29 What Is Enum Javascript
Although enum is a reserved word in JavaScript, JavaScript has no support for traditional enums. However, it is fairly easy to define enums using objects in JavaScript.For example, TypeScript has support for enums: enum Direction { Up, Down, Left, Right } At runtime, TypeScript compiles the above code into the below enum-like object: An enum is perfect for this task. An enum in Swift looks like this: enum LoadingState { case pending case success case failure } The same in TypeScript: enum LoadingState { pending, success, failure } The syntax here is very similar and pretty straightforward. LoadingState can be one of these 3 member values.
Using Enums Enumerations In Javascript
May 24, 2020 - In creating advanced Enum types ... and advanced features. The next logical step would be to form a parallel pattern for enums in Javascript — for cases where a Javascript environment is preferred or required....
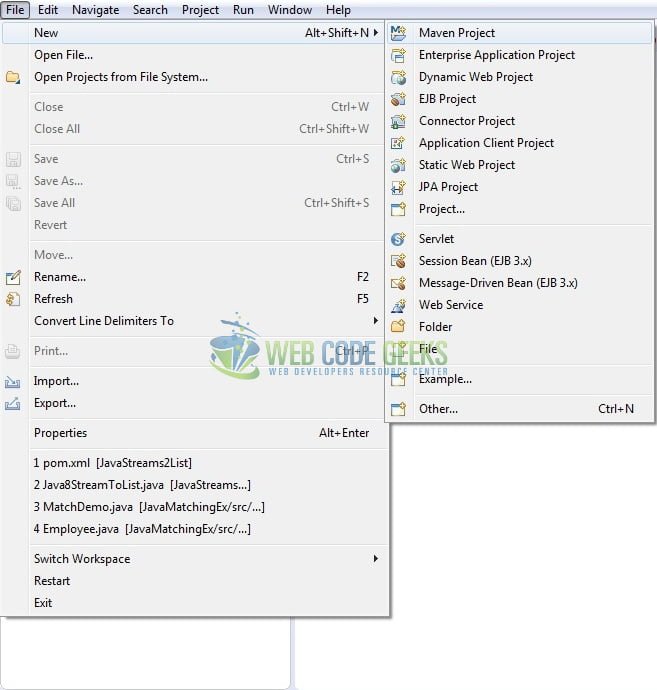
What is enum javascript. JavaScript, however, does not have the enum data type, but they are now, fortunately, available in TypeScript since version 2.4. Enums allow us to define or declare a collection of related values that can be numbers or strings as a set of named constants. Unlike some of the types available in TypeScript, enums are preprocessed and are not ... Nov 30, 2017 - For this minification, Closure Compiler is the best I have yet to find. Online access can be found here. Closure compiler is able to take all of this enumeration data and inline it, making your Javascript be super duper small and run super duper fast. Thus, Minify with Closure Compiler. Observe. There is a very simple and obvious solution for a enum variable type in Javascript, a object. Let’s assume we have three values which represent the state of a client in our fictional chat example. ... Not every user can kick others out of the chat, so if a user sends the kick command we have to verify if he is ...
JavaScript doesn't have true enums. Most workarounds to this problem involve using a map where the keys represent the enum constants, and the values are integers or string-representations of the enum constants. Enums are not supported in JavaScript natively. We can however create Enums using Object.freeze by creating objects containing all the enumerable properties and then freezing the object so that no new enum can be added to it. Example Nov 16, 2019 - Disclaimer, we aren’t referring to strict enumeration seen in strongly typed languages. This isn’t strictly possible with JavaScript. We look at the next best thing we have in JavaScript without jumping into TypeScript. If you’ve used Redux or Flux you’ve likely come across this form ...
Using enums (enumerations) in javascript 📃 August 21, 2017. There are lots of times where enums are useful, and sometimes even necessary. Although javascript does not natively support enums, there are a lot of options when it comes to implementing enums using javascript. The naive way of implementing "enums" In reality Javascript does not support enumerated types, but what you can do is define a global constant object that looks and behaves like an Enum. We look ... TypeScript Data Type - Enum. Enums or enumerations are a new data type supported in TypeScript. Most object-oriented languages like Java and C# use enums. This is now available in TypeScript too. In simple words, enums allow us to declare a set of named constants i.e. a collection of related values that can be numeric or string values.
Enumeration is a user defined datatype in C language. It is used to assign names to the integral constants which makes a program easy to read and maintain. The keyword "enum" is used to declare an enumeration. The enum keyword is also used to define the variables of enum type. JavaScript enums are used to define multiple constants in a single entity. Const keyword is used for enums in JavaScript. This enum constants are always in capital letter only, this is not mandatory but standard convention in programming. Recommended Articles. This is a guide to JavaScript Enum. Using Enums in pure JavaScript. Oleh Baranovskyi. Mar 8, 2018 · 4 min read. Enum(or Enumerated type) is a special type used to define collections of constants. To give an illustration of what means Enum, we should have a look on Java example first:
Enums. An enum is a special "class" that represents a group of constants (unchangeable variables, like final variables).. To create an enum, use the enum keyword (instead of class or interface), and separate the constants with a comma. Note that they should be in uppercase letters: Enumeration is a user defined datatype in C language. It is used to assign names to the integral constants which makes a program easy to read and maintain. The keyword "enum" is used to declare an enumeration. The enum keyword is also used to define the variables of enum type. Enums in javascript - Simply answer for this problem we doesn't involved indeep what the values are like 0,1,2.. We want only correct output values
Enumeration (enumerated types) allows you to define constants for use within your code. In computer programming, an enumerated type (also called enumeration, enum, or factor in the R programming language, and a categorical variable in statistics) is a data type consisting of a set of named values called elements, members, enumeral, or ... In this blog post, I present enumify, a library for implementing enums in JavaScript. The approach it takes is inspired by Java's enums. Enum patterns ... Enum is a new datatype introduced in Javascript/typescript which holds strings or numbers only. Some times It is necessary to check whether declared string or number exists in a Enum object. This blog post covers checking string or number value exists in Enum in javascript or typescript. You can check my other posts on typescript Enum object
Aug 03, 2018 - Enum(or Enumerated type) is a special type used to define collections of constants. The last guide on enums you will read! JavaScript is aware of 7 data types. Naming them: number, string, boolean, object, function, symbol and undefined. If you take it seriously, you can count ... Java Enum is a data type which contains fixed set of constants. It can be used for days of the week (SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY and SATURDAY) , directions (NORTH, SOUTH, EAST and WEST) etc. The enum constants are static and final implicitely. It is available from Java 5. Enums can be thought of as classes that have fixed set of constants.
