22 Create Array Of Json Objects In Javascript
In this section we specify array's main charasteristics and restrictions that may apply to them using a single JSON Schema document. First of all, we want to ensure that the document we are validating is an array using the type restriction. This is the basic array schema: { " type ": "array" } This schema specifyies arrays and no other JSON ... I needed to send a JSON formatted text as the body of an http POST request. For clarification, The JSON body holds data from Maximo objects which will be sent to the external system to create associated records there. For single objects we use JSONObject alone which is an easy task. You can ...
How To Work With Json In Javascript Digitalocean
Converting a JSON Text to a JavaScript Object. A common use of JSON is to read data from a web server, and display the data in a web page. For simplicity, this can be demonstrated using a string as input. First, create a JavaScript string containing JSON syntax:
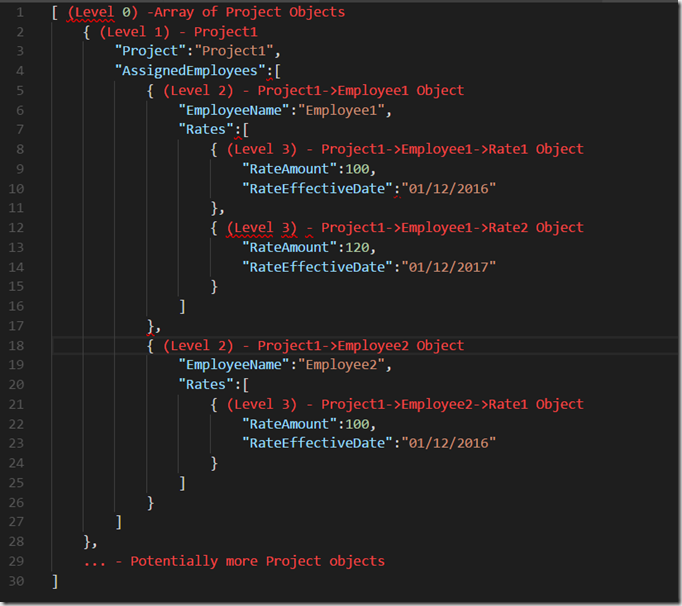
Create array of json objects in javascript. iterate json object array in javascript; how to update object in javascript; copy an object with object.assign.keys() array; create object javascript; js rename property; javascript extract array from object; javascript sort array of objects multiple fields; js create new object; javascript extend object; search array for property js; deep copy js May 28, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Jun 27, 2019 - Answer: It creates a new array with the results of calling a function on every element in the calling array. Let us solve a problem using map method. We have a JSON object say “orders” with lot of…
17/2/2021 · The first line creates an array of the property keys from the first object in the array (in this case, ["name", "age"]), and the second line maps through our JSON array and returns an Object using the keys array as the order in which the properties are added. To add more rows to the table, add more objects to items, making sure that each object has an id different from the existing objects in the array. Conclusion In this guide, you've created a component that has no state but populates a list of JavaScript objects often derived from a JSON object. 22/10/2020 · From JSON object to an array in JavaScript. Javascript Web Development Front End Technology. We are required to create an array out of a JavaScript object, containing the values of all of the object's properties. For example, given this object −. { "firstName": "John", "lastName": "Smith", "isAlive": "true", "a }
Nov 21, 2020 - We are required to write a JavaScript function that takes in an object like this − · The function should then map the "rssi" property of all the nested objects to a corresponding nested array of arrays. First, the json is to read the user data from the web servers and displayed the data in the web page first is converted into the string format using JSON.stringify () and next is again converted string into arrays using JSON.parse () method.This method parses the JSON string and returns the value for javascript is equal for whatever the user ... JSON stands for JavaScript Object Notation. It's a light format for storing and transferring data from one place to another. So in looping, it is one of the most commonly used techniques for transporting data that is the array format or in attribute values.
