23 Check If Number Javascript
Check if a string contains numbers in javascript using for loop and isNaN () The isNan () function in javascript determines if the input passed is a number or not. This function returns true if the parameter is Not a Number. Else returns false. In this example, you will learn to write a JavaScript program that will check if a number is a float or an integer value.
Check If The Url Bar Contains A Specified Or Given String In
The JavaScript Number type is a double-precision 64-bit binary format IEEE 754 value, like double in Java or C#. This means it can represent fractional values, but there are some limits to what it can store. A Number only keeps about 17 decimal places of precision; arithmetic is subject to rounding.
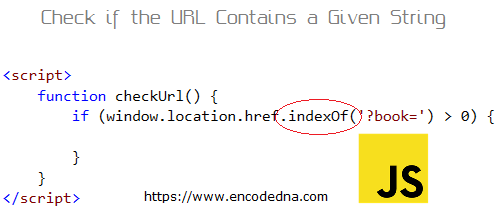
Check if number javascript. Jul 25, 2019 - how to write a function that checks the number of strings in the string and returns it in javascript ... write a function that, given a string S of length N, returns True if the string S represents a valid phone number and False if it doesn't Use the Number () Function to Check Whether a Given String Is a Number or Not in JavaScript. The Number () function converts the argument to a number representing the object's value. If it fails to convert the value to a number, it returns NaN. We can use it with strings also to check whether a given string is a number or not. May 8, 2021 June 25, 2021 amine.kouis 0 Comments check if value is number javascript, isnan javascript, javascript check if string is number, js S ometimes the user needs to fill in one or more fields with numbers (0-9) in an HTML form.
The expression "number" - 32223 results in NaN as happens when you perform a subtraction operation between a string and number. JavaScript typeof Examples The following code snippet shows the type check result of various values using the typeof operator. The Number.isInteger() method in JavaScript is used to check whether the value passed to it is an integer or not. It returns true if the passed value is an integer, otherwise, it returns false. Syntax: Number.isInteger(value) Parameters: This method accepts a single parameter value which specifies the number which the user wants to check for ... In JavaScript, there are multiple ways to check if an array includes an item. You can always use the for loop or Array.indexOf() method, but ES6 has added plenty of more useful methods to search through an array and find what you are looking for with ease.. indexOf() Method The simplest and fastest way to check if an item is present in an array is by using the Array.indexOf() method.
August 7th, 2020. JavaScript. In JavaScript, there are different ways to check whether a value is a number or something else. The most common way is to use the typeof operator: const value = 5 console.log(typeof value) One way you can use it in a practical context is to check if a form is filled out correctly, by using typeof in a conditional ... Post Link : Check If Variable Is A Number In JavaScript . This tutorial explains how to check variable is a number in javascript. Lets see the below example, where we have used typeof operator and isNaN() function to verify the variable is number or not. typeof - If variable is a number, it will returns a string named "number". JavaScript: Check if Variable is a Number. Marcus Sanatan. Introduction. JavaScript is a dynamically typed language, meaning that the interpreter determines the type of the variable at runtime. In practice, this allows us to use the same variable to store different types of data in the same code. It also means that without documentation and ...
To check for all numbers in a field. To get a string contains only numbers (0-9) we use a regular expression (/^ [0-9]+$/) which allows only numbers. Next, the match () method of the string object is used to match the said regular expression against the input value. Here is the complete web document. HTML Code. Write a function that checks whether a person can watch an MA15+ rated movie javascript One of the following two conditions is required for admittance: checkbox Type 'Event' is not assignable to type 'boolean'. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
In JavaScript Number.isInteger () method works with numbers, it will check whether a value an integer. This method returns true if the value is of the type Number and an integer. Otherwise, it will return false. If the target value is an integer, return true, otherwise, return false. If the value is NaN or Infinity, return false. Output. Enter a number: 27 The number is odd. In the above program, number % 2 == 0 checks whether the number is even. If the remainder is 0, the number is even. In this case, 27 % 2 equals to 1. Hence, the number is odd. The above program can also be written using a ternary operator. Jul 29, 2021 - JavaScript exercises, practice and solution: Write a JavaScript function to test if a number is a power of 2.
The isNaN () function determines whether a value is an illegal number (Not-a-Number). This function returns true if the value equates to NaN. Otherwise it returns false. This function is different from the Number specific Number.isNaN () method. The global isNaN () function, converts the tested value to a Number, then tests it. Java check if string contains letters and numbers Hafsa_Rao - Jul 31: Javascript check for null or empty string Lalit Kumar - Oct 2, 2020: Check if a string contains special characters java MallikaShaik672 - Nov 4, 2020: Check if a string contains a substring javascript Saravana - May 20: Check if string is a number JavaScript sakshi - May 25 First, we check whether str is a string or not with the typeof operator. This makes sure we're checking a string instead of something else. Then we call isNaN to see if we can convert it to a number and then check if the converted value is a number. If isNaN returns false , then we know there's a good chance that it's a number.
javaScript check if variable is a number: isNaN () JavaScript - isNaN Stands for "is Not a Number", if a variable or given value is not a number, it returns true, otherwise it will return false. typeof JavaScript - If a variable or given value is a number, it will return a string named "number". In JavaScript, the typeof operator ... 2019: Practical and tight numerical validity check. Often, a 'valid number' means a Javascript number excluding NaN and Infinity, ie a 'finite number'. To check the numerical validity of a value (from an external source for example), you can define in ESlint Airbnb style : Sep 17, 2020 - Usually we check types in javascript by using typeOf method but javascript doesn't support float type so it return a Number when you check a type of float number. Like: typeOf(4.56) = Number ... Explanation: If the mod of a number divided by 1 is not equal to zero then it must a float number.