Dec 11, 2019 - In one of my project I need some enums defined in C# to able also for JavaScript code. After some quick research in internet I came out with simple and easy extension method to convert C# enums to JavaScript. Here it is. Let’s get started with example enum and suppose we want to be able to ... JavaScript is weakly typed, variables are not declared with a type beforehand and it does not have a native enum data type. Examples provided here may include different ways to simulate enumerators, alternatives and possible trade-offs. Enum Types. An enum type is a special data type that enables for a variable to be a set of predefined constants. The variable must be equal to one of the values that have been predefined for it. Common examples include compass directions (values of NORTH, SOUTH, EAST, and WEST) and the days of the week. Because they are constants, the names of ...
Enums to the Rescue. An enum is a user-defined type consisting of a set of named constants called enumerators. The colors of the rainbow would be mapped like this.: Now internally, the compiler will use an int to hold these and if no values are supplied, red will be 0, orange is 1 etc. Enums are used to represent a number of fixed values that can be evaluated. Unfortunately, there is no native JavaScript function for enums though there are ways we can define a set of enumerable values that will remain useable throughout a program. Two enum values Jan 14, 2020 - An enum is a type that consists of a set of values. For example, TypeScript has built-in enums and with them, we can define our own boolean type: ... This TypeScript code is compiled to the following JavaScript code (a few details are omitted, to make things easier to understand):
C# Enums. An enum is a special "class" that represents a group of constants (unchangeable/read-only variables). To create an enum, use the enum keyword (instead of class or interface), and separate the enum items with a comma: Example. enum Level { Low, Medium, High } You can access enum items with the dot syntax: Maybe if someone has studied java, but javascript is not java, and this is a javascript forum, so to expect people that post here to know what an Enumeration is is unrealistic. But what JavaScript lacks in syntactic sugar it makes up for in terms of flexibility. The easiest way to define an enum would be to use Object.freeze () in combination with a plain object. This will ensure that the enum object cannot be mutated. const daysEnum = Object.freeze({ monday: 0, tuesday: 1, wednesday: 2, thursday: 3, friday: 4 ...
Enums. Enums are one of the few features TypeScript has which is not a type-level extension of JavaScript. Enums allow a developer to define a set of named constants. Using enums can make it easier to document intent, or create a set of distinct cases. TypeScript provides both numeric and string-based enums. An introduction to using enum’s in JavaScript and TypeScript This article is an introduction to enumerated types or enum’s in both JavaScript and TypeScript. Unlike TypeScript, JavaScript doesn’t directly support enumerations. So we will look at a way to get some of the benefits of enumerated ... Including properties in the enum avoids having to write switch statements (and possibly forgetting new cases to the switch statements when an enum is extended). The example also shows the enum properties and types documented with the JSDoc enum annotation.
Aug 21, 2017 - An interesting fact, is that we can also use the original seasons.SUMMER enum value as well. Since object values are passed by reference, any two variables referencing seasons.SUMMER will be equal. ... The reasons for using enums in javascript are the same as the reasons you should use it for ... Background information. The format described above is the result of quite some time of thinking about enums in Javascript. It tries to combine the best of both worlds of using primitives as the enum values (safe for de/serializing) and using objects as the values (allows properties on the values).
Enum In Java Learn The Basics Of Enumeration With Examples
Enums In C With Real Time Examples Dot Net Tutorials
What Is C Enum And How To Use Enums In C
C Converting String To Enum Or Enum To String In C Qa
What Is C Enum And How To Use Enums In C
Working With Enumerations Enums In Typescript By Uday
Define Enum In Javascript Code Example
What Do Need Know About Enumerations In Typescript By
Working With Enumerations Enums In Typescript By Uday
Javascript Enums Example Web Code Geeks 2021
Make String Enum Index Object Typescript Code Example
Dealing With Enums In Vue Js Dev Community
How To Use Enums In Typescript Digitalocean
How To Use Enum With Entity Framework 5 Codeproject
Javascript Enums Example Web Code Geeks 2021
Typescript Enum Guide Get Started In 5 Minutes
Feature Request Add Preserveconstenums Option To Transform
Javascript Enums Example Web Code Geeks 2021
Javascript Enums Example Web Code Geeks 2021
Typescript A Developer Friendly Javascript
Fundamentals Of Java Enum Types Sitepoint
Github Vivin Enumjs Type Safe Enums In Javascript
Creating Enum Types In Javascript
How Can I Guarantee That My Enums Definition Doesn T Change
Why Typescript Enums Suck Logrocket Blog
0 Response to "29 What Is Enum Javascript"
Post a Comment