28 Dom Manipulation Javascript Tutorial
DOM manipulation: considered useful. ... and nesting them inside existing elements on the page — all via JavaScript. ... A good starting place is our Manipulating documents tutorial. Functions 1. For the first task, you have to create a simple function — chooseName() ... In this JavaScript DOM Tutorial, we will get familiar with the Document Object Model of a webpage and learn how to manipulate the DOM by creating a basic web application. JavaScript is absolutely essential for web development, and in case you want to choose web development as your career you'd surely come across this language.
Dom In Javascript With Examples Document Object Model
The Document Object Model, usually referred to as the DOM, is an essential part of making websites interactive. It is an interface that allows a programming language to manipulate the content, structure, and style of a website. JavaScript is the client-side scripting language that connects to the DOM in an internet browser.
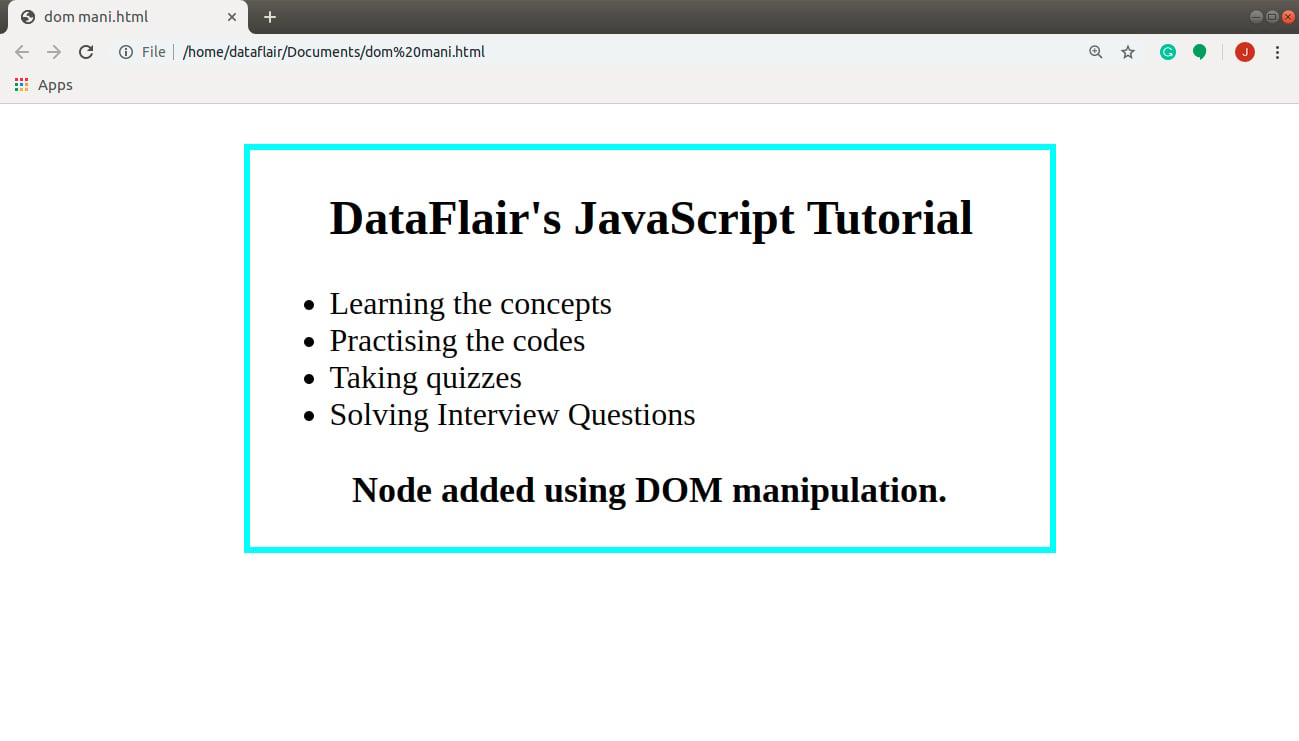
Dom manipulation javascript tutorial. The ability to manipulate the DOM is one of the most unique and useful abilities of JavaScript. The image below gives a visual representation of what the DOM tree looks like. Here we have the document object. This is the core/foundation of the DOM. To perform any form of DOM manipulation, you have to access the document object first. In this article, We understood about JavaScript Fundamental Concept DOM Manipulation. We conclude that : The document loaded in a browser is represented by the document object model (DOM). Moreover, it is a data representation of the objects in the HTML page. Also, the ID, Tag Name, or Class Name of the HTML elements can access the DOM elements. Sebastian Seitz gives you a crash course in DOM manipulation with vanilla JavaScript, abstracting the more verbose parts into a set of helper functions.
