20 How To Declare An Object In Javascript
However, the advantage of the literal or initializer notation is, that you are able to quickly create objects with properties inside the curly braces. You notate a list of key: value pairs delimited by commas.. The following code creates an object with three properties and the keys are "foo", "age" and "baz".The values of these keys are a string "bar", the number 42, and another object. Working with objects in JavaScript Summary. Objects are integral to javascript development and understanding how objects are generated and used is essential. JavaScript is designed on a simple object-based paradigm: An object represents a collection of properties; each property is an association between a name/value pair.
Angularjs Expressions Array Objects Eval Strings Examples
Jan 26, 2021 - Congratulations, you've reached the end of our first JS objects article — you should now have a good idea of how to work with objects in JavaScript — including creating your own simple objects. You should also appreciate that objects are very useful as structures for storing related data ...
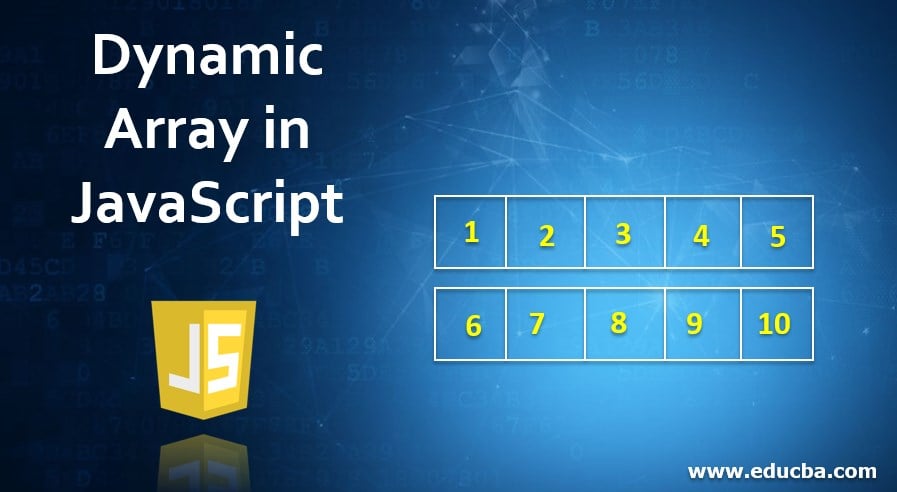
How to declare an object in javascript. 1. Creating objects using object literal syntax. This is really simple. All you have to do is throw your key value pairs separated by ':' inside a set of curly braces ( { }) and your object is ready to be served (or consumed), like below: This is the simplest and most popular way to create objects in JavaScript. 2. The Difference Between Array() and []¶ Using Array literal notation if you put a number in the square brackets it will return the number while using new Array() if you pass a number to the constructor, you will get an array of that length.. you call the Array() constructor with two or more arguments, the arguments will create the array elements. If you only invoke one argument, the argument ... Jul 23, 2020 - One of the easiest way to instantiate an object in JavaScript. Constructor is nothing but a function and with help of new keyword, constructor function allows to create multiple objects of same flavor as shown below: Output: Explanation: A class in OOPs have two major components, certain parameters and few member functions. In this method we declare ...
The returned value. The context this when the function is invoked. Named or an anonymous function. The variable that holds the function object. arguments object (or missing in an arrow function) This post teaches you six approaches to declare JavaScript functions: the syntax, examples and common pitfalls. The Date object is a built-in object in JavaScript that stores the date and time. It provides a number of built-in methods for formatting and managing that data. By default, a new Date instance without arguments provided creates an object corresponding to the current date and time. This will be created according to the current computer's ... JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined in the browser, you can define your own objects. This chapter describes how to use objects ...
How to Declare Constants in JavaScript? ES6 provides a new way of declaring a constant by using the const keyword which creates a read-only reference to a value: Watch a video course JavaScript - The Complete Guide (Beginner + Advanced) const CONSTANT_VALUE = "someValue"; This works in almost all browsers. When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ... Mar 09, 2018 - In this article we will learn multiple ways to create objects in JavaScript like Object Literal, Constructor Function, Object.Create method…
May 03, 2017 - Quality Weekly Reads About Technology Infiltrating Everything With JavaScript, you can define and create your own objects. There are different ways to create new objects: Create a single object, using an object literal. Create a single object, with the keyword new. Define an object constructor, and then create objects of the constructed type. Above, p1 and p2 are the names of objects. Objects can be declared same as variables using var or let keywords. The p1 object is created using the object literal syntax (a short form of creating objects) with a property named name.The p2 object is created by calling the Object() constructor function with the new keyword. The p2.name = "Steve"; attach a property name to p2 object with a string ...