how to display this type of array of json having sub array of json in the form of table in javascript ... Error during serialization or deserialization using the JSON JavaScriptSerializer. The length of the string exceeds the value set on the maxJsonLength property. ... The "chunk" argument must be one of type string or Buffer. Received type object ... The most simplest and commonly used method among developers is by using JSON methods, which is apparently the slowest method of all. I tested out few of the commonly used methods to clone and copy arrays and objects and below are the results with a sample size of an array with 10000 objects of 1 key-value pair each. Introduction to JavaScript Array Array.from () method In ES5, to create an array from an array-like object, you iterate over all elements and add each of them to an intermediate array as shown in the following example:
May 24, 2016 - When jq returns just one JSON object, the ‘Compact Output’ option will produce a one-line result. If you want to access just the first (or the n-th) item in an array, put a digit in the [] operator: ... IMPORTANT: you access the first element of an array with 0, not 1. This is because JavaScript... Convert Array to JSON Object JavaScript You can use JSON.stringify to convert an array into a JSON formatted string in JavaScript. Suppose there is an array such as " [1, 2, 3, 4]". If you want to convert this array to JSON Object in javascript. 2 weeks ago - JSON array can store string, number, boolean, object or other array inside JSON array. In JSON array, values must be separated by comma. Arrays in JSON are almost the same as arrays in JavaScript.
20/4/2020 · So, how can you add an array element into a JSON Object in JavaScript? This is done by using the JavaScript native methods .parse()and .stringify() We want to do this following: Parse the JSON object to create a native JavaScript Object; Push new array element into the object using .push() Use stringify() to convert it back to its original format. In the previous section of JavaScript-JSON tutorial you have known that how to create an object and now in this tutorial we have provided you the way array of objects are declared in JavaScript-JSON. In our example file we have created an object "students" which contains two array objects " Maths " and " Science " containing name, marks and age of two students. function Restaurant(location, city_state, phone, distance) { this.location = location; this.city_state = city_state; this.phone = phone; // here you can add some logic for the distance field, if you like: this.distance = distance; } // create an array restaurants var restaurants = []; // add objects to the array restaurants.push(new Restaurant("123 Road Dr", "MyCity ST", "555-555-5555", 0)); …
The JSON functions are particularly useful for working with data structures in Javascript. They can be used to transform objects and arrays to strings. JSON.parse() can be used to convert JSON data to a Javascript Object or Array: var obj = JSON.parse(data); obj.notes.push(addition); var json = JSON.stringify(obj, null, 2); In the following code, we declare a variable named obj that parses the data from the code.json file. We then "push" the object addition into the notes array. Next, in the same above code, we stringify i.e. create an object into a JSON string. Now ... Jul 11, 2016 - In this article, we’ll discuss all about JavaScript JSON Array. We’ll see how to create a JSON object in JavaScript and converting it to JSON string.
Using Object.fromEntries() In ES2019+, you can achieve that by using the Object.fromEntries() method in the following way: // ES2019+ const obj = Object.fromEntries(pairs); console.log(obj); // {foo: 'bar', baz: 'qux'} Using a for Loop. To create an object from an array of key/value pairs, you can simply use a for loop in the following way: JS Examples JS HTML DOM JS HTML Input JS HTML Objects JS HTML Events JS Browser JS Editor JS Exercises JS Quiz JS Certificate ... Arrays in JSON are almost the same as arrays in JavaScript. The "chunk" argument must be one of type string or Buffer. Received type object nodejs ... DataTables warning: table id=example-dt - Invalid JSON response. ... Cannot deserialize the current JSON array (e.g. [1,2,3]) into type
33 Javascript Create Array Of Json Objects. Written By Leah J Stevenson Tuesday, August 10, 2021 Add Comment. Edit. 14/5/2020 · Add a new object at the end - Array.push. To add an object at the last position, use Array.push. let car = { "color": "red", "type": "cabrio", "registration": new Date('2016-05-02'), "capacity": 2 } cars.push(car); Add a new object in the middle - Array.splice. To add an object in the middle, use Array.splice. This function is very handy as it can also remove items. Watch out for its parameters: … Morioh is the place to create a Great Personal Brand, connect with Developers around the World and Grow your Career!
