for loop will perform one cycle for each element in ... JavaScript for loop. In this tutorial, you will learn about the loops and about for loops in JavaScript with the help of examples. In programming, loops are used to repeat a block of code. For example, if you want to show a message 100 times, then you can use a loop. It's just a simple example; you can achieve much more with loops. Using unlabeled JavaScript continue statement The unlabeled continue statement skips the current iteration of a for, do...while, or while loop. The continue statement skips the rest of the code to the end of the innermost body of a loop and evaluates the expression that controls the loop.
The for statement creates a loop that is executed as long as a condition is true. The loop will continue to run as long as the condition is true. It will only stop when the condition becomes false. JavaScript supports different kinds of loops: Loops are used in JavaScript to perform repeated tasks based on a condition. Conditions typically return true or false when analysed. A loop will continue running until the defined condition returns false. The three most common types of loops are. for. while. do while. You can type js for, js while or js do while to get more info on any of these. An initializer can be specified before starting for loop. The condition and increment statements can be included inside the block. ... TutorialsTeacher is optimized for learning web technologies step by step. Examples might be simplified to improve reading and basic understanding.
Jun 23, 2017 - I have an array with 100 elements, I want to take an element from 10th to 15th (10, 11, 12, 13, 14, 15), than from 20th to 25th, than from 30th to 35th, from 40th to 45th and from 50th to 55th, so I Statement 1 sets a variable before the loop starts (let i = 0). Statement 2 defines the condition for the loop to run (i must be less than 5). Statement 3 increases a value (i++) each time the code block in the loop has been executed. Statement 1 Code language: CSS (css) How it works. First, declare a variable counter and initialize it to 1.; Second, display the value of counter in the Console window if counter is less than 5.; Third, increase the value of counter by one in each iteration of the loop.; Since the for loop uses the var keyword to declare counter, the scope of counter is global. Therefore, we can access the counter ...
Mar 13, 2015 - Of all the loop types in JavaScript, the for loop is arguably the most versatile. It's concise, easy to use, and can handle just about any looping tasks that you might be faced with. In fact, you can do a lot more with for loops that you probably ever thought possible. Jun 11, 2020 - Get code examples like "javascript for loop step size" instantly right from your google search results with the Grepper Chrome Extension. Apr 28, 2021 - Loops are used in JavaScript to perform repeated tasks based on a condition. Conditions typically return true or false when analysed. A loop will continue running until the defined condition returns false. The three most common types of loops are forwhiledo whileYou can type js for, js while or js
In this article, we will look at four different ways to looping over object properties in JavaScript. Before ES6, the only way to loop through an object was the for...in loop. The Object.keys () method was introduced in ES6 to make it easier to iterate over objects. Later in ES8, two new methods were added, Object.entries () and Object.values (). Categories of Loops in JavaScript The 'For' Loop. The For Loop comes first because of its simplicity and ease of use. It is a very user-friendly kind of loop that runs with a method of using a counter. ... Once that was done, the next step looped all the array and changed its color. ... Use for statement to loop through all elements in an array. 3.4.6. Reversed for loop. 3.4.7. Set loop step to 5 in for loop. 3.4.8. Check the loop counter. 3.4.9. Counting backward through a for loop.
Implementing loops with a step other than one is precisely as easy (or as fiddly) as implementing loops with a step equal to one. This example program uses a loop to perform integer division. It should be run with the dividend in storage location 21 and the divisor in storage location 22. May 04, 2016 - When coding JavaScript, I find myself using the for loop fairly often. Recently, when I coded, then re-coded these drop-down menus, during the re-code I ran my JavaScript/jQuery through JSLint using the option called "The Good Parts". I don't know a whole lot about JSLint, but I figured that ... JavaScript Variables — Declare Variables in JavaScript, Assign a Value with Example. 👉 Lesson 3. JavaScript Array Methods — Create Array with Example in JavaScript. 👉 Lesson 4. JavaScript Loops — For, While and Do While LOOP in JavaScript (with Example) 👉 Lesson 5. Conditional Statements — IF, Else, Else IF Conditional ...
JavaScript for Loop: Main Tips The for loop JavaScript is used to run a piece of code a set amount of times. Loops are extremely useful when used with arrays or when you want the same line of code executed multiple times without writing a lot of repetitive code. Most common loop types are for, for/in, while, and do/while. Then, we declare another for loop. This for loop makes comparisons between each element in the list. For each iteration of our inner loop, our program executes an if statement. This JavaScript if statement checks if the number on the left of a comparison is greater than the number on the right. If it is, our program swaps the numbers. The JavaScript For Loop is used to repeat a block of statements for a given number of times until the given condition is False. JavaScript For loop is one of the most commonly used loops. Let us see the syntax of the for loop in JavaScript Programming language: for (initialization; test condition; increment/decrement operator) { //Statement 1 ...
for(i = 0; i Jun 11, 2020 - This project uses AndroidX dependencies, but the 'android.useAndroidX' property is not enabled · Module not found: Can't resolve '@date-io/date-fns' in 'C:\Users\61455\Documents\React apps\mya2o\src\pages' · TypeError: undefined is not an object (evaluating '_reactNativeImagePicker.defau... 19/10/2020 · A JavaScript for loop executes a block of code as long as a specified condition is true. JavaScript for loops take three arguments: initialization, condition, and increment. The condition expression is evaluated on every loop. A loop continues to run if the expression returns true.
The combination "infinite loop + break as needed" is great for situations when a loop's condition must be checked not in the beginning or end of the loop, but in the middle or even in several places of its body. Continue to the next iteration. The continue directive is a "lighter version" of break. It doesn't stop the whole loop. The continue statement can be used to restart a while, do-while, for, or label statement.. When you use continue without a label, it terminates the current iteration of the innermost enclosing while, do-while, or for statement and continues execution of the loop with the next iteration. In contrast to the break statement, continue does not terminate the execution of the loop entirely. This loop logs only enumerable properties of the iterable object, in arbitrary order. It doesn't log array elements 3, 5, 7 or hello because those are not enumerable properties, in fact they are not properties at all, they are values.It logs array indexes as well as arrCustom and objCustom, which are.If you're not sure why these properties are iterated over, there's a more thorough explanation ...
For Loops In Different Programming Languages Martin Thoma
How To Write A While Loop 8 Steps With Pictures Wikihow
For Loop Javascript Old School Loops In Javascript For
Ilovecoding Page 6 13 Javascript Cheat Sheet On This
Looping In Javascript Web Design Amp Development Tutorials
Javascript Foreach How To Loop Through An Array In Js
Set Loop Step To 5 In For Loop In Javascript
Javascript Event Loop And Promises By Gerardo Fernandez
Iterating A Loop Using Lambda Aws Step Functions
How To Write A While Loop 8 Steps With Pictures Wikihow
For Loop In Javascript The Engineering Projects
4 Ways To Do Looping In Javascript Array
Simplest Jquery Hello World Example Crunchify
C For Loop In C Programming With Example
Javascript Tutorial For Beginners Learn Javascript Basics In 2 Minutes Javascript Loops
Understanding Generators In Es6 Javascript With Examples By
How To Write A While Loop 8 Steps With Pictures Wikihow
Python Break Continue Pass Statements With Examples
Looping In Javascript Web Design Amp Development Tutorials
Run Javascript In The Console Chrome Developers
Looping Statement In Javascript
Introduction To Javascript 6 While Loops Mvcode
0 Response to "24 Javascript For Loop Step"
Post a Comment