Jan 27, 2021 - I was building a form the other day in Vue and I had to write number validation for the field so had... The for loop is used to iterate through the positive numbers to check if the number entered by the user is divisible by positive numbers (2 to user-entered number minus 1).. The condition number % i == 0 checks if the number is divisible by numbers other than 1 and itself.. If the remainder value is evaluated to 0, that number is not a prime number.; The isPrime variable is used to store a ... Description. If the target value is an integer, return true, otherwise return false. If the value is NaN or Infinity, return false. The method will also return true for floating point numbers that can be represented as integer.
How to check if a value is a number in JavaScript How is it possible to determine if a variable value is a number? Published Jun 21, 2020. We have various ways to check if a value is a number. The first is isNaN(), a global variable, assigned to the window object in the browser: Javascript Web Development Front End Technology Object Oriented Programming. Let's say we have the following variables −. var value1 = 10; var value2 = 10.15; Use the Number () condition to check that a number is float or integer −. Number (value) === value && value % 1 !== 0; } The Number.isInteger() method determines whether a value an integer. This method returns true if the value is of the type Number, and an integer (a number without decimals). Otherwise it returns false.
Jul 20, 2021 - You can use this, for example, to test whether an argument to a function is arithmetically processable (usable "like" a number), or if it's not and you have to provide a default value or something else. This way you can have a function that makes use of the full versatility JavaScript provides ... Jan 21, 2021 - Previous:Write a JavaScript program to find the position of a rightmost round number in an array of integers. Returns 0 if there are no round number. Next: Write a JavaScript program to find the number of elements which presents in both of the given arrays. Check if a number is Prime or not In this tutorial let us look at different methods to check if a number is prime or not in JavaScript and understand where exactly are these useful. A prime number is a natural number greater than 1 that cannot be obtained by multiplying two smaller natural numbers.
javascript even number; javascript check if a value is an int; js check if number has decimals; javascript check if in array; javascript check if number is hexadecimal; javascript if not; javascript check if a value is a integer number; js test if array; javascript check if is array; javascript check if string is number; check if function ... Write a function that takes in an input and returns true if it's an integer and false otherwise. ... Use the JavaScript documentation to find a method on the built-in Number object that checks if a number is an integer. 26/2/2020 · Write a JavaScript function to check if a number is a whole number or has a decimal place. Note : Whole Numbers are simply the numbers 0, 1, 2, 3, 4, 5, ... (and so on). No Fractions! Test Data : console.log (number_test (25.66)); "Number has a decimal place." console.log (number_test (10));
29/8/2012 · In JavaScript, there are two ways to check if a variable is a number : isNaN () – Stands for “is Not a Number”, if variable is not a number, it return true, else return false. typeof – If variable is a number, it will returns a string named “number”. Note. Nov 19, 2020 - Numbers are a primitive type in JavaScript, but just using typeof will not differentiate numbers from NaN or Infinity. Dr. Derek Austin 🥳 ... It is useful to know how to check if a value is of the primitive type number, since JavaScript is a dynamically typed language. In JavaScript, there are two ways to check if a variable is a number : isNaN () - Stands for "is Not a Number", if variable is not a number, it return true, else return false. typeof - If variable is a number, it will returns a string named "number".
How To Check If A Javascript Array Is Empty Or Not With Length
How To Check If A Value Is A Number In Javascript Anjan Dutta
How To Check If An Element Is Hidden Or Visible With Javascript
Checking If An Array Contains A Value In Javascript
4 Ways To Check If The Property Exists In Javascript Object
Q1 Write A Javascript Function That Will Return Chegg Com
How To Check If Array Is Empty In Javascript Codekila
Javascript Check If Variable Exists Is Defined Initialized
How To Check If Variable Is An Array In Javascript
How To Check If String Contains Only Digits In Javascript
Javascript String Contains Check If A String Contains A
Check If An Array Is Empty In Javascript
Javascript Check If Checkbox Is Checked Or Unchecked Example
Testing Javascript With Jest Vegibit
How To Check If A Variable Is A String In Javascript
How To Check If Object Is Empty In Javascript Upmostly
How To Check If An Object Is An Array In Javascript
How To Check If An Integer Is Safe In Javascript Spursclick
Javascript Check If Variable Is Number Code Example
Javascript Math Check If A Number Is A Whole Number Or Has A
How To Check If A String Contains Substring Or A Word Using
Short Essay 9 Javascript Basics Prime Student Chegg Com
0 Response to "23 Check If Number Javascript"
Post a Comment