21 Javascript Append And Remove
In modern JavaScript, it makes no sense to load the complete jQuery library just to do some simple DOM manipulations. In this article, you'll learn how to add, remove, and toggle CSS classes in vanilla JavaScript without jQuery. Using className Property. The simplest way to get as well as set CSS classes in JavaScript is by using the className ... I think remove and add are the most used methods of the classList class, but here are all of them, with brief examples: remove() Removes a class from an element’s list of classes. If class does not exist in the element's list of classes, it will not throw an error or exception. add() Adds a class to an element’s …
How To Remove Text From A String In Javascript Geeksforgeeks
26/5/2011 · Now I need to remove the created div element if the label is again clicked. if (click == 1) { var prevWin = document.createElement("div"); prevWin.id = 1; prevWin.innerHTML = outMsg; document.body.appendChild(prevWin); } else { var prevWin = document.body.getElementsById(1); document.body.removeChild(prevWin); }
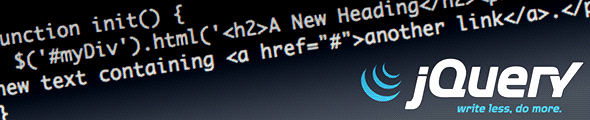
Javascript append and remove. In this video, you will learn how to add and remove class onclick in javascript. Also, you will learn how to check if class exists, toggle class and add or ... Below is some code to add and remove images to a page from a local file system. Tested in IE 6 and Firefox, modify for your purpose. If you are trying to add an image, then remove it within the one script, I suggest you split the add and remove bits to separate functions and call them from your main script. IE and other browsers The first parameter (2) defines the position where new elements should be added (spliced in). The second parameter (0) defines how many elements should be removed. The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. The splice () method returns an array with the deleted items:
If the given child is a reference to an existing node in the document, appendChild () moves it from its current position to the new position (there is no requirement to remove the node from its parent node before appending it to some other node). This means that a node can't be in two points of the document simultaneously. For that purpose, I decided to write this article about some of the best methods we can use to add and remove elements in arrays in Javascript. In this article we'll be covering push , pop , shift , unshift , concat , splice , slice and also on how to use the ES6 spread operator for javascript arrays. Dynamically Add/Remove Items From List JavaScript. by MemoryNotFound · November 4, 2016. This tutorial shows how to dynamically add and/or remove items from a list using JavaScript. create new element: We can dynamically create new elements using the document.createElement function. append element: We can append elements using the appendChild ...
There are two ways to append HTML code to a div through JavaScript. Using the innerHTML attribute. Using the insertAdjacentHTML () method. Using the innerHTML attribute: To append using the innerHTML attribute, first select the element (div) where you want to append the code. Then, add the code enclosed as strings using the += operator on ... This code creates a new <p> element: const para = document. createElement ( "p" ); To add text to the <p> element, you must create a text node first. This code creates a text node: const node = document. createTextNode ( "This is a new paragraph." ); Then you must append the text node to the <p> element: para. appendChild (node); Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Here is syntax for JavaScript Append method is as follows: document. getElementById ("div_name").innerText += "data" ; We can use jQuery too, for appending content at the end of the selected elements, by using following syntax : $ (selector).append (content , function (index.html)) Into the above syntax content is the required parameter ... JavaScript object operations - How to add / remove item from a JavaScript object Getting into JavaScript frameworks like vue.js and react.js, now it's time to review some of the JavaScript fundamentals. Introduction to JavaScript append () method. The parentNode.append () method inserts a set of Node objects or DOMString objects after the last child of a parent node: The append () method will insert DOMString objects as Text nodes. Note that a DOMString is a UTF-16 string that maps directly to a string. The append () method has no return value.
