35 Javascript Array Index Exists
The indexOf() method returns the first index at which a given element can be found in an array. It returns -1 if the element does not exist in the array. Let's go back to our example. Let's find the index of 3 in the array. const array = [10, 11, 3, 20, 5]; const indexOfThree = array.indexOf(3); console.log(indexOfThree)//2 We are going to check for a value's existence in an array in 2 different ways using jQuery and Javascript ... If you are someone strongly committed to using the jQuery library, you can use the .inArray( ) method. If the function finds the value, it returns the index position of the value and ...
Javascript Array Splice Delete Insert And Replace
JavaScript provides us an alternate array method called lastIndexOf (). As the name suggests, it returns the position of the last occurrence of the items in an array. The lastIndexOf () starts searching the array from the end and stops at the beginning of the array. You can also specify a second parameter to exclude items at the end.
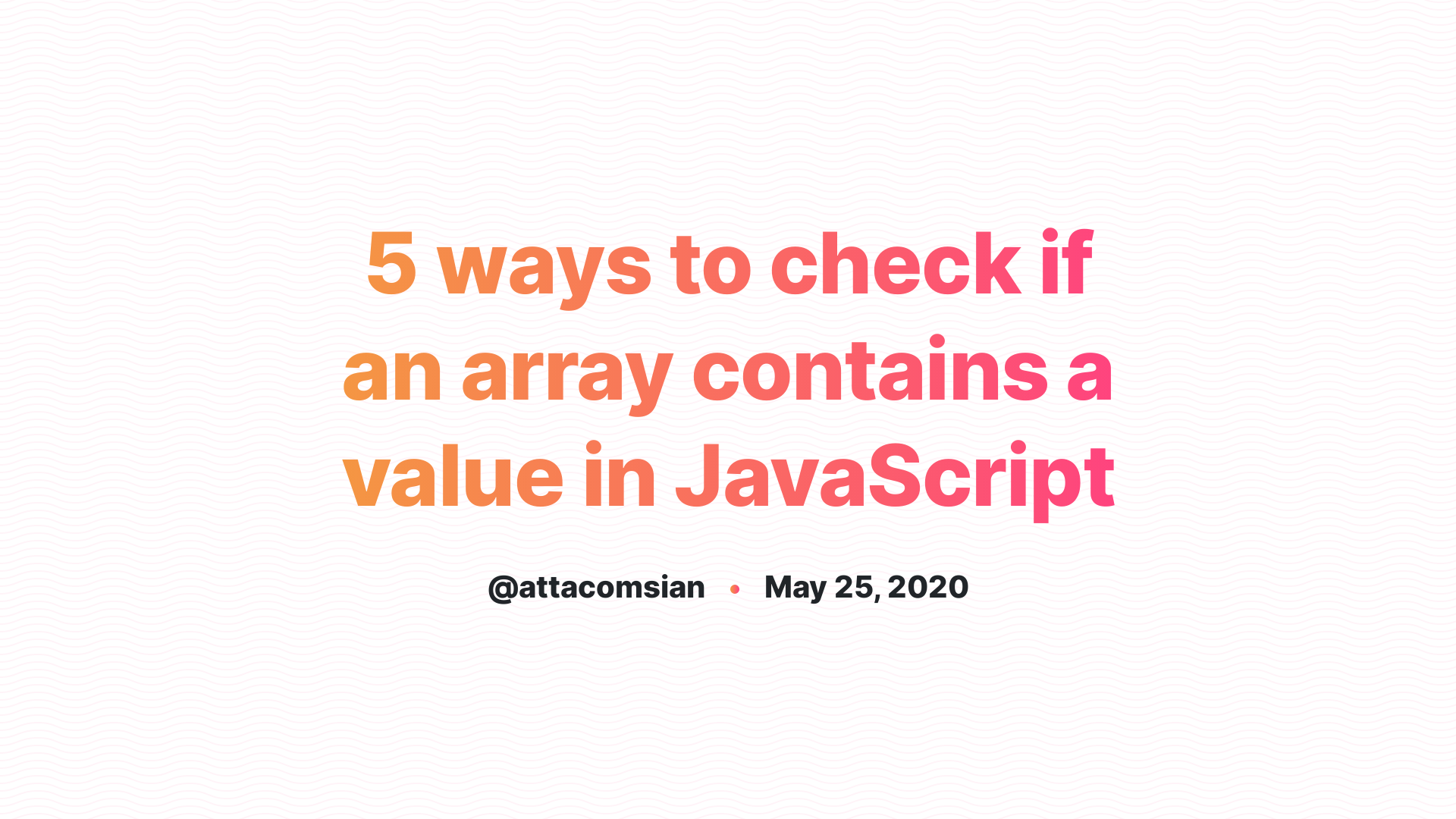
Javascript array index exists. array_key_exists() returns TRUE if the given key is set in the array. key can be any value possible for an array index. 17/2/2020 · Viewed 62k times. 21. I'm trying to check whether an array index exist in TypeScript, by the following way (Just for example): var someArray = []; // Fill the array with data if ("index" in someArray) { // Do something } However, i'm getting the following compilation error: This array method helps us to find out the item in the array in JavaScript. If element exists in the array it returns the index position of the value and if the value doesn't exist then it returns -1.