Nov 22, 2020 - Note that all “for” constructs allow us to declare the looping variable inside the loop, like let key here. Also, we could use another variable name here instead of key. For instance, "for (let prop in obj)" is also widely used. ... Are objects ordered? In other words, if we loop over an ... Mar 19, 2011 - They do not allow scripts that use the contructor to use or modify the properties directly. They only allow the methods of the object itself to use and modify them. Unlike many other languages, JavaScript does not declare each variable type as 'public' or 'private'. It all depends on how you ... Using Object.create() method: var a = Object.create(null); This method creates a new object extending the prototype object passed as a parameter. Using the bracket's syntactig sugar: var b = {}; This is equivalent to Object.create(null) method, using a null prototype as an argument. Using a function constructor
Related: How to Declare Variables in JavaScript. Looking at the student class constructor above, you can discern that an object of this class will have three attributes. Creating an Object Example. Below, you'll see an example for creating an object in JavaScript. //create a new object const john = new Student('John', 'Brown', '2018'); TypeScript Type Template. Let's say you created an object literal in JavaScript as −. var person = { firstname:"Tom", lastname:"Hanks" }; In case you want to add some value to an object, JavaScript allows you to make the necessary modification. Suppose we need to add a function to the person object later this is the way you can do this. Sep 29, 2006 - Introduction JavaScript is a very flexible object-oriented language when it comes to syntax. In this article you can find three ways of defining and instantiating an object. Even if you have already picked your favorite way of doing it, it helps to know some alternatives in order to read other pe
Aug 20, 2020 - Remember that functions are independent units of behavior in JavaScript. They are not necessarily part of an object. When they are, we need to have a reference that allows the function to access other members on the same object. this is the function context. It gives access to other properties. May 07, 2015 - So far I saw three ways for creating an object in JavaScript. Which way is best for creating an object and why? I also saw that in all of these examples the keyword var is not used before a property — why? Is it not necessary to declare var before the name of a property as it mentioned that ... JavaScript Declare Empty Array Example Difference Between Two Ways to Declare Empty Array in JavaScript JavaScript has different ways to declare an empty array. One way is to declare the array with the square brackets, like below. var array1 = []; The other way is to use the constructor method by leaving the parameter empty.
While Object in Javascript can be almost anything, {} only points to javascript objects, for the test how it works, do below in your javascript code or console: var n = new Object(1); //Number {[[PrimitiveValue]]: 1} CodeProject, 20 Bay Street, 11th Floor Toronto, Ontario, Canada M5J 2N8 +1 (416) 849-8900 We hope you had fun writing your own real world random bouncing balls example, using various object and object-oriented techniques from throughout the module! This should have given you some useful practice in using objects, and good real world context.
Objects have keys and every key has a corresponding value. These values can be as simple as a number or string or maybe some complex types like function or even another object itself. How to declare an Object. We can declare an object using something called object literals (there are other ways too). A JavaScript object is a variable that can hold many different values. It acts as the container of a set of related values. For example, users of a website, payments in a bank account, or recipes in a cookbook could all be JavaScript objects. In JavaScript, objects can store two kinds of values: properties for static values; methods for dynamic ... In JavaScript we use var keyword to declare valuable. The variables declared as such have function scope. In ES6 however two block scope declarations let and const were introduced. The const is used to create constants and need to be initialized d...
Jul 29, 2020 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Create Javascript objects using the "new" keyword with the Object() constructor. Now, let's look at how declaring objects with the "new" keyword and the Object() constructor. It's as simple as specifying the "new" keyword followed by the Object() constructor. This would create a new, but empty (undefined) object for you to play ... Object methods, "this". Objects are usually created to represent entities of the real world, like users, orders and so on: let user = { name: "John", age: 30 }; And, in the real world, a user can act: select something from the shopping cart, login, logout etc. Actions are represented in JavaScript by functions in properties.
Set.prototype.values() - It returns all the values from the Set in the same insertion order. Syntax: set1.values(); Parameter: No parameters Returns: An iterator object that contains all the values of the set in the same order as they are inserted. Set.prototype.keys() - It also returns all the values from the Set in the insertion order. Note: - It is similar to the values() in case of Sets Mar 24, 2020 - by Kaashan Hussain We all deal with objects in one way or another while writing code in a programming language. In JavaScript, objects provide a way for us to store, manipulate, and send data over the network. There are many ways in which objects in JavaScript differ from objects in In this JavaScript tutorial you will learn about JavaScript Object Oriented Programming, which is a much more efficient way of creating JavaScript code. We w...
The enum in JavaScript is used to define set of same type constants in single entity. As it is used for constants, JavaScript used "const" keyword for achieving enum feature. As we know in standard programming convention constants are declared with all capital letters. This enum can be used for week days like {"SUNDAY, MONDAY, TUESDAY ... How to declare a variable in JavaScript? Similar to other programming languages, creating a variable is called as "Declaring" a variable in JavaScript. In addition to this, the declaration of the variable happens with the help of the "var" keyword. Example. var test; where the test is the name of the declared variable. The reserved words cannot be used as JavaScript variables, functions, methods, loop labels, or any object names. Here is a list of such reserved words in JavaScript: abstract
How To Declare A Watch On The This Object In A Factory Method
Dynamic Array In Javascript Using An Array Literal And
Javascript Array Of Objects Tutorial How To Create Update
Recap Creating Objects In Javascript Eat Sleep Code
Object Destructuring In Javascript
Javascript Const Geeksforgeeks
Tools Qa What Are Javascript Objects And Their Useful
6 Ways To Create An Object In Javascript
Javascript Object Create How To Create Object In Js
Javascript Create Object How To Define Objects In Js
Javascript Objects And Functions
3 Ways To Clone Objects In Javascript Samanthaming Com
How To Create Object In Javascript Using New Operator
Angularjs Expressions Array Objects Eval Strings Examples
Learn How To Get Current Date Amp Time In Javascript
The Flavors Of Object Oriented Programming In Javascript
2 Classes Object Oriented Programming In Javascript Es6
0 Response to "20 How To Declare An Object In Javascript"
Post a Comment