The delete() method removes a specified value from a Set object, if it is in the set. The modern approach to remove an element is to use the remove () method. Just call this method on the element you want to remove from the DOM, like below: const elem = document.querySelector('#hint'); elem.remove(); This method was introduced in ES6 and, at the moment, only works in modern browsers. However, you can use a polyfill to make it ... "add and remove javascript" Code Answer's. javascript delete element . javascript by Ankur on Apr 10 2020 Donate . 11 js delete element . javascript by Ariel Ferdman on Sep 10 2020 Donate . 1. Add a Grepper Answer . Javascript answers related to "add and remove javascript" ...
Using Splice to Remove Array Elements in JavaScript. The splice method can be used to add or remove elements from an array. The first argument specifies the location at which to begin adding or removing elements. The second argument specifies the number of elements to remove. Javascript: Add & Remove HTML Elements. Matt Morgante. July 12, 2017. In this post, I'm going to walk through the simplest possible example of how Javascript enables the creation and deletion of HTML elements. Using a list, we will access elements based on their tag name, add a new item to the list, and delete an item from the list. This ... Select the HTML element which need to remove. Use JavaScript remove() and removeChild() method to remove the element from the HTML document. Example 1: This example uses removeChild() method to remove the HTML element.
1) Add or Appending CSS Class Using Javascript. 1.1) CSS Class Using Javascript className method. 1.2) CSS Class Using Javascript classList method. 2) Browser Support. 3) Polyfill. In this tutorial, we will see the different methods for To Add and Remove CSS Class Using Javascript by taking the reference of the element ID. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Summary: in this tutorial, you will learn how to dynamically add options to and remove options from a select box in JavaScript. The HTMLSelectElement type represents the <select> element. It has the add() method that dynamically adds an option to the <select> element and the remove() method that removes an option from the <select> element:
Add a Class to an HTML Element Using className. The className property can be used to get or set the value of the class attribute of any DOM element. The returned string contains all the classes of current element separated by a space. We can simply use the += operator to append any new classes to our element.. JavaScript 9/8/2012 · Active Oldest Votes. 5. Specify window.close in your html handler: <a onclick="window.close ();">close<a>. http://jsfiddle /ZTwd7/1/. Otherwise close () refers to the native close method, which doesn't do anything. Here's how you would emulate it with a "javascript" (as opposed to inline html attribute) handler: Element.remove () is unscopable. The remove () method is not scoped into the with statement. See Symbol.unscopables for more information. with( node) { remove(); } Copy to Clipboard.
JavaScript suggests several methods to remove elements from existing Array. You can delete items from the end of an array using pop (), from the beginning using shift (), or from the middle using splice () functions. Definition and Usage. The remove() method is used to remove an option from a drop-down list. Tip: To add an option to a drop-down list, use the add() method. 29/4/2013 · When removing an element with JavaScript, you must go to its parent first instead. This was always odd and not so straightforward. According to DOM level 4 specs, which is the current version in development, there are some new handy mutation methods available: append() , prepend() , before() , after() , replace() , and remove() .
Attaching Event Handlers To Dynamically Created Javascript
Ds With Js Linked Lists Data Structures With Javascript
Call A Function With Parameters In Javascript Append Stack
Dynamically Append View With Mvc Partial View
Append Add And Remove Html Elements Jquery
Expressing An Algorithm Ap Csp Article Khan Academy
How To Dynamically Add Remove Table Rows Using Jquery
Dynamically Add Remove Table Rows Using Jquery Rowfy Free
Best Way To Add And Remove Css Class Using Javascript
Add Or Remove Input Fields Dynamically In Php Using Jquery
How To Remove Space In List Python Example Code
Python List Array Methods Remove Insert Pop Reverse
Add Remove Input Fields Dynamically Using Jquery And Bootstrap
Node Js How To Remove Or Delete Directory Fs Rmdir
Add Remove Input Fields Dynamically Using Jquery Demo
Removing Replacing And Moving Elements In Jquery
Add Remove Multiple Input Fields Dynamically With Jquery
Php Jquery Dynamic Textbox Phppot
Javascript Code In Bracket Software Stock Image Image Of
Javascript Array Splice Delete Insert And Replace
0 Response to "21 Javascript Append And Remove"
Post a Comment