3 weeks ago - This array method helps us to find out the item in the array in JavaScript. If element exists in the array it returns the index position of the value and if the value doesn’t exist then it returns -1. It works with both string and an array in JavaScript. Get index of an element within an array. Check if value exists in array. Check if it exists with includes. Conclusion. Introduction: Sometimes you need to find the position of a certain element, or maybe just check if it exists within an array, array, vector, or list. In other words, we will see how to check if an element is present . Recently ... When you have an array of the elements, and you need to check whether the certain value exists or not. Solution 1: includes (): We can use an includes (), which is an array method and return a boolean value either true or false. If the value exists returns true otherwise it returns false. Let's write an example of the same.
ajax angular angularjs api arrays asynchronous axios css d3.js discord discord.js dom dom-events ecmascript-6 express firebase forms function google-apps-script google-chrome google-cloud-firestore google-sheets html javascript jestjs jquery json mongodb mongoose node.js object php promise python react-hooks react-native react-router reactjs ... 1 week ago - The indexOf() method returns the first index at which a given element can be found in the array, or -1 if it is not present. Check if the elements from the first array exist in the object or not. If it doesn't exist then assign properties === elements in the array. Loop through second array and check if elements in the second array exists on created object. If element exist then return true else return false.
See the Pen JavaScript - Find to if an array contains a specific element- array-ex- 32 by w3resource (@w3resource) on CodePen. Contribute your code and comments through Disqus. Previous: Write a JavaScript function to remove a specific element from an array. Next: Write a JavaScript script to empty an array keeping the original. JavaScript Array Contains: A Step-By-Step Guide. The JavaScript includes () method searches an array for an item. This method returns True if the element in the array exists. The filter () method lets you find an item in a list. Unlike includes (), the filter () method returns the item for which you have been searching. The Array.prototype.findIndex () method returns an index in the array if an element in the array satisfies the provided testing function; otherwise, it will return -1, which indicates that no element passed the test. It executes the callback function once for every index in the array until it finds the one where callback returns true.
Dec 07, 2020 - In this tutorial, we'll go over examples of how to check if an array includes/contains an element or value in JavaScript. An array in JavaScript is a type of global object that is used to store data. Arrays consist of an ordered collection or list containing zero or more datatypes, and use numbered indices starting from 0 to access specific items. Aug 21, 2020 - How to determine if a JavaScript array contains a particular value, being a primitive or object.
Array.indexOf and Array.findIndex are similar because they both return the index of the first matching element found in our Array, returning us -1 if it's not found. To check if an element exists, we simply need to check if the returned value is -1 or not. Code Recipe to check if an array includes a value in JavaScript using ES6 Definition and Usage. The includes () method returns true if an array contains a specified element, otherwise false. includes () is case sensitive.
Returns the first (least) index of an element within the array equal to an element, or -1 if none is found. Array.prototype.join() Joins all elements of an array into a string. Array.prototype.keys() Returns a new Array Iterator that contains the keys for each index in the array. Array.prototype.lastIndexOf() Returns the last (greatest) index ... Array.find. The Array.find() method returns the value of the first element in an array that passes a given test.There are a few rules: Test must be provided as a function. find() method executes a callback function once for each element in the array until it finds a value that returns true. If nothing passes, undefined is returned. find() does not mutate or change the original Array. You can use the indexOf () method to check whether a given value or element exists in an array or not. The indexOf () method returns the index of the element inside the array if it is found, and returns -1 if it not found. Let's take a look at the following example:
Jul 29, 2016 - How do I check if a numerical index exists in the javascript array? ... How could an index not exist in an array? What you mean is it's value is undefined, right? – acdcjunior Jun 21 '13 at 22:49 Given an array arr of integers, check if there exists two Input ... Write a function that checks whether a person can watch an MA15+ rated movie javascript One of the following two conditions is required for admittance: Definition and Usage. The indexOf () method searches an array for a specified item and returns its position. The search will start at the specified position (at 0 if no start position is specified), and end the search at the end of the array. indexOf () returns -1 if the item is not found.