Tutorial 2: Manipulating the DOM. This tutorial will teach you how to manipulate the DOM from JavaScript. Knowledge of how to access the DOM from JavaScript is assumed. There are five short lessons, followed by one exercise. It is highly recommended that you open your browser's JavaScript console now. Document Object Model (DOM) The Document Object Model (DOM) connects web pages to scripts or programming languages by representing the structure of a document—such as the HTML representing a web page—in memory. Usually it refers to JavaScript, even though modeling HTML, SVG, or XML documents as objects are not part of the core JavaScript ... 10/8/2021 · Syntax: – var cell = HTMLTableRowElement.insertCell (index = -1); HTMLTableRowElement is a reference to an HTML table row element. index is the cell index of the new cell. cell, is assigned a reference to the new cell. If index is -1 or equal to the number of cells, the cell is …
JavaScript DOM Manipulation. In this tutorial you will learn how to manipulate elements in JavaScript. Manipulating DOM Elements in JavaScript. Now that you've learnt how to select and style HTML DOM elements. In this chapter we will learn how to add or remove DOM elements dynamically, get their contents, and so on. Adding New Elements to DOM JavaScript allows you to select elements from the DOM of a website and then make changes to those elements.This video covers getElementById, getElementsByCla... Donate via PayPal - https://www.paypal.me/thenetninjaDonate via Patreon - https://www.patreon /thenetninjaHey gang, welcome to your very first JavaScript ...
30/1/2019 · Check out my courses and become more creative!https://developedbyed Javascript Dom Manipulation | Javascript Tutorial For BeginnersIn this video series we... Manipulating documents. When writing web pages and apps, one of the most common things you'll want to do is manipulate the document structure in some way. This is usually done by using the Document Object Model (DOM), a set of APIs for controlling HTML and styling information that makes heavy use of the Document object. Hi, in this tutorial you’ll learn what DOM is, what selectors are and how you can use them to manipulate it using Javascript. What is the DOM? DOM, also known as Document Object Model, is a javascript object containing all the information about a webpage that is …
The DOM Programming Interface. The HTML DOM can be accessed with JavaScript (and with other programming languages). In the DOM, all HTML elements are defined as objects. The programming interface is the properties and methods of each object. A property is a value that you can get or set (like changing the content of an HTML element). All of the properties, methods, and events available for manipulating and creating web pages are organized into objects. For example, the document object that represents the document itself, any table objects that implement the HTMLTableElement DOM interface for accessing HTML tables, and so forth, are all objects.. The DOM is built using multiple APIs that work together. Section 4. Manipulating elements. createElement() - create a new element. appendChild() - append a node to a list of child nodes of a specified parent node. textContent - get and set the text content of a node.; innerHTML - get and set the HTML content of an element.; innerHTML vs. createElement - explain the differences beetween innerHTML and createElement when it comes to creating ...
This crash course focuses on the DOM WITHOUT JQUERY. In this part we will talk about what the JavaScript DOM (Document Object Model) is and we will look at t... 7/7/2021 · With JavaScript, we can easily manipulate the DOM to bring our web pages to life. This tutorial will focus on adding an element to the DOM, removing element from the DOM with JavaScript. By the end of this tutorial, you will be equipped with the tools needed to interact with the DOM using JavaScript. Prerequisites jQuery vs JavaScript. jQuery was created in 2006 by John Resig. It was designed to handle Browser Incompatibilities and to simplify HTML DOM Manipulation, Event Handling, Animations, and Ajax. For more than 10 years, jQuery has been the most popular JavaScript library in the world. However, after JavaScript Version 5 (2009), most of the jQuery ...
