29 Javascript Copy Of Object
Using the spread syntax or Object.assign () is a standard way of copying an object in JavaScript. Both methdologies can be equivalently used to copy the enumerable properties of an object to another object, with the spread syntax being the shorter of the two. Using the spread syntax or Object. assign () is a standard way of copying an object in JavaScript. Both methodologies can be equivalently used to copy/merge the enumerable properties of an object to another object. Problem with these two approaches is that it will just do the shallow copy. A shallow copy is a bit-wise copy of an object.
3/1/2020 · Summary: in this tutorial, you will learn how to copy objects in JavaScript, including shallow copy and deep copy. To copy an object in JavaScript, you have three options: Use the spread (...) syntax; Use the Object.assign() method; Use the JSON.stringify() and JSON.parse() methods; The following illustrates how to copy an object using three methods above:
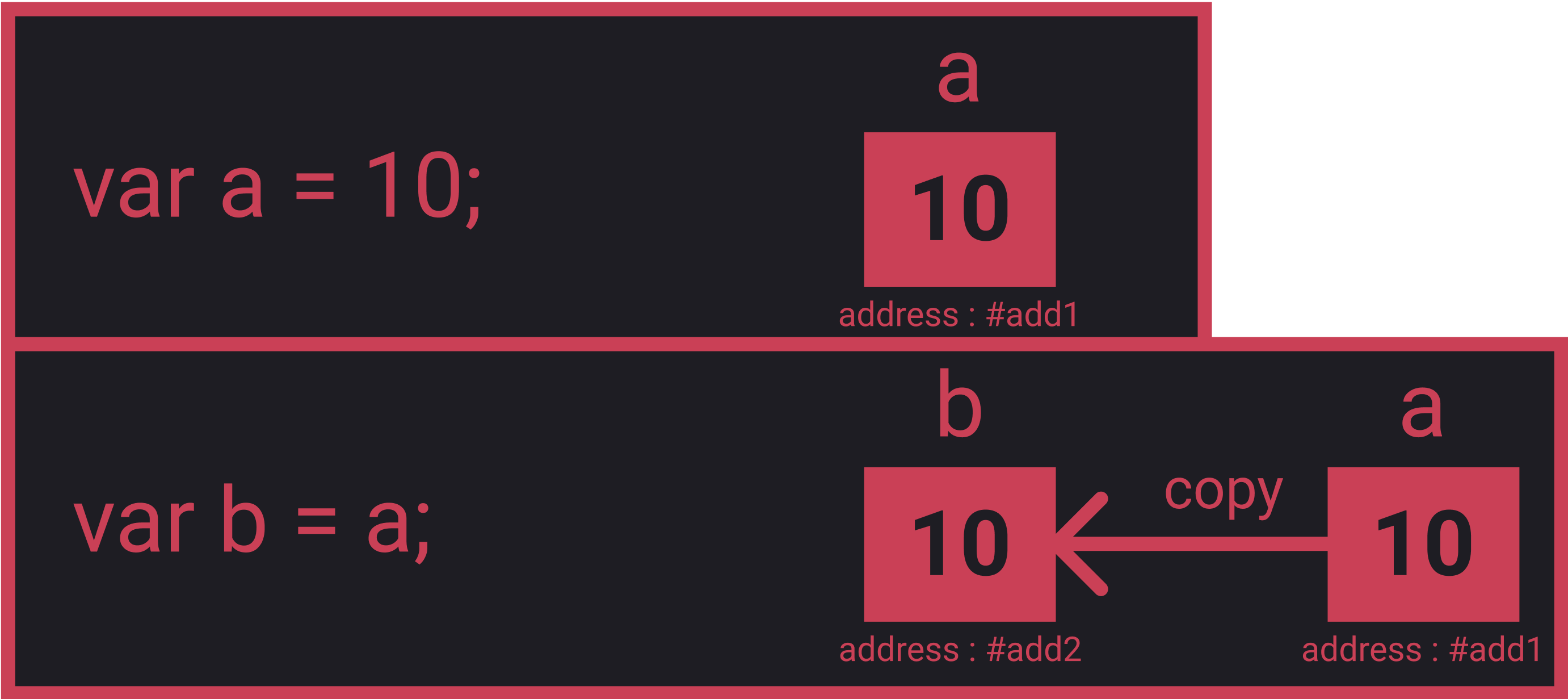
Javascript copy of object. Here's how to create a copy of an object in JavaScript, but without certain properties. Let's say I want to make a copy of this statueObj object below, BUT I don't want the company property in my new object. const statueObj = { city: "Sydney", height: 238, material: "bronze", company: "Haddonstone" } The following code will do just this ... Javascript will take all the properties on the second object and copy them to the first object. It will return the new object. That's the copy which will be assigned to objectCopy. Unlike our quick-and-dirty method, this will copy functions and objects properly. Problem is, it won't copy more than one level of the object. To shallow copy, an object means to simply create a new object with the exact same set of properties. We call the copy shallow because the properties in the target object can still hold references to those in the source object.