/** * JavaScript function that checks to see whether an array * contains a certain value. * @param arr The array you want to search. * @param searchFor The value you want to search for. * @returns {boolean} TRUE if the value exists in the array. FALSE otherwise. Example 1 returns true if an element exists in a 2d array, otherwise false Example 2 returns the 2d array index i.e. [row, col] if found, otherwise false. Problem: indexOf() doesn't work for 2-d Javascript arrays. Here's the one-liner solution. There are a lot of solutions out there that loop through a 2d array but that's not necessary. The find () method is an Array.prototype (aka built-in) method which takes in a callback function and calls that function for every item it iterates over inside of the array it is bound to. When it finds a match (in other words, the callback function returns true ), the method returns that particular array item and immediately breaks the loop.
The includes () method returns either a true or a false if a value exists in an array or not. This is the basic syntax: arr.includes(valueToFind [, fromIndex]); The first parameter, valueToFind, is the value to match in the array. Aug 07, 2020 - Get code examples like "javascript check array index if exists" instantly right from your google search results with the Grepper Chrome Extension. For checking if an object exists in an array, we need to use the indexOf method on the array. If the object is not found, -1 is returned, else its index is returned.
The call to new Array(number) creates an array with the given length, but without elements. The length property is the array length or, to be precise, its last numeric index plus one. It is auto-adjusted by array methods. If we shorten length manually, the array is truncated. We can use an array as a deque with the following operations: Apr 16, 2020 - Search Options · Search Answer Titles · Search Code · browse snippets » · Loading · Hmm, looks like we don’t have any results for this search term. Try searching for a related term below · or Browse Code Snippets · Related Searches · Grepper · Features Reviews Code Answers Search ... That is, JavaScript arrays are linear, starting with zero and going to a maximum, and arrays don't have a mechanism for excluding certain values or ranges from the array. To find out if a value exists at a given position index (where index is 0 or a positive integer), you literally just use
Array.prototype.some () The some () method tests whether at least one element in the array passes the test implemented by the provided function. It returns true if, in the array, it finds an element for which the provided function returns true; otherwise it returns false. It doesn't modify the array. Jun 04, 2021 - In this tutorial, I show How you can check whether an Array already contains a specific value or not. This requires within the program in some cases like - Stop new value from insert if it already exists in an Array, execute script when the Array contains the particular value, etc.. by Rohit JavaScript array includes is used to check an element is exists in the array or not. It's an inbuilt function and returns true if the element is present in Array.
An array through which to search. ... The index of the array at which to begin the search. The default is 0, which will search the whole array. The indexof () method in Javascript is one of the most convenient ways to find out whether a value exists in an array or not. The indexof () method works on the phenomenon of index numbers. This method returns the index of the array if found and returns -1 otherwise. Let's consider the below code: Mar 14, 2016 - The OP was looking to see if the given index number exists. This is checking if a given value exists. ... This also works for undefined and null values in the array, which none of the other answers here do.
This post will discuss how to determine whether an element exists at the specified index in an array in JavaScript. An array in JavaScript can store elements of any type such as number, string, boolean, bigint, null, undefined, symbol, or an object. 1. Check for undefined value. An undefined value automatically gets assigned in JavaScript, where no value has been explicitly assigned. Close your apps when you're not using them. Never prop up your notebook on a pillow. These and six more easy tips will help you squeeze longer battery life out of your Windows 10 or Mac laptop · Whether you're looking for a connected light bulb, security camera, or thermostat, start with the ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
How To Check A Key Exists In Javascript Object Geeksforgeeks
Working With Arrays In Javascript I Adding Removing
Java Exercises Find The Index Of An Array Element W3resource
Check If Element Exists In Array Javascript Indexof
5 Ways To Check If An Array Contains A Value In Javascript
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
How To Check If An Element Exists In Selenium Testim Blog
Check If Value Exists In Array Jquery And Javascript
Find The Next Greater Element For Every Element In An Array
Modifying Json Data Using Json Modify In Sql Server
The Firebase Blog Better Arrays In Cloud Firestore
How To Check If An Element Exists In Selenium Testim Blog
Php Check If Array Key Exists In Another Array
Binary Search In Javascript Dev Community
Algodaily Array Intersection Description
Arraylist In Java With Example Programs Collections Framework
Visualize Embedded Objects And Arrays Mongodb Charts
How To Check If An Element Exists In Selenium Testim Blog
How To Get The Index Of An Item In A Javascript Array
Remove Element From Array Using Slice Stack Overflow
How Do I Check If An Array Includes A Value In Javascript
Js Add To Array If Element Exists Remove Code Example
How Do I Check In Javascript If A Value Exists At A Certain
Javascript Check If Element Exists In Array Of Objects
How To Check If Array Includes A Value In Javascript
How To Check An Element With Specific Id Exists Using
Arrays In Javascript How To Create Arrays In Javascript
Javascript Array Contains How To Use Array Includes Function
Javascript Array Findindex How To Find First Element Index
Negative Indexes In Javascript Arrays Using Proxies By
The Beginner S Guide To Javascript Array With Examples
Check Element Exists In A Slice Golangcode
0 Response to "35 Javascript Array Index Exists"
Post a Comment