This tutorial shows you how to create a DOM element and attach it to the DOM tree. To create a DOM element, ... Manipulate Elements: splice() Sort Elements: sort() ... The JavaScript Tutorial website helps you learn JavaScript programming from scratch quickly and effectively. This code creates a new <p> element: const para = document. createElement ( "p" ); To add text to the <p> element, you must create a text node first. This code creates a text node: const node = document. createTextNode ( "This is a new paragraph." ); Then you must append the text node to the <p> element: para. appendChild (node); JavaScript DOM Events Example. JavaScript code can be executed when any changes happen in DOM or any event occurs, let's understand following scenario. When a web page has loaded completely. When the mouse moves over an element. When a user clicks the mouse. When any value changes in input field or dropdown list.
27/6/2018 · Introduction to DOM manipulation. Manipulation of DOM is one of the most important aspects of jQuery and thereby JavaScript. DOM stands for Document Object Model. It is a manipulation of interacting and representing with XML, HTML or XHTML documents. Using DOM, you can manipulate and navigate your documents through a programming language. The Document Object Model (DOM) is the data representation of the objects that comprise the structure and content of a document on the web. This is what we use when we work with Javascript and interact in any way dynamically with the document. If you use Angular, Vue, React, or any other library or framework to create modern web applications ... 🚨 IMPORTANT:JavaScript Simplified Course: https://javascriptsimplified DOM manipulation is tough. There are lots of methods and techniques you need to ma...
JavaScript DOM Tutorials. These interactive tutorials will teach you how to access and manipulate the DOM from JavaScript. No libraries are used. Basic knowledge of JavaScript is assumed. Tutorial 1: Accessing the DOM Use getElementById, then walk the DOM to find any node. Tutorial 2: Manipulating the DOM Create and delete nodes. Tutorial 3 ... The HTML DOM is a standard object model and programming interface for HTML. It defines: The HTML elements as objects. The properties of all HTML elements. The methods to access all HTML elements. The events for all HTML elements. In other words: The HTML DOM is a standard for how to get, change, add, or delete HTML elements. 12/3/2019 · In this tutorial, we will learn how to manipulate the DOM with vanilla JavaScript. With no further ado, let’s jump right into it. 1. First things first. Before we dive into coding, let’s learn what the Dom really is: The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the page so that ...
Advanced Functions. Passing By Value. Returning Multiple Values. Function Type. The call () Method. The apply () method. The bind () Method. Recursive Functions. Closures. DOM Manipulation Methods in jQuery. jQuery provides various methods to add, edit or delete DOM element (s) in the HTML page. The following table lists some important methods to add/remove new DOM elements. Method. Description. append () Inserts content to the end of element (s) which is specified by a selector.
All You Need To Know About Dom In Javascript Edureka
Javascript Dom Element Manipulation Events Examples
Manipulating Documents Learn Web Development Mdn
Dhtml Javascript Geeksforgeeks
A Vanilla Js Guide On Mastering The Dom Dev Community
How To Understand And Modify The Dom In Javascript Digitalocean
What Is Dom Javascript Document Object Model Manipulation
Javascript Dom Manipulation Blog Codecoda
Manipulating Dom In Javascript For Beginners Dev Community
Github Khristos Netninja Javascript Dom Tutorial Original
Dom Manipulation With Javascript Engineering Education
Develop A To Do List App In Vanilla Javascript By Carlos Da
Tools Qa What Is Dom In Javascript How To Access Dom
Javascript Dom Document Object Model Guide For Novice
Tooljs Dom Manipulation Using Tooljs
Html Attribute Vs Dom Property Dot Net Tutorials
What Is Document Object Model Dom How Js Interacts With
Javascript Dom Tutorial What Is Dom In Javascript Javascript Tutorial For Beginners Simplilearn
Javascript Dom Document Object Model Guide For Novice
Javascript Lesson 34 Dom Manipulating Attributes In
How To Understand And Modify The Dom In Javascript Digitalocean
Javascript Dom Document Object Model Guide For Novice
Javascript Dom Nodes Tutorial Republic
An Introduction To The Javascript Dom
Beginner S D3 Js Tutorial Learn Data Visualization With Js
Javascript Tutorial An Ultimate Guide For Beginners
Best Resources That Teach Dom Manipulation Using Only
0 Response to "28 Dom Manipulation Javascript Tutorial"
Post a Comment