Arrays in JSON are almost the same as arrays in JavaScript. In JSON, array values must be of type string, number, object, array, boolean or null . In JavaScript, array values can be all of the above, plus any other valid JavaScript expression, including functions, dates, and undefined. Apr 26, 2021 - As described above, JSON is a string whose format very much resembles JavaScript object literal format. You can include the same basic data types inside JSON as you can in a standard JavaScript object — strings, numbers, arrays, booleans, and other object literals. This allows you to construct ... Using ES6 Example ES6 introduced Spread Operator and Object assign method to process array.. First called array map() method is call a function for every element during iteration and return a new array for each element.; This new array contains index=0 as id: "1" and index=1 as name: "kiran" Create an Object with this values in map chain to return new array Next step deep copy the ...
How to form JavaScript object array? 1. How to create JSON array..? 3. ... Create JavaScript array (JSON format) for DataTables aoColumnDefs. 0. unable to concatenate my Int ObjectId with my Byte[] timestamp inside my Razor view. See more linked questions. Related. 4665. How to create a GUID / UUID. For example if mention the array size as topping[10] it is suppose to create a payload of 10 objects and push those 10 objects of similar type inside the array topping ** Is it possible to dynamically create json objects and post the request in postman?? Kind note : The size of the array should be parameterized. Please let me know. Get code examples like"javascript create json object from array". Write more code and save time using our ready-made code examples.
Jul 30, 2021 - Given a JSON string and the task is to convert the JSON string to the array of JSON objects. This array contains the values of JavaScript object obtained from the JSON string with the help of JavaScript. There are two approaches to solve this problem which are discussed below: Jan 24, 2019 - i am not able to array of json object. data population is missing and returning as empty. ... I see you have the expected response, can you also share the response you get that you are trying to change. What are you getting in the response.content ? ... How can I use the JavaScript policy to ... In this tutorial, you'll learn how to create JSON Array dynamically using JavaScript. This is one of most common scenarios and you'll see two ways of creating JSON array dynamically. The first method will use for loop for creating JSON array from an input array.
Oct 30, 2020 - Get code examples like "create json array in javascript" instantly right from your google search results with the Grepper Chrome Extension. Transforming JSON Data in JavaScript. The JSON format is syntactically similar to the way we create JavaScript objects. Therefore, it is easier to convert JSON data into JavaScript native objects. JavaScript built-in JSON object provides two important methods for encoding and decoding JSON data: parse() and stringify(). JSON Arrays, Arrays as JSON Objects In JSON, array values must be of type string, number, object, array, boolean or null. In JavaScript Nested Arrays in JSON Objects. In the query below, the JSON_VALUE functions extract at the ‘higher’ array – the Customer (‘Customer.Id’ and ...
Let's look at another use case where you have to create a JSON object dynamically from an array and push to another array. Now let's try to open Json Object using IE or any other javaScript enabled browser. It produces the following result −. Creating Array Objects. The following example shows creation of an array object in javascript using JSON, save the below code as json_array_object.htm −
Json Concepts And Article Structure Apple Developer
Convert Array To Json Object Javascript Tuts Make
Working With Json In Swift With Swiftyjson Learnappmaking
How To Print Json Object Or Convert It To An Array Ionic V3
How To Parse Custom Json Data Using Excel The Excel Club
How To Set Multiple Json Data To A One Time Request Using
Jetbrains Kotlin Tip Need To Create A Json Object From
Json Format Example Json Table Function Ibm Developer
Importing Complex Json Files Using Ssis
Jquery Convert Json String To Array Sitepoint
Converting Json With Nested Arrays Into Csv In Azure Logic
Json Generated By Javascript Code Returns Arrays As Objects
The Json Query Function To Extract Objects From Json Data
How To Access Nested Json Object With Nested Ng Repeat In
Vue Js Accessing Complex Nested Json Objects Pakainfo
Create Json Array With Object Name Android Stack Overflow
3 Ways To Convert Datatable To Json String In Asp Net C
Json Tutorial Learn How To Use Json With Javascript
Tutorial Query A Database And Write The Data To Json
Json Handling With Php How To Encode Write Parse Decode
0 Response to "22 Create Array Of Json Objects In Javascript"
Post a Comment