Reference and Copy Variables in JavaScript. In this article, we will talk about pass-by-value and pass-by-reference in JavaScript. JavaScript always passes by value, but in an array or object, the value is a reference to it, so you can 'change' the data. JavaScript has 5 primitive data types that are passed by value, they are Boolean, NULL ... 16/6/2020 · Object.assign () Method The simplest and faster way to create a shallow copy of an object is by using ES6's Object.assign (target, source1, soure2,...) method. This method copies all enumerable own properties of one or more source objects to a target object, and returns the target object: The Object.assign () method copies all enumerable own properties from one or more source objects to a target object. It returns the modified target object.
The Object.assign () method is used to copy all enumerable values from a source object to the target object. 7/3/2014 · You have to be careful when working with Javascript objects and the equals (=) operator. This operator does not create a copy of the object, but only assigns a reference to the original internal object. That means that in your case, b does not store the value (s) of a, but after calling var b = a; heroClone is a clone object of hero, meaning that it contains all the properties of hero.. hero === heroClone evalutes to false — hero and heroClone are, nevertheless, difference object instances.. 1.1 Object spread bonus: add or update cloned props. An immediate benefit of using object spread is that you can update or add new properties to the cloned object in place if you need it.
How to Copy Objects in JavaScript. Scott Robinson. Introduction. A very common task in programming, regardless of language, is to copy (or clone) an object by value, as opposed to copying by reference. The difference is that when copying by value, you then have two unrelated objects with the same value or data. Copying by reference means that ... The reason is how the javascript engine works internally: JS passes the primitive values as value-copy and the compound values as reference-copy to the value of the primitives on that Object. So, when copied the Object containing the nested Object, that will create a shallow-copy of that Object: Here's the magical series of built-in methods that can be used to create a copy of an instance of a custom-implemented class: function copyInstance (original) { var copied = Object.assign( Object.create( Object.getPrototypeOf(original) ), original ); return copied; } That's quite horrid syntax on first and second glance, but on third glance….
A JavaScript object is a complex data type that can contain various data types. For example, const person = { name: 'John', age: 21, } Here, person is an object. Now, you can't clone an object by doing something like this. const copy = person; console.log(copy); // {name: "John", age: 21} In javascript, When creating copies of arrays or objects one can make a deep copy or a shallow copy. so we will see what's deep copy and shallow copy, and how to handle this problem. Deep copy or... We can clone the object as one of the main tasks in JavaScript because it is most widely used; it takes some time consumption because the entire object property and attributes are also copied the same in the destination or target object in the script. By using this cloning concept, it will be achieved in three different ways.
