32 Date String To Date Javascript
Calling new Date () (the Date () constructor) returns a Date object. If called with an invalid date string, it returns a Date object whose toString () method returns the literal string Invalid Date. Calling the Date () function (without the new keyword) returns a string. The toLocaleDateString () method returns a string with a language sensitive representation of the date portion of this date. The new locales and options arguments let applications specify the language whose formatting conventions should be used and allow to customize the behavior of the function.
Sql Server Functions For Converting A String To A Date
18/6/2021 · Date object one method called toDateString() which returns date in the form of string Here is an example for Converting from Date to String var date = new Date ( '2017 - 03 - 07T10 : 00 : 00' ); let str = date . toDateString (); console . log ( str ) //Tue Mar 07 2017 console . log ( typeof str ) // string
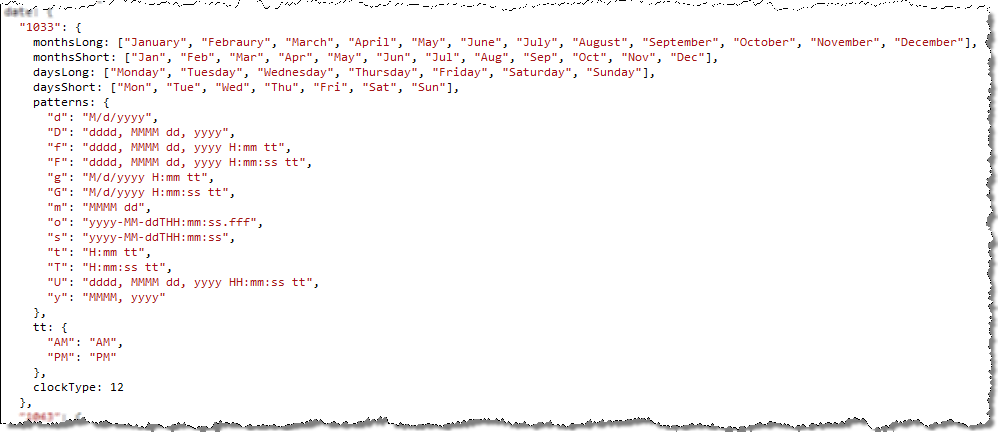
Date string to date javascript. 10/8/2019 · In order to parse dates from this string format to Date objects in JS, all you need to do is pass this string to the Date constructor. For example, Example let a = '2019-08-10'; console.log(new Date(a)) Output. This will give the output −. Sat Aug 10 2019 05:30:00 GMT+0530 (India Standard Time) Note that the new date is created at 0000 hrs UTC. How to validate if input date (start date) in input field must be before a given date (end date) using express-validator ? 17, Aug 20 How to convert UTC date time into local date time using JavaScript ? date.getFullYear()+'-' + (date.getMonth()+1) + '-'+date.getDate();//prints expected format.
JavaScript Date: Create, Convert, Compare Dates in JavaScript. JavaScript provides Date object to work with date & time, including days, months, years, hours, minutes, seconds, and milliseconds. Use the Date () function to get the string representation of the current date and time in JavaScript. Use the new keyword in JavaScript to get the Date ... Use the getTime Method. We can use the getTime method of a Date instance to convert the date string into a timestamp. To use it, we write: const toTimestamp = (strDate) => { const dt = new Date (strDate).getTime (); return dt / 1000; } console.log (toTimestamp ('02/13/2020 23:31:30')); We create the Date instance with the Date constructor. The Date object is used for representing date and time in JavaScript. It includes both of them. As a rule, the months start from zero and end with 11. The weekdays are also counted starting from zero.
20/6/2020 · The toString() method returns the string representation of the specified date. We can also access individual information of the date by using the following methods. getDate(): It returns the day of the month. getMonth(): It returns the month of a year, where 0 is January and 11 is December. getFullYear(): It returns the year in four-digit format (YYYY). JavaScript's Date Object JavaScript has a built-in Date object that stores the date and time and provides methods for handling them. To create a new instance of the Date object, use the new keyword: const date = new Date (); JavaScript Date Output By default, JavaScript will use the browser's time zone and display a date as a full text string: You will learn much more about how to display dates, later in this tutorial.
The toString () method converts a Date object to a string. Note: This method is automatically called by JavaScript whenever a Date object needs to be displayed as a string. I am stuck in a weird situation and unfortunately, even after doing some RnD and googling, I am unable to solve this problem. I have a date string in ISO format, like 2014-11-03T19:38:34.203Z and i want to convert it into a date object with new Date() method.. But when i do so, output is: In the date-string method, you create a date by passing a date-string into new Date. new Date('1988-03-21') We tend towards the date-string method when we write dates. This is natural because we've been using date strings all our lives.
javascript calculate age given date string; javascript get years since a date; jquery today date; milliseconds to days javascript; javascript date to string; javascript date is an object; excel date to javascript date; current month in javascript; convert milliseconds to minutes and seconds javascript; js get timezone name; js get iana timezone ... These methods can be used for getting information from a date object: Method. Description. getFullYear () Get the year as a four digit number (yyyy) getMonth () Get the month as a number (0-11) getDate () Get the day as a number (1-31) Use the new Date() Function to Convert String to Date in JavaScript The most commonly used way to convert a string to date is with the new Date() function. new Date() takes arguments in various forms described below but returns a date object.
Using the Date.parse () Method. One way to check if a string is date string with JavaScript is to use the Date.parse method. To do this, we write: console.log (Date.parse ('2020-01-01')) console.log (Date.parse ('abc')) Date.parse returns a timestamp in milliseconds if the string is a valid date. Otherwise, it returns NaN . new Date(date) === 'Invalid Date' only works in Firefox and Chrome. IE8 (the one I have on my machine for testing purposes) gives NaN. As was stated to the accepted answer, Date.parse(date) will also work for numbers. So to get around that, you could also check that it is not a number (if that's something you want to confirm). The toDateString () method returns the date portion of a Date object in English in the following format separated by spaces: First three letters of the week day name First three letters of the month name Two digit day of the month, padded on the left a zero if necessary
JavaScript date parse format dd/mm/yyyy. If you have the MM/DD/YYYY format which is the default for JavaScript, you can simply pass your string to Date (string) constructor. It will parse it for you. var dateString = "07/30/202020"; // July 30 var dateObject = new Date (dateString); Date and time in JavaScript are represented with the Date object. We can't create "only date" or "only time": Date objects always carry both. Months are counted from zero (yes, January is a zero month). Days of week in getDay () are also counted from zero (that's Sunday). 10/4/2011 · I have created a fiddle for this, you can use toDate() function on any date string and provide the date format. This will return you a Date object. https://jsfiddle /Sushil231088/q56yd0rp/ "17/9/2014".toDate("dd/MM/yyyy", "/")
JavaScript Date Time Format. The above shown date time is the default format. It's not so straight to change JavaScript date time format. Change Date Format dd-mmm-yyyy. To convert date to format dd-mmm-yyyy you need to extract the date, month and year from the date object. Date Input - Parsing Dates. If you have a valid date string, you can use the Date.parse() method to convert it to milliseconds. Date.parse() returns the number of milliseconds between the date and January 1, 1970: Previous JavaScript Date Reference Next ... Definition and Usage. The toDateString() method converts the date (not the time) of a Date object into a readable string. Browser Support. Method; toDateString() Yes: Yes: Yes: Yes: Yes: Syntax. Date.toDateString() Parameters. None: Technical Details. Return Value: A String, representing the date as a ...
A JavaScript date is fundamentally specified as the number of milliseconds that have elapsed since midnight on January 1, 1970, UTC. This date and time are not the same as the UNIX epoch (the number of seconds that have elapsed since midnight on January 1, 1970, UTC), which is the predominant base value for computer-recorded date and time values. 1508330494000 The large number that appears in our output for the current timestamp represents the same value as above, October 18th, 2017. Epoch time, also referred to as zero time, is represented by the date string 01 January, 1970 00:00:00 Universal Time (UTC), and by the 0 timestamp. We can test this in the browser by creating a new variable and assigning to it a new Date instance based on ... 10/4/2020 · How to Convert a String into a Date in JavaScript The best format for string parsing is the date ISO format with the JavaScript Date object constructor. But strings are sometimes parsed as UTC and sometimes as local time, which is based on browser vendor and version. It is recommended is to store dates as UTC and make computations as UTC.
The standard string representation of a date time string is a simplification of the ISO 8601 calendar date extended format. (See the section Date Time String Format in the ECMAScript specification for more details.). For example, "2011-10-10" (date-only form), "2011-10-10T14:48:00" (date-time form), or "2011-10-10T14:48:00.000+09:00" (date-time form with milliseconds and time zone) can be ...
How To Get Yesterday S Date Using Javascript
Learn How To Get Current Date Amp Time In Javascript
How To Get Format Date As Iso 8601 In Javascript Helloadmin
How To Set Input Type Date In Dd Mm Yyyy Format Using Html
Javascript Date Function Javascript Date Get And Set Methods
Javascript Dates Are A Day Off Stack Overflow
How To Format Dates In Javascript With Examples
Convert Date Objects Into Iso 8601 Utc Strings Isoformat
Javascript Date Format Conversion Stack Overflow
Javascript Extract Date From String Regex Extract Date
Demystifying Datetime Manipulation In Javascript Toptal
Convert String Into Date Using Javascript Geeksforgeeks
Display Updating Javascript Triggered In Format String Of
Javascript Date Formatting Net Style Waldek Mastykarz
Program To Convert String To Date In Java Codez Up
Javascript Date Formatting Net Style Waldek Mastykarz
Javascript Date Formatting Net Style Waldek Mastykarz
Everything You Need To Know About Date In Javascript Css Tricks
Date To String Format Javascript Code Example
Cannot Create Date Variable Using Javascript In Script Task
Sql Server Functions For Converting A String To A Date
Convert Date String To Date Javascript Code Example
Master Handling Date Time In E2e Fiori Scenario Handling
Javascript Convert Date Time String To Epoch Stack Overflow
Javascript Date And Time In Detail By Javascript Jeep
How To Manipulate Date And Time In Javascript
Changing The Date Format Knime Analytics Platform Knime
C Datetime Is Generated As String In Javascript
How To Implement Javascript Date Date Object Methods Edureka
Javascript Date Format Function Convert Datetime Like Php
Modified Java Script Value Hitachi Vantara Lumada And
0 Response to "32 Date String To Date Javascript"
Post a Comment