As we know that there are two types of objects in Javascript, mutable and immutable and an array is a mutable object. Here is an example of what it means. originalArray = [1,2,3,4,5] clone = originalArray #Here begins the problem clone = 100 console.log(originalArray) # [100,2,3,4,5] console.log(clone) #[100, 2,3,4,5] 3 Ways to Clone Objects in JavaScript. Because objects in JavaScript are references values, you can't simply just copy using the =. But no worries, here are 3 ways for you to clone an object 👍. const food = { beef: '🥩', bacon: '🥓' } { ...food } Object.assign({}, food) JSON.parse(JSON.stringify(food)) Objects are Reference Types. 1. Cloning an object in JavaScript a task that is almost always used in any project, to clone everything from simple objects to the most complicated ones. As it may seem simple for not seasoned…
JSON.parse and JSON.stringify (Deep copy) JSON.stringify turns an object into a string. JSON.parse turns a string into an object. Combining them can turn an object into a string, and then reverse the process to create a brand new data structure. 12/3/2021 · To make a “real copy” (a clone) we can use Object.assign for the so-called “shallow copy” (nested objects are copied by reference) or a “deep cloning” function, such as _.cloneDeep (obj). JavaScript offers many ways of copying objects, but they do not provide a deep copy. Performing shallow copies is the default behavior in most of the cases. We should note that ES6 provides two shorter syntaxes for shallow copying objects in the language.
7/4/2009 · function clone(object) { /* Deep copy objects by value rather than by reference, exception: `Proxy` */ const seen = new WeakMap() return clone(object) function clone(object) { if (object !== Object(object)) return object /* —— Check if the object belongs to a primitive data type */ if (object instanceof Node) return object.cloneNode(true) /* —— Clone DOM trees */ let _object // The clone of object … In case a property inside the original object is an object, it can be shared between the copy and the original one. Notice that by nature, JavaScript objects are changeable and are stored as a reference. So, when assigning the object to another variable, you assign the memory address of the object to the variable. Almost all objects in JavaScript are instances of Object which sits on the top of the prototype chain. Introduction. As you know, the assignment operator doesn't create a copy of an object, it only assigns a reference to it, let's look at the following code:
Initialize a new object and assign it to the variable testObject. This object should have letters a, b, and c as keys and 1, 2, and 3 as values, respectively. Create the object in JavaScript: let testObject = { a: 1, b: 2, c: 3 }; Copy. Now, try to create a copy of this object to manipulate by assigning the testObject to a new variable called ... The cloneNode () method creates a copy of a node, and returns the clone. The cloneNode () method clones all attributes and their values. Tip: Use the appendChild () or insertBefore () method to insert the cloned node to the document. Tip: Set the deep parameter value to true if you want to clone all descendants (children), otherwise false. For object slice copies object references into the new array. Both the original and new array refer to the same object. If an object changes, the changes are visible to both the new and original arrays. For strings, numbers and booleans (not String, Number and Boolean objects), slice copies the values into the new array.
Cloning In Javascript Object Ta Digital Labs
Deep And Shallow Copy With Object Assign Javascript By
Copy Array By Value Stack Overflow
Shallow Copying Cloning Objects In Javascript By Serdar
Best Way To Copy An Object In Javascript Dev Community
Shallow Copy And Deep Copy In C Geeksforgeeks
Copy Shallow Copy And Deep Copy Of Objects In Javascript
How To Clone An Array In Javascript
Different Methods To Copy An Object In Javascript
3 Ways To Clone Objects In Javascript Samanthaming Com
Copy In Python Deep Copy And Shallow Copy Geeksforgeeks
Javascript How To Duplicate An Array
Ways To Clone An Object In Javascript By Tran Son Hoang
I Would Simply Use Recursion For A Deep Copy Function
Different Methods To Copy An Object In Javascript Codementor
What Is Shallow Copy And Deep Copy In Javascript
Dan On Twitter Define Copy Semantics Matter
Javascript Clone Understand Shallow And Deep Copy Examples
What Is The Most Efficient Way To Deep Clone An Object In
3 Ways To Clone Objects In Javascript Samanthaming Com
Javascript Object Get A Copy Of The Object Where The Keys
A Deep Dive Into Shallow Copy And Deep Copy In Javascript
Different Methods To Copy An Object In Javascript
Deep Copy Object Oriented Javascript Third Edition
Create A Copy Of An Object In Javascript
Copying Javascript Objects In An Efficient Way Dev Community
How Do I Correctly Clone A Javascript Object Stack Overflow
How To Clone Object In Javascript Example Tutorial
0 Response to "29 Javascript Copy Of Object"
Post a Comment